In C++, a right reference (often referred to as an rvalue reference) allows you to bind a temporary object and enables move semantics, optimizing resource management.
Here’s a code snippet demonstrating the concept:
#include <iostream>
#include <utility>
void processValue(int&& value) {
std::cout << "Processing value: " << value << std::endl;
}
int main() {
processValue(42); // 42 is an rvalue
return 0;
}
Understanding C++ References
Definition of C++ References
In C++, a reference acts as an alias for another variable. By using a reference, you can manipulate the original variable rather than working with a copy, which leads to more efficient code. The syntax to declare a reference is straightforward:
int a = 10;
int &ref_a = a; // ref_a is now a reference to a
Importance of References in C++
References play an essential role in performance optimization in C++. They allow functions to modify arguments passed to them without copying the data, which is especially beneficial for large data structures. This not only reduces memory usage but also enhances execution time significantly. Unlike pointers, references are inherently safer because they cannot be null and must always be initialized upon creation.
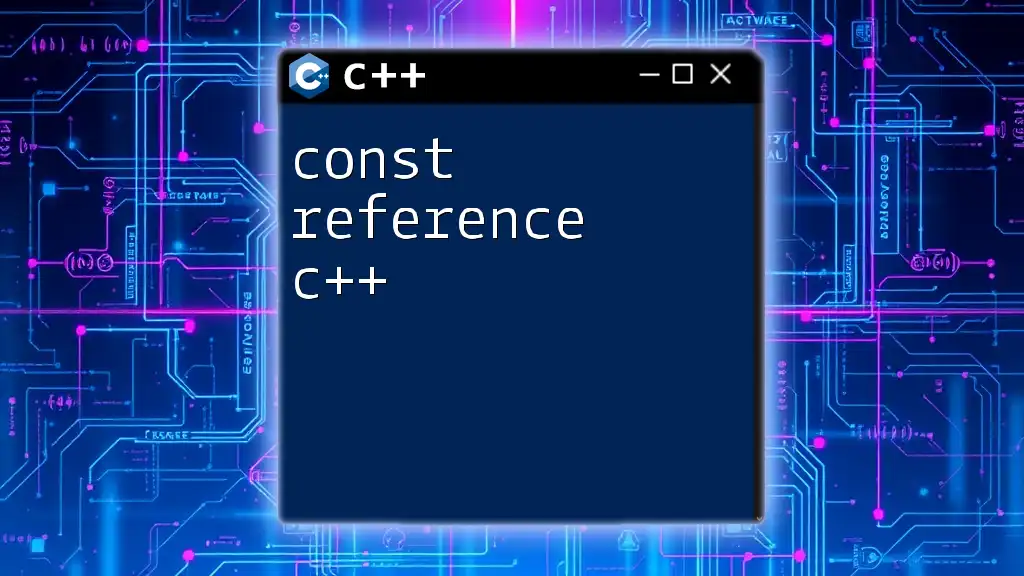
Understanding Rvalue References in C++
What are Rvalue References?
An rvalue reference is a specific type of reference that can bind to an rvalue (temporary object). An rvalue is typically an expression that does not persist beyond the expression that uses it. For example:
int getTemporaryValue() {
return 5; // This is an rvalue
}
int &&temp_ref = getTemporaryValue(); // temp_ref is an rvalue reference
The Syntax of Rvalue References
The syntax for declaring an rvalue reference involves two ampersands (`&&`):
int &&myRvalue = 10; // myRvalue is an rvalue reference to the literal 10
Rvalue references enable new ways of working with temporary objects, particularly in the context of move semantics.
The Role of Rvalue References in Move Semantics
Introduction to Move Semantics
Move semantics are a feature introduced in C++11 designed to optimize the performance of applications by allowing the resources held by temporary objects to be moved rather than copied. This is crucial for resource management, where copying can be expensive in terms of performance.
Using Rvalue References for Efficient Resource Management
Rvalue references are integral to move constructors and move assignment operators, allowing developers to effectively transfer ownership of resources. For instance, consider a simple class with a move constructor:
class MyClass {
public:
MyClass(MyClass &&other) noexcept {
this->ptr = other.ptr; // Transfer ownership
other.ptr = nullptr; // Ensure the moved-from object is left in a valid state
}
};
In this example, the move constructor eliminates the need to perform deep copies, significantly reducing overhead.
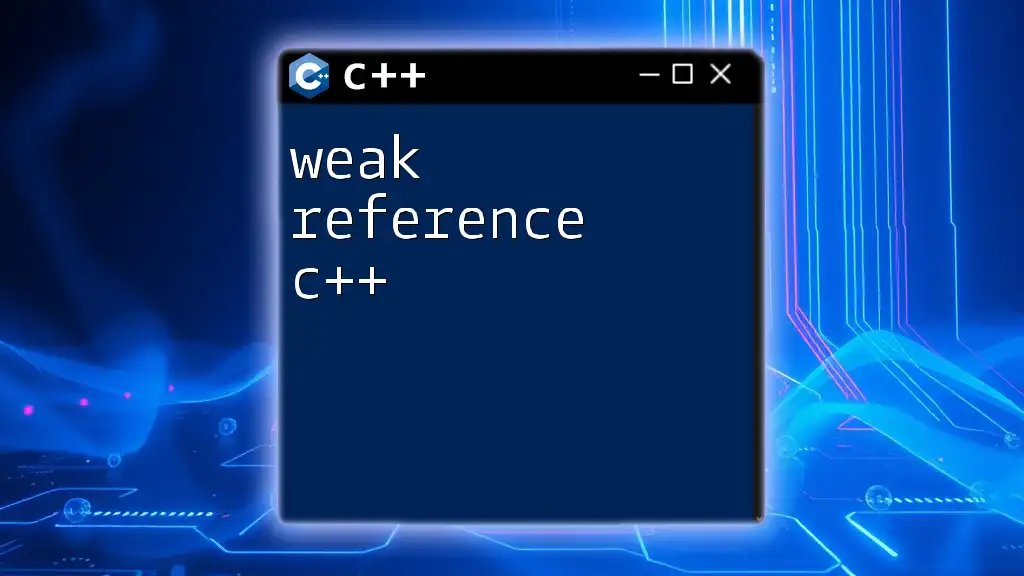
Practical Applications of Rvalue References
Implementing Move Constructors
When you implement a move constructor, you can optimize the transfer of resources by utilizing rvalue references. Here’s an example:
class Buffer {
public:
Buffer(size_t size) : data(new int[size]), size(size) {}
Buffer(Buffer &&other) noexcept
: data(other.data), size(other.size) {
other.data = nullptr; // Nullify the moved-from object's data pointer
other.size = 0; // Reset size
}
~Buffer() {
delete[] data; // Cleanup resource
}
private:
int* data;
size_t size;
};
In this implementation, if a `Buffer` object is moved instead of copied, the constructor efficiently transfers ownership of the underlying data, thereby enhancing performance.
Implementing Move Assignment Operators
Just like move constructors, move assignment operators allow you to transfer resources effectively. Here’s how you might implement a move assignment operator:
Buffer &operator=(Buffer &&other) noexcept {
if (this != &other) {
delete[] data; // Clean up existing resources
data = other.data; // Transfer ownership
size = other.size;
other.data = nullptr; // Leave the moved-from object in a valid state
other.size = 0;
}
return *this;
}
The use of the `noexcept` specifier indicates that this function does not throw exceptions, enhancing its safety and efficiency. The operator first checks for self-assignment before moving the resources.
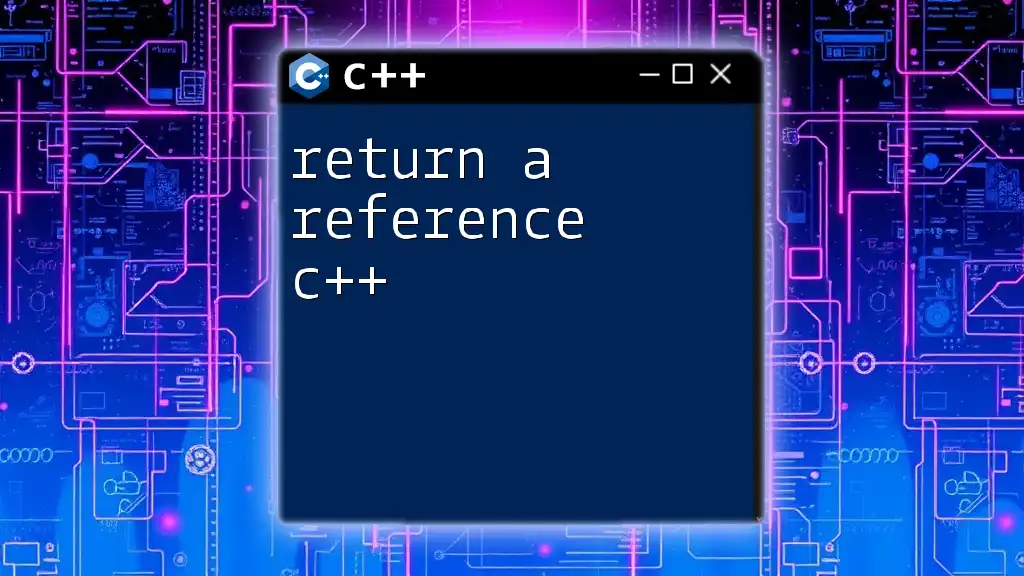
Common Pitfalls with Rvalue References
Misusing Rvalue References
One common pitfall with rvalue references is using them incorrectly, which can lead to ambiguous situations or unintended behavior. For example, mistakenly trying to bind an lvalue (a variable that has a persistent state) to an rvalue reference will result in a compilation error.
Lifetime Issues with Rvalue References
Managing the lifetime of objects is crucial when working with rvalue references. If an rvalue reference is used beyond its valid scope, it can lead to undefined behavior. For example, returning a local variable wrapped in an rvalue reference must be handled carefully:
Buffer createBuffer() {
return Buffer(1024); // Rvalue returned by value
} // The returned value is safely moved to the caller
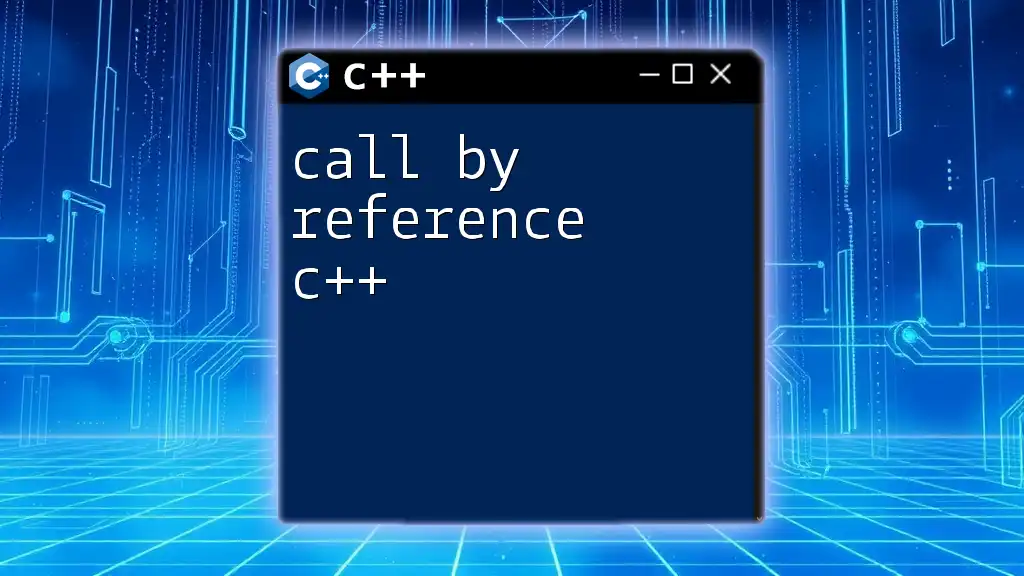
Best Practices for Using Rvalue References
When to Prefer Move Semantics
Move semantics should be your go-to choice when working with resources that are expensive to copy, such as large data structures or objects that manage their own dynamic memory. Using move semantics not only improves performance but also makes your code cleaner and more efficient.
Considerations for Overloaded Functions
Be mindful of function overload resolution involving rvalue references. It's critical to define functions correctly to ensure that the appropriate version is called:
void process(const Buffer &buf); // Copy version
void process(Buffer &&buf); // Move version
By distinguishing these overloads, you empower your classes to handle both values without sacrificing performance.
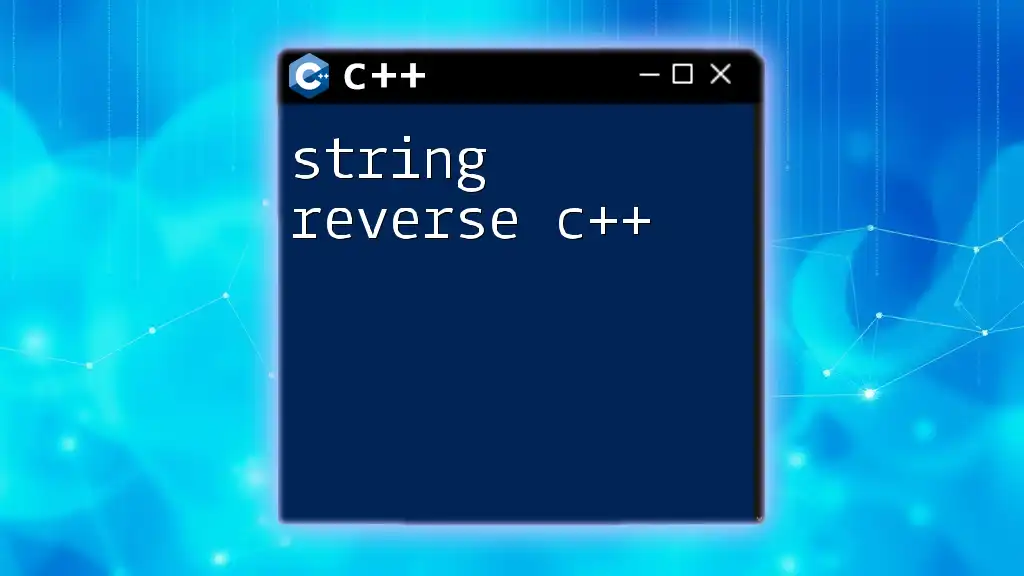
Conclusion
Summary of Key Points
Rvalue references represent a powerful feature in C++ that connects seamlessly with move semantics, enabling efficient resource management. Understanding how to effectively implement and use rvalue references can dramatically enhance the performance of your C++ applications.
Encouragement to Experiment with Rvalue References
I encourage you to apply these principles in your projects. Testing and experimenting with rvalue references will deepen your understanding and improve the quality of your code significantly.
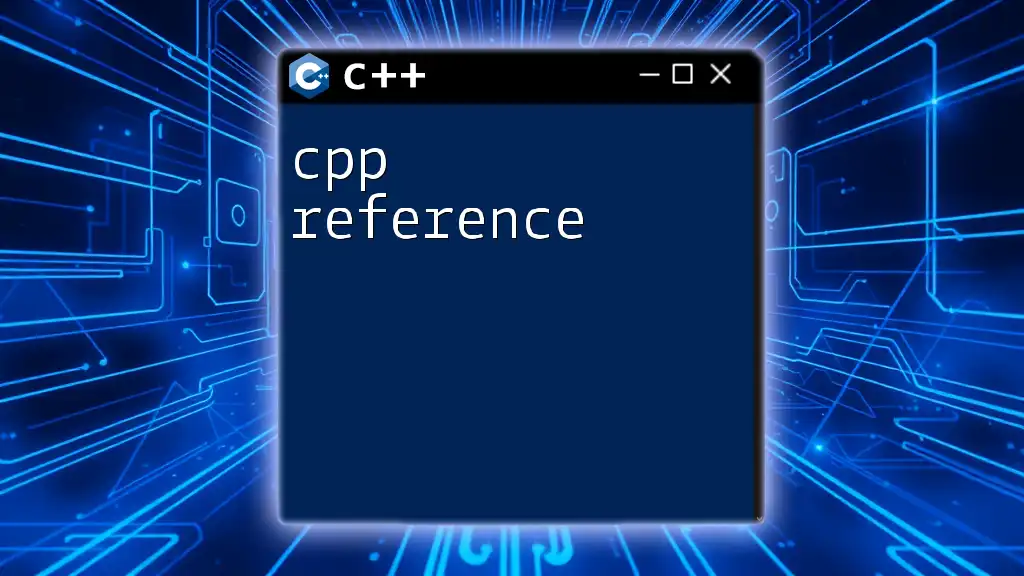
Further Reading & Resources
Recommended Books and Articles
Studying additional resources can help solidify your understanding of rvalue references and move semantics. Exploring books such as "Effective Modern C++" by Scott Meyers can provide insights and best practices.
Online Communities and Forums
Engage with the C++ community through forums and discussion platforms where you can ask questions, share knowledge, and gain new perspectives on the use of rvalue references and other advanced C++ features.