The `getcurrenttime` function in C++ can be implemented using the `<chrono>` library to fetch the current time in a concise manner. Here's a simple code snippet demonstrating how to achieve this:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << "Current time: " << std::ctime(¤tTime);
return 0;
}
Understanding Time Representation in C++
What is Time in C++?
In C++, time is a crucial data type used for various programming applications, such as logging events, scheduling tasks, or simply displaying the current date and time. Understanding how C++ represents time, especially differentiating between system time (the time returned by the operating system) and wall clock time (the time perceivable by users) is vital.
It is also essential to be aware of time zones since they can significantly affect how time is represented and displayed in applications, especially those that operate globally.
C++ Date and Time Libraries
C++ offers two primary libraries for handling date and time: `ctime` and `chrono`.
- `ctime` is a part of the C standard library, allowing for basic time operations. It's often simpler but may lack flexibility and precision.
- `chrono`, introduced in C++11, provides a more robust approach through its support for high-resolution time measurements, making it the preferred choice for modern applications.
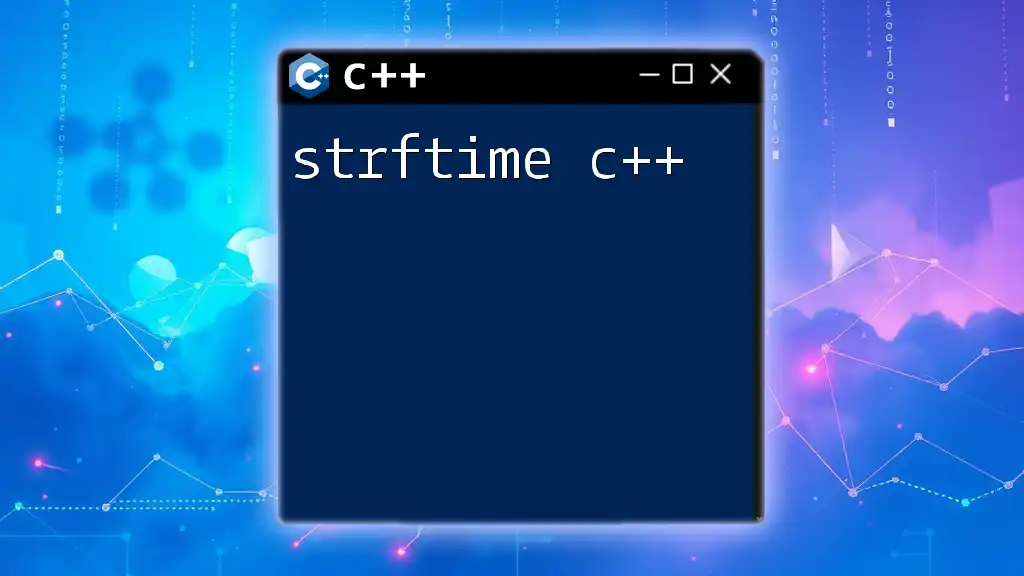
Getting Current Time Using C++ Commands
Using the `ctime` Library
To get current time quickly in C++, the `ctime` library is often the starting point.
Explanation and Setup
To utilize this library, include it in your C++ source file:
#include <ctime>
Code Snippet
Here’s a simple example to illustrate how to fetch and display the current time using this library:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(0); // Get current time
std::cout << "Current time: " << std::ctime(¤tTime); // Convert to string
return 0;
}
Explanation of Code
In this code snippet, we call `std::time(0)`, which retrieves the current time as a `time_t` object—the number of seconds since January 1, 1970 (the Unix epoch). The `std::ctime()` function then converts this `time_t` object into a human-readable string format. This approach is beneficial for quickly logging or displaying current times in systems that require basic time information.
Using the `chrono` Library
Introduction to `chrono`
The advent of the `chrono` library marks a significant enhancement in C++ time management capabilities, providing not just current time retrieval but also features for precise duration calculations and time points.
Fetching Current Time with `chrono`
To employ the `chrono` library effectively, include it in your project as follows:
#include <chrono>
Code Snippet
Using `chrono` to get the current time is straightforward:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now(); // Get current time
std::time_t currentTime = std::chrono::system_clock::to_time_t(now); // Convert to time_t
std::cout << "Current time: " << std::ctime(¤tTime);
return 0;
}
Explanation of Code
In this example, `std::chrono::system_clock::now()` captures the current point in time as a `time_point` object. We then convert this object to `time_t` using `std::chrono::system_clock::to_time_t()`, and finally, we output it in a human-readable format. The `chrono` library is preferable for applications requiring high-resolution time measurements or timing operations since it allows for precise control and easier manipulation of time values.
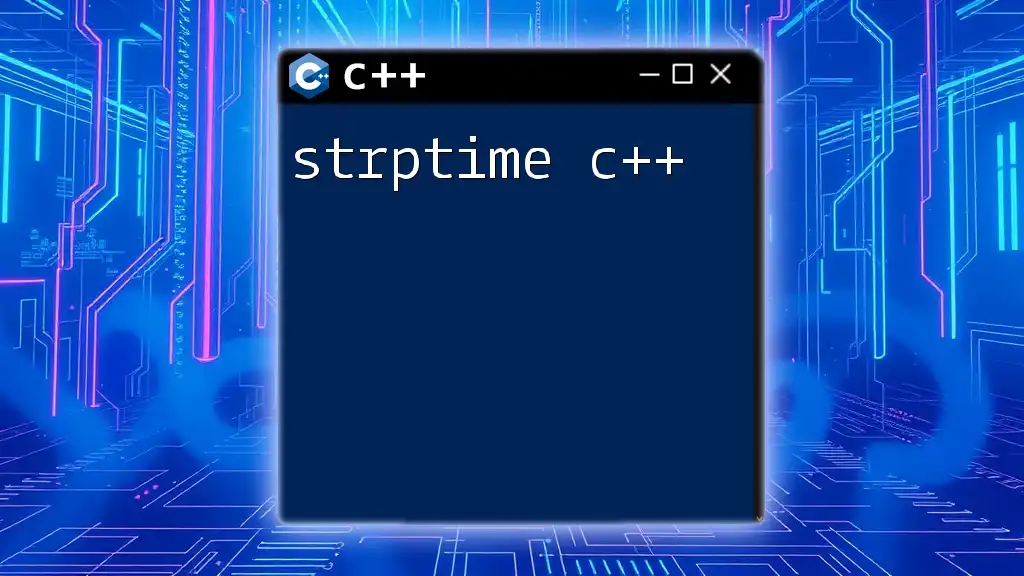
Enhancing Time Retrieval
Formatting the Current Time
For various applications, you might require the current time in a specific format. Use the `strftime` function, which allows for customizable string formatting.
Using `strftime` for Custom Formatting
To format the output of the current time, you might consider the following approach:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(0);
std::tm *tmPtr = std::localtime(¤tTime);
char buffer[80];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d %H:%M:%S", tmPtr);
std::cout << "Formatted current time: " << buffer << std::endl;
return 0;
}
Explanation of Code
Here, `std::localtime()` converts the `time_t` object to a `tm` structure, which breaks the time into its components (year, month, day, etc.). Using `strftime`, we can format the output using placeholders—`%Y` for the year, `%m` for the month, and so on—enabling tailored display formats that meet application requirements.
Example: Display Current Time in Different Time Zones
Handling time zones can be intricate, particularly when dealing with applications that operate across various regions. While `ctime` provides limited support for time zones, using options like `boost::date_time` or third-party libraries can simplify such tasks.
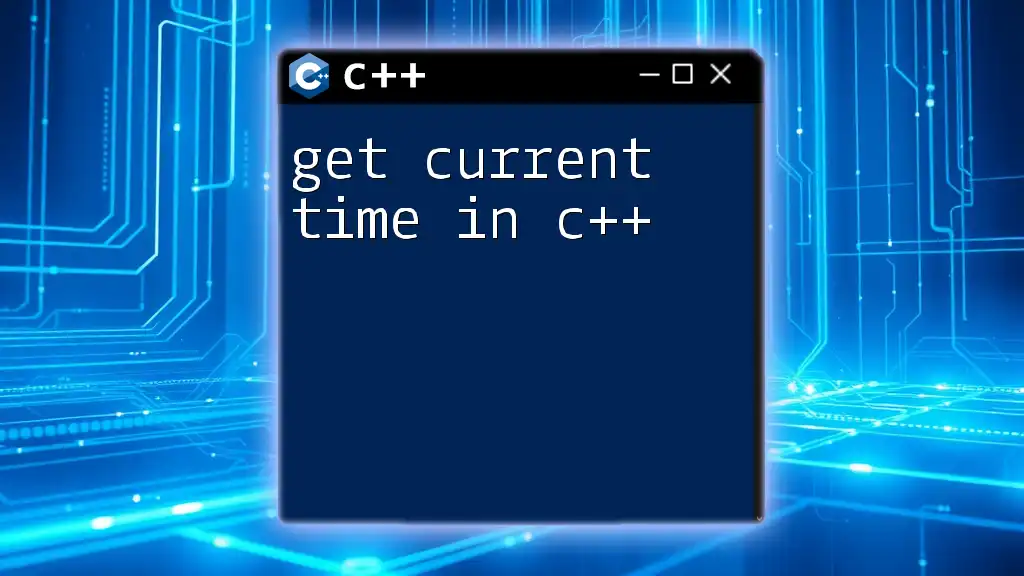
Common Pitfalls and Best Practices
Handling Time Zone Issues
A common pitfall in getting the current time arises from confusion about system time versus local time. An application fetching UTC time without considering the local settings may lead to user confusion—even errors if the application needs to interact with other time-sensitive entities.
Precision and Performance Considerations
When deciding between `ctime` and `chrono`, consider the performance implications and your application’s precision requirements. `chrono` provides more capabilities for complex timing needs, while `ctime` may suffice for simpler applications. Always choose the library that aligns best with your application requirements to ensure optimal performance and readability.
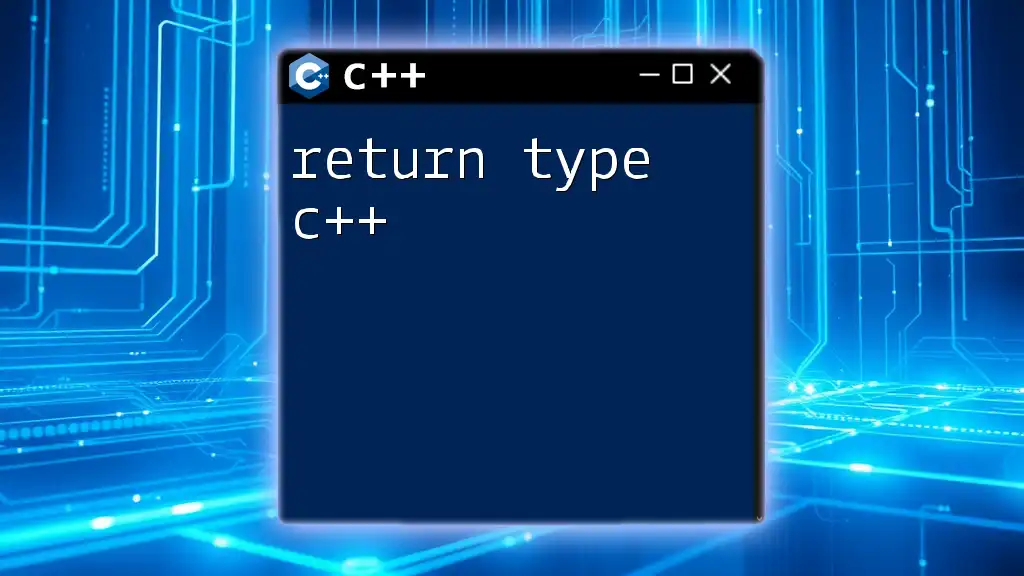
Conclusion
Understanding how to getcurrenttime c++ is essential for any developer looking to work effectively with time in C++. By leveraging the vast capabilities of both the `ctime` and `chrono` libraries, you can elegantly manage time-related data in your applications. Experiment with the provided code examples, customize the time formats to your needs, and explore further enhancements with external libraries to elevate your programming skills in C++.