In C++, the newline character can be represented using `\n` within strings, which causes the cursor to move to the next line in the output.
#include <iostream>
int main() {
std::cout << "Hello, World!\nWelcome to C++!" << std::endl;
return 0;
}
Understanding Newline in C++
What is a Newline?
A newline character is a special character that indicates the end of a line of text. In C++, the newline character serves to enhance readability by organizing output into distinct lines. This is crucial for ensuring that the program's output is easily interpretable by users and developers alike.
Types of Newline Characters
LF (Line Feed)
Commonly used in UNIX/Linux systems, the Line Feed character marks the transition to a new line without returning the cursor to the beginning.
CR (Carriage Return)
Historically, the Carriage Return character moves the cursor back to the start of the line, without advancing down to the next line. It was used in older printer mechanisms.
CRLF (Carriage Return Line Feed)
This combination of CR and LF characters is the standard for newlines in Windows systems. It first returns the cursor to the beginning of the line and then moves it down to the next line.
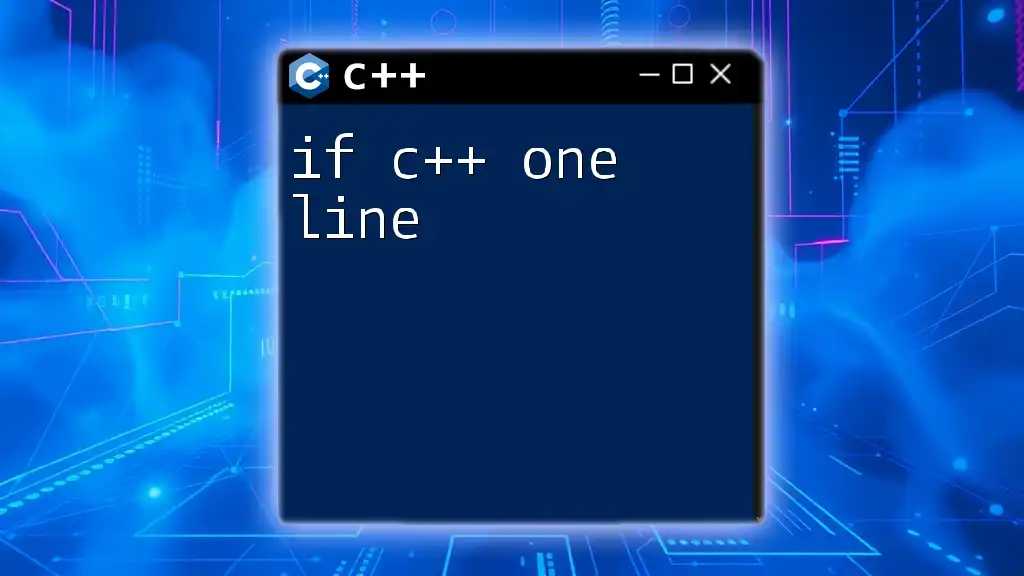
Using Newline in C++
The `\n` Character
The escape sequence `\n` is the most straightforward way to create a newline in C++. It appends a newline character to the output stream, allowing the rest of the output to appear on the succeeding line.
Example:
#include <iostream>
int main() {
std::cout << "Hello, World!\n";
return 0;
}
In this example, the output will display "Hello, World!" followed by a newline, which means any subsequent output will begin on a new line. Use `\n` when you prefer a lightweight way to introduce newlines without flushing the output buffer.
Using `std::endl`
Another method to insert newlines is by using `std::endl`. This not only adds a newline but also forces the output buffer to flush, ensuring that all output preceding it is displayed immediately.
Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::endl` performs functionally similar to `\n` but with the additional benefit of flushing the output. However, keep in mind that excessive use of `std::endl` can lead to performance issues, especially in loops, due to the constant flushing of the output buffer.
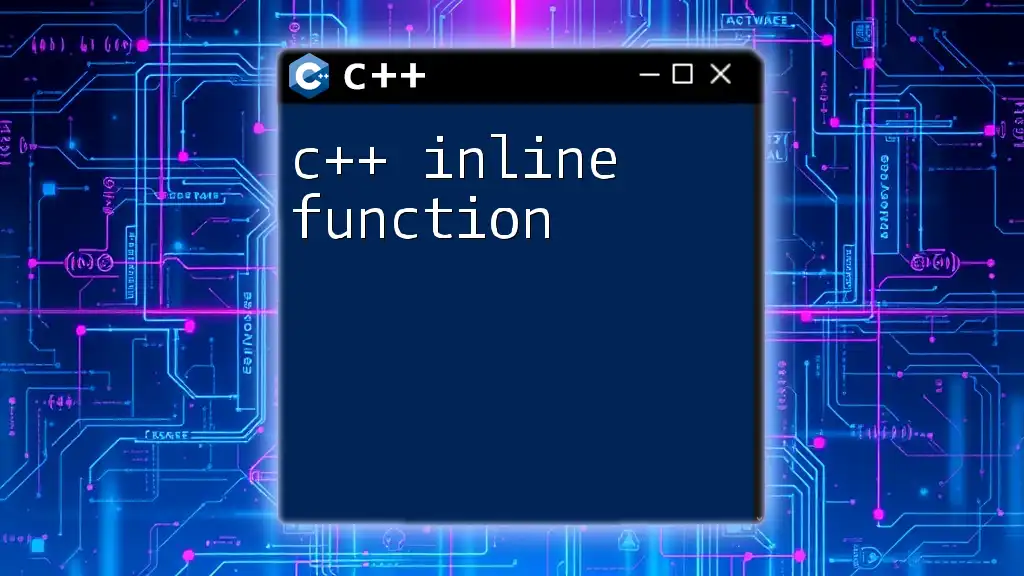
Best Practices for Newline Usage
Formatting Output
Effective output formatting enhances the user experience. Well-structured outputs can significantly improve clarity and usability of the program.
Example:
#include <iostream>
int main() {
std::cout << "Line 1\nLine 2\nLine 3" << std::endl;
return 0;
}
Here, multiple outputs on separate lines allow users to quickly grasp the information presented. Using newlines strategically can turn a cluttered output into a structured and readable format.
Readability and Debugging
Newlines are key for improving both readability and ease of debugging. By separating output clearly, you create a more understandable workflow.
Example:
#include <iostream>
int main() {
int x = 10, y = 20;
std::cout << "Values:\n"
<< "X: " << x << "\n"
<< "Y: " << y << std::endl;
return 0;
}
In this output, the use of newlines aids developers in quickly identifying the variable values. This structured output is essential during debugging and helps communicate the program's state.
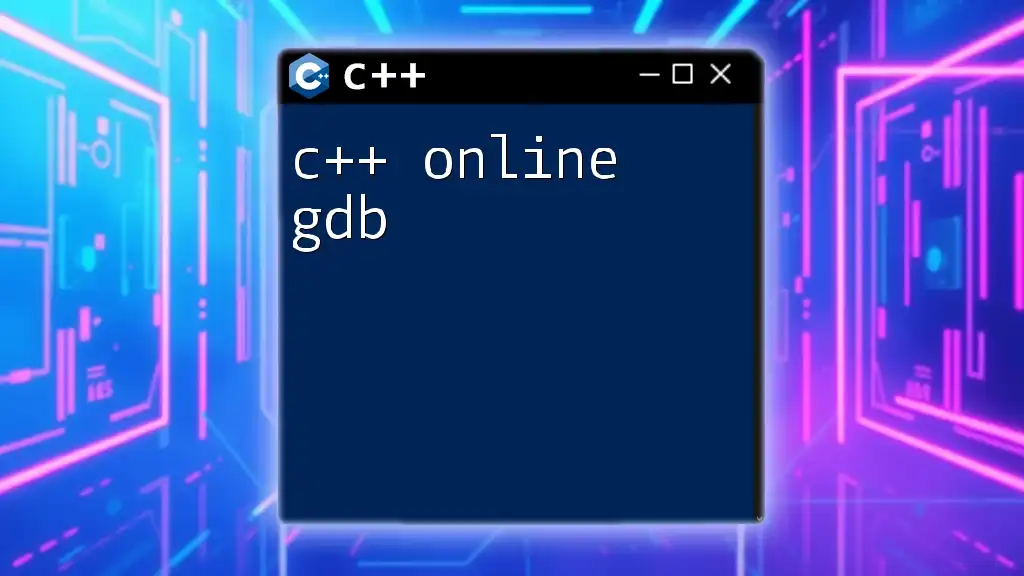
Common Mistakes with Newline in C++
Forgetting to Use Newline
Neglecting to use newlines can lead to compressed output that is difficult to read.
Example:
#include <iostream>
int main() {
std::cout << "Hello, World!"; // No newline, hard to read
// Output: Hello, World! (No line break)
return 0;
}
In this situation, the absence of a newline can create confusion, especially in longer outputs where the information merges. Always ensure to use newlines when appropriate.
Confusing Newline Characters
Understanding newline characters, especially the differences between platforms, is vital. Using `\n` consistently can often lead to unexpected results when running code on different operating systems.
Consider cases where newline characters may not render properly, depending on the platform's expectations. Employing methods, like utilizing the C++ standard library, can help to ensure more consistent behavior across platforms.
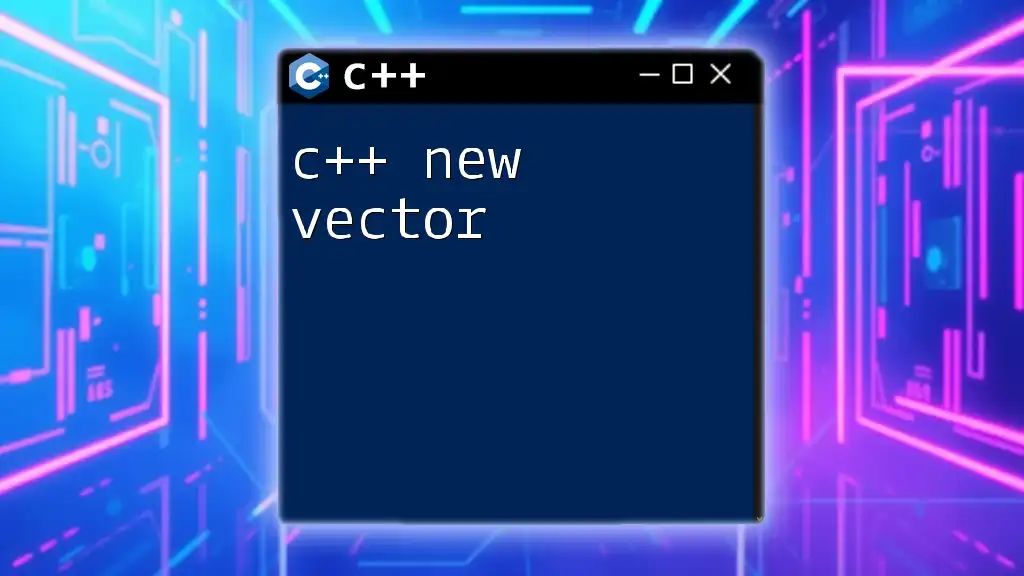
Conclusion
The role of newline characters in C++ is fundamental for both output structure and clarity. Properly using newline characters enhances user engagement with program output and fosters good coding practices. As you experiment and implement newline usage in your C++ projects, take heed of when to prefer `\n` or `std::endl`.
By embracing the importance of newline functionality, you can significantly enhance the quality and readability of your code. Happy coding as you explore and master the effective use of c++ newline commands!