Multiple inheritance in C++ allows a class to inherit features from more than one base class, enabling the reuse of code and the creation of complex class hierarchies.
Here’s a simple code snippet demonstrating multiple inheritance:
#include <iostream>
class Base1 {
public:
void displayBase1() {
std::cout << "Base Class 1" << std::endl;
}
};
class Base2 {
public:
void displayBase2() {
std::cout << "Base Class 2" << std::endl;
}
};
class Derived : public Base1, public Base2 {
};
int main() {
Derived obj;
obj.displayBase1();
obj.displayBase2();
return 0;
}
Understanding Multiple Inheritance in C++
Definition of Multiple Inheritance
Multiple inheritance in C++ allows a class (derived class) to inherit features from more than one base class. This capability stands in contrast to single inheritance, where a class derives from a single parent class. By enabling the derived class to inherit the attributes and methods of multiple base classes, multiple inheritance opens up diverse avenues for designing flexible and reusable code structures.
Syntax of Multiple Inheritance in C++
The syntax for implementing multiple inheritance in C++ is relatively straightforward. It involves specifying more than one base class while defining the derived class. Here’s a basic structure of how you would implement it:
class Base1 {
public:
void displayBase1() {
cout << "Base1" << endl;
}
};
class Base2 {
public:
void displayBase2() {
cout << "Base2" << endl;
}
};
class Derived : public Base1, public Base2 {
// Class implementation
};
In this example, `Derived` class inherits from both `Base1` and `Base2`, allowing it to access their respective methods.
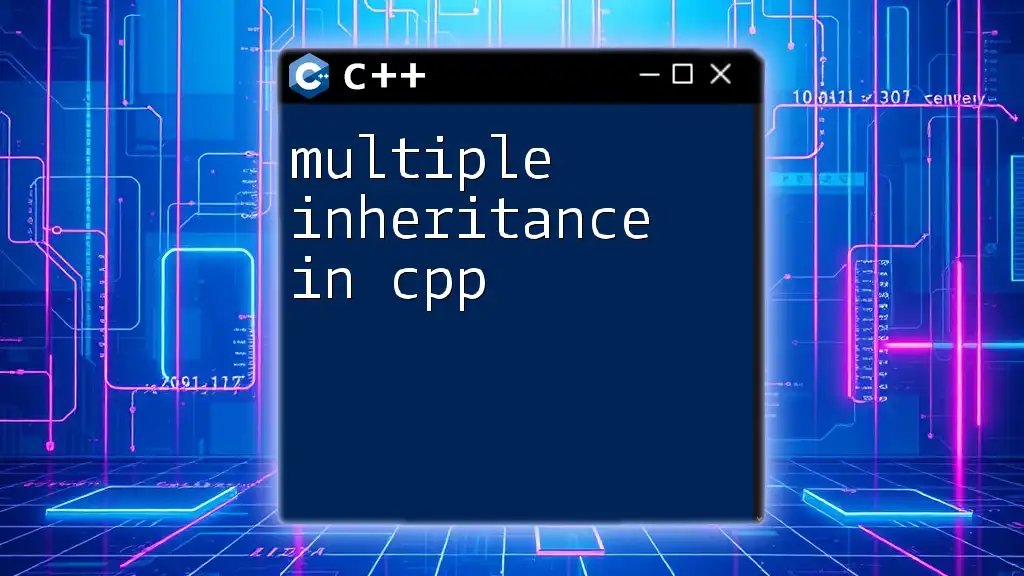
Advantages of C++ Multiple Inheritance
Code Reusability
One of the core advantages of multiple inheritance is code reuse. This principle allows developers to define common functionality in base classes and implement it across various derived classes. Without the need to repeat code, this not only makes the code shorter and more manageable but also adheres to the DRY (Don't Repeat Yourself) principle.
Enhanced Functionality
Multiple inheritance also enhances the functionality of classes. By inheriting from multiple sources, a derived class can benefit from various functionalities without needing to reimplement them. For instance, a class `Smartphone` can derive features from classes `Camera` and `Phone` to create a comprehensive structure.
Flexibility in Design
Another significant benefit of multiple inheritance is the flexibility it provides in terms of class design. Developers can compose specialized classes that amalgamate the behavior of several classes. This can be especially beneficial in larger systems where behaviors are modularized.
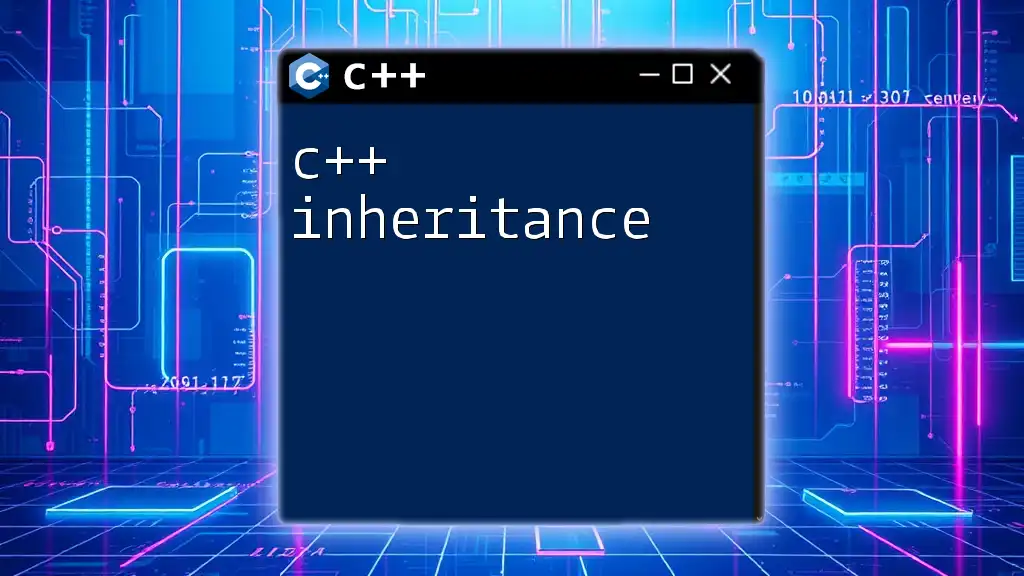
Challenges and Considerations in Multi-Inheritance C++
The Diamond Problem
An essential challenge of multiple inheritance is known as the diamond problem. This occurs when two base classes inherit from a common base class. In such cases, ambiguity arises in determining which path to follow when accessing members of the common base class.
For example:
class A {
public:
void display() {
cout << "Display from A" << endl;
}
};
class B : public A {
};
class C : public A {
};
class D : public B, public C {
// Class implementation
};
In this scenario, class `D` inherits from both `B` and `C`, which both inherit from `A`. The issue arises when trying to call `display()` from `A`. Is it `B::display()` or `C::display()`? This ambiguity highlights the necessity for careful design considerations.
Ambiguity Resolution
To resolve ambiguities stemming from multiple inheritance, C++ provides a mechanism that utilizes the scope resolution operator `::`. This operator allows you to specify which base class's member function you intend to access, thus clarifying the call.
D d;
d.B::display(); // Calls display from class A through B
d.C::display(); // Calls display from class A through C
This clarity is indispensable for maintaining understandable code as it avoids confusion and maintains clean inheritance paths.
Performance Implications
While multiple inheritance provides numerous benefits, it also comes with performance overheads. In certain scenarios, the compiler may need to generate additional pointers or virtual tables to accommodate the complexities involved in resolving which base class's methods or variables should be accessed. Such implications can lead to slower performance, particularly in high-performance applications.
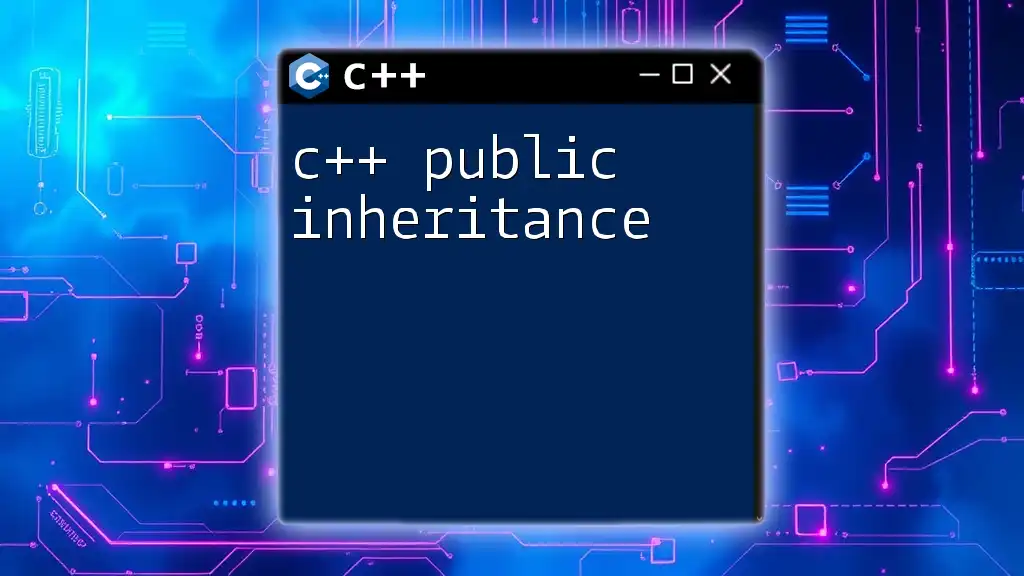
Best Practices for Using C++ Multiple Inheritance
When to Use C++ Multiple Inheritance
It's crucial to understand when to employ multiple inheritance. While it can be advantageous for creating robust class architectures, it should be used judiciously. Best practice dictates that multiple inheritance is sensible only when classes genuinely need to share behaviors without excessive complexity.
Implementing Interfaces with Abstract Base Classes
One effective way to leverage multiple inheritance without incurring some of its pitfalls is to use abstract base classes. By creating base classes that define interfaces through pure virtual functions, you streamline the design and ensure derived classes implement necessary functionalities.
class Interface1 {
public:
virtual void func() = 0; // Pure virtual function
};
class Interface2 {
public:
virtual void func2() = 0; // Pure virtual function
};
class Concrete : public Interface1, public Interface2 {
public:
void func() override {
cout << "Implementing func" << endl;
}
void func2() override {
cout << "Implementing func2" << endl;
}
};
In this example, `Concrete` implements two interfaces, maintaining a clear separation between functionality while allowing flexibility and modularity in the design.
Clear and Consistent Documentation
With the complexity of multiple inheritance comes the necessity for clear and consistent documentation. Code documentation should thoroughly describe the inheritance relationships among classes, making it easier for other developers (and future you) to understand the structure and purpose of each class. Utilizing comments, diagrams, and other documentation tools can greatly enhance comprehension.
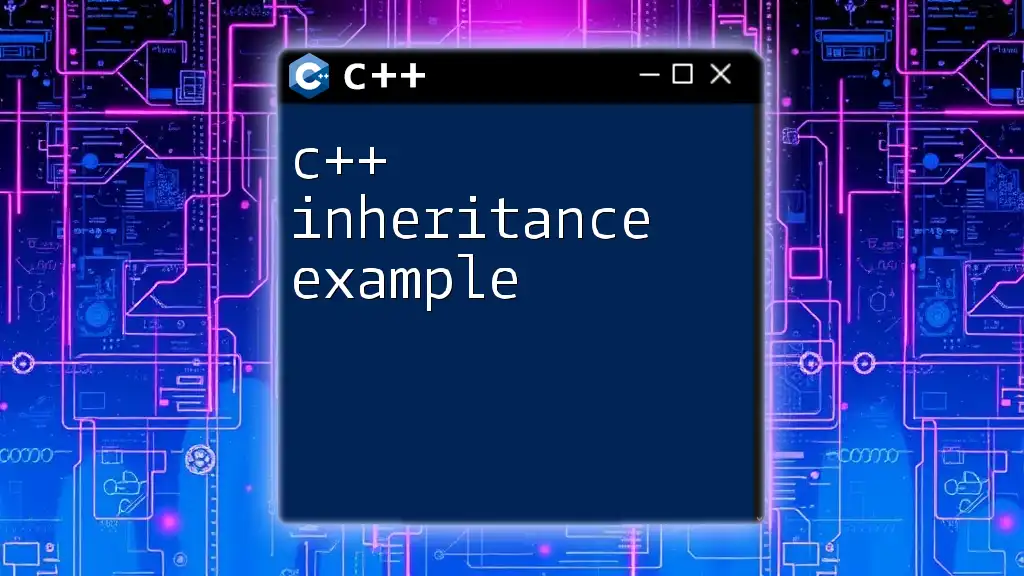
Conclusion
In summary, multiple inheritance in C++ offers a powerful mechanism for combining functionalities from various base classes into a single derived class. However, it also brings challenges, particularly concerning ambiguity and performance. By understanding the advantages and pitfalls of `multiple inheritance c++`, developers can employ best practices to design clean, efficient, and maintainable code. Emphasis on documentation and strategic use of abstract classes can further mitigate the complexities involved in managing multiple inheritance scenarios. The key lies in balancing flexibility with clarity, ensuring that the architecture meets both current and future needs efficiently.
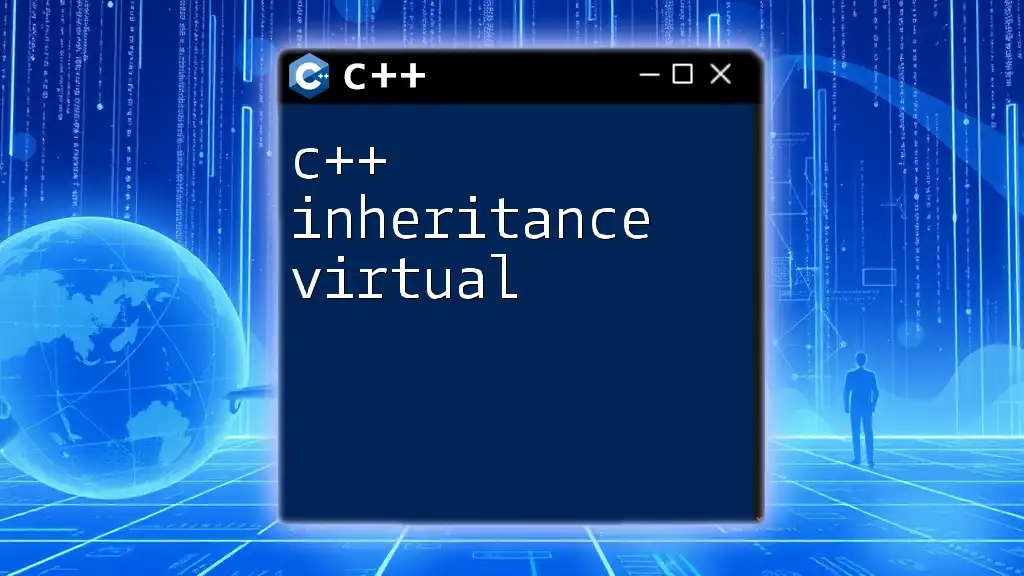
Further Reading and Resources
For more insights and practical examples about multiple inheritance and other related C++ concepts, consider exploring additional programming resources, online courses, and forums that delve into Object-Oriented Programming (OOP) principles.