C++ inheritance allows a class (derived class) to inherit attributes and methods from another class (base class), promoting code reusability and establishing a hierarchical relationship among classes.
class Base {
public:
void show() {
std::cout << "Base class method" << std::endl;
}
};
class Derived : public Base {
public:
void display() {
std::cout << "Derived class method" << std::endl;
}
};
int main() {
Derived obj;
obj.show(); // Inherited method
obj.display(); // Derived method
return 0;
}
What is Inheritance?
C++ inheritance is a fundamental concept in object-oriented programming (OOP) that allows one class (derived class) to inherit the properties and behaviors (methods) of another class (base class). This mechanism promotes code reusability and establishes a natural hierarchy among classes. With inheritance, you can create a more flexible and manageable code structure by allowing the derived classes to extend and enhance the functionality of base classes.
The Concept of Base and Derived Classes
In the context of inheritance, the base class is the class from which properties and methods are inherited, while the derived class is the class that inherits from the base class. Think of it as a parent-child relationship where the derived class can access and modify the characteristics of its base class.

Types of Inheritance
Each type of inheritance serves a different purpose and offers unique functionalities.
Single Inheritance
Single inheritance occurs when a derived class inherits from a single base class. This is the most straightforward type of inheritance and is commonly used.
class Animal {
public:
void speak() { std::cout << "Animal speaks"; }
};
class Dog : public Animal {
public:
void bark() { std::cout << "Dog barks"; }
};
In this example, the `Dog` class inherits the `speak` method from the `Animal` class. Thus, a `Dog` object can call both `speak` and `bark`, promoting code reuse and clarity.
Multiple Inheritance
Multiple inheritance allows a derived class to inherit from more than one base class. This can lead to greater flexibility but also poses challenges, such as the diamond problem, where ambiguities can arise from inheriting the same base class from multiple paths.
class Canine {
public:
void hunt() { std::cout << "Hunting"; }
};
class Pet {
public:
void play() { std::cout << "Playing"; }
};
class Dog : public Canine, public Pet {};
Here, `Dog` inherits from both `Canine` and `Pet`, gaining access to both the `hunt` and `play` methods. Developers must be cautious, however, to manage the complexities that can arise with multiple inheritance.
Multilevel Inheritance
Multilevel inheritance involves a chain of inheritance where a class is derived from another derived class. This structure allows flowing attributes through different levels of abstraction.
class Animal {
public:
void info() { std::cout << "This is an Animal"; }
};
class Dog : public Animal {
public:
void bark() { std::cout << "Dog barks"; }
};
class Puppy : public Dog {
public:
void whine() { std::cout << "Puppy whines"; }
};
In this hierarchy, `Puppy` inherits from `Dog`, which in turn inherits from `Animal`. Each class can extend the capabilities provided by its parent class, allowing for specialized methods that pertain specifically to the derived class.
Hierarchical Inheritance
In hierarchical inheritance, multiple derived classes inherit from a single base class. This allows for shared functionality while specializing behavior in derived classes.
class Shape {
public:
void area() { std::cout << "Calculating area"; }
};
class Circle : public Shape {
public:
void draw() { std::cout << "Drawing Circle"; }
};
class Square : public Shape {
public:
void draw() { std::cout << "Drawing Square"; }
};
Both `Circle` and `Square` classes inherit from `Shape`, allowing them to utilize the `area` method while also implementing their specific `draw` methods.
Hybrid Inheritance
Hybrid inheritance is a combination of the different styles outlined above. It offers the flexibility to mold the class hierarchy as needed but requires careful attention to avoid complications from conflicting base classes.

Access Specifiers in Inheritance
C++ allows you to specify how members of a base class are accessed by derived classes through access specifiers: public, protected, or private.
Public Inheritance
With public inheritance, the public and protected members of the base class remain accessible within the derived class.
class Base {
public:
void show() { std::cout << "Base class"; }
};
class Derived : public Base {};
In this case, `Derived` can access the `show` method of `Base` freely.
Protected Inheritance
Protected inheritance limits access to the base class’s members, allowing only the derived class and its further descendants to access them.
class Base {
protected:
void display() { std::cout << "Protected method"; }
};
class Derived : protected Base {};
Here, `display` is inaccessible outside of `Derived` and its subclasses, providing a layer of encapsulation.
Private Inheritance
With private inheritance, base class members are accessible only to the derived class itself, not to other classes, even if they derive from it.
class Base {
public:
void show() { std::cout << "Show from Base"; }
};
class Derived : private Base {};
In this case, even derived classes from `Derived` cannot access `show`.
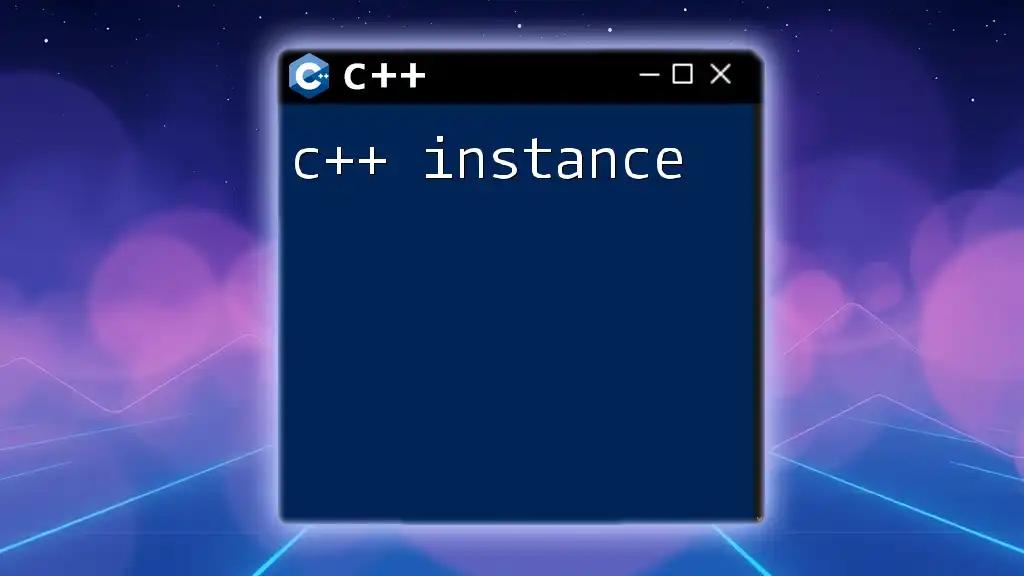
Virtual Inheritance
What is Virtual Inheritance?
Virtual inheritance is a technique used to resolve the diamond problem by ensuring that a class is only instantiated once in an inheritance hierarchy, despite appearing multiple times.
Implementing Virtual Inheritance
To implement virtual inheritance, use the `virtual` keyword when specifying base class inheritance.
class Base {
public:
void greet() { std::cout << "Hello from Base"; }
};
class A : virtual public Base {}; // virtual inheritance
class B : virtual public Base {};
class C : public A, public B {};
In this situation, `C` only has a single instance of `Base`, resolving ambiguity and ensuring that all features of `Base` are accessible.
Implications and Use Cases
Virtual inheritance is particularly useful in complex class hierarchies where a single base class should provide a common interface or functionality to multiple derived classes. This ensures a clean and manageable inheritance structure.
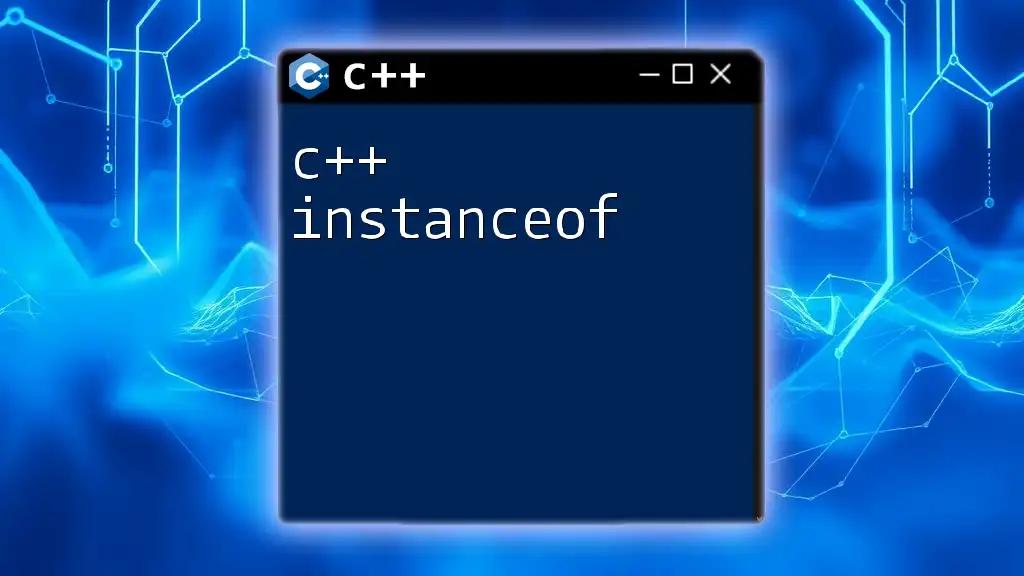
Polymorphism and Inheritance
Runtime Polymorphism
Polymorphism, specifically runtime polymorphism, allows methods to be called on objects without knowing their exact type at compile time. This is often achieved using virtual functions.
class Base {
public:
virtual void sound() { std::cout << "Some sound"; }
};
class Dog : public Base {
public:
void sound() override { std::cout << "Bark"; }
};
class Cat : public Base {
public:
void sound() override { std::cout << "Meow"; }
};
Using virtual functions allows you to call the `sound` method on any `Base` pointer, resulting in behavior that depends on the actual object type, whether it be `Dog`, `Cat`, or another derived class.
Method Overriding
Method overriding occurs when a derived class provides a specific implementation of a method that is already defined in its base class. This enhances class flexibility and behavior customization while maintaining the overarching class structure.

Conclusion
In this comprehensive guide on C++ inheritance, we explored the core principles of inheritance, its various types, and their practical applications in programming. By understanding these concepts, developers can build efficient, scalable, and reusable code structures in C++.
Best practices for using inheritance include carefully structuring your class hierarchy, preferring composition over inheritance when applicable, and fully leveraging polymorphism to achieve dynamic behavior. With a solid grasp of C++ inheritance, you can significantly improve your programming capabilities, making your code more expressive and maintainable.