A user interface in C++ can be created using libraries such as Qt or SFML to facilitate interaction between the user and the application.
Here's a simple example using the basic SFML library to create a window:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "My Window");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.display();
}
return 0;
}
What is a User Interface?
A user interface (UI) refers to the point of interaction between the user and a software application. It encompasses all elements that allow users to communicate with the system, such as buttons, menus, text fields, and more. A well-crafted user interface is crucial because it significantly impacts user experience and overall satisfaction.
The Role of C++ in User Interface Design
C++ is a powerful programming language that combines high performance with a robust set of features, making it suitable for UI development. Through the use of various frameworks, C++ allows developers to create responsive, visually appealing user interfaces. Notable frameworks for C++ UI development include Qt, wxWidgets, and FLTK. Each of these frameworks offers unique capabilities that cater to different UI requirements.
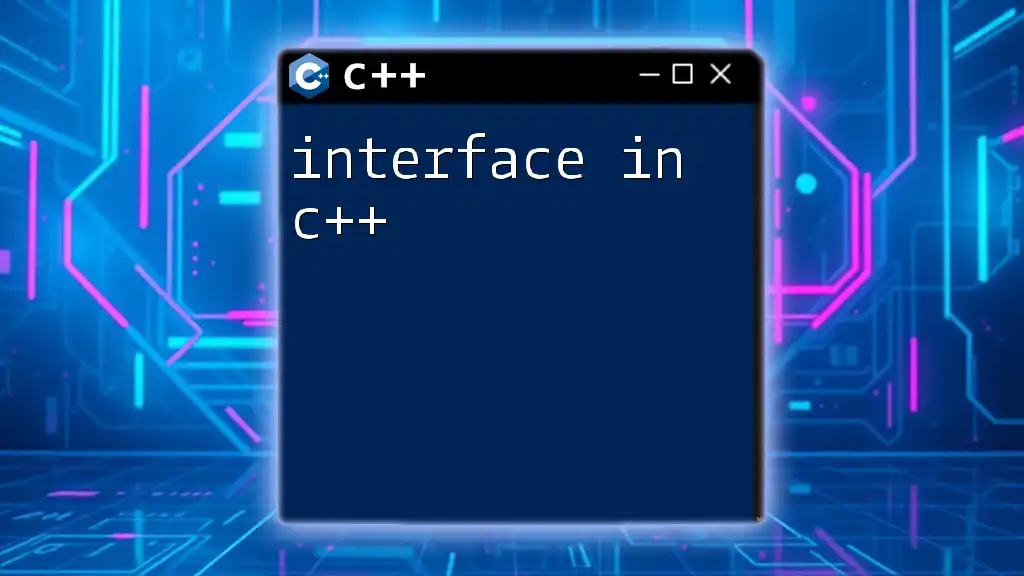
Types of User Interfaces
Graphical User Interface (GUI)
A Graphical User Interface (GUI) utilizes visual components like windows, icons, and buttons to interact with users. The advantages of GUIs include intuitiveness, ease of use, and the ability to convey a large amount of information visually. GUIs allow users to perform complex tasks with minimal effort, which is crucial for user adoption.
Command Line Interface (CLI)
A Command Line Interface (CLI) operates through text-based commands, requiring users to input commands directly through a console. Although they can seem less user-friendly compared to GUIs, CLIs are powerful for more advanced users who appreciate speed and direct control over their actions. Familiarity with CLI can be beneficial, especially when working with scripts and automated tasks.
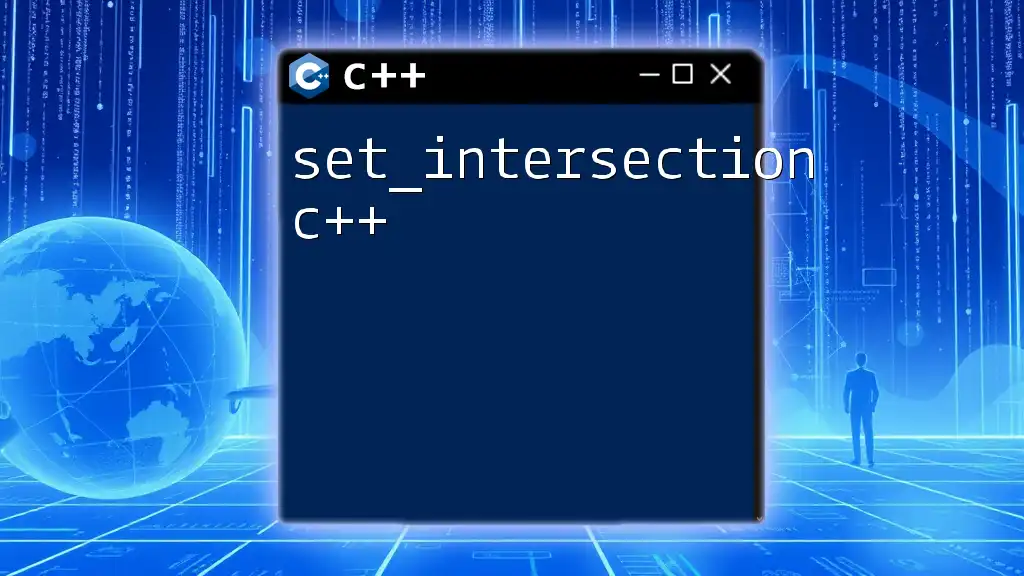
Choosing the Right Framework for C++ User Interface Development
Overview of Popular C++ UI Frameworks
When developing a user interface in C++, selecting the right framework is essential. Here's a look at some of the most popular C++ UI frameworks:
Qt
Qt is one of the most widely used frameworks for C++ user interfaces. Its user-friendly design and comprehensive set of libraries make it a top choice for both beginners and experienced developers.
Example: Creating a Simple Window with Qt
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Simple Qt Window");
window.show();
return app.exec();
}
wxWidgets
wxWidgets is another versatile framework that allows developers to create applications with a native look and feel. It provides extensive support for various platforms.
Example: A Basic wxWidgets Application
#include <wx/wx.h>
class MyApp : public wxApp {
public:
virtual bool OnInit();
};
class MyFrame : public wxFrame {
public:
MyFrame() : wxFrame(NULL, wxID_ANY, "Simple wxWidgets Window") {
SetSize(320, 240);
}
};
bool MyApp::OnInit() {
MyFrame *frame = new MyFrame();
frame->Show(true);
return true;
}
wxIMPLEMENT_APP(MyApp);
FLTK
FLTK (Fast, Light Toolkit) is lightweight and focused on simplicity, making it easy to use for developing graphical applications.
Example of a Minimal FLTK Application
#include <FL/Fl.H>
#include <FL/Fl_Window.H>
int main() {
Fl_Window *window = new Fl_Window(320, 240, "Simple FLTK Window");
window->end();
window->show();
return Fl::run();
}
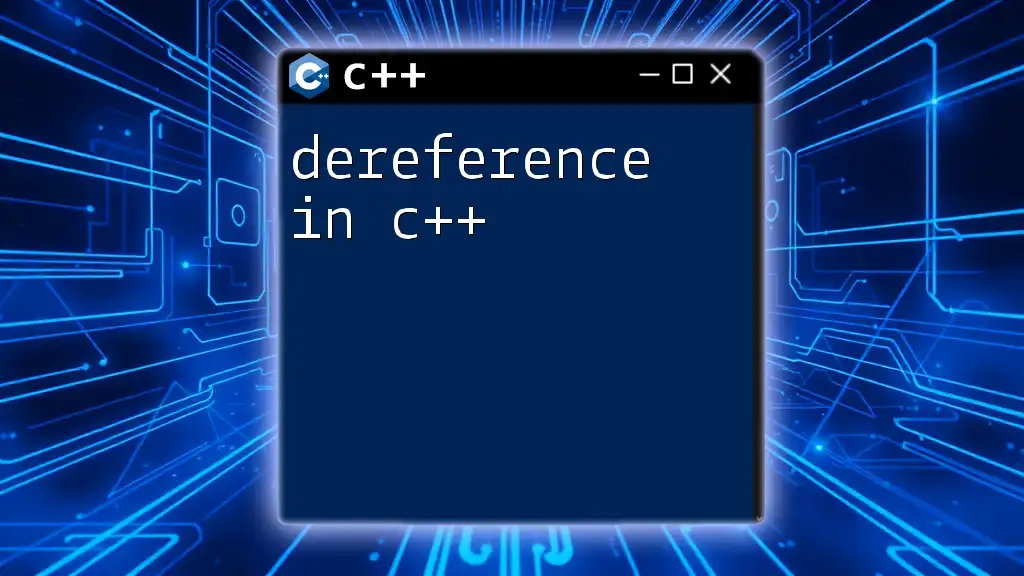
Designing a User-Friendly Interface
Principles of User Interface Design
Creating a user-friendly interface involves adhering to certain principles. Simplicity and clarity in design make it easier for users to navigate applications. It's essential to minimize unnecessary elements that could overwhelm users.
Consistency is another key factor; a cohesive design helps users build a mental model of the application, allowing them to predict how to interact with it. Implementing feedback mechanisms, such as visual or auditory notifications, informs users about their actions and system responses.
Accessibility Considerations
In today's diverse environment, accessibility is vital for making interfaces usable for everyone. This means integrating features that cater to users with various abilities, such as screen reader support, keyboard navigation, and adjustable text sizes. C++ UI developers should prioritize accessibility to ensure inclusivity.
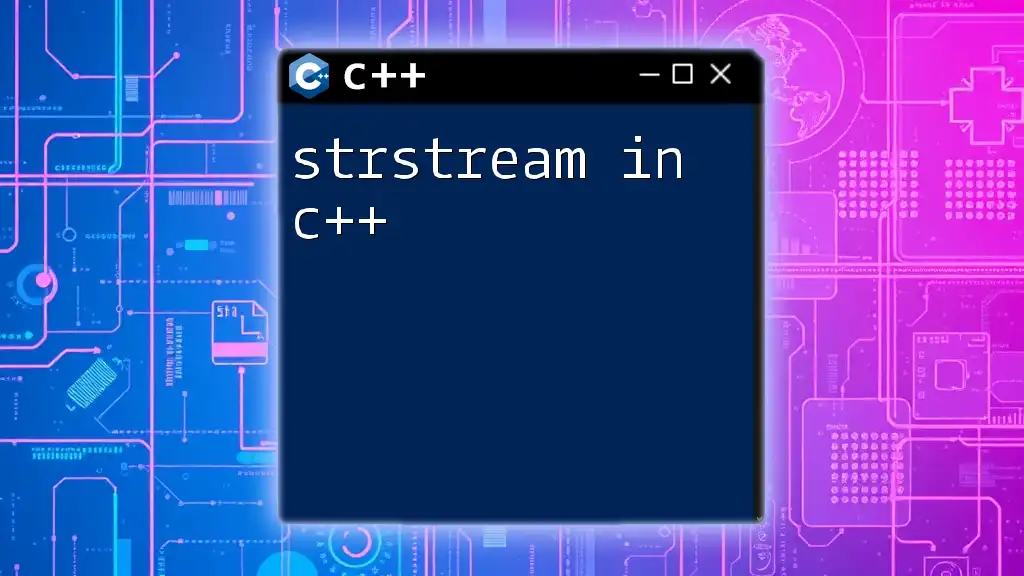
Common User Interface Components
Buttons
Buttons are fundamental components of any user interface. In C++, creating and managing buttons involves framework-specific implementations. They usually trigger functions or commands based on user interaction.
Text Boxes and Input Fields
Text boxes and input fields are essential for accepting user input. This includes gathering data, like names or search queries. C++ frameworks provide built-in methods to handle user input and validate it for correctness.
Menus and Navigation Bars
Effective structuring of navigation through menus and navigation bars is crucial for enhancing user experience. A well-designed navigation scheme allows users to find information quickly and efficiently.
Forms and Data Entry Fields
When it comes to data entry, understanding how to effectively validate user input is paramount. In C++, this typically involves checking user inputs for correctness, format, and required conditions before processing the data.
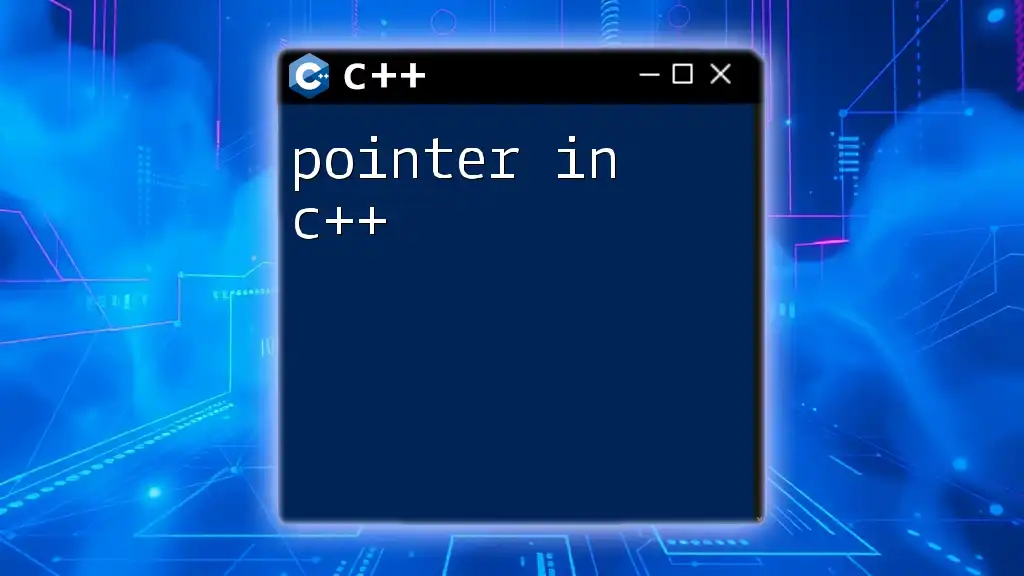
Event Handling in User Interfaces
Understanding Event-Driven Programming
Event-driven programming is a paradigm where the flow of the program is determined by events, such as user actions or messages from other programs. This approach is essential in UI development, as it allows applications to respond to user inputs dynamically.
Implementing Event Handlers in C++
Event handlers enable developers to define responses to specific user actions. For instance, with Qt, setting up a button click event can be implemented as follows:
QObject::connect(button, &QPushButton::clicked, []() {
qDebug() << "Button clicked!";
});
By connecting events to corresponding handlers, developers can create a highly interactive user experience.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Testing and Debugging User Interfaces
Importance of Testing UI Components
To ensure reliability and usability, testing UI components is vital. Regular user acceptance testing (UAT) helps gauge whether the interface meets the expectations and needs of users. Automated testing can also streamline the process, allowing for efficient checks of interface functionality.
Common Debugging Techniques in C++ UI Development
C++ developers often utilize debugging tools to identify and resolve issues in UI applications. Techniques include using integrated development environment (IDE) debuggers, memory checking tools, and manual testing approaches. This ensures that the application is stable and performs as expected.
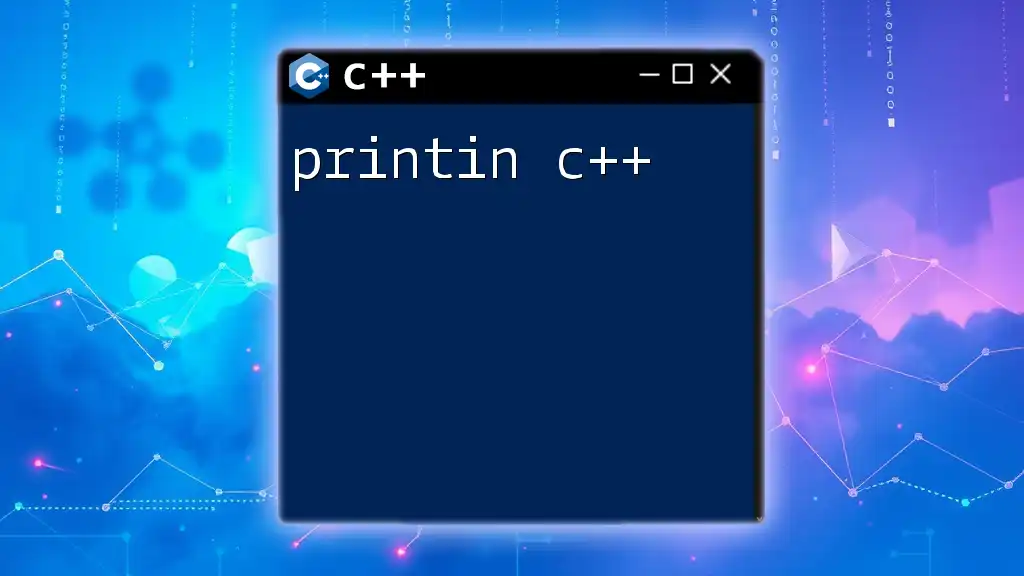
Conclusion
In summary, the user interface in C++ is a multifaceted area that combines design principles, technical implementation, and user experience considerations. By understanding the various types of interfaces, selecting the right framework, and applying sound design principles, developers can create effective user interfaces that enhance user satisfaction. Aspiring C++ UI developers should continuously experiment and learn, leveraging the many resources available to master this dynamic field.
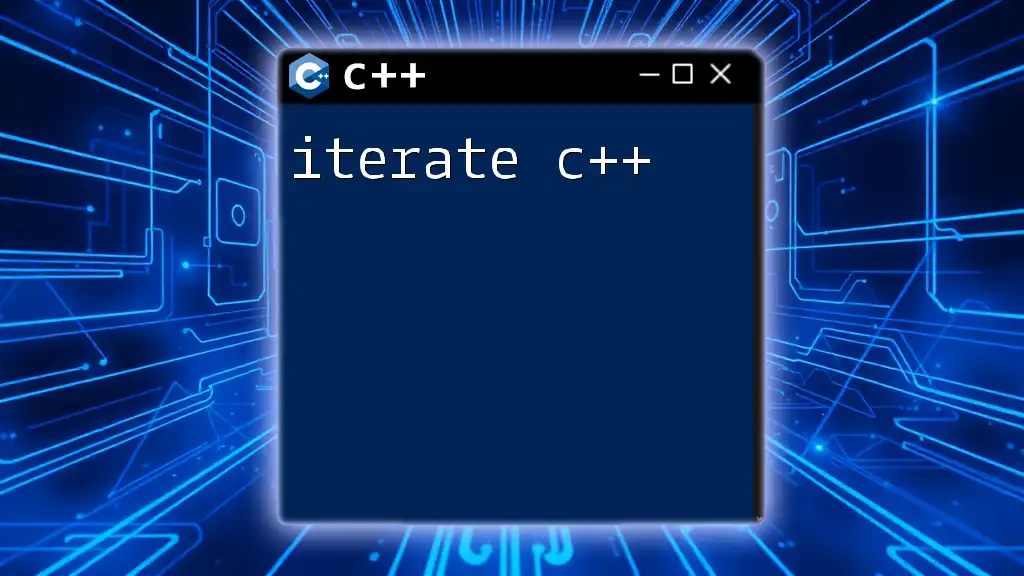
Additional Resources
To further your understanding of user interfaces in C++, consider exploring books on UI design, online courses focusing on C++ frameworks, and engaging with online communities where developers share insights and solutions. These resources can provide additional context and support as you pursue your journey in C++ user interface development.