In C++, the return type specifies the data type of the value a function will return, allowing the compiler to check for type correctness when the function is called. Here's a simple example:
int add(int a, int b) {
return a + b;
}
Understanding Return Types
Defining Return Types in C++
A return type in C++ indicates the data type of the value that a function will return to the calling code. It is important as it defines what kind of values can be used after calling the function, thus influencing how the function behaves.
The return type does not merely dictate the value; it also offers features related to the safety and usability of the code. By knowing the return type, programmers can ascertain how to handle the function's result effectively.
Basic Return Types
Primitive Data Types like `int`, `float`, and `char` are the fundamental building blocks of C++. Here’s how they typically function when used as return types:
Code Example: Returning an `int` and a `float`
int add(int a, int b) {
return a + b; // Returns an integer value
}
float divide(float a, float b) {
if(b != 0) {
return a / b; // Returns a float value
}
return 0.0f; // Return 0 if division by zero
}
In this example, the `add` function returns an integer value which is the sum of two integers. The `divide` function operates similarly for floating-point numbers, exhibiting real use cases of basic return types.
Void Return Type
The `void` type is notable in its indication that a function does not return a value. Functions that perform operations, like logging or updating UI elements, often use the `void` return type.
Code Example: `void` Function
void displayMessage() {
std::cout << "Hello, World!" << std::endl; // No return value
}
In the above example, the `displayMessage` function does not return any value but performs a side effect by printing a message to the console. Understanding when to use `void` is key to structuring functions appropriately in C++.
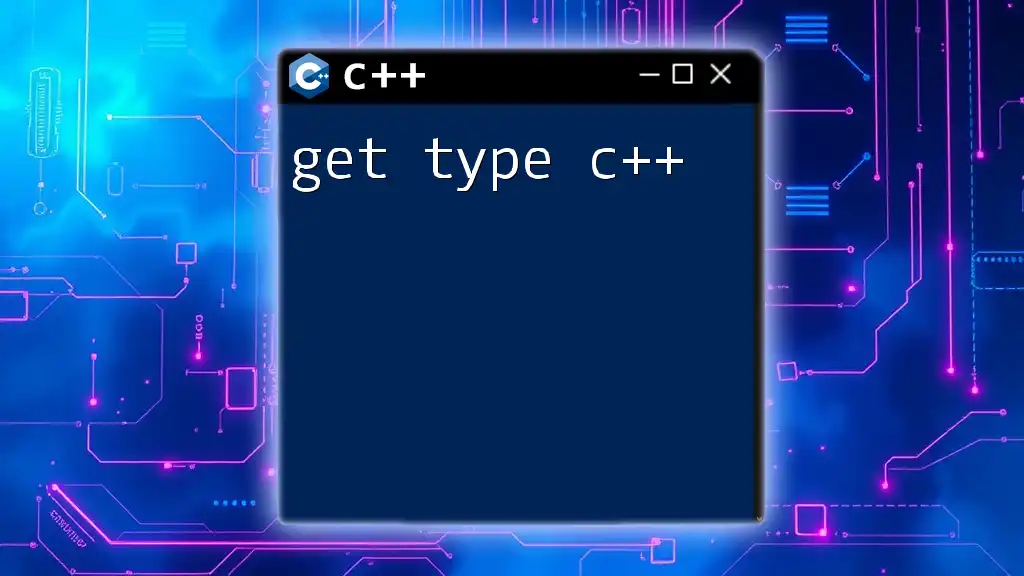
Advanced Return Types
User-Defined Types
C++ allows the use of custom data types such as structs and classes as return types. This adds significant flexibility when dealing with complex data structures.
Code Example: Returning a Struct
struct Person {
std::string name;
int age;
};
Person createPerson(std::string name, int age) {
return Person{name, age}; // Returns a custom struct
}
In this code, the `createPerson` function constructs and returns an instance of the `Person` struct. This flexibility makes C++ a powerful tool for modeling real-world entities.
Pointers and References
Returning Pointers can be useful when dealing with dynamically allocated memory. However, this approach requires diligent memory management to avoid memory leaks.
Code Example: Returning a Pointer
int* allocateMemory() {
int* ptr = new int(5); // Dynamically allocated integer
return ptr; // Important to free this memory later
}
In this example, the `allocateMemory` function returns a pointer to an integer. Upon using this function, it is critical to free the allocated memory using `delete` to prevent memory leaks.
Returning References can also enhance performance by allowing modifications to the original variable without creating a copy.
Code Example: Returning a Reference
int& getElement(std::vector<int>& vec, size_t index) {
return vec[index]; // Returns a reference to a vector element
}
Here, `getElement` returns a reference to an element in a vector, enabling efficient access and modification without the overhead of copying the value.
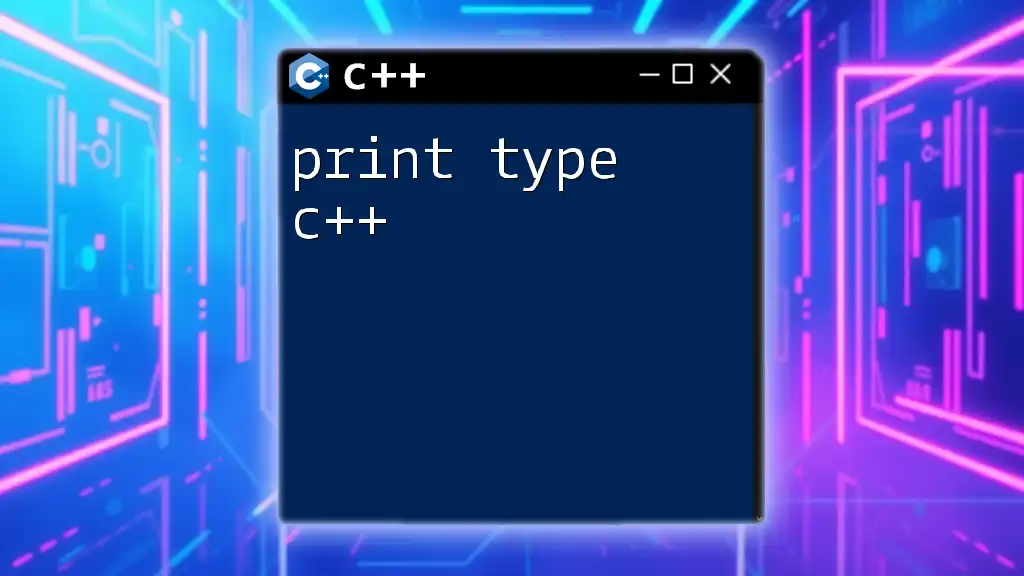
Special Return Types
Using `auto` for Return Type
Using the `auto` keyword can simplify function definitions by allowing the compiler to deduce the return type automatically based on the return statement. This allows for cleaner code and easier maintenance.
Code Example: Using `auto`
auto multiply(float a, float b) {
return a * b; // Compiler deduces return type as float
}
In this function, the return type is automatically determined as `float` since both parameters are floats. Using `auto` may make the code more concise without sacrificing type safety.
Function Overloading and Return Types
Function overloading allows multiple functions to have the same name with different parameter types. However, the return type alone cannot distinguish overloaded functions; the parameters must differ.
Code Example: Overloaded Functions with Different Return Types
float calcArea(float radius) {
return 3.14f * radius * radius; // Calculates area for circle
}
int calcArea(int length, int width) {
return length * width; // Calculates area for rectangle
}
In this example, we have two versions of `calcArea`. Although they have different return types, they also differ in parameter types, allowing both functions to coexist.
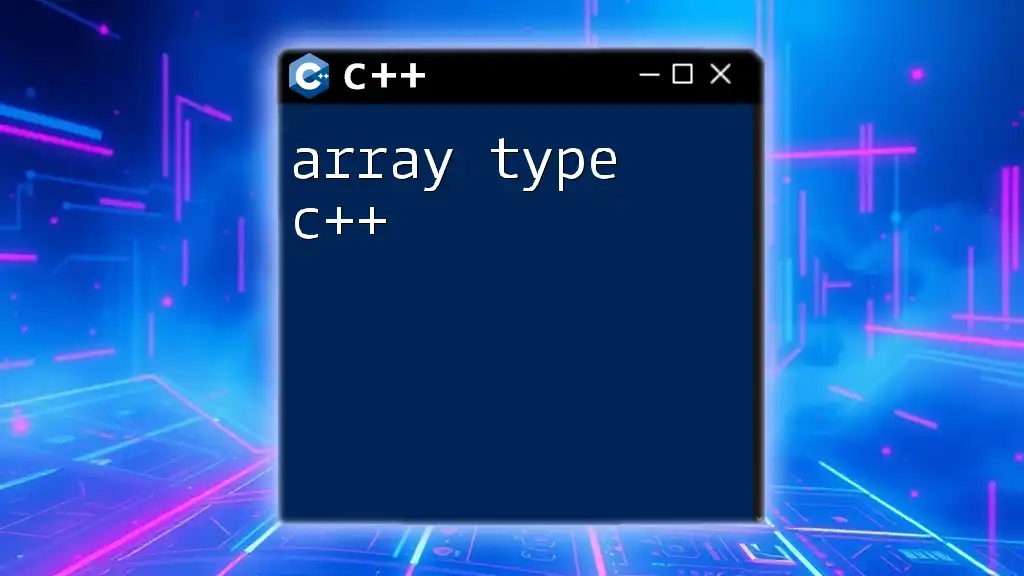
Best Practices for Return Types
Choosing the Right Return Type
When deciding on a return type, consider the expected outputs and how they will be utilized in C++. Choosing the appropriate type enhances code clarity and ensures more predictable behavior in calling functions.
Performance Considerations
The return type affects performance, particularly when returning large objects. By returning objects by value, you may invoke unnecessary copying, which can hinder performance. Move semantics can be a better option in such cases.
Additionally, using smart pointers like `std::unique_ptr` and `std::shared_ptr` can provide automatic memory management and decrease the likelihood of memory leaks when returning pointers.
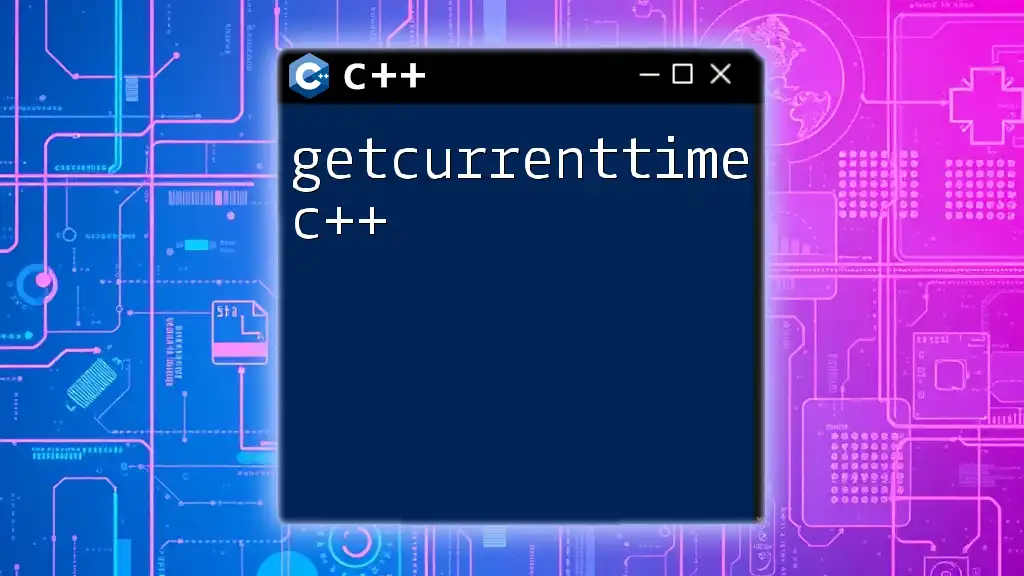
Conclusion
Understanding the concept of return type in C++ is fundamental to effective programming within the language. By mastering various return types, their usage, and their implications on performance and code safety, you enhance your ability to write robust, efficient C++ code.
Join Us for More Learning!
Stay engaged with our community for more tips and tutorials. Continued practice and exploration of return types and their functionalities will enrich your capacity as a C++ programmer.