A weak reference in C++ allows you to create a non-owning reference to an object managed by a `std::shared_ptr`, preventing circular references and enabling the object to be deleted when no strong references remain.
#include <iostream>
#include <memory>
class MyClass {
public:
MyClass() { std::cout << "MyClass created\n"; }
~MyClass() { std::cout << "MyClass destroyed\n"; }
};
int main() {
std::shared_ptr<MyClass> sharedPtr = std::make_shared<MyClass>();
std::weak_ptr<MyClass> weakPtr = sharedPtr;
if (auto lockedPtr = weakPtr.lock()) { // Check if the object is still alive
std::cout << "Object is still alive\n";
} else {
std::cout << "Object has been destroyed\n";
}
sharedPtr.reset(); // Reset the shared_ptr, destroying the object
if (auto lockedPtr = weakPtr.lock()) {
std::cout << "Object is still alive\n";
} else {
std::cout << "Object has been destroyed\n";
}
return 0;
}
What are Weak References?
A weak reference in C++ is an essential concept in memory management, particularly in the context of smart pointers. Unlike strong references, which maintain ownership of the pointed-to object, weak references do not contribute to the reference count. This makes them crucial in avoiding unwanted memory retention and allowing for more flexible memory management.
Comparison with Strong References and Raw Pointers
-
Strong References: These are typically represented by `std::shared_ptr`. When you create a `shared_ptr`, it increases the reference count of the object it points to, ensuring that the object remains alive as long as there are strong references to it.
-
Raw Pointers: Raw pointers (e.g., `Type*`) do not manage object lifetimes. This can result in dangling pointers if an object is deleted when other pointers still reference it, leading to undefined behavior.
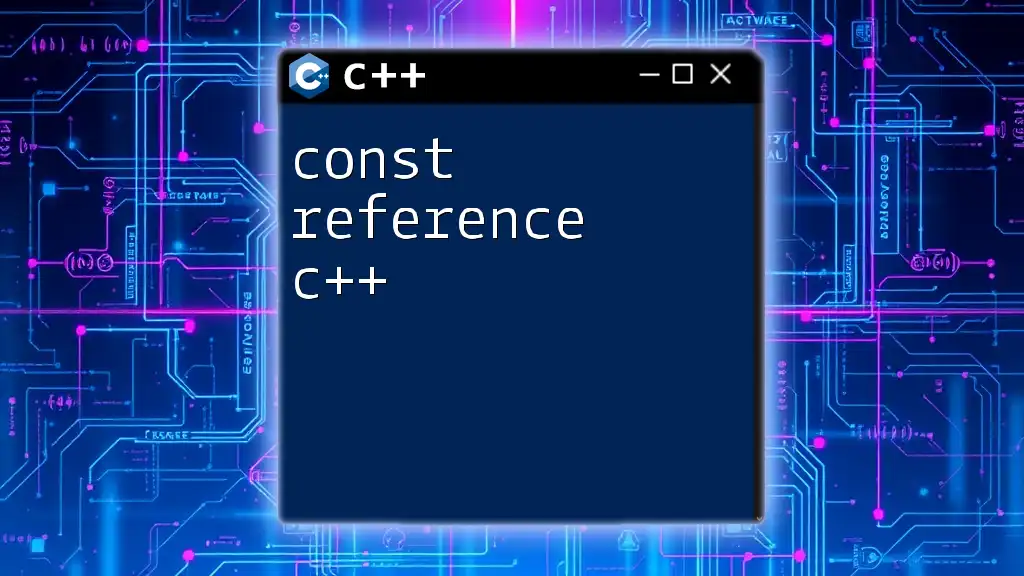
When to Use Weak References
Weak references are particularly useful in several scenarios:
-
Avoiding Circular References: When two objects reference each other using strong references, they can lead to memory leaks, as neither object's reference count will drop to zero.
-
Managing Shared Resources: In cases where an object should not extend the lifetime of another object unnecessarily, weak references can provide a way to reference the object without affecting its lifecycle.
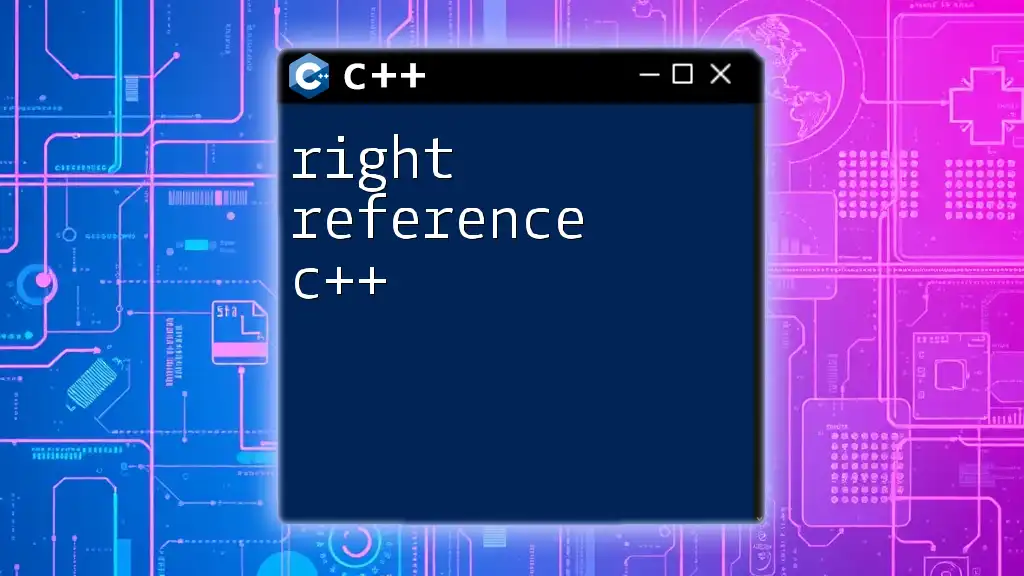
Understanding Smart Pointers in C++
Overview of Smart Pointers
C++ offers several types of smart pointers to facilitate easier and safer memory management:
-
`std::shared_ptr`: A smart pointer that allows multiple pointers to share ownership of an object. The object is deleted when the last `shared_ptr` pointing to it is destroyed.
-
`std::unique_ptr`: A smart pointer that maintains exclusive ownership of an object, preventing the object from being accidentally shared.
-
`std::weak_ptr`: A smart pointer that acts as a secondary reference to an object managed by `shared_ptr`, without affecting its reference count.
The Role of `std::weak_ptr`
The `std::weak_ptr` plays a vital role in complex data structures. By holding a weak reference to an object managed by `shared_ptr`, `weak_ptr` helps to prevent memory leaks that could occur from circular dependencies. It can be used to safely check if an object still exists before accessing it.
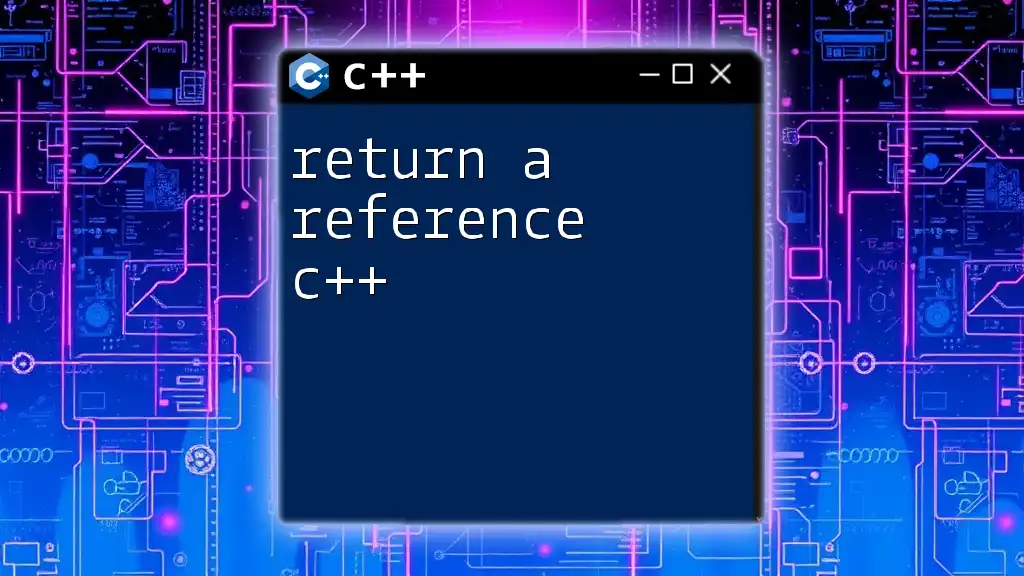
How to Use `std::weak_ptr`
Syntax and Declaration
Declaring a weak pointer in C++ is straightforward. Here's how you do it:
#include <memory>
std::weak_ptr<Type> weakPtrName;
This line reserves space for a weak pointer without holding any ownership of the object.
Creating a Weak Reference
To create a weak reference, you first need a `shared_ptr`. Here’s an example:
std::shared_ptr<Type> sharedPtr = std::make_shared<Type>();
std::weak_ptr<Type> weakPtr = sharedPtr;
In this example, `weakPtr` now holds a weak reference to the object pointed to by `sharedPtr` without increasing the reference count.
Locking Weak References
Accessing the object that a `weak_ptr` points to requires locking it, which creates a temporary `shared_ptr`. This ensures that the object is still valid before usage:
if (auto sharedPtr2 = weakPtr.lock()) {
// Use sharedPtr2 safely.
} else {
// The object no longer exists because its reference count is zero.
}
The above code snippet shows that by using `lock()`, we can check the validity of the referenced object and act appropriately.
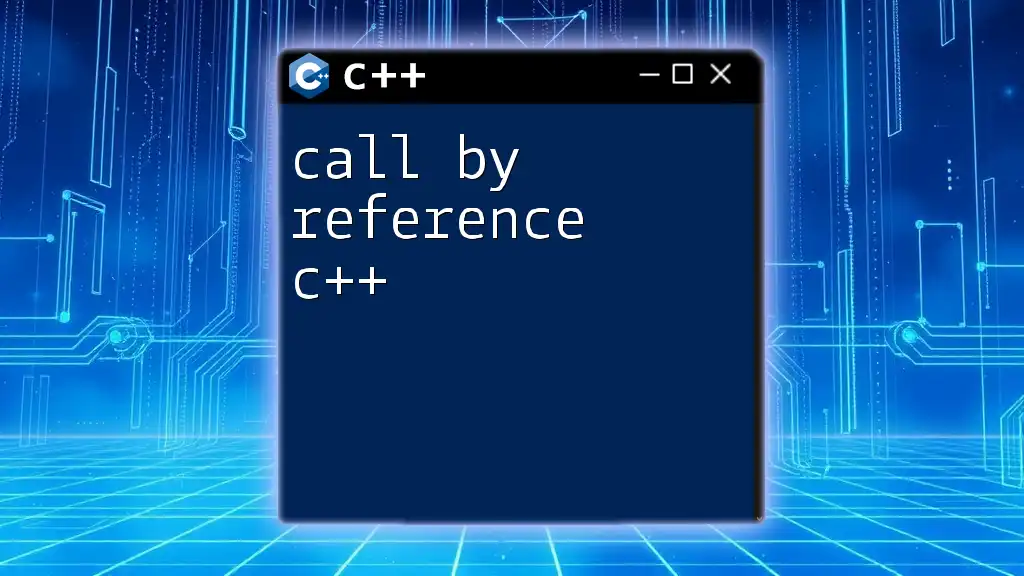
Practical Examples of Weak Pointers
Example 1: Avoiding Circular References
Consider two classes that reference each other:
class A;
class B {
public:
std::shared_ptr<A> ptrA;
};
class A {
public:
std::weak_ptr<B> ptrB; // Use weak_ptr to avoid circular reference
};
In this scenario, class `A` contains a `weak_ptr` to class `B`, preventing a situation where both classes prevent each other's deletion due to each holding a strong reference to the other.
Example 2: Caching and Object Lifetime Management
Using `weak_ptr` can significantly enhance caching mechanisms. Consider the following simple cache implementation:
#include <unordered_map>
#include <memory>
class Object {
// Object definition
};
class Cache {
std::unordered_map<int, std::shared_ptr<Object>> items;
public:
std::shared_ptr<Object> retrieve(int id) {
auto it = items.find(id);
if (it != items.end()) {
return it->second;
}
return nullptr; // or create new object
}
};
In this code, we can extend this by adding weak references to cached items so that they can be automatically cleared when not in use, freeing up resources without manual intervention.
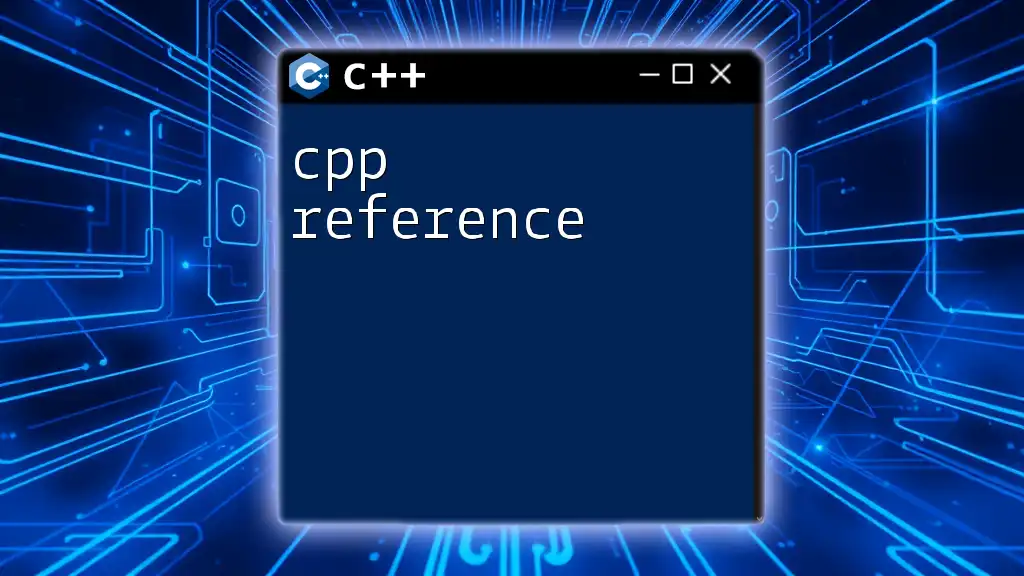
Best Practices When Using Weak References
Use Cases for Weak References
Weak references should be utilized in situations where it is essential to prevent strong ownership from influencing an object’s lifetime, such as:
- Event Listeners: Having listeners keep weak pointers to prevent event callbacks from keeping listeners alive unnecessarily.
- Observer Patterns: Allowing observers to be removed when the subject (observed entity) no longer requires them.
Common Pitfalls
While using `std::weak_ptr`, developers should be mindful of the following pitfalls:
-
Overusing Weak Pointers: Relying heavily on `weak_ptr` can complicate the design of your code and may lead to inefficient memory use.
-
Not Check Expiration: It is crucial to check if a weak pointer is still valid. Failing to do so can lead to runtime errors when attempting to access an expired object.
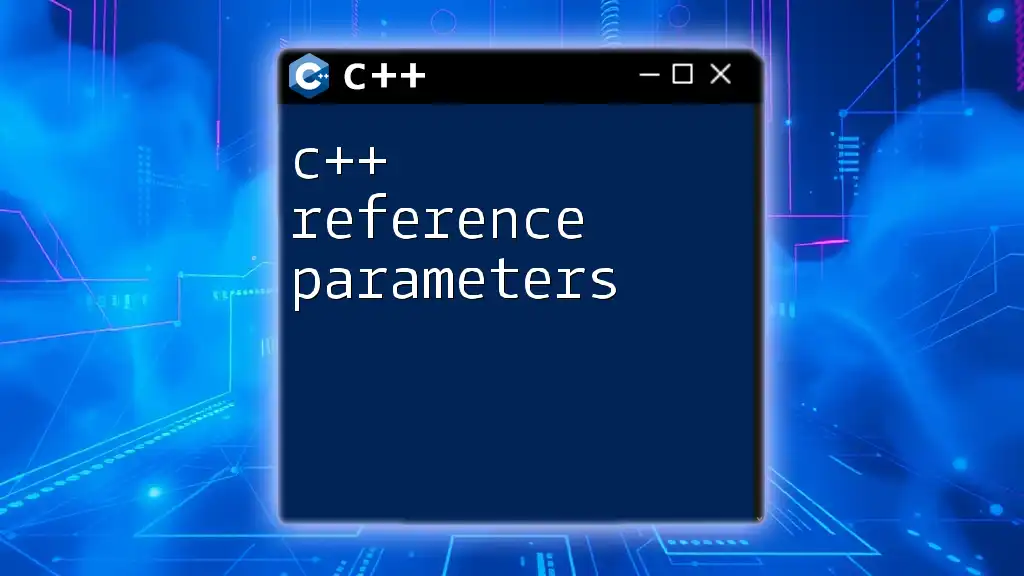
Performance Considerations
Cost of Using Weak References
While `std::weak_ptr` helps with memory management, it comes with a slight overhead in terms of memory usage and performance due to reference counting. These include:
- Increased memory footprint due to additional control blocks.
- Small performance overhead when locking to access the underlying object.
Profiling and Optimization Tips
To ensure optimal usage of weak references:
- Use profiling tools to analyze memory consumption and identify potential bottlenecks.
- Always evaluate if a raw pointer may suffice over a weak pointer to prevent unnecessary complexity when managing object lifetimes.
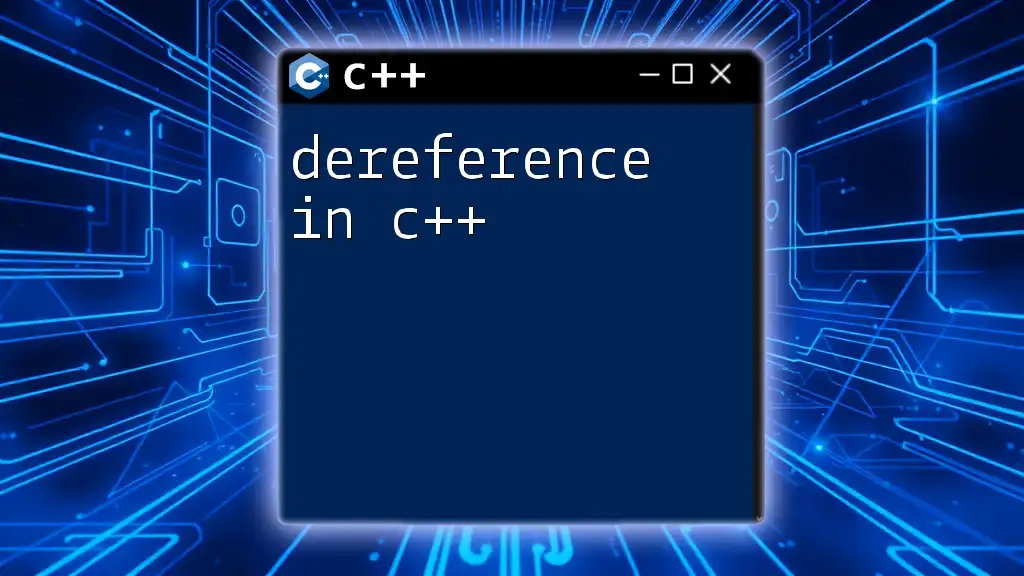
Conclusion
In summary, weak references in C++ are a powerful tool for memory management, allowing developers to design more robust and efficient systems. By recognizing when to use `std::weak_ptr`, programmers can prevent memory leaks and build cleaner codebases focused on lifecycle management. This article has highlighted important concepts, practical examples, and best practices that can help you leverage weak references in your C++ projects effectively. Now, it's time to experiment with `weak_ref` in your own code!
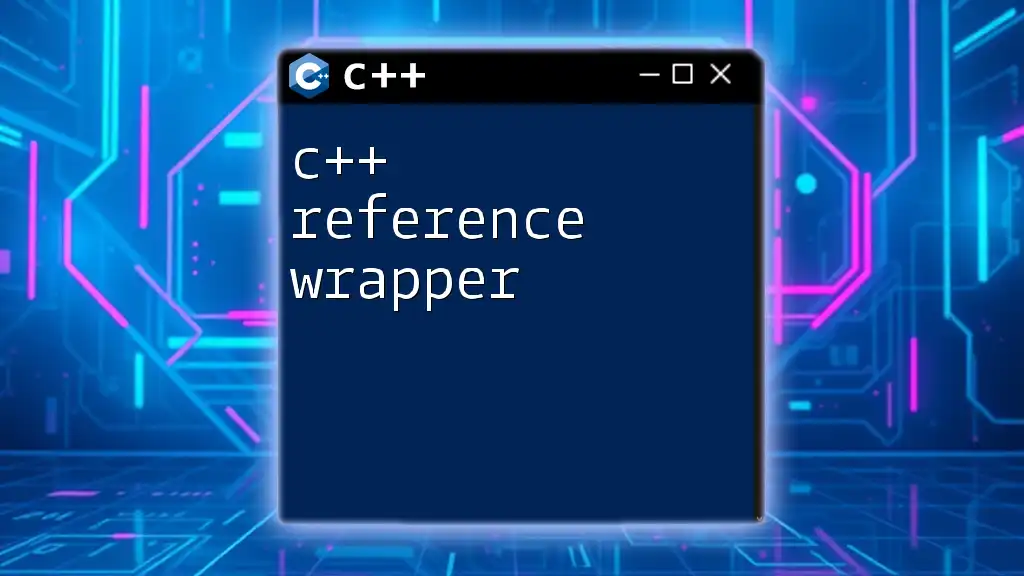
Additional Resources
For those looking to deepen their understanding of smart pointers and weak references in C++, consider the following resources:
- Books focusing on modern C++ standards and best practices.
- Comprehensive articles and documentation from the C++ standards organization and community forums.
- Online coding platforms for hands-on tutorials and examples.