"Feature C++ refers to the modern use of C++ programming language features such as auto, smart pointers, and lambda expressions that enhance code efficiency and readability."
Here’s an example using a lambda function with `std::for_each`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n * n << ' ';
});
return 0;
}
Key Features of C++
Object-Oriented Programming
Encapsulation is one of the cornerstone principles of object-oriented programming (OOP). It allows the bundling of data with the methods that operate on that data, restricting direct access to some of the object's components. This provides an important layer of security and logical separation in a program.
class BankAccount {
private:
double balance; // Encapsulated attribute
public:
BankAccount() : balance(0.0) {} // Constructor
void deposit(double amount) {
if (amount > 0) {
balance += amount;
}
}
double getBalance() const {
return balance; // Accessor method
}
};
In this example, `balance` is a private variable, and access to it is controlled via public methods.
Inheritance promotes code reusability by allowing a new class to inherit properties and behaviors from an existing class. It can be categorized into several types, including single, multiple, and hierarchical inheritance.
class Account {
protected:
double balance;
public:
Account(double initialBalance) : balance(initialBalance) {}
void deposit(double amount) {
balance += amount;
}
};
class SavingsAccount : public Account {
public:
SavingsAccount(double initialBalance) : Account(initialBalance) {}
double getBalance() const {
return balance;
}
};
In this code, `SavingsAccount` inherits the properties of `Account`, allowing it to use its methods and data.
Polymorphism allows methods to do different things based on the object it is acting upon, hence enhancing flexibility. It exists in two forms: compile-time (method overloading) and runtime (method overriding).
class Shape {
public:
virtual void draw() const {
// Default implementation (if any)
}
};
class Circle : public Shape {
public:
void draw() const override {
// Drawing a circle
}
};
class Rectangle : public Shape {
public:
void draw() const override {
// Drawing a rectangle
}
};
In this example, the `draw` method can behave differently depending on whether a `Circle` or `Rectangle` object calls it.
Templates
Templates enable developers to write generic and reusable code. They act as blueprints for creating functions and classes with a placeholder for types.
Function Templates make it possible to create a single function definition that works with different data types without rewriting code for each type.
template <typename T>
T maximum(T a, T b) {
return (a > b) ? a : b;
}
This function template can be used to determine the maximum value of various data types like `int`, `float`, or custom objects.
Class Templates allow for creating class definitions that can operate with any data type.
template <typename T>
class Stack {
private:
std::vector<T> elements;
public:
void push(const T& element) {
elements.push_back(element);
}
T pop() {
T elem = elements.back();
elements.pop_back();
return elem;
}
};
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides a rich set of algorithms and data structures, significantly speeding up the development process.
Containers are collections that store objects. The STL includes several types:
- Vector: A dynamic array that grows in size.
- List: A doubly linked list.
- Set: A collection of unique elements.
- Map: A collection of key-value pairs.
For example, a `vector` can be used to store and iterate over elements efficiently.
#include <vector>
#include <iostream>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
for (int value : myVector) {
std::cout << value << " ";
}
// Output: 1 2 3 4 5
}
Algorithms in STL facilitate common operations such as sorting and searching without the need for detailed implementations. For instance:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {5, 3, 2, 4, 1};
std::sort(numbers.begin(), numbers.end()); // Sort the vector
}
Iterators act as pointers for accessing elements within containers, allowing for easy navigation through data structures.
#include <vector>
#include <iostream>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
std::cout << *it << " ";
}
// Output: 1 2 3 4 5
}
Memory Management
Effective memory management is crucial in C++ programming.
Dynamic vs Static Memory Allocation: Static memory allocation occurs at compile time, while dynamic memory allocation allows a programmer to request memory at runtime. Understanding when to use each is critical.
Smart Pointers have been a significant advancement in C++ memory management, as they help to automatically manage memory resource, reducing memory leak risks.
- std::unique_ptr: Represents exclusive ownership of a resource.
- std::shared_ptr: Allows multiple pointers to own the same resource.
- std::weak_ptr: Provides a way to reference resources without affecting their lifetime.
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> ptr1(new int(42)); // Unique ownership
std::shared_ptr<int> ptr2 = std::make_shared<int>(10);
}
Manual Memory Management is done using the `new` and `delete` operators. Proper care should be taken here to avoid memory leaks.
int* myArray = new int[10]; // Dynamic allocation
delete[] myArray; // Deallocating memory
Exception Handling
Exception handling allows C++ programs to deal with errors and unexpected behavior gracefully. It ensures that error conditions can be handled without crashing the program.
The try-catch-throw mechanism is central to C++ exception handling.
#include <iostream>
#include <stdexcept>
void divide(int a, int b) {
if (b == 0) {
throw std::invalid_argument("Division by zero");
}
std::cout << "Result: " << a / b << std::endl;
}
int main() {
try {
divide(10, 0);
} catch (const std::invalid_argument& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
}
In this example, an exception is thrown when division by zero is attempted, allowing graceful handling in the `catch` block.
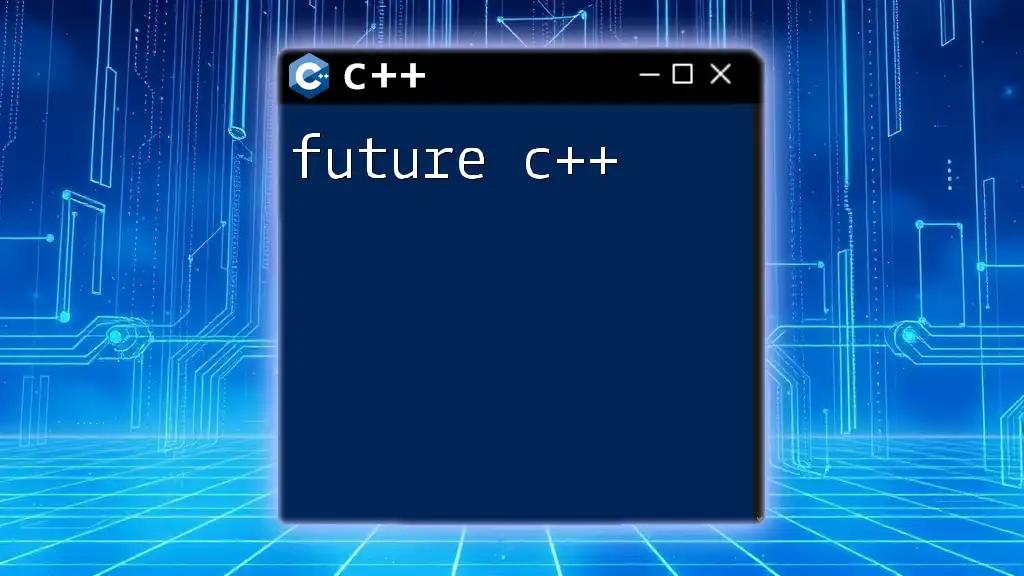
C++11 and Beyond: New Features
C++11 introduced several new features that enhance the expressiveness and usability of the language.
Lambda Expressions make it possible to write inline anonymous function objects, which are especially useful in algorithms.
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> values = {1, 2, 3, 4, 5};
std::for_each(values.begin(), values.end(), [](int n) { std::cout << n << " "; });
}
Range-based For Loops simplify iteration over containers.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& number : numbers) {
std::cout << number << " ";
}
}
Auto Keyword allows the compiler to automatically deduce the type of a variable, simplifying code and improving maintainability.
auto myVariable = 42; // Compiler infers myVariable as int
Best Practices in C++
Maintaining code readability and maintainability is essential for long-term project success. Adopting consistent naming conventions, organizing code into coherent units, and writing clear comments can significantly enhance the quality of your C++ code.
Performance considerations should also be a priority. Optimizing algorithms, avoiding unnecessary copies, and utilizing efficient data structures can result in significant performance gains.
Continuous learning and resources are critical in mastering C++. Books like C++ Primer or Effective C++ are excellent resources, alongside numerous online courses and vibrant communities that facilitate learning and sharing experiences.
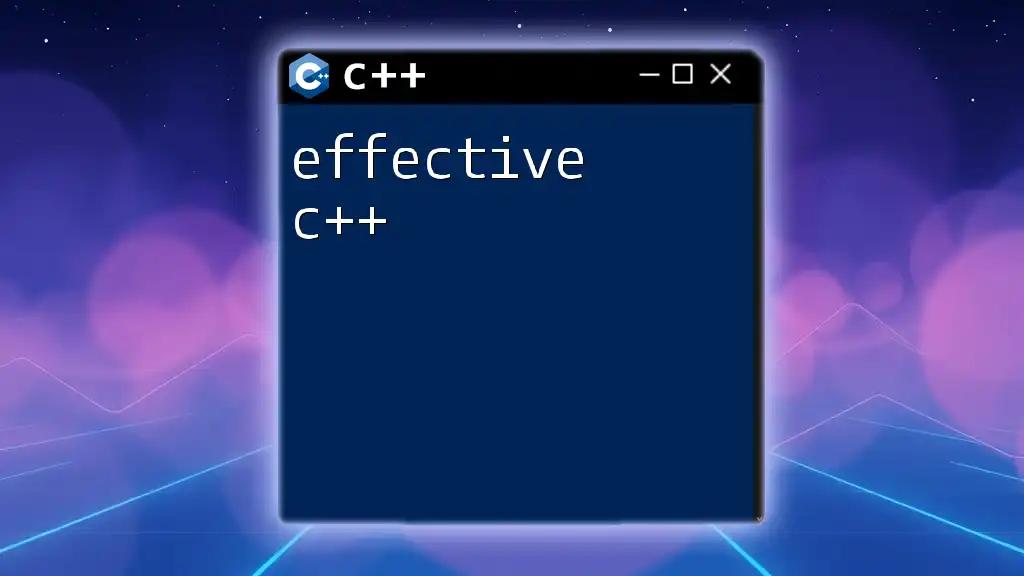
Conclusion
Understanding and leveraging key feature C++ provisions is imperative for effective programming. From object-oriented concepts to the modern capabilities introduced in C++11 and beyond, these features empower developers to create robust, efficient applications. The future of C++ looks bright, with ongoing updates ensuring that it remains relevant in an ever-evolving technological landscape.
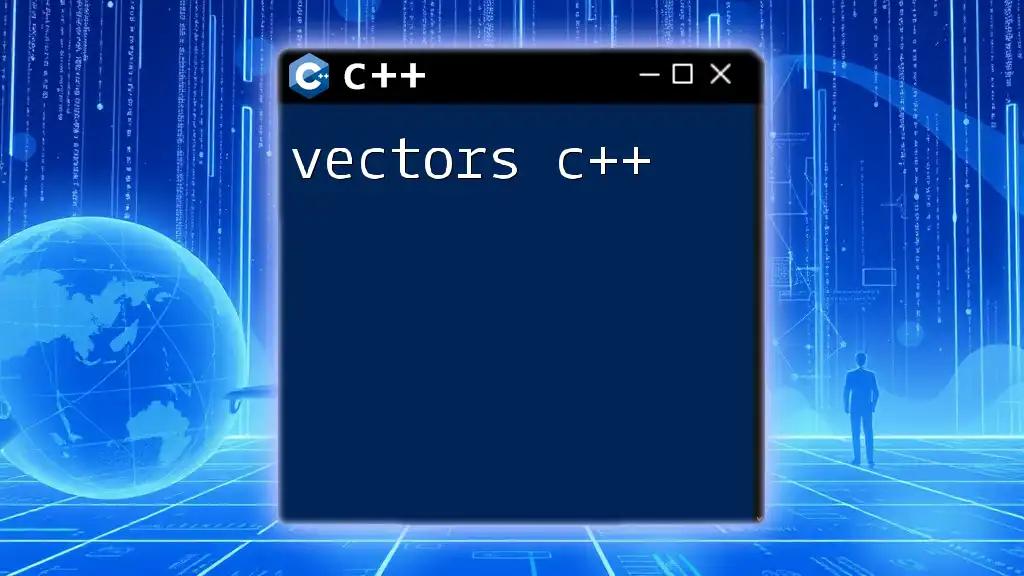
Call to Action
Join us in mastering C++ features and enhancing your programming skills. Engage with our courses and resources, and don’t hesitate to share your thoughts and experiences in the C++ community!