Multiple inheritance in C++ allows a class to inherit from more than one base class, enabling it to combine features and functionality from multiple sources.
Here's a simple example:
#include <iostream>
using namespace std;
// Base class A
class A {
public:
void displayA() {
cout << "Display A" << endl;
}
};
// Base class B
class B {
public:
void displayB() {
cout << "Display B" << endl;
}
};
// Derived class C inheriting from both A and B
class C : public A, public B {
};
int main() {
C obj;
obj.displayA(); // Calling function from base class A
obj.displayB(); // Calling function from base class B
return 0;
}
What is Multiple Inheritance?
Multiple inheritance in C++ refers to the capability of a class to inherit from more than one base class. This feature allows the derived class to inherit characteristics and functionalities from multiple sources, thereby promoting code reusability and leveraging existing class functionalities. It is significant in object-oriented programming because it models real-world relationships more naturally. For example, if a `Car` class inherits both `Vehicle` and `Engine` classes, it can utilize properties from both, making it a multi-faceted object.
Overview of C++ Inheritance
To fully grasp multiple inheritance in C++, it’s essential to understand its counterpart, single inheritance. In single inheritance, a derived class acquires properties and behaviors from only one base class. This linear relationship is straightforward but may not suffice for complex models where an entity has multiple roles or attributes.
Contrast this with multiple inheritance, where a derived class has two or more parent classes. This multidirectional approach can represent more complex designs but introduces additional complexity, particularly concerning member access and the risk of ambiguity.
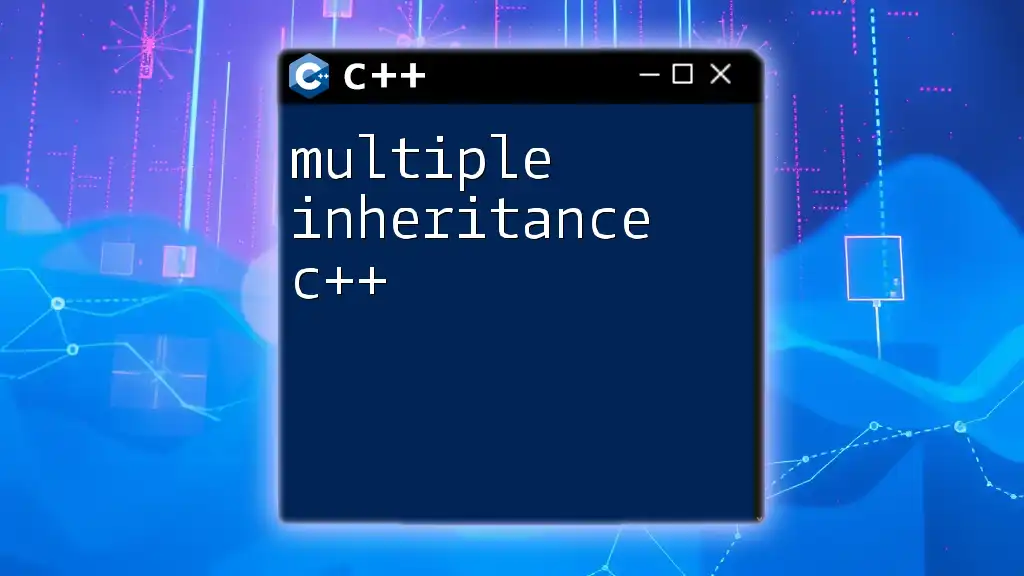
The Mechanics of Multiple Inheritance
How Multiple Inheritance Works
When using multiple inheritance, a derived class inherits attributes and methods from more than one base class. This capability allows it to combine features from various classes, thus granting it a richer set of functionalities that differentiate it.
For instance, consider a UML diagram where a triangle class inherits from geometric shapes like `Polygon` and `Color`. Here, `Triangle` encapsulates both the characteristics of polygons plus its unique color attributes.
Syntax of Multiple Inheritance
The syntax employed in multiple inheritance is notably straightforward. Below is a basic structure indicating how one class derives from multiple base classes:
class Base1 {
// Base class 1 members
};
class Base2 {
// Base class 2 members
};
class Derived : public Base1, public Base2 {
// Derived class members
};
In this example, `Derived` class inherits from both `Base1` and `Base2`. This structure allows `Derived` to utilize features from both base classes, emphasizing the concept of code reuse.
Accessing Members from Base Classes
One significant aspect of multiple inheritance is accessing members from the base classes. To reference members from each base class in the derived class, you should specify the base class’s name. Here’s an illustrative example:
class A {
public:
void displayA() {
std::cout << "Display from Class A\n";
}
};
class B {
public:
void displayB() {
std::cout << "Display from Class B\n";
}
};
class Derived : public A, public B {
public:
void display() {
displayA(); // Accessing A's method
displayB(); // Accessing B's method
}
};
In the example above, the `Derived` class calls methods from both `A` and `B`, demonstrating the flexibility that multiple inheritance provides.
Ambiguity in Multiple Inheritance
Ambiguity is a common issue arising in multiple inheritance, particularly when the derived class inherits from two classes containing a method or member variable with the same name. This can lead to confusion in the compiler regarding which member to reference. For instance:
class A {
public:
void display() {
std::cout << "Display from Class A\n";
}
};
class B {
public:
void display() {
std::cout << "Display from Class B\n";
}
};
class Derived : public A, public B {
// Ambiguity arises here
};
In the `Derived` class, simply calling `display()` results in an ambiguity error. The compiler does not know whether to call `A::display()` or `B::display()`. To resolve this, you must use scope resolution:
A::display(); // Calls A's display method
B::display(); // Calls B's display method
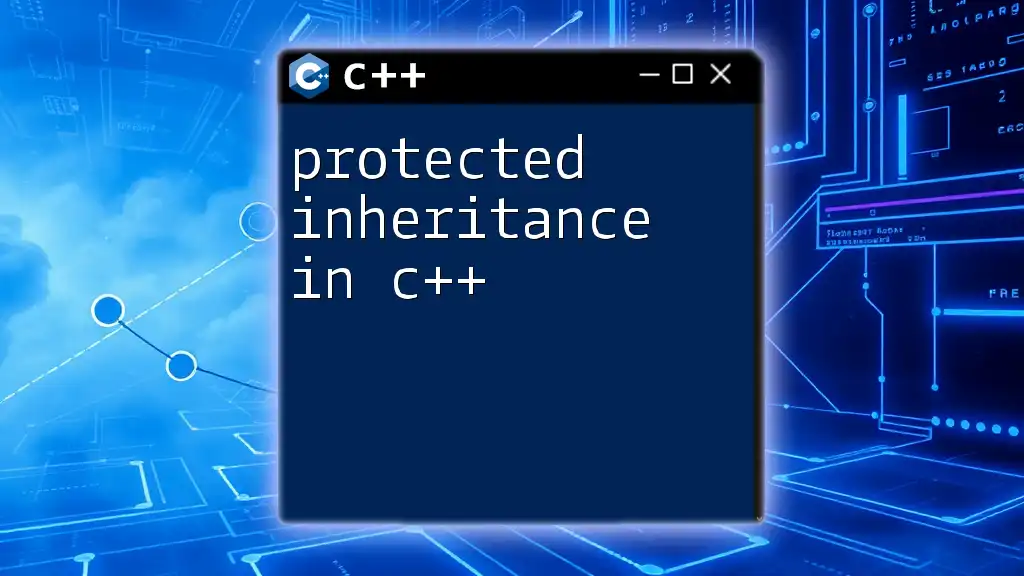
Types of Multiple Inheritance
Diamond Problem
The diamond problem is a specific type of ambiguity that occurs when a class inherits from two classes that have a common base class. This results in the derived class having two copies of the base class, leading to confusion about which base class's methods or properties should be referenced.
Here's an example illustrating the diamond problem:
class Base {
public:
void display() {
std::cout << "Display from Base\n";
}
};
class Derived1 : public Base {
public:
void display() {
std::cout << "Display from Derived1\n";
}
};
class Derived2 : public Base {
public:
void display() {
std::cout << "Display from Derived2\n";
}
};
class FinalDerived : public Derived1, public Derived2 {
// Diamond problem occurs here
};
In this case, `FinalDerived` has two instances of `Base`, leading to ambiguity about which `display()` function should be called.
Virtual Inheritance
Virtual inheritance is a technique used to resolve the diamond problem. It ensures that only one instance of the common base class is created, thereby eliminating ambiguity. To implement virtual inheritance, the `class` declaration for the base class needs to be prefixed with the `virtual` keyword:
class Base {
public:
void display() {
std::cout << "Display from Base\n";
}
};
class Derived1 : virtual public Base {
public:
void display() {
std::cout << "Display from Derived1\n";
}
};
class Derived2 : virtual public Base {
public:
void display() {
std::cout << "Display from Derived2\n";
}
};
class FinalDerived : public Derived1, public Derived2 {
// Now there's one instance of Base
};
Using virtual inheritance ensures that `FinalDerived` has a single shared instance of `Base`, resolving any ambiguity related to member function calls.
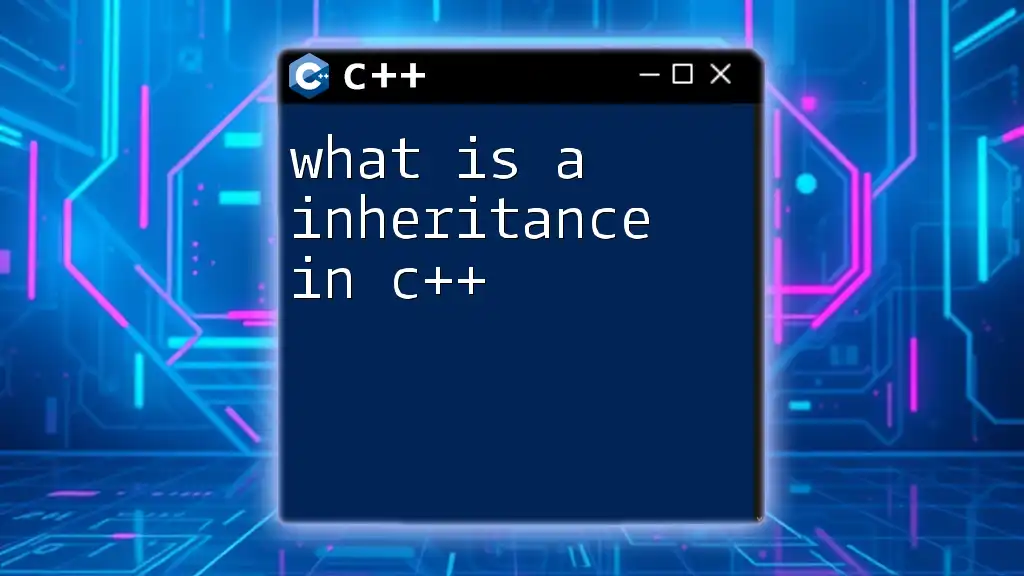
Best Practices and Use Cases of Multiple Inheritance
When to Use Multiple Inheritance
While multiple inheritance can be powerful, it is essential to know when to employ it effectively. It can be beneficial in scenarios where:
- A class naturally fits multiple roles (e.g., a class representing a logging utility that can both write to a file and print to the console).
- Complex relationships exist that require functionality from various classes.
Real-world examples include frameworks that are designed to combine diverse functionalities — such as a GUI class library that extends both visual components and event handling capabilities.
Common Pitfalls to Avoid
Despite its advantages, multiple inheritance introduces complexities that can lead to several pitfalls, including:
- Increased complexity: Code can become difficult to understand and maintain.
- Ambiguity issues: As discussed, navigating ambiguous member names can complicate debugging.
- Inheriting non-polymorphic behaviors: It may lead to unexpected behavior if parent classes are not designed with multiple inheritance in mind.
To safely navigate these pitfalls, prefer composition over inheritance where practical and ensure proper design considerations are made when implementing multiple inheritance. Always prioritize clarity in your code structure.
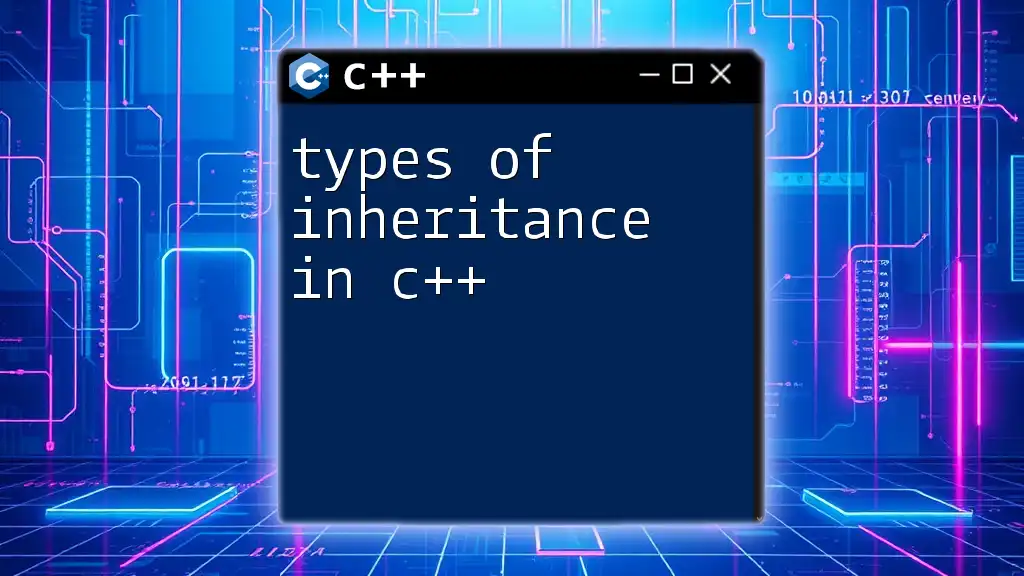
Conclusion
In summary, multiple inheritance in C++ is a powerful feature that allows a derived class to inherit attributes from multiple base classes. While it offers benefits in terms of code reuse and design flexibility, it also comes with challenges like ambiguity and complexity. By understanding these principles and employing virtual inheritance when necessary, programmers can effectively manage these challenges, enriching their codebase while avoiding potential pitfalls.
Further Reading and Resources
For those keen on delving deeper into multiple inheritance in C++, consider exploring the following resources:
- C++ Primer by Stanley B. Lippman et al.
- Effective C++ by Scott Meyers
- Online documentation and tutorials on C++ inheritance and polymorphism.
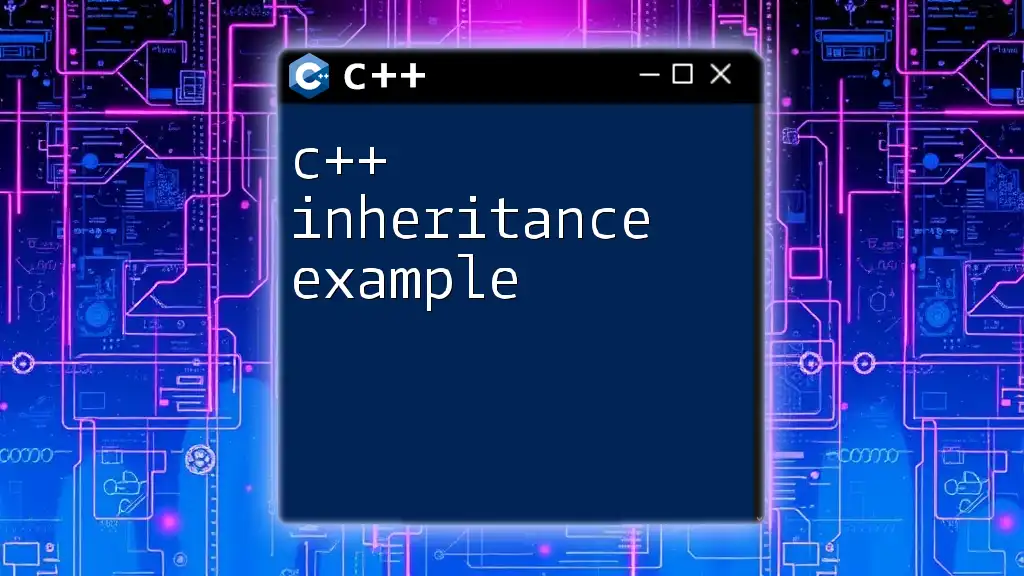
Complete Example of Multiple Inheritance
To further solidify your understanding, consider the following complete example of multiple inheritance:
class A {
public:
void displayA() {
std::cout << "Display from Class A\n";
}
};
class B {
public:
void displayB() {
std::cout << "Display from Class B\n";
}
};
class C : public A, public B {
public:
void displayC() {
std::cout << "Display from Class C\n";
}
};
int main() {
C obj;
obj.displayA(); // Output: Display from Class A
obj.displayB(); // Output: Display from Class B
obj.displayC(); // Output: Display from Class C
return 0;
}
This example illustrates the seamless blending of functionalities from multiple base classes in a derived class, emphasizing the core idea of multiple inheritance in C++. Engaging with hands-on examples will deepen your comprehension as you explore this intricate topic.