In C++, the `decltype` keyword can be used to determine the type of an expression, enabling you to retrieve the type of a variable or return type of a function concisely.
Here’s an example:
#include <iostream>
int main() {
int a = 5;
decltype(a) b = 10; // b is of the same type as a (int)
std::cout << "Type of b: " << typeid(b).name() << std::endl; // Output: Type of b: int
return 0;
}
Understanding Variable Types in C++
What are Variable Types?
In programming, variable types (also known as data types) define the kind of value a variable can hold and the operations that can be performed on it. Understanding variable types is crucial in C++, as it directly impacts memory allocation, performance, and the behavior of your code.
Common Variable Types in C++
C++ provides several variable types, which can be broadly categorized into:
-
Primitive Types: These include fundamental data types such as:
- `int` (for integer values)
- `char` (for characters)
- `bool` (for boolean values)
- `float` (for single-precision floating-point values)
- `double` (for double-precision floating-point values)
-
User-Defined Types: These allow programmers to create their own data types. Common examples include:
- `struct` (to create composite data types)
- `class` (for object-oriented programming)
- `enum` (for defining enumeration types)
Understanding the differences between these types can lead to more efficient and readable code.
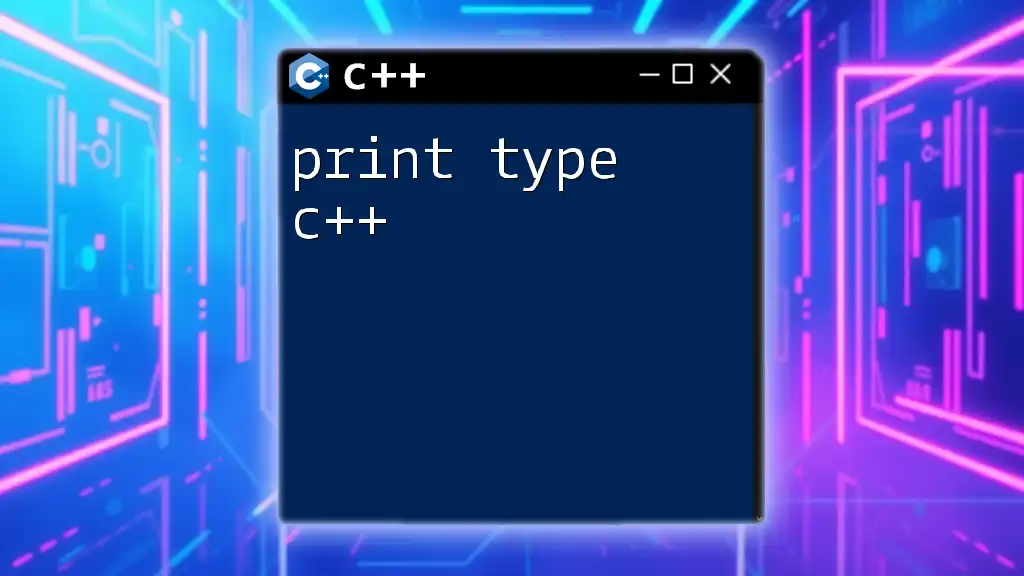
Getting the Type of a Variable in C++
Using `typeid`
The `typeid` operator is a powerful way to get the type of a variable in C++. It returns a `type_info` object that contains information about the type of the variable at runtime.
Here's a simple example:
#include <iostream>
#include <typeinfo>
int main() {
int a = 5;
std::cout << "Type of a: " << typeid(a).name() << std::endl;
return 0;
}
In this code, `typeid(a).name()` will typically return a string representing the type of variable `a`, which is `int`. However, the actual output may vary based on the compiler implementation. One limitation of `typeid` is that it may not work well with polymorphic types, specifically when dealing with base class pointers.
Using the `decltype` Keyword
The `decltype` keyword allows you to retrieve the variable's type without evaluating it. This is particularly useful in template programming or when declaring new variables based on existing ones.
Consider the following example:
#include <iostream>
int main() {
double b = 3.14;
decltype(b) c = 2.71; // c will be of type double
std::cout << "Type of c: " << typeid(c).name() << std::endl;
return 0;
}
In this example, `decltype(b)` determines the type of `b` and assigns it to `c`, ensuring that `c` is also a `double`.
Using `std::remove_reference` and `std::remove_cv`
C++ provides utilities in the `<type_traits>` header that help in determining the type of a variable, especially when dealing with references and const-volatile qualifiers.
Here’s how you can use `std::remove_reference` in combination with `std::remove_cv`:
#include <iostream>
#include <type_traits>
int main() {
const int& ref = 10;
using T = std::remove_reference<decltype(ref)>::type;
std::cout << "Type of ref: " << typeid(T).name() << std::endl; // Should output "int"
return 0;
}
In this code, `std::remove_reference` removes any reference from the type of `ref`, ensuring that we retrieve just the base type, which in this case is `int`.
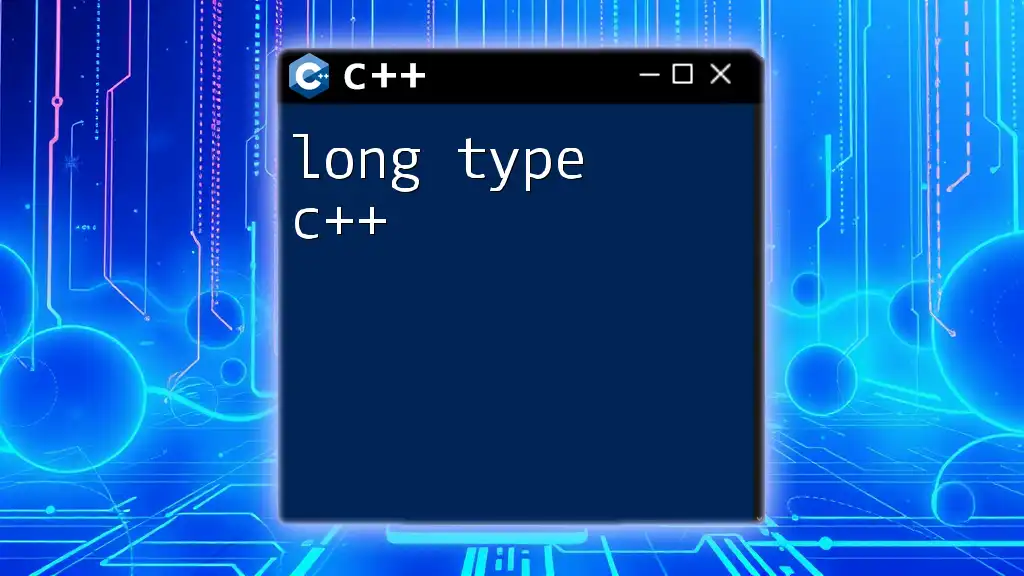
Practical Examples
Example 1: Finding Variable Type Dynamically
You can also define a function that takes variables of different types and prints their types dynamically:
#include <iostream>
#include <typeinfo>
template <typename T>
void getType(T var) {
std::cout << "Type: " << typeid(var).name() << std::endl;
}
int main() {
getType(5); // int
getType(5.5); // double
getType("Hello"); // const char*
return 0;
}
The `getType` function demonstrates how templates can help ascertain the variable type at compile time, allowing for versatility and type safety.
Example 2: Type Inference with Templates
Type inference allows C++ to determine the type of a variable automatically. This is particularly useful when implementing functions that need to introduce flexibility in type declarations:
#include <iostream>
template <typename T>
void showType(T variable) {
std::cout << "The type of the variable is: " << typeid(variable).name() << std::endl;
}
int main() {
showType(100);
showType(3.14f);
showType("Sample String");
return 0;
}
In this example, the `showType` function prints the type of the variable passed to it, showcasing the power of templates in providing type information at runtime.
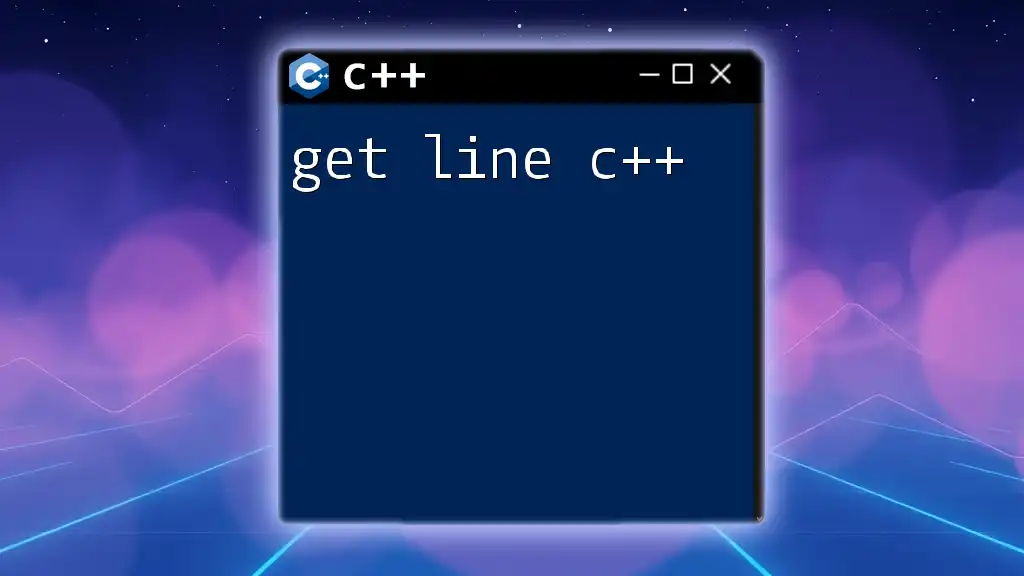
Advanced Techniques
Type Traits Overview
Type traits are part of C++'s `<type_traits>` library, enabling programmers to inspect and manipulate types at compile time. They are helpful for conditional type definitions and template programming.
Using `std::is_same`
One of the most popular type traits is `std::is_same`, which can check if two types are the same (including user-defined types). Here’s how you can use it:
#include <iostream>
#include <type_traits>
int main() {
int a = 0;
if (std::is_same<decltype(a), int>::value) {
std::cout << "a is of type int." << std::endl;
}
return 0;
}
This snippet will confirm that the variable `a` is indeed of type `int`, offering a compile-time check that can enhance type safety in templates.
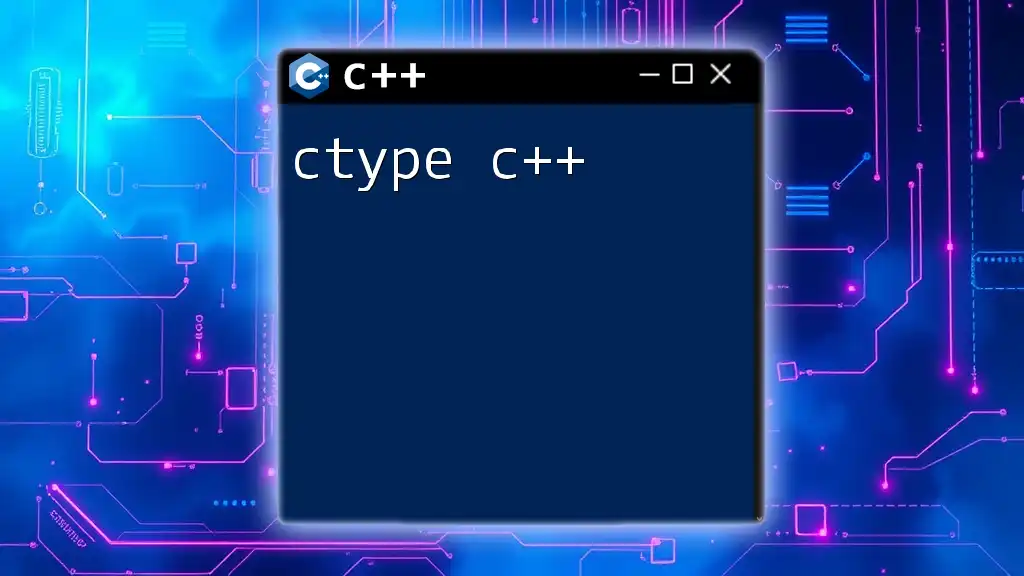
Conclusion
In this guide, we have explored various methods to get type in C++. From using the `typeid` operator to employing `decltype`, `std::remove_reference`, and advanced type traits, we've delved into effective ways to retrieve and work with variable types in C++. Understanding variable types not only helps in debugging but also leads to better-optimized and clearer code.
I encourage you to experiment with the examples provided and integrate the concepts into your coding practices. Through these techniques, you'll gain more flexibility and power in manipulating types in your C++ projects.
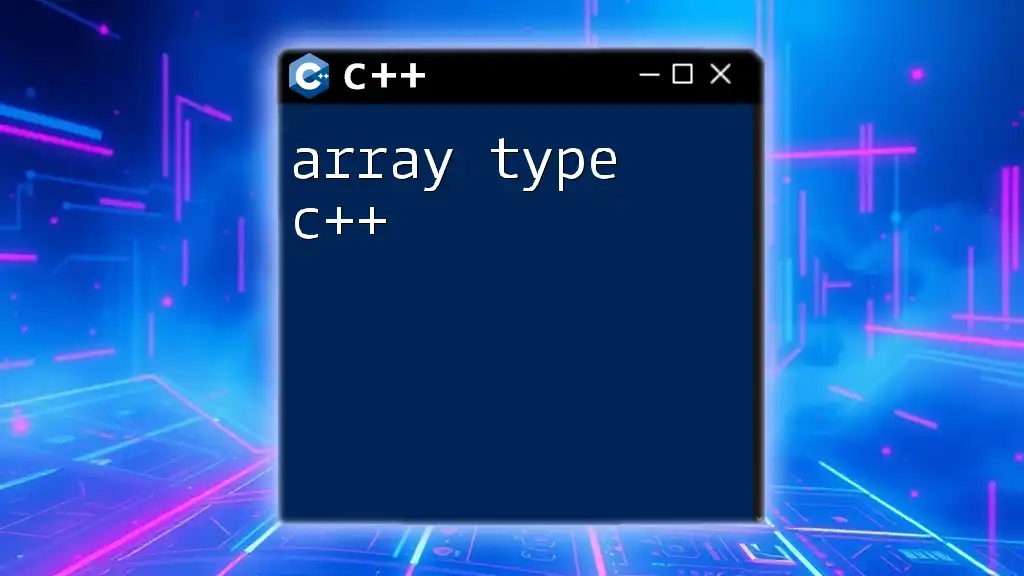
Additional Resources
For further reading on C++ data types, type traits, and advanced programming techniques, consider exploring reputable C++ tutorials and reference sites that can deepen your understanding and enhance your skills in this versatile programming language.