C++ linear algebra involves using C++ libraries and functionalities to perform mathematical operations on vectors and matrices efficiently.
Here is a simple code snippet demonstrating basic operations with vectors using the Eigen library:
#include <Eigen/Dense>
#include <iostream>
int main() {
Eigen::Vector3d v1(1.0, 2.0, 3.0);
Eigen::Vector3d v2(4.0, 5.0, 6.0);
Eigen::Vector3d v3 = v1 + v2; // Vector addition
std::cout << "v1 + v2 = " << v3.transpose() << std::endl; // Output: (5, 7, 9)
return 0;
}
Understanding Vectors in C++
Definition of Vectors
In linear algebra, a vector is a mathematical object that has both a magnitude and a direction. Vectors can be represented with coordinates in multi-dimensional space, which makes them foundational to various fields such as physics, engineering, and computer science.
They can be classified into different types based on their dimensions:
- Row vectors consist of a single row of elements.
- Column vectors consist of a single column of elements.
Understanding how to work with vectors in C++ is essential for implementing linear algebra concepts efficiently in your applications.
Using Vectors in C++
To get started with vectors in C++, you can use the Standard Template Library (STL). Here’s how you can declare and initialize a vector:
#include <vector>
std::vector<int> vec = {1, 2, 3, 4, 5}; // Definition of a vector
Vectors support a variety of operations. Let’s explore some fundamental vector operations like addition, subtraction, and scaling:
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
// Vector addition
for(size_t i = 0; i < vec1.size(); ++i) {
vec1[i] += vec2[i];
}
This example illustrates how to add two vectors element-wise. Similarly, you can perform other operations such as scaling by multiplying each element by a scalar.
Real-World Application: Vectors are widely used in data science for representing data points and in graphics programming for defining positions and movements in space.
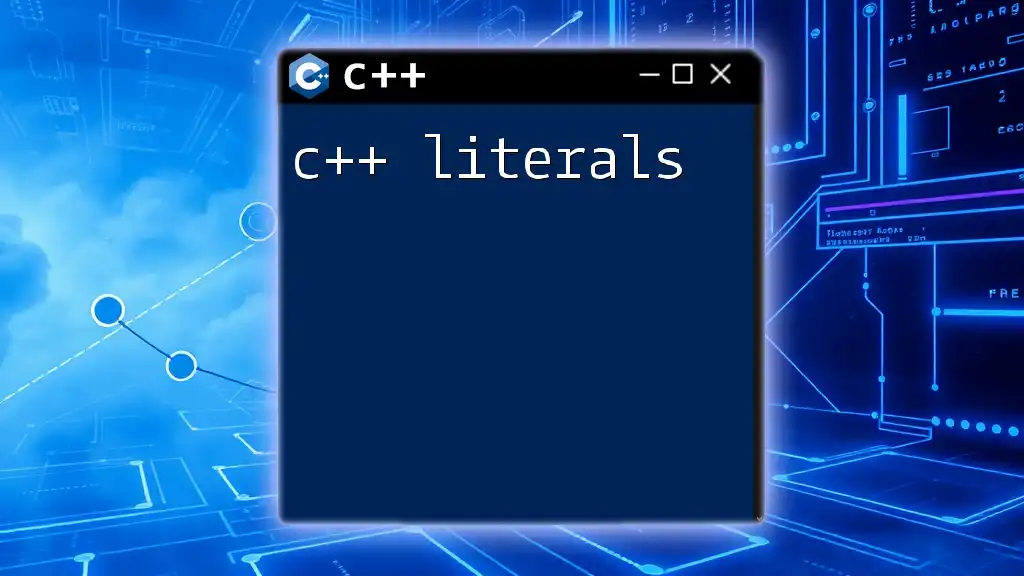
Matrices in C++
Introduction to Matrices
A matrix is a rectangular array of numbers arranged in rows and columns. Matrices are key components in linear algebra and are used for a variety of applications, including systems of equations, computer graphics, and machine learning.
Matrices are classified into several types:
- Row Matrices have only one row.
- Column Matrices have only one column.
- Square Matrices have an equal number of rows and columns.
Creating and Manipulating Matrices
In C++, you can represent matrices effectively using 2D vectors. Here’s an example of how to create and initialize a matrix:
#include <vector>
std::vector<std::vector<int>> matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Matrices also allow for operations like addition, subtraction, and scalar multiplication. Here’s how you can perform a simple scalar addition on a matrix:
// Matrix addition example
for(int i = 0; i < matrix.size(); ++i) {
for(int j = 0; j < matrix[i].size(); ++j) {
matrix[i][j] += 1; // Scalar Addition
}
}
Application in Real Life: Matrices play a crucial role in image processing, where each pixel can be represented as an element in a matrix.
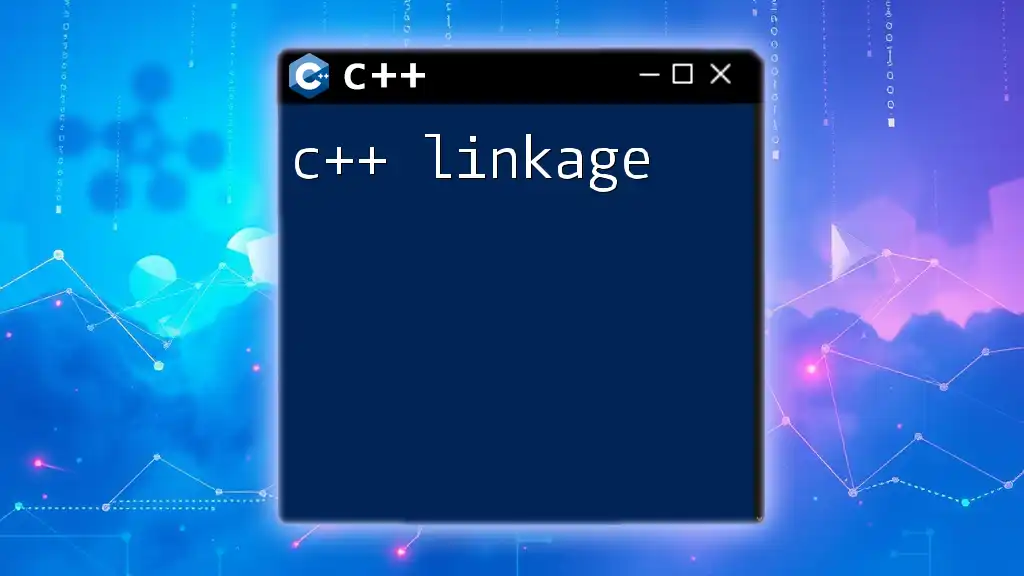
C++ Linear Algebra Libraries
Overview of Popular Libraries
When working on advanced linear algebra operations in C++, leveraging dedicated libraries can greatly streamline your coding process. Some well-known libraries include:
- Armadillo: A high-quality C++ linear algebra library that provides a straightforward interface and high performance.
- Eigen: It is highly regarded for its simplicity and speed, making it ideal for applications needing matrix and vector algebra.
- Blaze: This library focuses on high-performance linear algebra and is designed for modern C++.
- LAPACK: Although more complex, LAPACK is a powerful library for solving linear algebra problems, particularly for large matrices.
Installing and Setting Up a C++ Linear Algebra Library
Choosing the right linear algebra library can greatly enhance your development experience. For beginners, Eigen is often recommended due to its ease of integration.
To install Eigen, you can use the following command:
# Instructions for installing Eigen
sudo apt-get install libeigen3-dev
Once installed, you're ready to utilize it in your projects. Here’s an example demonstrating basic vector and matrix operations using Eigen:
#include <Eigen/Dense>
using namespace Eigen;
int main() {
Vector3d v(1, 2, 3);
Matrix3d m;
m << 1, 2, 3,
4, 5, 6,
7, 8, 9;
Vector3d result = m * v;
// Output result
}
This code snippet shows how to create a vector and a matrix, then multiply them together, showcasing how easy it is to perform complex operations with a library designed specifically for linear algebra.
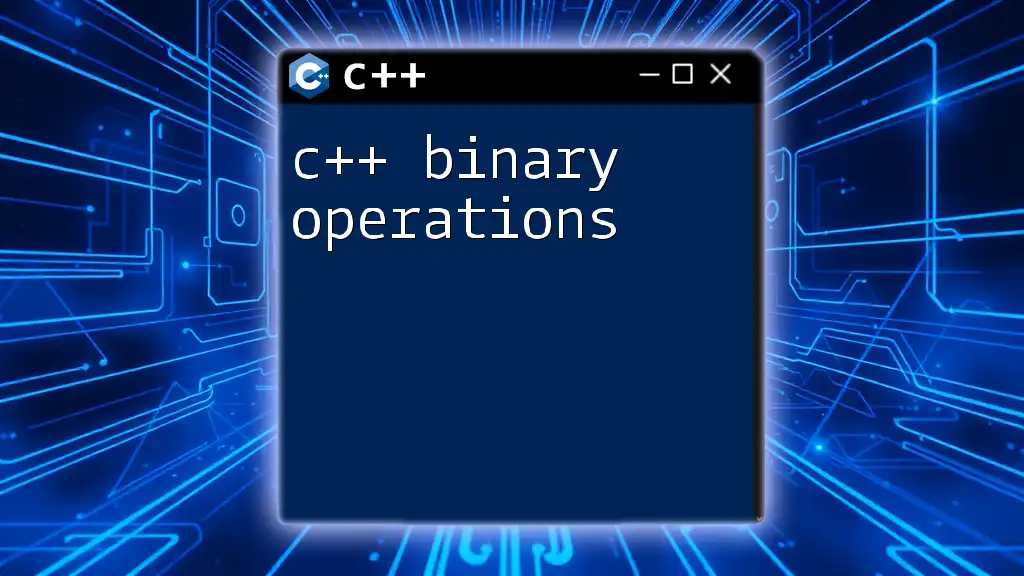
Applications of Linear Algebra in C++
Linear Transformations
Linear transformations are mappings that transform vectors while preserving operations like vector addition and scalar multiplication. In practical applications, these transformations can be represented as matrix multiplication.
Solving Systems of Linear Equations
Linear algebra is heavily utilized in solving systems of equations, which can be expressed in the form Ax = b. Here, A is the matrix of coefficients, x is the vector of unknowns, and b is the vector of results. You can efficiently solve such systems using C++ libraries:
#include <Eigen/Dense>
MatrixXd A(2, 2);
A << 1, 2, 3, 4;
VectorXd b(2);
b << 5, 6;
VectorXd x = A.colPivHouseholderQr().solve(b); // Solve Ax = b
Here, the use of Eigen’s `colPivHouseholderQr` method allows for a robust solution to the system.
Graphics and Game Development
In graphics programming, linear algebra is foundational for transformations, lighting calculations, and rendering. Using matrices and vectors, you can efficiently perform tasks such as rotation, scaling, and translation of graphical objects.
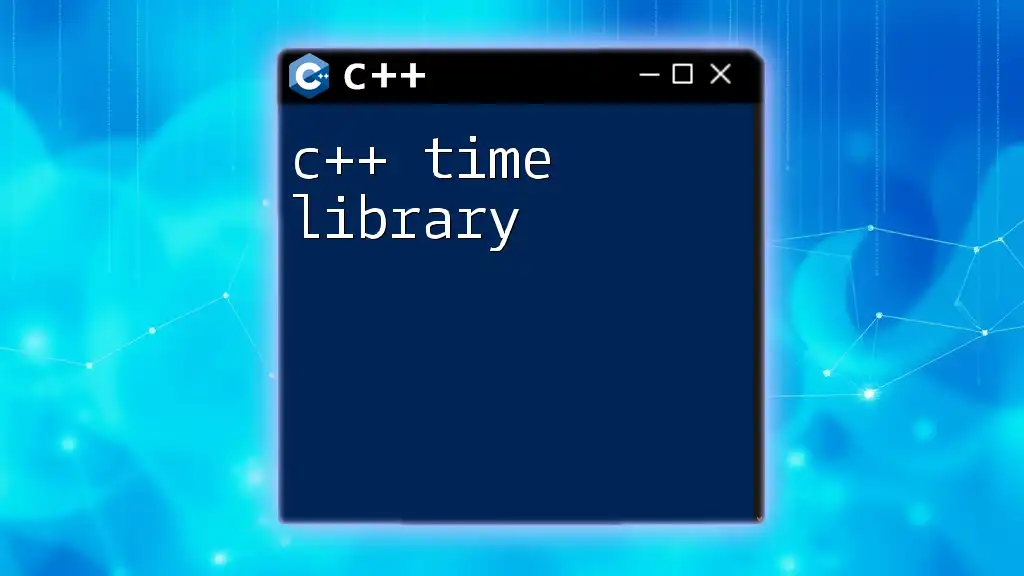
Conclusion
In summary, understanding C++ linear algebra opens the door to numerous applications in technology, science, and engineering. By mastering vectors and matrices, and exploring powerful libraries like Eigen, you can effectively implement linear algebra challenges in your projects.
Continue to explore this field, as the skills acquired can be invaluable across various domains.
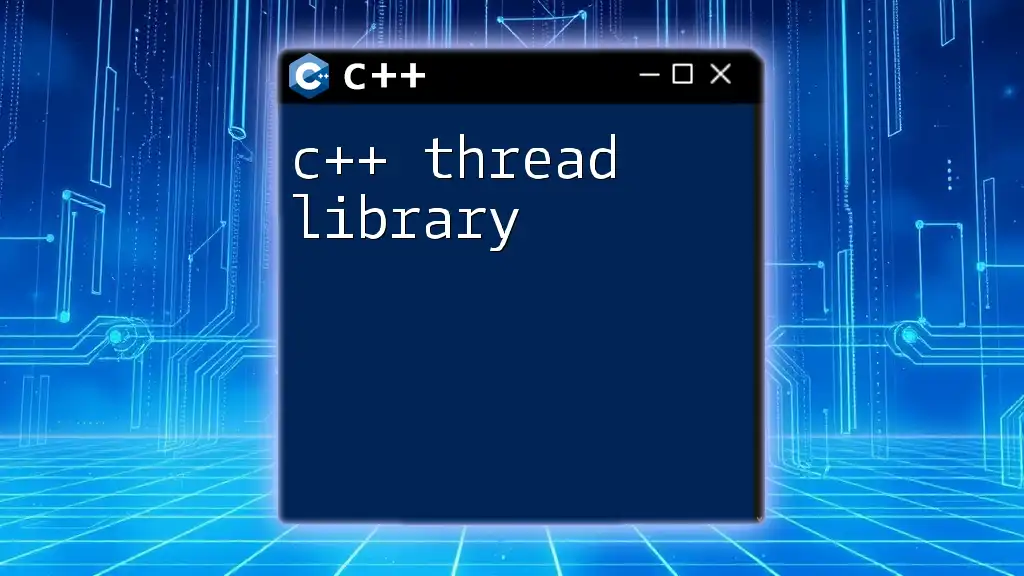
Additional Resources
For further reading and exploration, consider looking into recommended books, online courses, and community forums dedicated to C++ linear algebra. These resources can provide additional insights and aid in your journey to becoming proficient in C++.
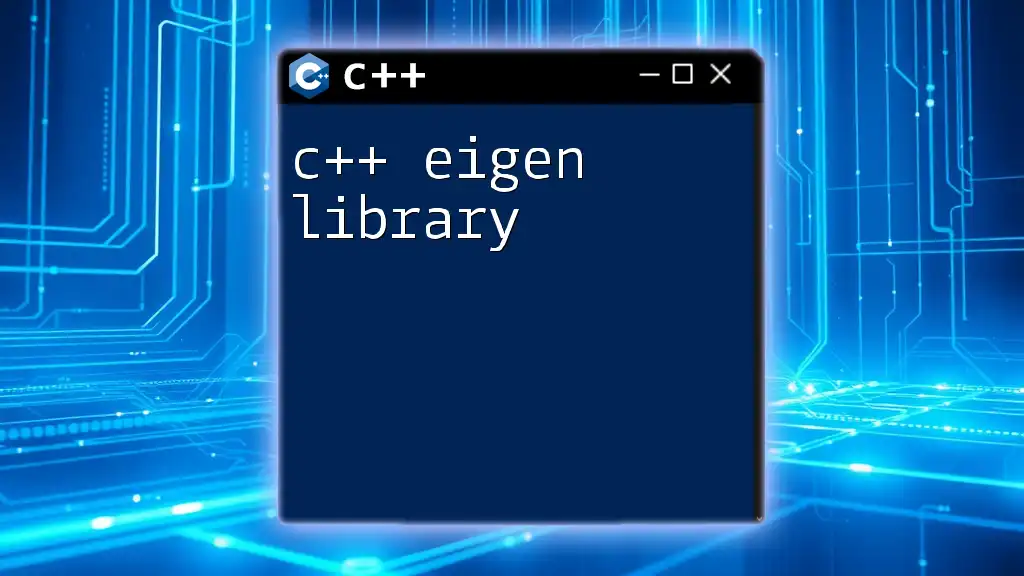
Call to Action
We encourage you to share your experiences and feedback regarding C++ and linear algebra. Join our community to gain more insights and learn quick, concise lessons on C++ commands!