"Effective C++ by Scott Meyers provides essential guidelines and best practices for writing clear, efficient, and maintainable code in C++."
Here's a code snippet demonstrating the Rule of Zero, which encourages resource management through automatic storage duration:
#include <iostream>
#include <vector>
class Resource {
public:
Resource() {
std::cout << "Resource acquired." << std::endl;
}
~Resource() {
std::cout << "Resource released." << std::endl;
}
};
class Container {
public:
void addResource() {
resources.emplace_back();
}
private:
std::vector<Resource> resources;
};
int main() {
Container c;
c.addResource();
return 0; // Resources are automatically managed
}
Understanding the Importance of C++
C++ stands as a key pillar in modern programming, prominently used in system/software development, game development, and performance-critical applications. Writing high-quality, efficient, and maintainable code is paramount, especially in C++, where complexity can quickly escalate if not managed properly.
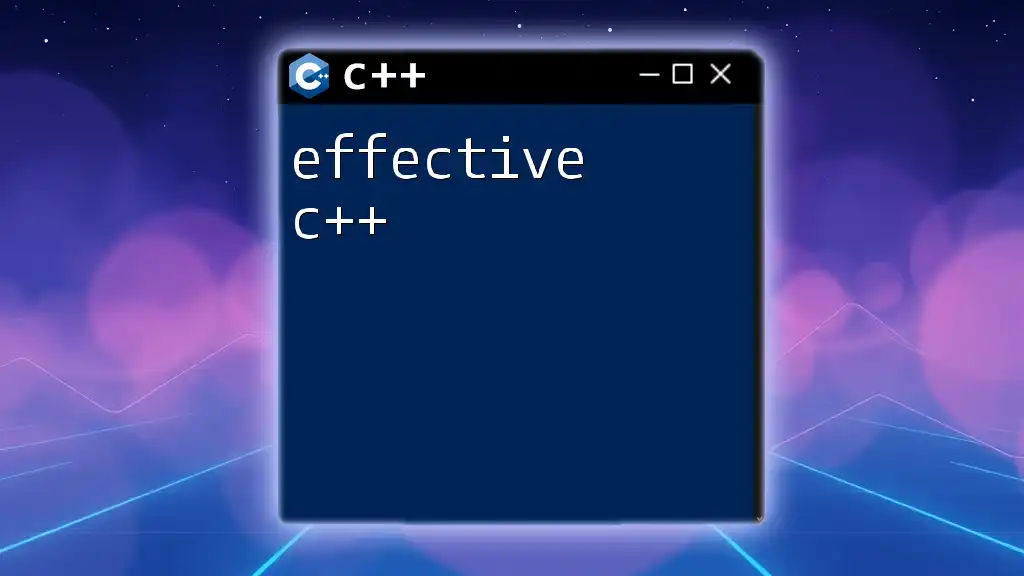
Who is Scott Meyers?
Scott Meyers is a notable figure in the C++ community, renowned for his insightful contributions and teachings related to C++. His book, "Effective C++," has become a seminal text, guiding programmers toward better practices when using the C++ language. By focusing on practical advice and real-world application, Meyers' work has shaped countless C++ developers' approaches, enhancing their productivity and code quality.
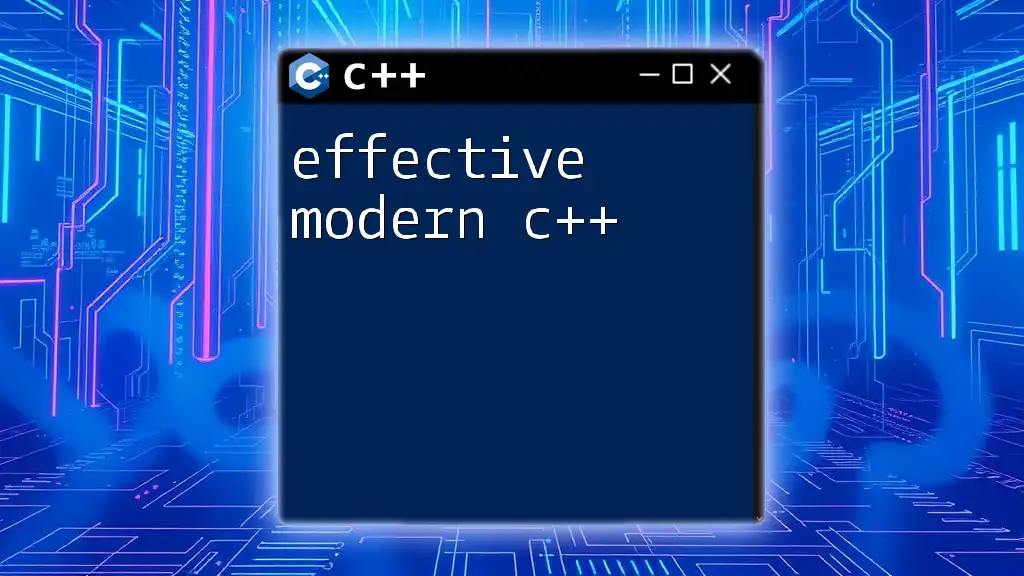
Core Principles of Effective C++
Resource Management
Effective C++ programming begins with understanding and managing resources efficiently. A fundamental principle here is RAII (Resource Acquisition Is Initialization). This technique emphasizes that resource allocation and deallocation should be tied to object lifespan, preventing resource leaks and dangling pointers.
Consider the following example that illustrates RAII by managing dynamic memory:
class Resource {
int* ptr;
public:
Resource(int size) : ptr(new int[size]) {}
~Resource() { delete[] ptr; }
};
In this code, the `Resource` class manages an integer array. When an object of this class goes out of scope, its destructor automatically frees the allocated memory, ensuring proper resource management.
Use of the Rule of Three
The Rule of Three is a C++ principle underscoring the significance of defining three special member functions: the copy constructor, the copy assignment operator, and the destructor. When a class manages a dynamically allocated resource, failing to implement any of these functions may lead to memory leaks or improper resource handling.
Here is an implementation of the Rule of Three:
class MyClass {
int* data;
public:
MyClass(int value) : data(new int(value)) {}
// Copy constructor
MyClass(const MyClass& other) : data(new int(*other.data)) {}
// Copy assignment operator
MyClass& operator=(const MyClass& other) {
if (this != &other) {
delete data; // Free existing resource
data = new int(*other.data); // Allocate new resource
}
return *this;
}
// Destructor
~MyClass() { delete data; }
};
This class correctly manages memory, ensuring that resources are allocated and freed appropriately across copy operations.
Const Correctness
Using `const` judiciously is a vital habit for effective C++ coding. It not only documents the intent but also provides compiler guarantees that certain data won't be modified. This leads to more robust, maintainable, and understandable code.
Consider a function taking a `const` reference as a parameter:
void process(const std::string& str) {
// str cannot be modified
}
In this example, `str` is guaranteed not to change within the `process` function, promoting const correctness and enhancing code reliability.
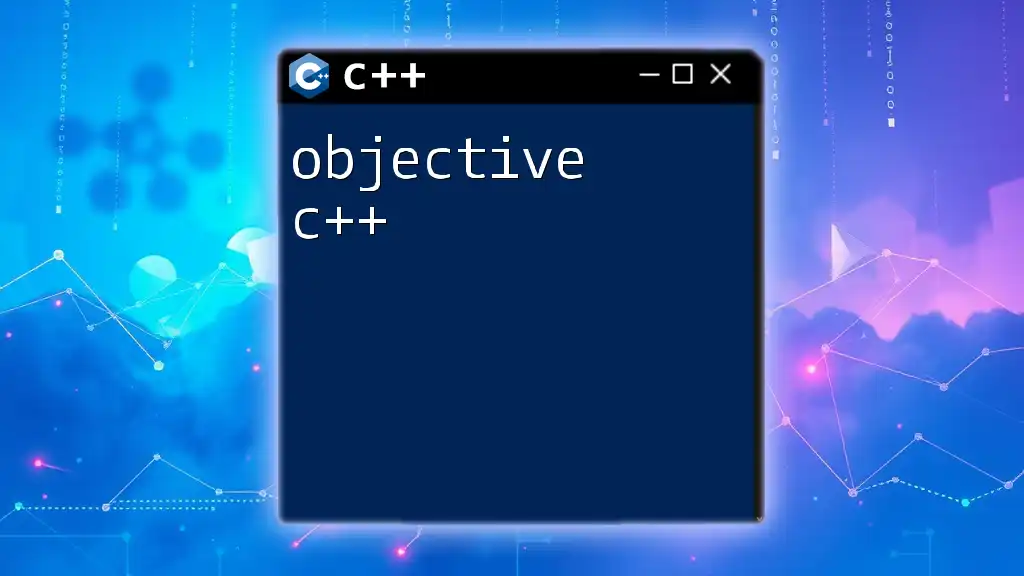
Smart Pointers and Memory Management
Overview of Smart Pointers
Smart pointers are essential for modern C++ programming, providing a safe and efficient means to manage dynamic memory. Unlike raw pointers, smart pointers automatically manage the memory they point to, helping to prevent memory leaks and dangling pointers.
Types of Smart Pointers
Unique Pointers (`std::unique_ptr`)
A `std::unique_ptr` provides exclusive ownership over a dynamically allocated object. Once a `unique_ptr` goes out of scope or is reset, the memory it manages is automatically deallocated.
Here’s an example:
std::unique_ptr<MyClass> myObj = std::make_unique<MyClass>(42);
Shared Pointers (`std::shared_ptr`)
`std::shared_ptr` allows multiple pointers to share ownership of a single object, using reference counting to manage memory. When the last `shared_ptr` referring to an object is destroyed, the memory is released.
Example:
std::shared_ptr<MyClass> sharedObj = std::make_shared<MyClass>(42);
Weak Pointers (`std::weak_ptr`)
`std::weak_ptr` complements `shared_ptr` by providing a non-owning reference to an object, preventing circular references that can cause memory leaks:
std::weak_ptr<MyClass> weakPtr = sharedObj; // weak reference
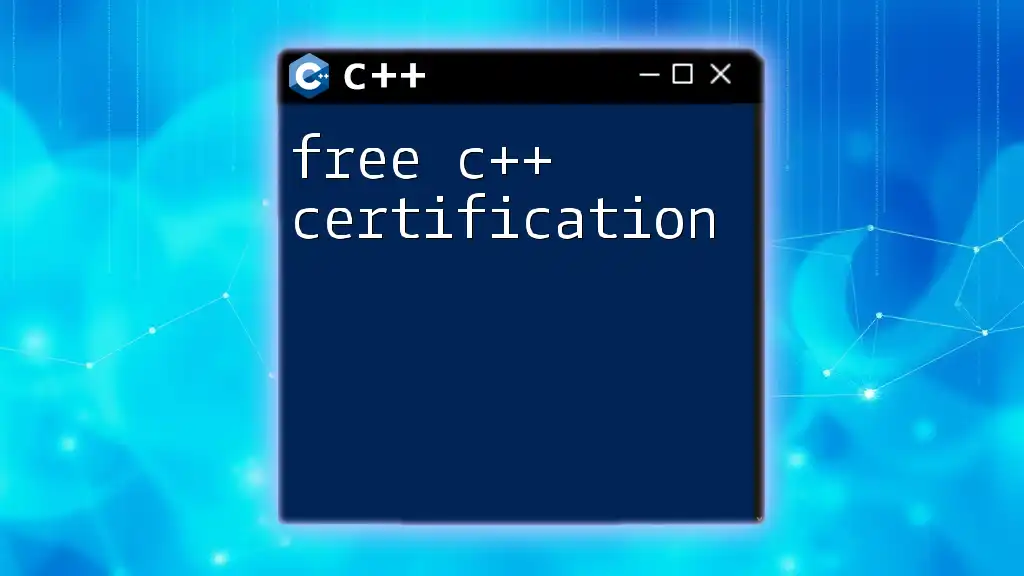
Template Programming with C++
Introduction to Templates
Templates are a powerful feature in C++ that allows programmers to write generic and reusable code. They enable the creation of functions and classes that work with any data type, thus enhancing code reusability.
Function Templates
Function templates allow you to define a function without specifying the exact type. Here's a simple example of a function template that finds the maximum of two values:
template<typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
Class Templates
You can also create class templates to define generic classes. Here’s how a simple stack class template can be structured:
template<typename T>
class Stack {
std::vector<T> elements; // Stack elements
public:
void push(const T& elem) { elements.push_back(elem); }
void pop() { elements.pop_back(); }
T top() const { return elements.back(); }
};
This `Stack` class can store elements of any type, thanks to the use of templates.
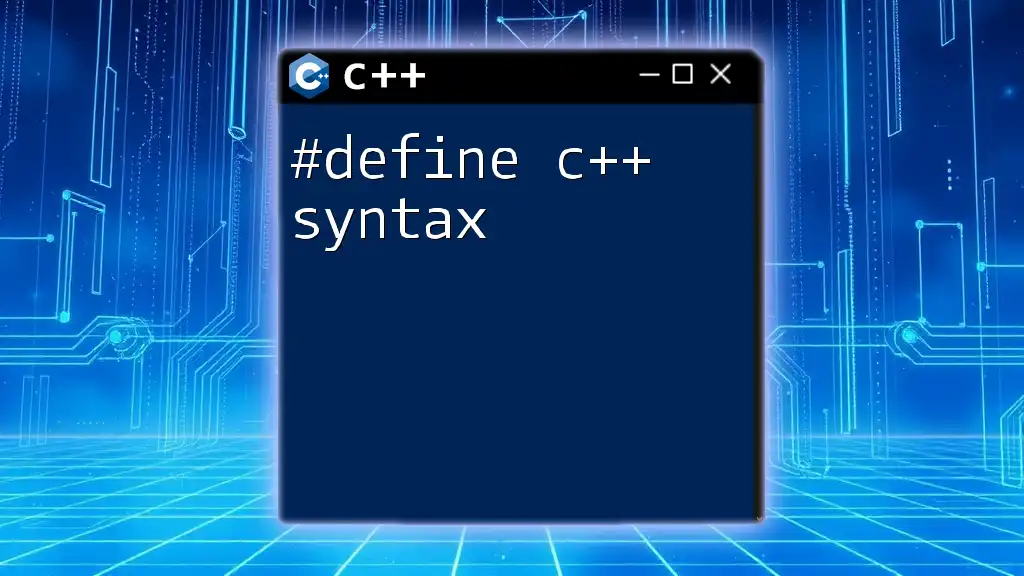
Advanced C++ Concepts
Move Semantics
Move semantics, introduced in C++11, enhance performance by allowing resources to be moved rather than copied. This is particularly advantageous for managing temporary objects and eliminating unnecessary copies.
Here’s an example illustrating move semantics:
class Movable {
int* data;
public:
Movable(int size) : data(new int[size]) {}
// Move constructor
Movable(Movable&& other) noexcept : data(other.data) {
other.data = nullptr; // Leave the source object empty
}
~Movable() { delete[] data; }
};
Lambda Expressions
Lambda expressions offer a concise way to create function objects. They facilitate writing inline functions, significantly simplifying syntax when handling callbacks or defining custom behaviors in algorithms.
Here’s an example of a lambda expression:
auto square = [](int x) { return x * x; };
This lambda allows easy definition of small, unnamed functions that can be passed around as needed.
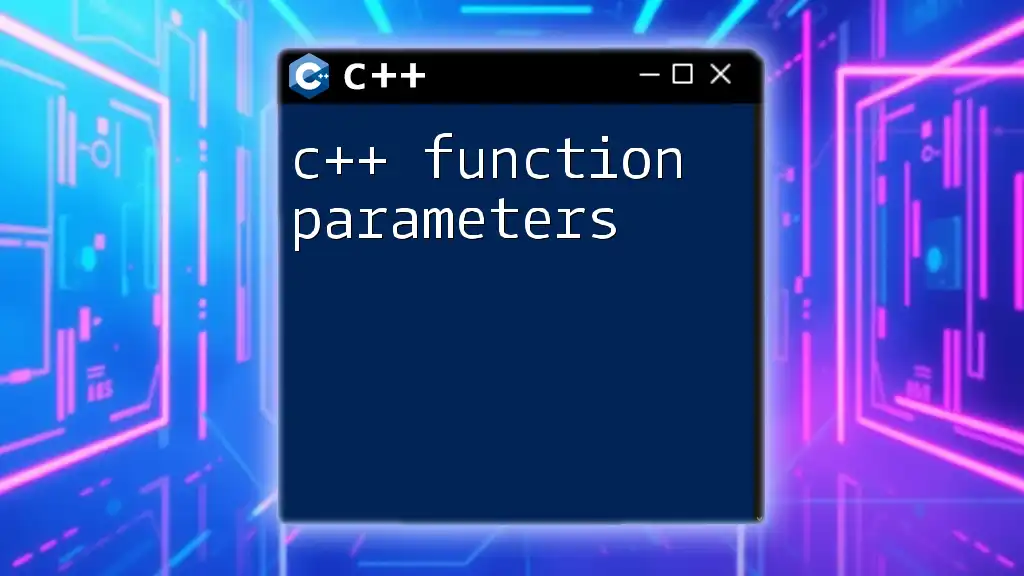
Best Practices and Common Pitfalls
Writing Clean and Maintainable Code
Developing effective C++ code relies heavily on clean and maintainable practices. Here are some guidelines:
- Use meaningful names: Class and function names should convey their intent.
- Write comments: Clear comments help others (and your future self) understand your code's purpose and functionality.
- Break down complex functions: Smaller functions are easier to test and understand.
Avoiding Undefined Behavior
Undefined behavior can lead to unpredictable results and security vulnerabilities. Common situations to watch for include:
- Dereferencing null or dangling pointers.
- Accessing memory out of bounds.
- Using uninitialized variables.
Being vigilant in these areas is essential to maintain robustness and reliability in your applications.
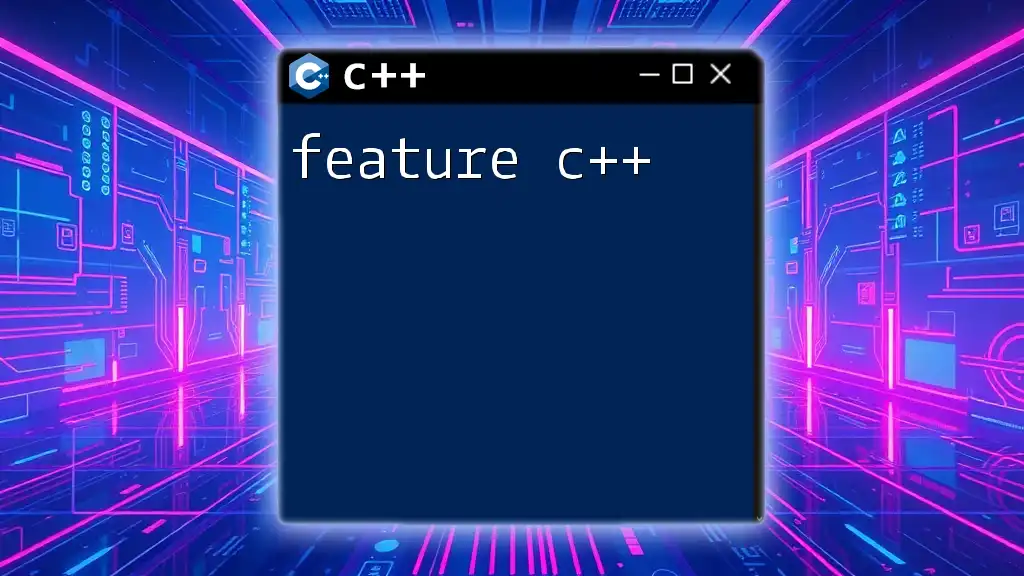
Conclusion
In this article, we've explored the principles and practices outlined in Effective C++ by Scott Meyers. These principles not only improve your C++ coding but also emphasize the importance of resource management, const correctness, and modern features like smart pointers and templates.
By adopting these practices, you'll enhance your ability to write clean, efficient, and maintainable code. For deeper insights, consider diving into Effective C++ and exploring the wealth of knowledge it contains for both novice and experienced developers alike.