In C++, images can be handled with libraries such as OpenCV, which allows for easy manipulation and processing of image files. Here's a simple example of loading and displaying an image using OpenCV:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("path/to/image.jpg"); // Load an image
if (image.empty()) {
return -1; // Check if the image was loaded successfully
}
cv::imshow("Displayed Image", image); // Show the image
cv::waitKey(0); // Wait for a keystroke in the window
return 0;
}
Understanding Image Representation in C++
An image is essentially a grid of pixels, where each pixel can carry color information. In C++, images are represented using two-dimensional arrays (matrices) comprised of these pixels. Each pixel generally contains color data in various models, with RGB (Red, Green, Blue) being the most common. Underlying image data can be complex, consisting of numerous channels, depths, and formats.
How Images Are Stored in Memory
Images are usually stored in contiguous memory, where each pixel's color information corresponds to its position in the array. In a typical RGB format, each pixel is represented using three separate values (1 for red, 1 for green, and 1 for blue), allowing for over 16 million possible color combinations when combined. Understanding how images are structured in memory is vital for efficient processing and manipulation.
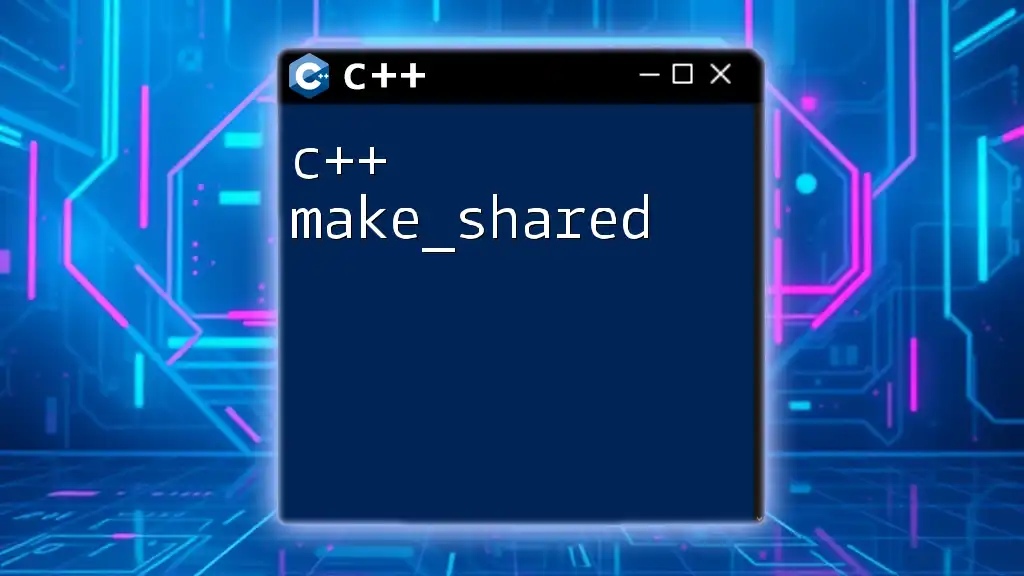
Libraries for Image Processing in C++
Popular C++ Libraries for Image Handling
When working with images in C++, it’s crucial to utilize efficient libraries designed for image processing. Here, we focus on three well-known libraries: OpenCV, CImg, and Boost.GIL.
OpenCV
OpenCV (Open Source Computer Vision Library) is a robust library extensively favored for image processing tasks.
Installing OpenCV: To install OpenCV, you can use a package manager like vcpkg or CMake. For example, using vcpkg, you can simply run:
vcpkg install opencv
Example: Loading and Displaying an Image:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("path/to/image.jpg");
if (image.empty()) {
std::cout << "Could not open or find the image!" << std::endl;
return -1;
}
cv::imshow("Display Image", image);
cv::waitKey(0);
return 0;
}
In this snippet, the `cv::imread` function reads the image from the specified path, while `cv::imshow` displays it in a window.
CImg
CImg is another handy C++ library that allows for straightforward manipulation of images.
Installing CImg: CImg can be easily included in your project by downloading the header file from its official website or GitHub repository.
Example: Basic Image Manipulations:
#include "CImg.h"
using namespace cimg_library;
int main() {
CImg<unsigned char> image("path/to/image.jpg");
image.blur(2.5);
image.save("output_image.jpg");
return 0;
}
Here, this code snippet demonstrates loading an image into a `CImg` object, applying a blur effect, and saving it back.
Boost.GIL
Boost.GIL (Generic Image Library) is a part of the Boost libraries used for image processing tasks.
Pros and Cons of Using Boost.GIL: Pros:
- Flexible and generic design
- Supports various pixel formats
Cons:
- More complex for beginners compared to OpenCV
- Limited documentation on advanced functionalities
Example: Simple Image Input and Output:
#include <boost/gil.hpp>
#include <boost/gil/extension/io/jpeg.hpp>
using namespace boost::gil;
int main() {
rgb8_image_t image;
read_image("path/to/image.jpg", image, jpeg_tag());
write_view("output_image.jpg", const_view(image), jpeg_tag());
return 0;
}
This snippet reads an image using Boost.GIL and saves it in JPEG format.
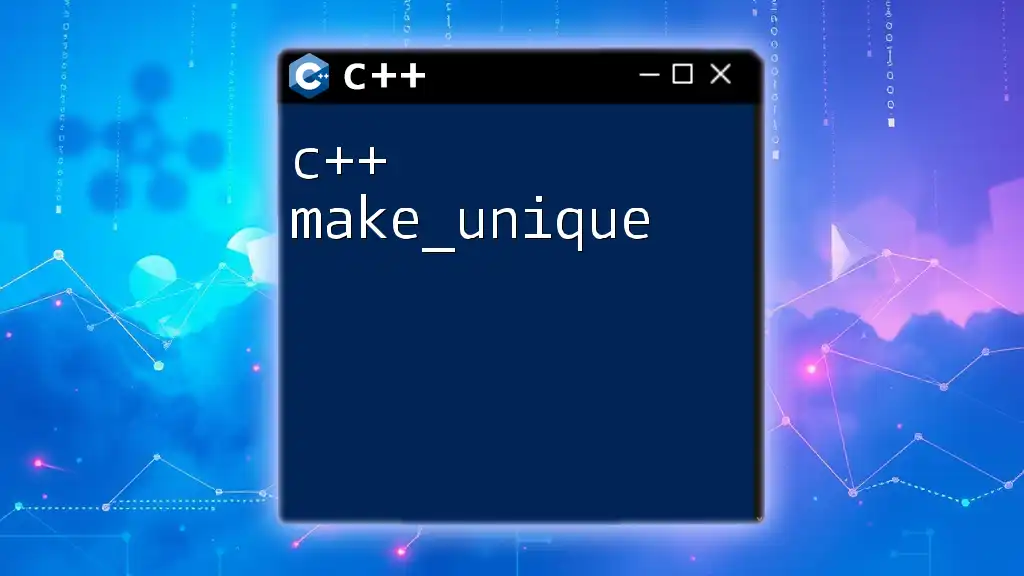
Basics of Image Manipulation with C++
Loading an Image
Loading an image is your first step toward image manipulation. Using OpenCV's `cv::imread()` function allows you to read the image from the file system effectively. If the path is incorrect or the file is missing, it returns an empty matrix.
Displaying an Image
To visualize the loaded image, you can use `cv::imshow()`, which creates a window displaying the image. The program will continue until you press a key, thanks to `cv::waitKey(0)`, which waits for a keystroke.
Saving an Image
You may want to save your manipulated images using OpenCV’s `cv::imwrite()` function, which requires the file path and the image matrix as parameters.
cv::imwrite("path/to/save/image.jpg", image);
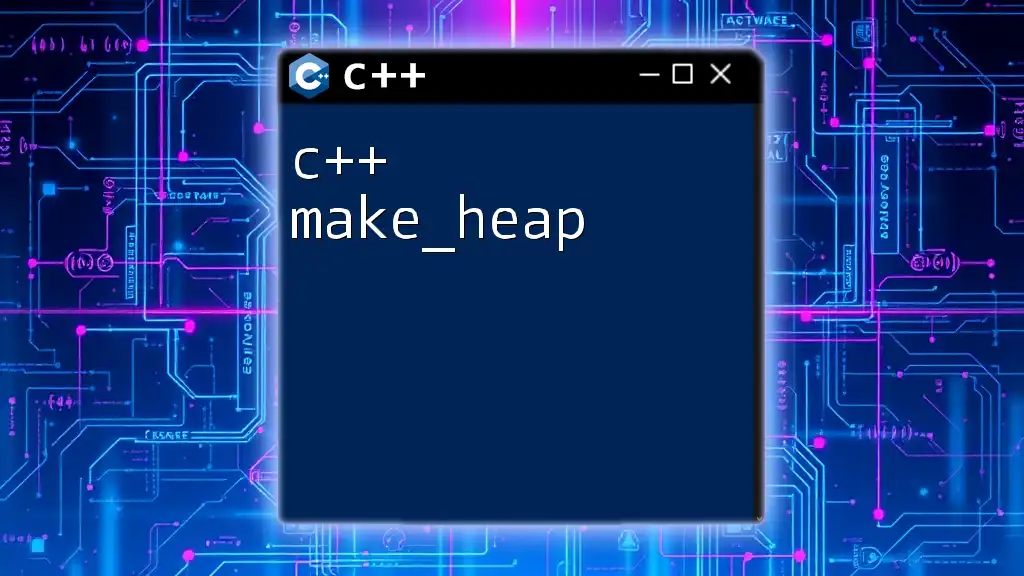
Image Formats and Conversion
Understanding Different Image Formats
Different image formats have distinct characteristics that affect quality, compression, and use cases. Common formats include:
- JPEG: Widely used, offers lossy compression.
- PNG: Supports transparency and lossless compression, ideal for graphics.
- BMP: Uncompressed format preserving quality but results in larger file sizes.
Converting Between Image Formats
To convert images between various formats using OpenCV, follow this example:
cv::Mat image = cv::imread("input.png");
cv::imwrite("output.jpg", image);
This reads an image in PNG format and saves it as JPEG. Understanding these formats enhances your ability to handle images effectively.
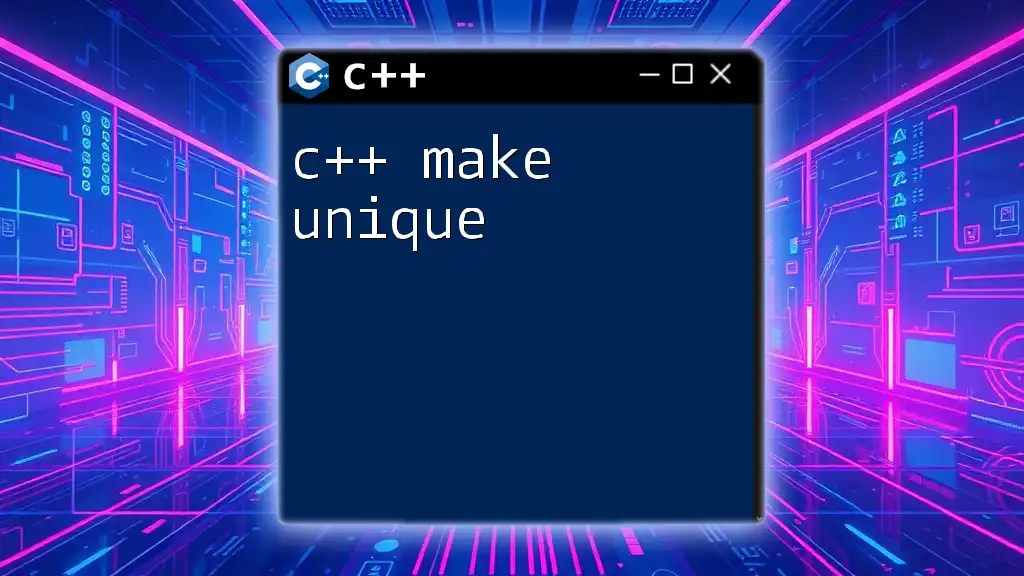
Advanced Image Manipulation Techniques
Image Filtering and Enhancement
Image filtering can significantly improve the appearance of images. Common filters include blurring and sharpening.
Example of a Gaussian Blur:
cv::GaussianBlur(image, output, cv::Size(5, 5), 0);
This applies a Gaussian blur, resulting in a smoother image ideal for noise reduction.
Image Transformation
Transformations can alter the size and orientation of images. Here are a few types of transformations:
-
Scaling:
cv::resize(image, resizedImage, cv::Size(newWidth, newHeight));
-
Rotation:
cv::rotate(image, rotatedImage, cv::ROTATE_90_CLOCKWISE);
-
Flipping:
cv::flip(image, flippedImage, 1); // Horizontal flip
These transformations are essential for preparing images for specific applications or display purposes.
Color Space Conversion
Different applications may require different color spaces. Common conversions are to grayscale or HSV.
Example of Converting Image to Grayscale:
cv::cvtColor(image, grayImage, cv::COLOR_BGR2GRAY);
This converts the original image to grayscale, making it simpler to process in many computer vision tasks.
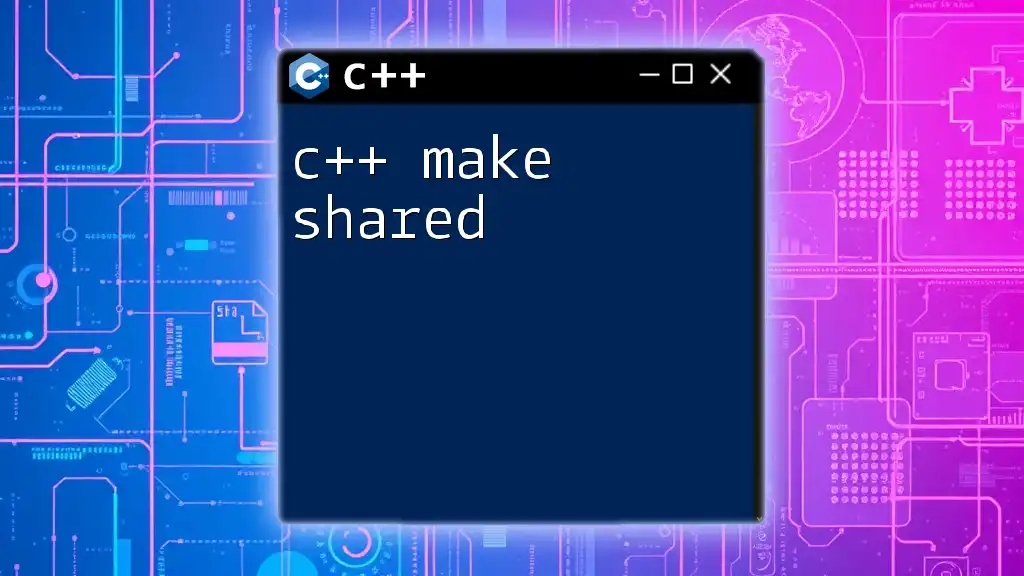
Image Analysis Using C++
Basic Image Analysis Techniques
Image segmentation and edge detection are critical for various applications.
Canny Edge Detection Example:
cv::Canny(image, edges, 100, 200);
cv::imshow("Edges", edges);
This highlights the edges within the image, which is beneficial for identifying shapes and structures.
Feature Detection
Feature detection helps in identifying significant elements within an image.
Examples of Feature Detectors: SIFT and ORB
cv::Ptr<cv::SIFT> sift = cv::SIFT::create();
std::vector<cv::KeyPoint> keypoints;
sift->detect(image, keypoints);
The SIFT detector extracts keypoints, which can be used for matching or recognizing objects within images.
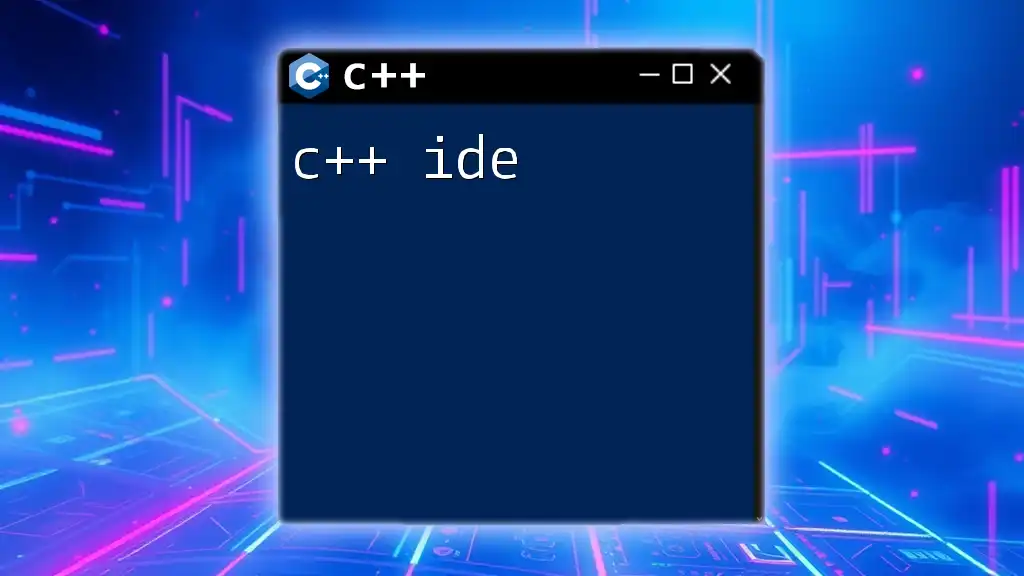
Integration of Image Processing in Applications
Building a Simple Image Processing Application
Developing an application allows you to apply various image processing techniques interactively.
- Overview of Application Structure: Create a user-friendly interface that allows users to upload, edit, and save images.
- Step-by-Step Tutorial: Implement functionalities like opening images, applying filters, and saving changes using the previously demonstrated snippets.
Code Snippets for Core Functionalities
For example, implement an open functionality using OpenCV:
cv::Mat image = cv::imread("path/to/uploaded/image.jpg");
cv::imshow("Uploaded Image", image);
Here, users can see their uploaded images immediately.
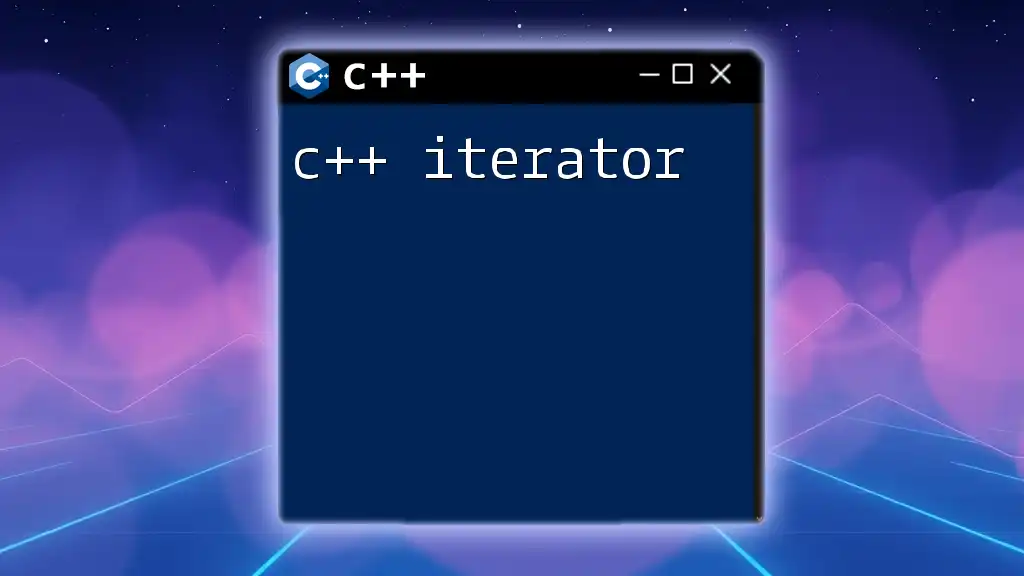
Debugging and Optimization Tips
Common Errors and Troubleshooting
While working with image processing in C++, you may encounter several errors, such as:
- File Not Found: Ensure the path to the image file is valid.
- Empty Matrices: Verify that images are loaded correctly with `cv::Mat::empty()`.
Keep an eye out for these common issues to streamline your debugging process.
Performance Optimization Strategies
Optimizing your image processing routines is crucial for performance, especially with high-resolution images. Some strategies include:
- Multithreading: Utilize concurrent processing to handle image operations.
- Efficient Memory Management: Use proper data types and structures to minimize memory overhead.
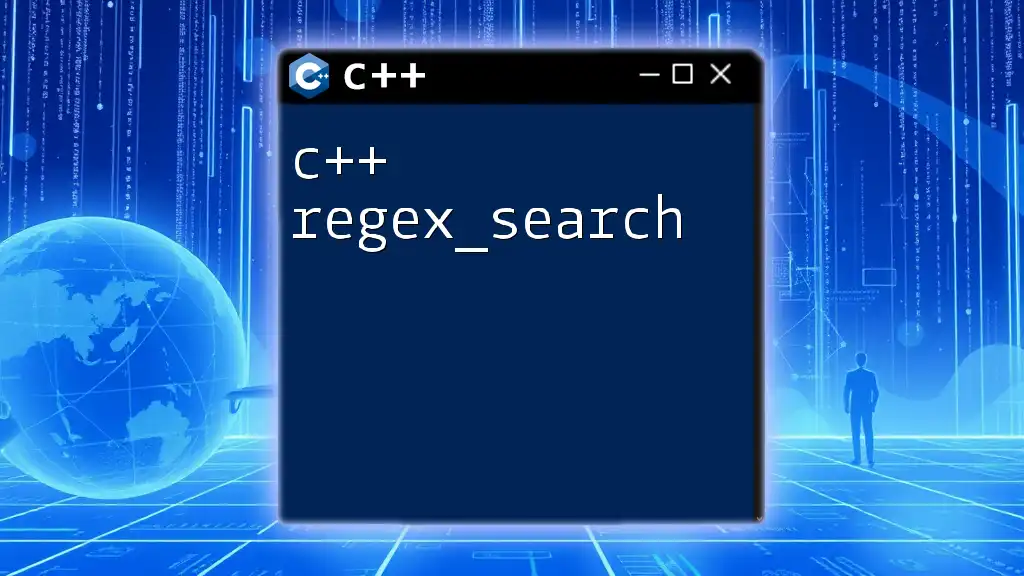
Conclusion
In conclusion, understanding how to manipulate, analyze, and optimize image processing using C++ is essential for developers and engineers in various fields, from computer graphics to machine learning. By leveraging the right libraries and techniques, you can significantly enhance your image processing capabilities.
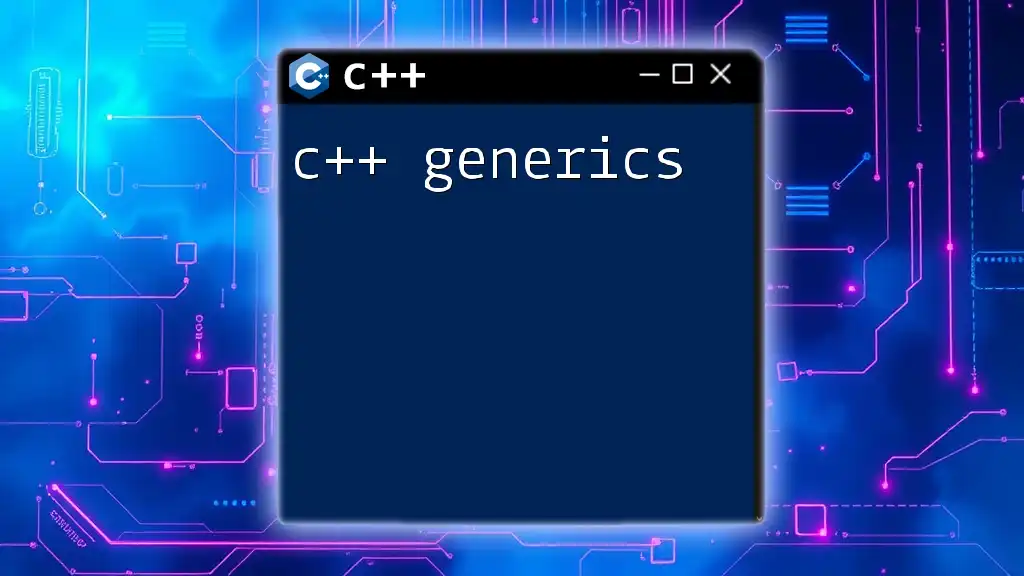
Resources for Further Learning
To deepen your understanding of C++ image processing, consider exploring:
- Books: Check out titles like "Learning OpenCV" or "Programming Computer Vision with Python."
- Online Courses: Several platforms offer specialized courses in computer vision and image processing techniques.
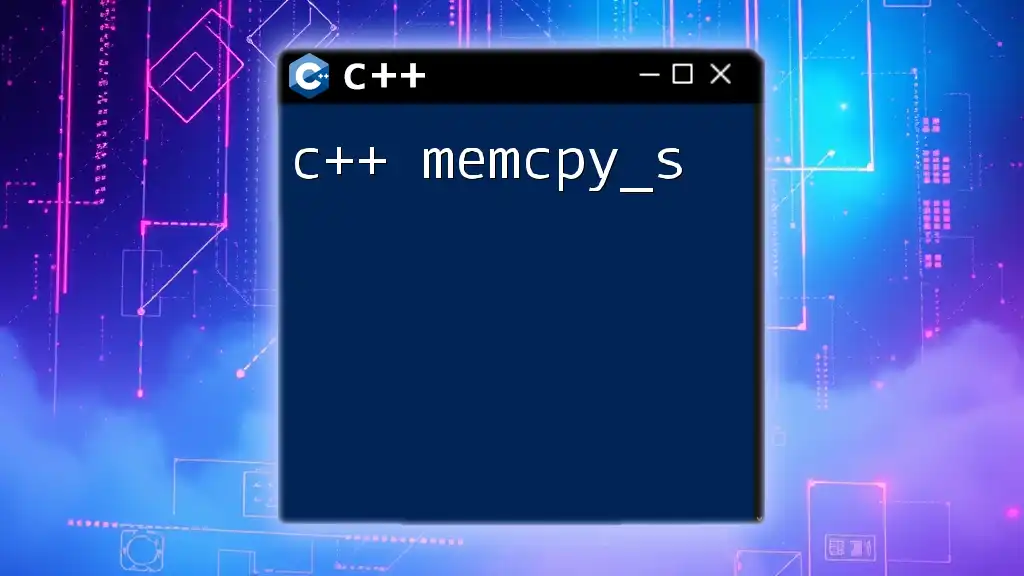
Call to Action
Now that you have a solid foundation in handling C++ images, don’t hesitate to experiment with the examples provided. Share your results and experiences, and feel free to visit our company for more hands-on C++ tutorials and personalized guidance!