C++ linkage refers to the visibility of variables and functions across different translation units, influencing how they can be accessed or overridden during the linking phase of compilation.
Here’s a code snippet demonstrating external linkage:
// file1.cpp
#include <iostream>
int globalVariable = 42; // External linkage
void display() {
std::cout << "Global Variable: " << globalVariable << std::endl;
}
// file2.cpp
extern int globalVariable; // Declaration with external linkage
void anotherFunction() {
std::cout << "Accessing Global Variable: " << globalVariable << std::endl;
}
What is Linkage?
Linkage in C++ defines the visibility of variables and functions across different translation units. Simply put, it determines how names can be resolved and accessed across files. Understanding linkage is crucial for managing scope and avoiding naming conflicts in larger programs.
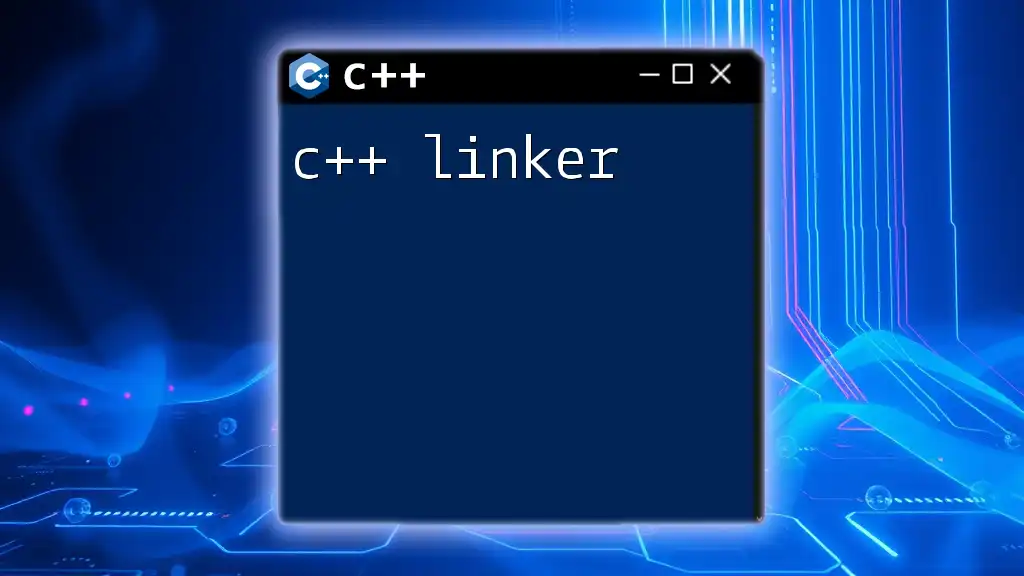
Types of Linkage
External Linkage
Variables or functions with external linkage can be referenced from other translation units. By default, global variables and functions have external linkage, enabling them to be utilized across multiple files.
Code Snippet:
// external_linkage.cpp
#include <iostream>
int globalVariable = 10; // This variable has external linkage
void display() {
std::cout << "Global Variable: " << globalVariable << std::endl;
}
In the code above, `globalVariable` can be accessed in other files by declaring it as `extern`. This approach aids in modular programming.
Internal Linkage
In contrast to external linkage, internal linkage restricts a variable or function's accessibility to the file in which it is defined. This is often accomplished using the `static` keyword.
Code Snippet:
// internal_linkage.cpp
#include <iostream>
static int internalVariable = 5; // This variable has internal linkage
void show() {
std::cout << "Internal Variable: " << internalVariable << std::endl;
}
Here, `internalVariable` cannot be accessed from outside its defining file, ensuring data encapsulation and protecting against accidental modifications.
No Linkage
Variables or functions declared inside a block (like within a function) have no linkage, meaning they are local to that block and cannot be accessed elsewhere.
Code Snippet:
// no_linkage.cpp
void function() {
int localVariable = 3; // This variable has no linkage
}
In this example, `localVariable` is confined to the `function` scope, demonstrating how local variables function in C++.
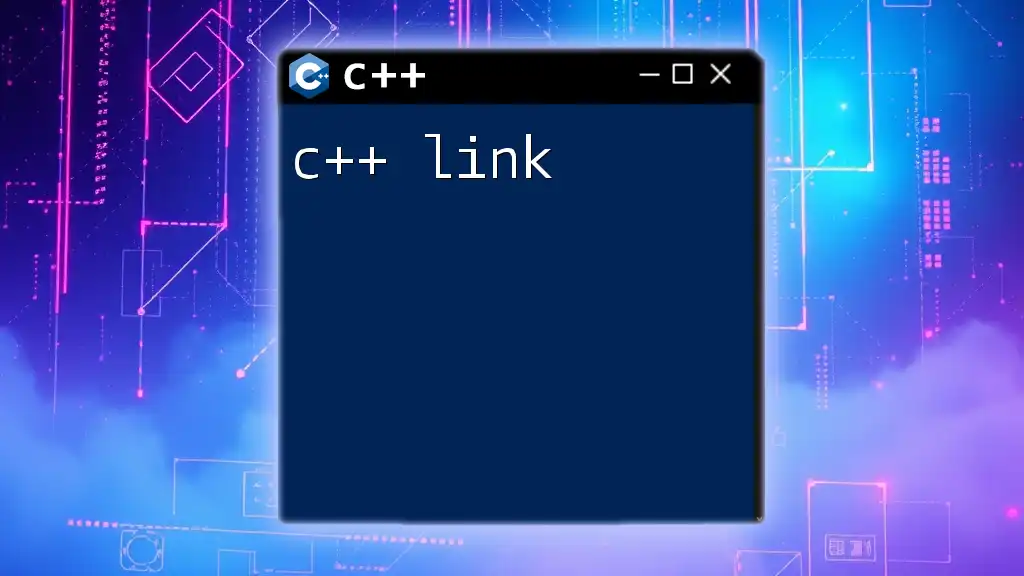
Storage Duration and Linkage
Static Storage Duration
Static storage duration refers to the lifetime of a variable that persists throughout the program's execution. While linkage can control visibility, the `static` storage duration can be used with both internal and external linkage.
Code Snippet:
// static_duration.cpp
#include <iostream>
static int staticVar = 100; // Static storage duration, internal linkage
void printStaticVar() {
std::cout << "Static Variable: " << staticVar << std::endl;
}
This ensures `staticVar` retains its value throughout the program but prevents external access.
Thread Storage Duration
Variables with thread storage duration are tied to the lifetime of a thread. They allow data to be accessed safely among threads. The `thread_local` keyword defines these variables, granting them unique instances for each thread while deciding on their linkage.
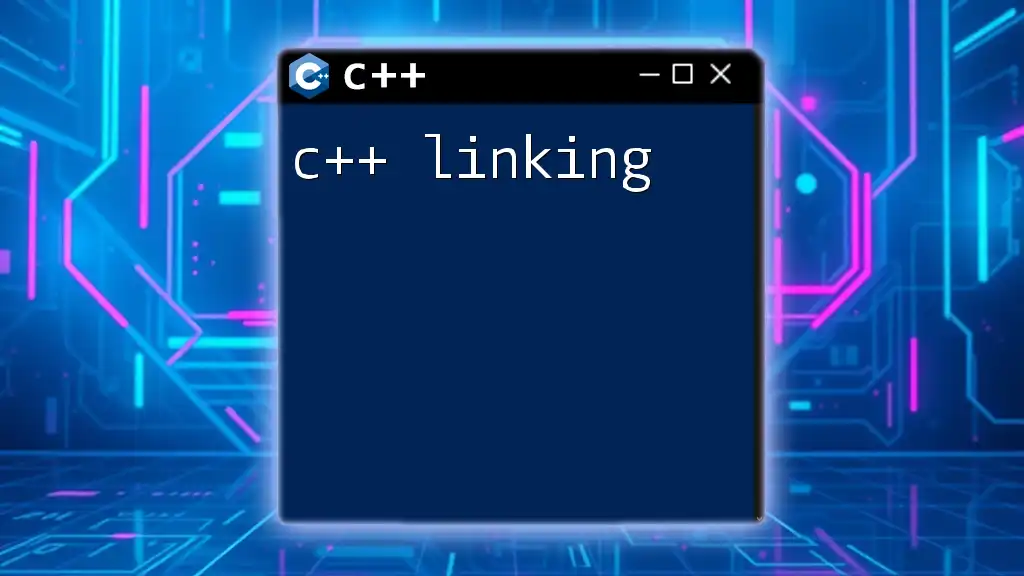
Function Linkage
Default Function Linkage Types
Functions declared outside of any class or block scope have external linkage by default. This means they can be accessed from any other translation unit, as long as properly defined.
Code Snippet:
#include <iostream>
void greet() {
std::cout << "Hello, welcome to C++!" << std::endl;
}
This function can be called from other files, demonstrating default linkage behavior.
Linkage of Function Overloading
In C++, functions can be overloaded, allowing multiple functions to share the same name with different parameters. Each overload maintains its linkage but requires unique signatures for proper resolution during linkage.
Code Snippet:
void print(int x);
void print(double y); // Function overloading with external linkage
Both functions can be called independently based on their argument types, leveraging external linkage to facilitate cleaner code.
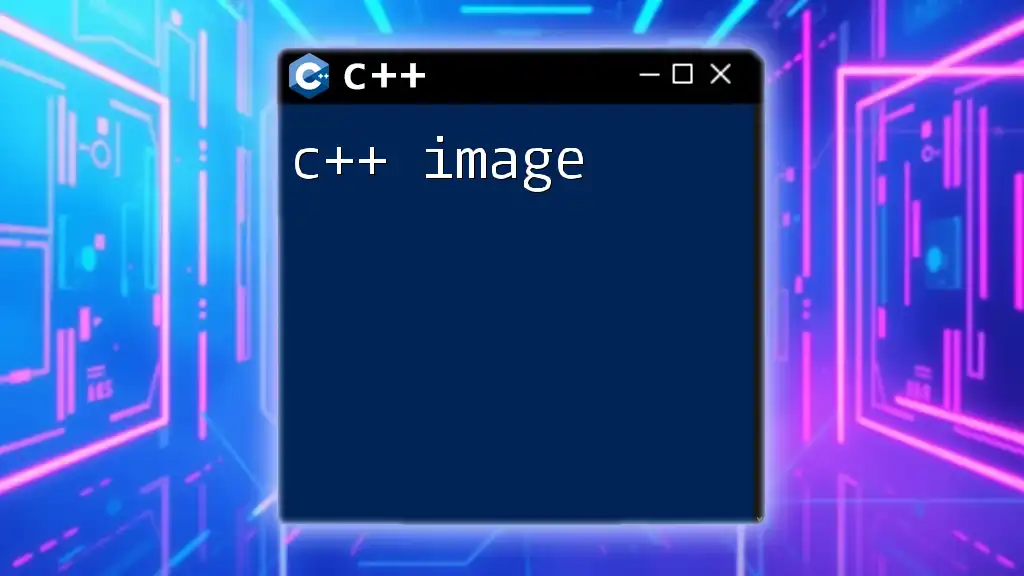
Variable Linkage
Global and Local Variables
Global variables possess external linkage, enabling them to be accessed freely across various files. In contrast, local variables, confined to their function scope, do not have linkage.
Const and Linkage
Constants in C++ also have external linkage by default unless specified otherwise. This behavior can become crucial when managing shared constants across files.
Code Snippet:
const int constVar = 20; // This constant has external linkage
In this example, `constVar` can also be referenced from other translation units, illustrating how linkage for constants operates in C++.
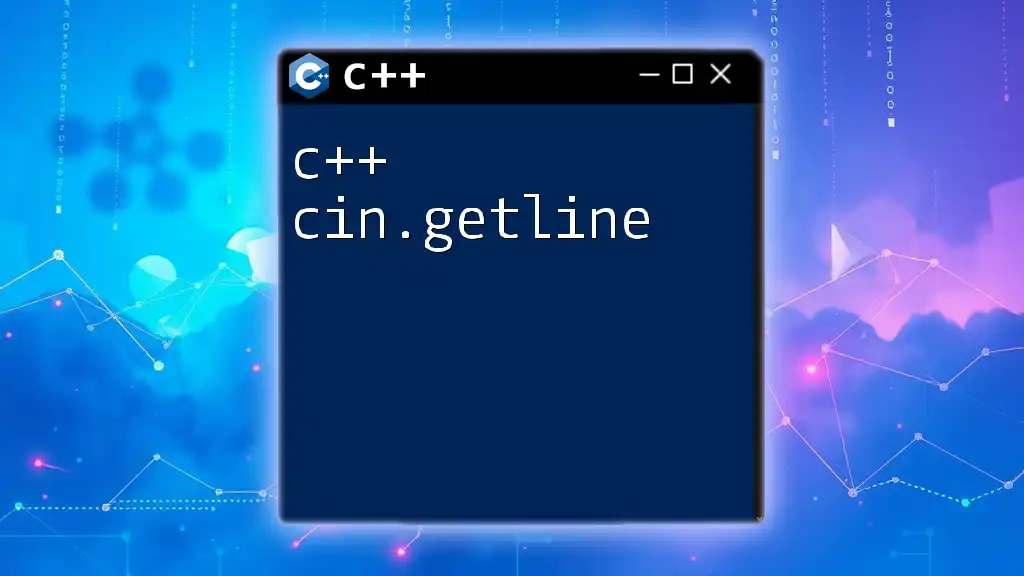
Namespaces and Linkage
Effect of Namespaces on Linkage
Namespaces regulate identifier scope, allowing for organized code while avoiding name clashes. Variables declared within a namespace still retain their linkage type, but their accessibility is limited to that scope.
Code Snippet:
namespace Example {
int namespaceVar = 30; // This variable has external linkage within the Example namespace
}
Here, `namespaceVar` can be accessed using the `Example::` qualifier, emphasizing the importance of namespaces in organizing code.
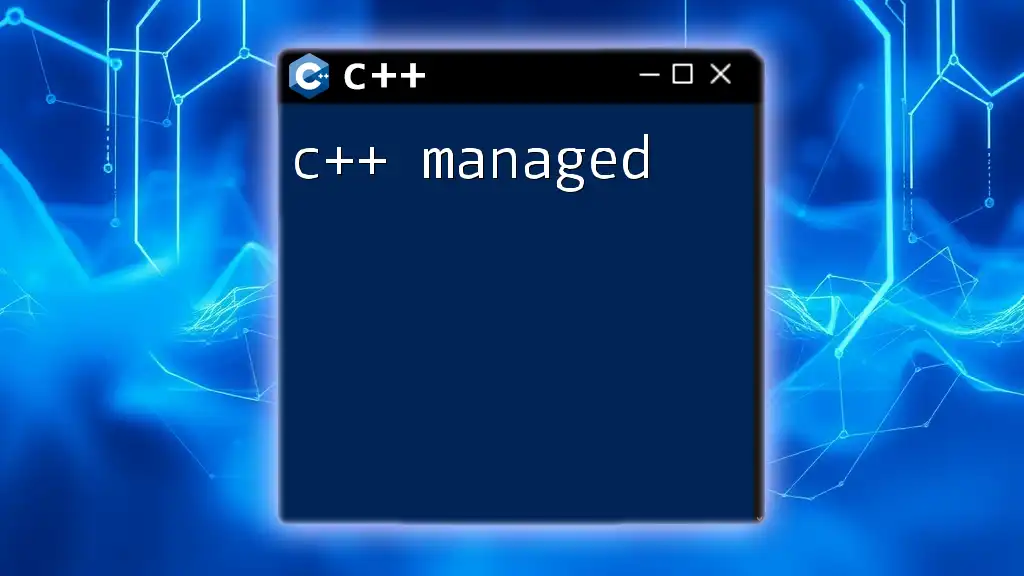
The Static Keyword and Linkage
Understanding the Use of `static`
The `static` keyword is pivotal in C++, controlling both storage duration and linkage. When applied within a function, it creates a variable that maintains its state between function calls, while at file scope, it restricts the variable’s visibility.
Code Snippet:
static void helperFunction() {
// This function has internal linkage
}
By using `static`, `helperFunction` prevents external access, safeguarding it within the file.
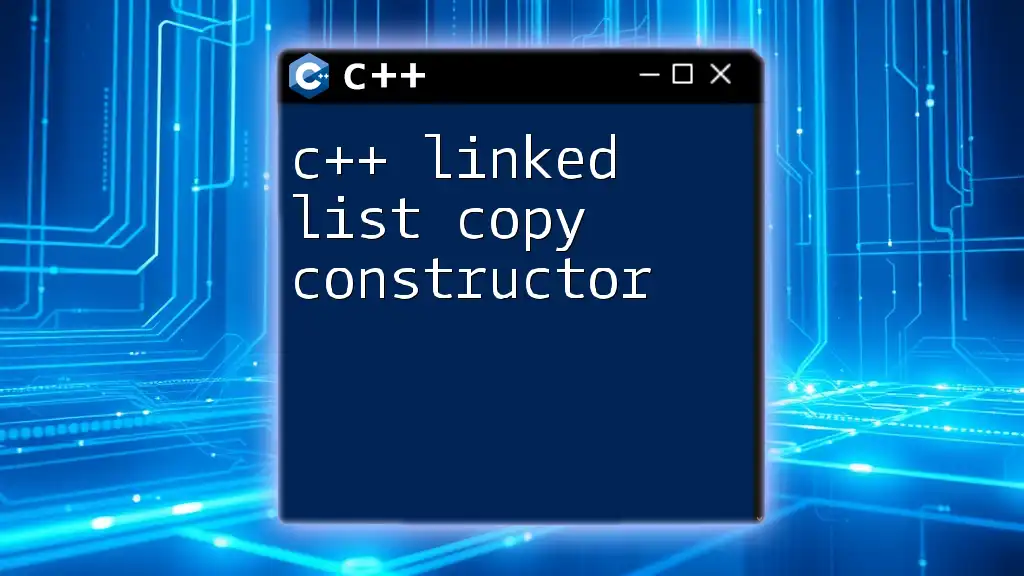
Linkage Specification in C++
Using `extern` for Linkage
The `extern` keyword explicitly declares a variable or function that is defined in another translation unit. This is crucial for modular programming and sharing data between files.
Code Snippet:
extern int globalVar; // Assume globalVar is defined elsewhere
This declaration informs the compiler of the existence of `globalVar`, allowing smooth linkage among different files.
Handling Linkage in C++ with `extern "C"`
C++ offers a mechanism to ensure compatibility with C code using `extern "C"`. This linkage specification prevents C++ name mangling, allowing C functions to be used in C++ programs seamlessly.
Code Snippet:
extern "C" {
void cFunction(); // This function uses C linkage
}
Using `extern "C"` provides a straightforward way to work with legacy C libraries, preserving their interoperability.
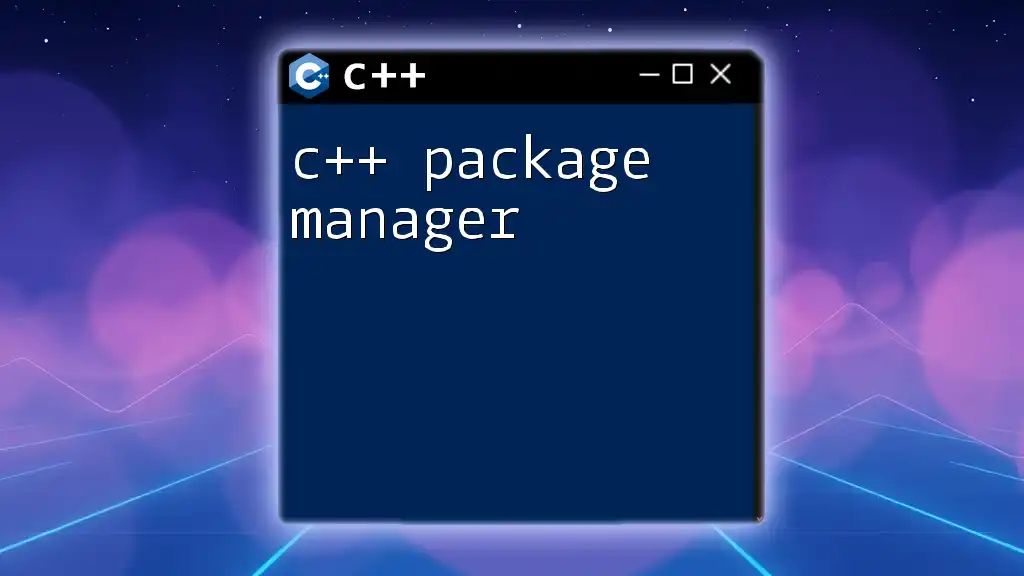
Common Linkage Issues
Linkage Errors in C++
Linkage is a source of many common errors in C++. Challenges such as undefined references or multiple definitions generally occur when the linkage is improperly handled. For instance, defining a variable in multiple translation units will yield linking errors.
Developers can resolve these issues by ensuring proper linkage use and following best practices like using `static` and `extern` judiciously.
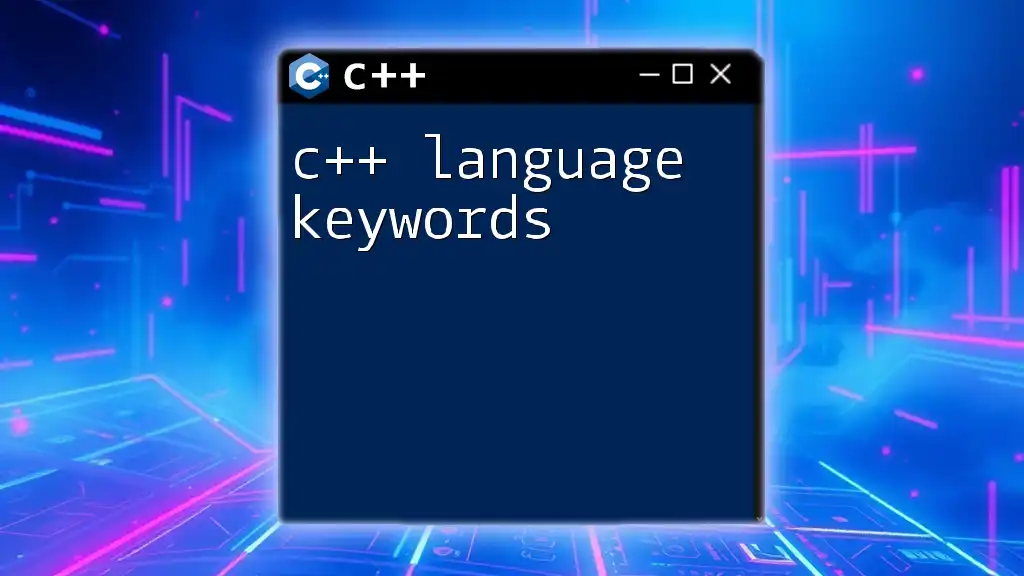
Conclusion
In summary, C++ linkage is a fundamental concept that governs how identifiers are accessed across multiple files. Whether it's determining whether a variable should be accessible globally or restricting a function's visibility, understanding the nuances of linkage is essential for effective C++ programming. Familiarizing oneself with linkage types and their implications can lead to cleaner, safer, and more efficient code.
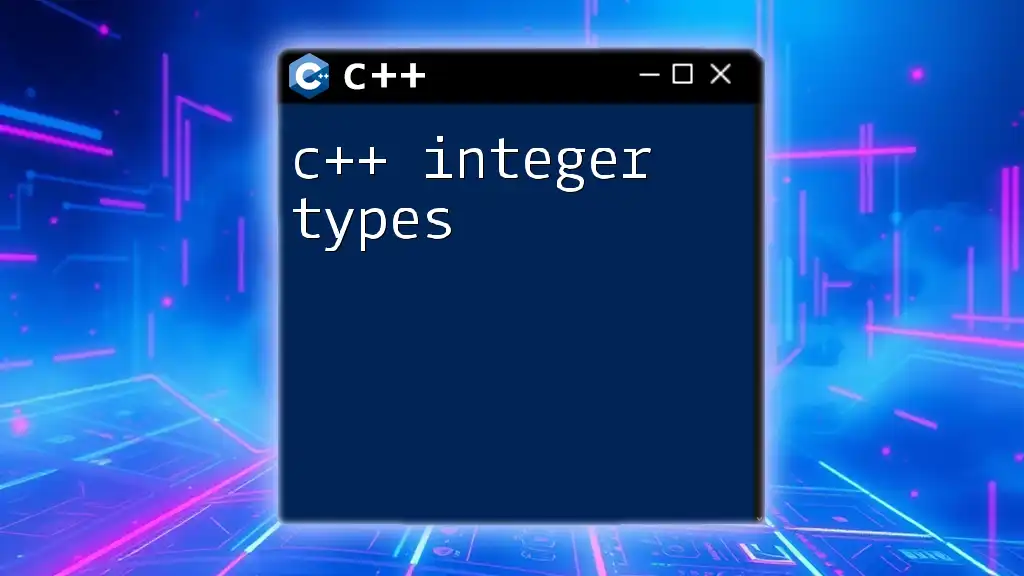
Additional Resources
For further exploration, consider consulting official C++ documentation, resources, and online forums where insights about linkage can be discussed and exchanged extensively. Engaging in programming communities can also enhance your understanding and application of these concepts effectively.