Dereferencing in C++ is the process of accessing the value that a pointer points to by using the dereference operator (`*`).
Here's a code snippet demonstrating dereferencing:
#include <iostream>
int main() {
int num = 10;
int* ptr = # // Pointer to num
std::cout << "Value of num via dereferencing ptr: " << *ptr << std::endl; // Dereference ptr to get the value of num
return 0;
}
What is Dereferencing?
Definition of Dereferencing
Dereferencing is the process of accessing the value that a pointer points to. It allows you to retrieve or modify the data stored at that memory address. Think of a pointer as a street address: the pointer itself is the address, and dereferencing allows you to enter the house at that address to interact with the contents inside.
Pointer Basics
Before diving deeper into dereferencing, it's essential to understand pointers. In C++, a pointer is a variable that stores the address of another variable. Here’s a simple example of how pointers work:
int num = 10;
int* ptr = # // ptr now stores the address of num
In this snippet, `ptr` is a pointer to the integer variable `num`, which holds the value `10`. The `&` operator is used to obtain the address of `num`.

Understanding the Dereference Operator
Syntax of the Dereference Operator
The dereference operator is denoted by the symbol `*`. It enables you to access the value stored at the memory address that a pointer points to. For instance:
int value = *ptr; // Dereferencing ptr to get the value of num
In this case, `value` will be set to `10`, as it retrieves the integer stored at the address held by `ptr`.
How the Dereference Operator Works
When you dereference a pointer, you effectively access the data stored in memory. This process is crucial for managing resource allocation, as it allows you to read or manipulate values dynamically allocated in memory. Understanding how to dereference correctly helps in scenarios like memory management, data manipulation, and even function arguing.

Practical Examples of Dereferencing in C++
Accessing Variable Values
To directly access the value of a variable through a pointer, dereferencing is straightforward. Consider this example:
int a = 20;
int* p = &a; // Pointer p points to a
std::cout << *p; // Prints 20
When you run this code, the output will be `20`, as `*p` retrieves the value of `a`.
Modifying Values through Dereferencing
Dereferencing is not only useful for reading values but also for modifying them. Here’s how you can change a variable's value through its pointer:
*p = 30; // Changes 'a' to 30
After executing this line, the variable `a` will now hold the value `30`, demonstrating how dereferencing allows direct manipulation of variable values.
Dereferencing Multiple Types
Dereferencing is not limited to integers. You can also dereference pointers to other data types, such as characters and floats.
Dereferencing Char Pointers
For characters, consider the following example:
char ch = 'A';
char* chPtr = &ch;
std::cout << *chPtr; // Prints 'A'
The character pointer `chPtr` points to `ch`, and dereferencing it retrieves the character value.
Dereferencing Float Pointers
Similarly, you can work with float types like this:
float pi = 3.14;
float* piPtr = π
std::cout << *piPtr; // Prints 3.14
Here, `piPtr` points to `pi`, and dereferencing retrieves the floating-point value.
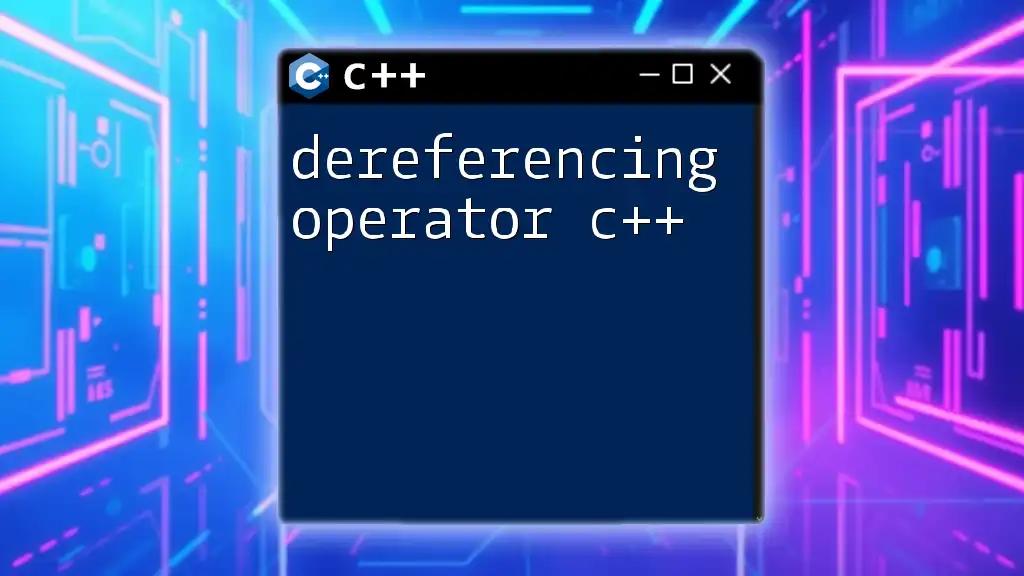
Common Scenarios for Dereferencing in C++
Array Access through Pointers
Pointers and arrays in C++ are closely related, allowing you to manipulate array elements using pointer arithmetic. For instance:
int arr[] = {1, 2, 3};
int* arrPtr = arr; // Implicit conversion to pointer
std::cout << *(arrPtr + 1); // Prints 2
By adding `1` to the pointer `arrPtr`, you access the second element of the array, highlighting the flexibility of using pointers for array access.
Functions that Use Dereferencing
One common use case for dereferencing is passing variables by reference to functions. This allows the function to modify the original variable. Here’s an example:
void updateValue(int* ptr) {
*ptr = 50; // Updates the value at the pointer address
}
int main() {
int number = 10;
updateValue(&number); // Passes the address of number
}
After calling `updateValue`, the variable `number` will now hold the value `50`, demonstrating how dereferencing enables modifications to variables outside their original scope.
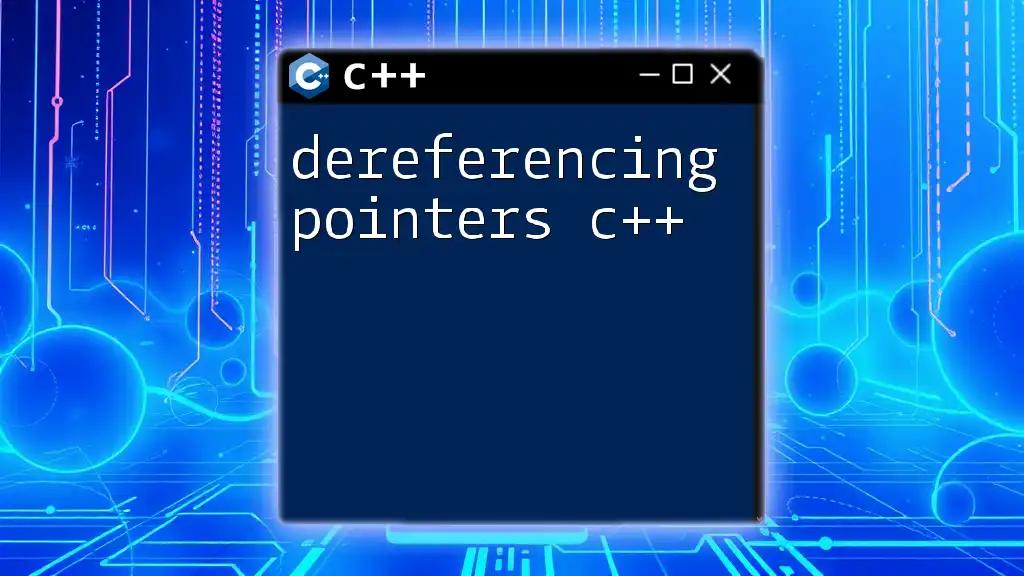
Common Mistakes with Dereferencing
Attempting to Dereference Null or Invalid Pointers
One of the most common pitfalls in dereferencing occurs when trying to dereference a pointer that is either `null` or has not been assigned a valid address. For example:
int* nullPtr = nullptr;
// std::cout << *nullPtr; // This will cause a runtime error
If you attempt to dereference `nullPtr`, it will result in a runtime error since you cannot access an invalid memory location.
Forgetting to Initialize Pointers
Another critical mistake is using uninitialized pointers. When a pointer declared but not initialized points to an unknown memory location, dereferencing it leads to undefined behavior:
int* uninitializedPtr;
// std::cout << *uninitializedPtr; // Undefined behavior
Always ensure that your pointers are initialized before dereferencing them.
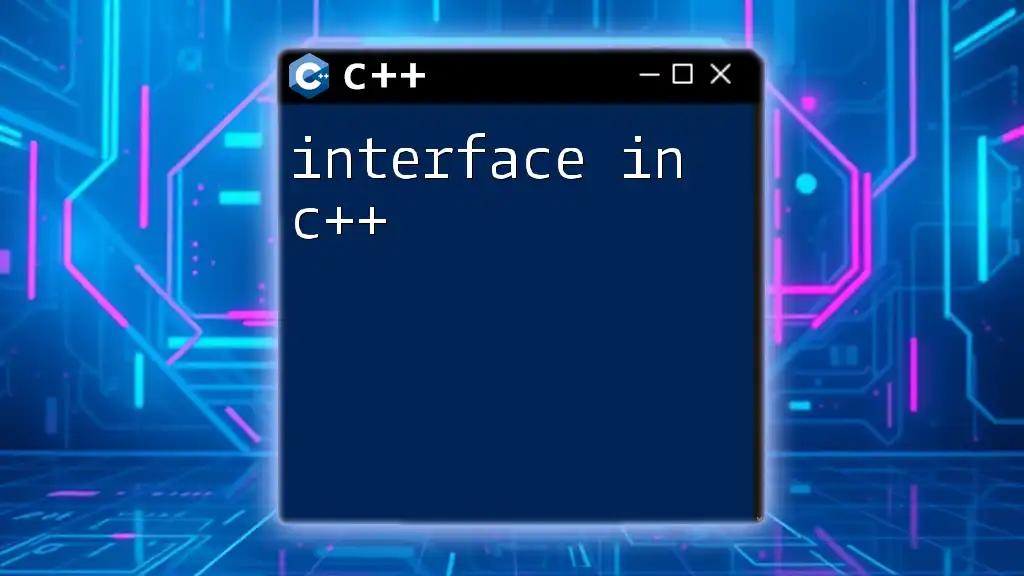
Debugging Dereferencing Issues
Tools and Techniques
When it comes to debugging dereferencing issues, tools such as Valgrind and GDB can be exceptionally helpful. These tools can help identify memory leaks, invalid memory access, and provide information on pointer values at runtime, which is invaluable for troubleshooting problems.
Tips for Diagnosing Dereferencing Issues in C++ Code
- Check Pointer Initialization: Always verify that your pointers are initialized before they are dereferenced.
- Use Debugging Tools: Leverage tools like GDB to step through your code and examine pointer values.
- Read Compiler Warnings: Many modern compilers provide warnings about issues related to pointers. Pay attention to these messages.
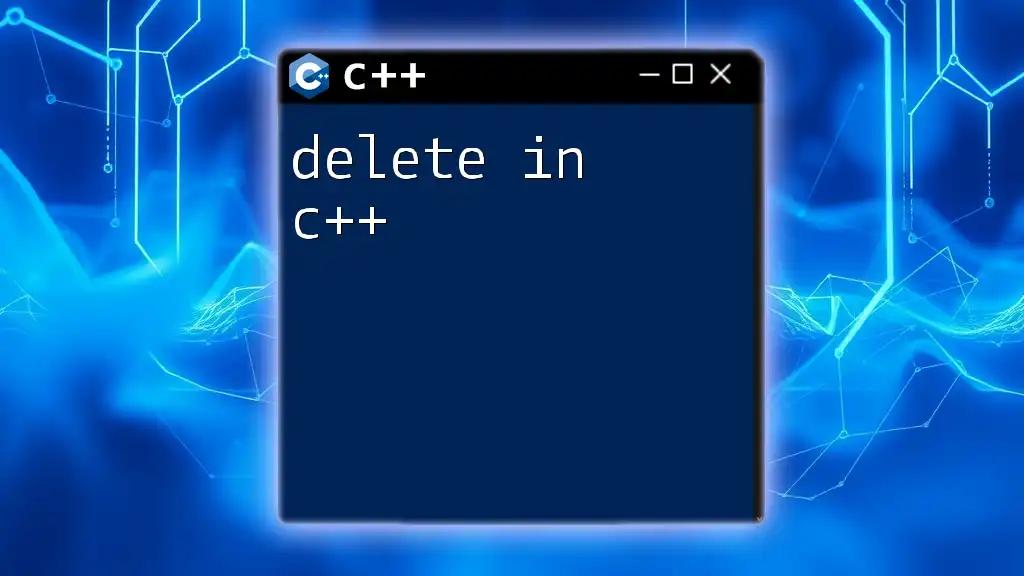
Conclusion
Dereferencing is a fundamental concept in C++ that allows you to interact with memory directly and manipulate data at any address. Understanding how to dereference properly empowers you as a programmer to write efficient and effective code. Whether you’re accessing variables, modifying arrays, or passing data to functions, knowing how to work with pointers and dereferencing will elevate your programming skills.
By practicing the techniques discussed and being mindful of the common pitfalls, you'll gain confidence in using dereferencing in your C++ projects. If you have further questions or need clarification on any points discussed, feel free to engage and ask!
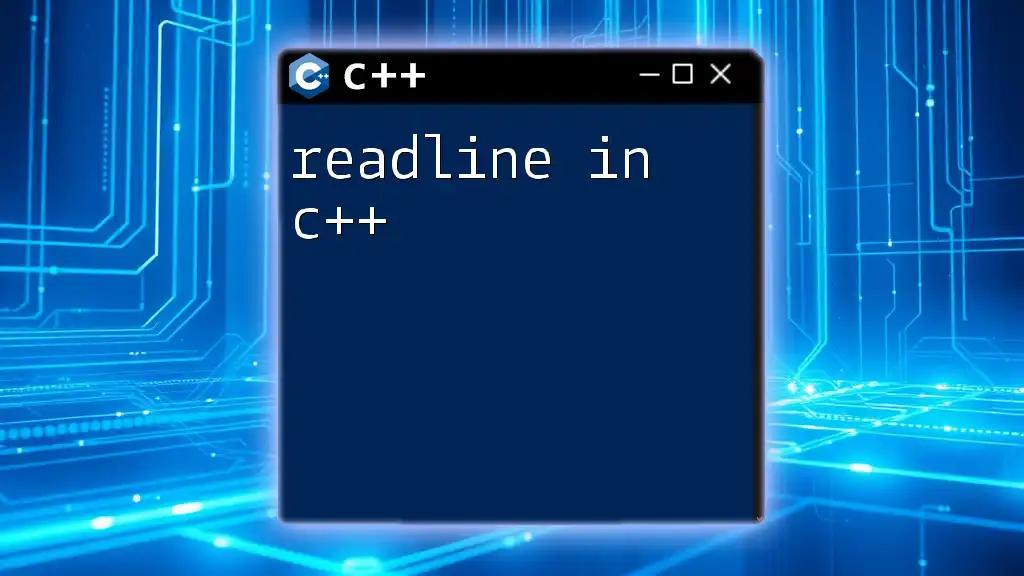
Additional Resources
For more in-depth exploration, check out the official C++ documentation on pointers and memory management. Books like "C++ Primer" and online platforms offering courses in C++ can provide further insights into mastering this powerful programming language.