The `CreateThread` function in C++ is used to create a new thread that runs concurrently with the calling thread, allowing for multitasking within applications.
Here's a simple code snippet that demonstrates its usage:
#include <windows.h>
#include <iostream>
// Function to be run in the new thread
DWORD WINAPI MyThreadFunction(LPVOID lpParam) {
std::cout << "Hello from the new thread!" << std::endl;
return 0;
}
int main() {
// Create a new thread
HANDLE hThread = CreateThread(
NULL, // default security attributes
0, // default stack size
MyThreadFunction, // thread function name
NULL, // argument to thread function
0, // default creation flags
NULL); // receive thread identifier
// Wait for the thread to finish
WaitForSingleObject(hThread, INFINITE);
// Close the thread handle
CloseHandle(hThread);
return 0;
}
What is `CreateThread`?
Definition and Purpose
`CreateThread` is a powerful Windows API function that allows developers to create a new thread within the context of a running application. Threads are essential for achieving concurrency and parallelism, enabling programs to perform multiple tasks simultaneously. By leveraging threads, developers can enhance application responsiveness and efficiency, allowing for smoother user experiences and more robust background operations.
Key Benefits of Using `CreateThread`
-
Improved Application Performance: Utilizing multiple threads can significantly decrease the time taken to execute concurrent tasks. For instance, a multithreaded application can perform I/O operations in one thread while processing data in another, leading to better utilization of CPU resources.
-
Better Resource Utilization: Threads share the same memory space, which allows them to quickly access shared data without the overhead of inter-process communication (IPC). This leads to enhanced performance, especially in memory-intensive applications.
-
Background Processing Capabilities: With `CreateThread`, applications can offload time-consuming tasks to background threads, providing a smoother experience for users as the main thread remains responsive.

The Syntax of `CreateThread`
Detailed Breakdown of Parameters
`CreateThread` comes with a specific syntax that includes several parameters, each serving a unique purpose:
-
`lpThreadAttributes`: This parameter specifies thread security attributes. If you don't require specific security settings, you can pass `NULL`.
-
`dwStackSize`: This defines the initial size of the stack for the new thread. If you set it to zero, the default size will be used.
-
`lpStartAddress`: This required parameter is a pointer to the function that will serve as the entry point for the thread. The function must adhere to the `LPTHREAD_START_ROUTINE` type, which returns a DWORD and accepts a pointer (`LPVOID`) as its parameter.
-
`lpParameter`: This optional parameter can pass data to the thread function. It can be anything, like a pointer to a structure containing multiple pieces of data.
-
`dwCreationFlags`: Here, you can specify creation flags that modify thread behavior. Common options include `CREATE_SUSPENDED`, which starts the thread in a suspended state.
-
`lpThreadId`: This is an optional pointer that can receive the thread identifier, making it easier to manage or reference the thread later.
Example of CreateThread Syntax
The basic syntax looks as follows:
HANDLE CreateThread(
LPSECURITY_ATTRIBUTES lpThreadAttributes,
SIZE_T dwStackSize,
LPTHREAD_START_ROUTINE lpStartAddress,
LPVOID lpParameter,
DWORD dwCreationFlags,
LPDWORD lpThreadId
);

How to Use `CreateThread`
Step-by-Step Guide
To effectively use `CreateThread`, you need to start by setting up your development environment. Ensure you have the necessary Windows libraries included in your project. This typically involves including `<windows.h>` at the beginning of your source file.
Example Code Snippet
Below is a concise example demonstrating how to use `CreateThread` to create a new thread:
#include <windows.h>
#include <iostream>
DWORD WINAPI ThreadFunction(LPVOID lpParam) {
std::cout << "Hello from the thread!" << std::endl;
return 0;
}
int main() {
HANDLE threadHandle;
DWORD threadId;
threadHandle = CreateThread(
NULL, // Default security attributes
0, // Default stack size
ThreadFunction, // Thread function name
NULL, // No parameters to thread function
0, // Default creation flags
&threadId); // Returns the thread identifier
// Wait until the thread has completed
WaitForSingleObject(threadHandle, INFINITE);
// Close the thread handle
CloseHandle(threadHandle);
return 0;
}
Explanation of the Example
In this example, we define a simple thread function, `ThreadFunction`, which outputs a message to the console. Inside the `main` function, we call `CreateThread` to create a new thread that executes `ThreadFunction`.
-
The `WaitForSingleObject` function is called to make sure that the main thread waits for the created thread to finish its execution before proceeding. This is crucial for resource management.
-
Finally, `CloseHandle` is used to release the thread handle, preventing memory leaks.

Error Handling with `CreateThread`
Common Error Codes
When working with `CreateThread`, it’s vital to incorporate error handling. If thread creation fails, the function returns `NULL`. You can use the `GetLastError` function to retrieve more details about the failure. Common error codes include `ERROR_INVALID_PARAMETER` if any parameter is incorrect.
Example of Error Handling
Consider this additional snippet to handle errors effectively:
if (threadHandle == NULL) {
std::cerr << "Error creating thread: " << GetLastError() << std::endl;
}
Incorporating error checks like this helps debug issues promptly and ensures your application is reliable.

Thread Termination
Graceful vs. Forced Termination
It's crucial to terminate threads properly to avoid resource leaks and ensure data integrity. You have two primary approaches: graceful and forced termination.
- Graceful Termination: This involves threads completing their tasks naturally before exiting, which is typically the best practice.
- Forced Termination: You can use `TerminateThread`, but this is dangerous as it does not allow the thread to release resources or save state.
Example of Graceful Termination
Here’s an enhanced mechanism for thread completion, ensuring that the thread knows when to exit:
struct ThreadData {
bool shouldProceed;
};
DWORD WINAPI ThreadFunction(LPVOID lpParam) {
ThreadData* data = (ThreadData*)lpParam;
while (data->shouldProceed) {
// Perform thread-related work
}
return 0;
}
In this code, you can control the thread’s lifecycle using the `shouldProceed` flag, allowing for a clean exit.

Best Practices for Using `CreateThread`
Thread Management
Managing multiple threads effectively is essential for maximizing performance and avoiding issues like race conditions. Always ensure that shared resources are adequately synchronized, as unsynchronized access can lead to unpredictable behavior.
Synchronization Techniques
To synchronize access to shared resources, consider using various mechanisms such as:
- Mutexes: Guarantee exclusive access to resources.
- Events: Are used to signal between threads.
Using these tools can vastly improve the stability and reliability of your applications.

Conclusion
In summary, mastering `CreateThread` in C++ opens new doors for building efficient and responsive applications. By understanding its parameters, implementation, error handling, and management strategies, you can effectively harness the power of multithreading. As you practice and explore threading further, consider expanding your knowledge on synchronization techniques and advanced threading models.
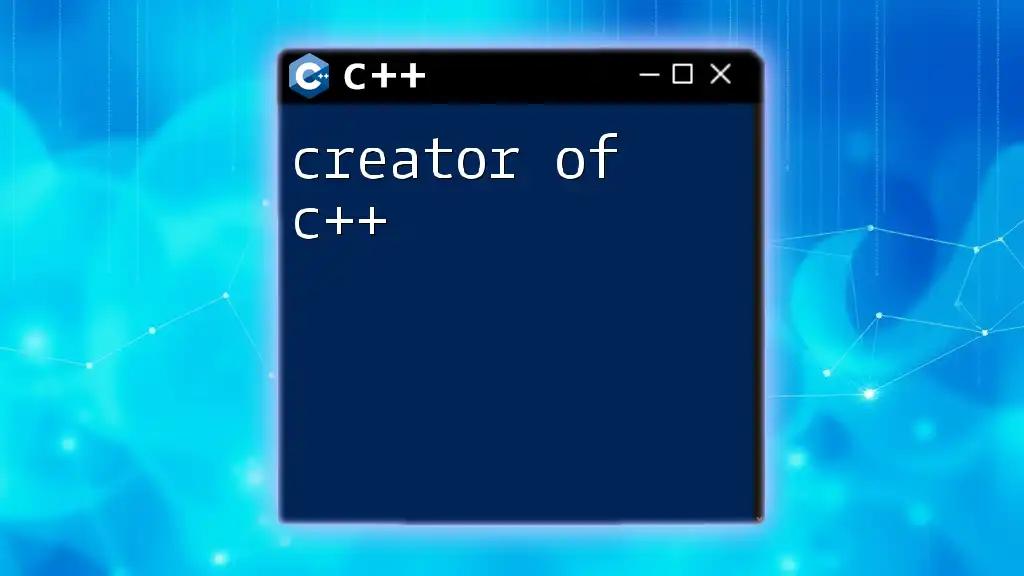
Call to Action
Experiment with threading in your own projects, and don't hesitate to share your experiences or questions in the comments! Stay tuned for more tips and insights on mastering C++ programming and threading techniques.