To get the current time in C++, you can use the `<chrono>` library alongside `<ctime>` to retrieve and format the current system time as follows:
#include <iostream>
#include <chrono>
#include <ctime>
int main() {
auto now = std::chrono::system_clock::now();
std::time_t currentTime = std::chrono::system_clock::to_time_t(now);
std::cout << std::ctime(¤tTime);
return 0;
}
Understanding Time in C++
What is Time in Programming?
In programming, time is a fundamental concept that plays a crucial role in multiple contexts, from scheduling tasks to logging events. It generally refers to the notion of measuring or evaluating the progression of time either in terms of wall-clock time, which relates to real-world time, or system time, which the operating system uses to schedule processes.
When working with C++, developers need to access and manipulate time effectively, which is why understanding how the C++ language handles time becomes essential.
C++ Standard Library Overview
The C++ Standard Library provides powerful, built-in functions for managing time through the use of specific headers. Two key headers for handling time are:
- `<ctime>`: This header offers traditional C-style time functions.
- `<chrono>`: Introduced in C++11, this provides a richer interface for dealing with time and duration, supporting high-resolution clocks.
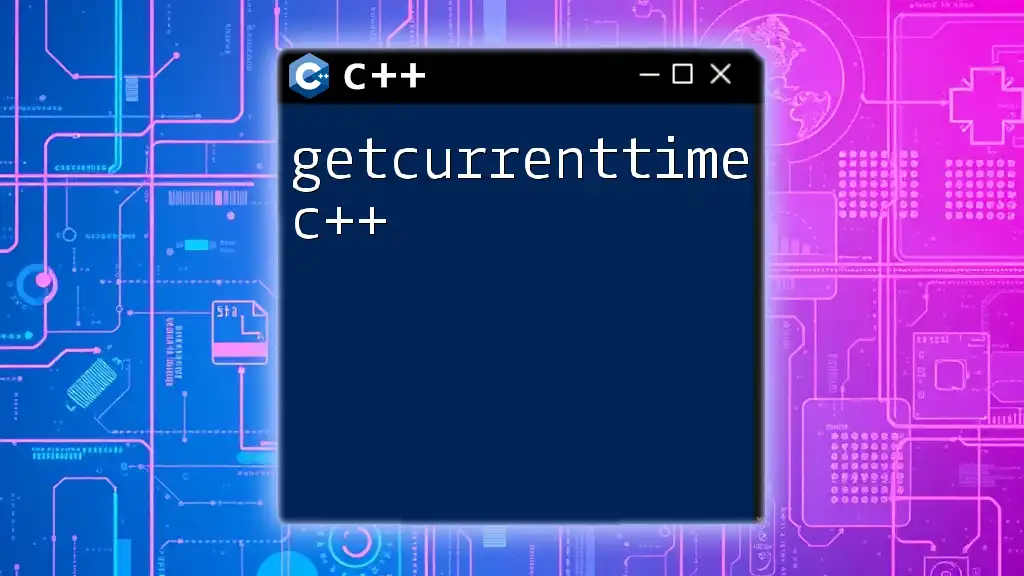
Getting Current Time in C++
Using `<ctime>` Header
To get the current time in C++, you can utilize the `<ctime>` header, which provides the `time` function. This function retrieves the current time as the number of seconds that have elapsed since the "epoch"—January 1, 1970, 00:00:00 UTC.
Here’s how to get the current time:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(0);
std::cout << "Current time in seconds since epoch: " << currentTime << std::endl;
return 0;
}
This code snippet demonstrates getting the current time in seconds since the epoch. Understanding epoch time is fundamental, as it serves as a universal reference point from which other time values can be calculated.
Formatting Current Time with `localtime`
While the raw epoch time is useful, it is often preferred to format it into a human-readable date and time. This is where the `localtime` function comes in handy. It converts the epoch time into a more familiar format.
Here's an example that showcases how to format the current time:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(0);
std::tm *ltm = std::localtime(¤tTime);
std::cout << "Current local time: " << (ltm->tm_year + 1900) << "-"
<< (ltm->tm_mon + 1) << "-"
<< ltm->tm_mday << " "
<< ltm->tm_hour << ":"
<< ltm->tm_min << ":"
<< ltm->tm_sec << std::endl;
return 0;
}
In this snippet, a `std::tm` structure retrieves the components of the current time. This structure contains valuable elements such as the year, month, day, hour, minute, and seconds. Keep in mind that the year needs to be adjusted by adding 1900, and the month is zero-indexed, meaning January is 0.
Using `<chrono>` Library
For more precise and versatile time handling, C++11 introduced the `<chrono>` library. This library supports high-resolution clocks and is generally recommended for modern C++ development.
Here’s how to use `std::chrono` to fetch the current time:
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::system_clock::now();
auto nowTimeT = std::chrono::system_clock::to_time_t(now);
std::cout << "Current time using chrono: " << std::ctime(&nowTimeT);
return 0;
}
Using `std::chrono`, the current time is fetched in a slightly different way but ultimately provides the same output as before. The primary advantage here is the flexibility `chrono` provides, especially for operations involving time calculations or durations.
Comparing `<ctime>` vs `<chrono>`
When discussing time functions in C++, there are key distinctions between using the `<ctime>` library and `<chrono>`.
- <ctime>: Traditional and straightforward, but primarily focused on basic operations.
- <chrono>: Offers more functionality, including high-resolution timers and the ability to work with various units of time (milliseconds, nanoseconds, etc.).
In practice, you might choose `<ctime>` for simplicity when you're only looking to display current time, while `<chrono>` is ideal for applications requiring precision or complex time calculations.
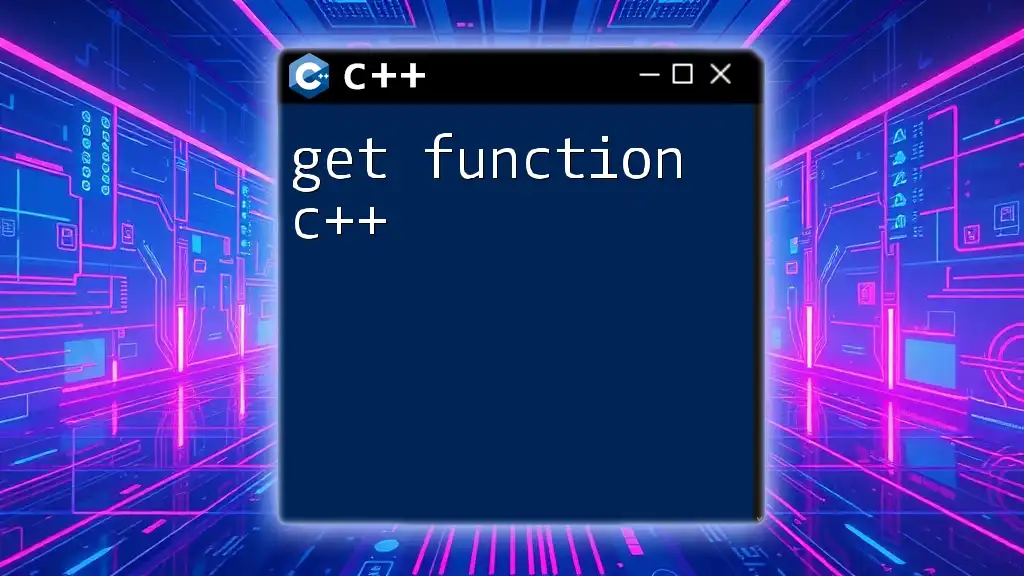
Advanced Techniques for Getting Current Time
Getting Time with Millisecond Precision
In certain applications—like profiling code performance—it can be imperative to obtain the current time with millisecond precision. This can be achieved using `std::chrono::high_resolution_clock`.
Here’s a demonstration:
#include <iostream>
#include <chrono>
int main() {
auto now = std::chrono::high_resolution_clock::now();
auto ms = std::chrono::duration_cast<std::chrono::milliseconds>(now.time_since_epoch()).count();
std::cout << "Current time in milliseconds since epoch: " << ms << std::endl;
return 0;
}
This capability is crucial in scenarios where understanding the elapsed time between events with high accuracy is required. High-resolution timing can make a significant difference in performance-sensitive applications.
Time Zones and Current Time
While we've primarily focused on formatting the local time using the aforementioned methods, working effectively with time zones is equally critical in many applications. Note that neither `<ctime>` nor `<chrono>` directly handle time zones.
For more complex requirements like converting between time zones, developers often turn to third-party libraries like `date.h` or `tz`. These libraries allow more comprehensive manipulation of dates and times along with time zone information, accommodating the global nature of modern applications.
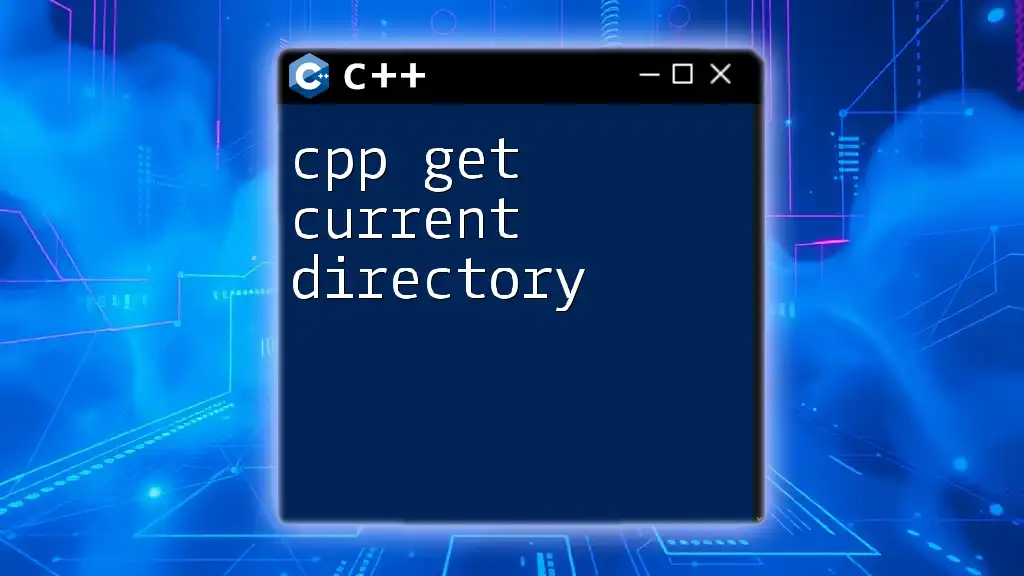
Conclusion
Understanding how to get current time in C++ is foundational for any C++ developer looking to create robust applications. Whether utilizing traditional methods through `<ctime>` or adopting modern practices with `<chrono>`, each approach offers unique advantages and options tailored to specific needs.
Experimenting with these code examples can widen your knowledge and prepare you for time-related programming challenges ahead. Dive in and explore further as you enhance your skills in managing time within your C++ projects!
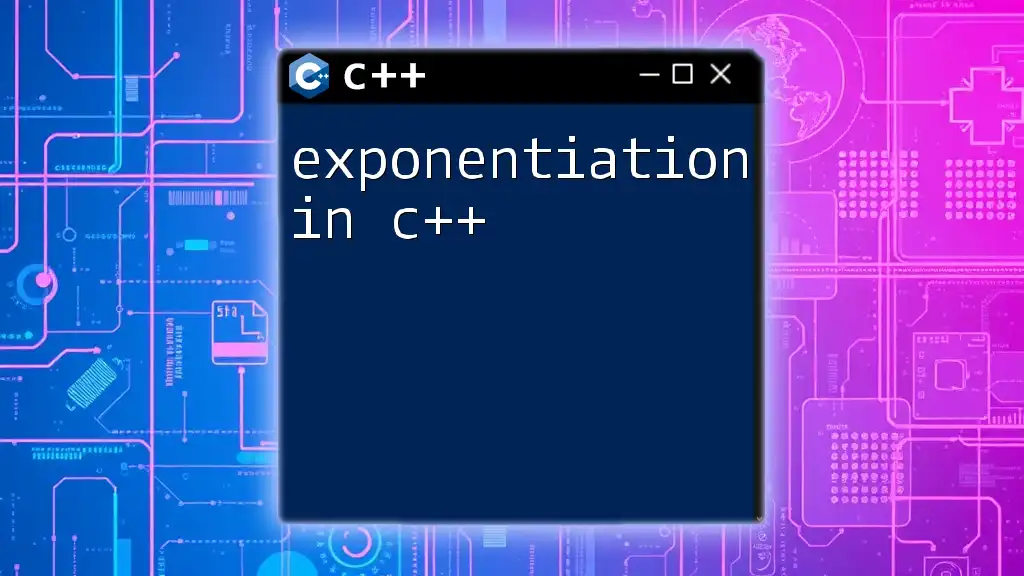
FAQs
Common Questions About Getting Current Time in C++
-
What are the differences between UTC and local time?
UTC (Coordinated Universal Time) is the time standard that the world regulates clocks by, while local time refers to the time in a specific geographic location, which can differ based on time zones. -
How can I measure elapsed time using C++?
You can utilize `std::chrono` to measure the duration between two time points by capturing the start and end times and calculating the difference. -
Can I get current time in a different time format?
Yes, you can format presentation strings in various styles, using functions like `strftime()` from the C library for custom formatted output.