C is generally faster than C++ for simple tasks due to its lower-level nature and lack of abstraction overhead, but C++ often outperforms C in complex applications because of advanced features like object-oriented programming and better optimization opportunities.
#include <iostream>
int main() {
// C example: simple factorial function
int factorial(int n) {
return (n == 0) ? 1 : n * factorial(n - 1);
}
std::cout << factorial(5) << std::endl; // Output: 120
return 0;
}
class Factorial {
public:
static int calculate(int n) {
return (n == 0) ? 1 : n * calculate(n - 1);
}
};
int main() {
std::cout << Factorial::calculate(5) << std::endl; // Output: 120
return 0;
}
Understanding Performance Metrics
What is Performance?
Performance in programming is a crucial metric that determines how efficiently a language executes tasks. This encompasses several key performance indicators, including:
- Speed: The time taken to execute a program or specific functions within it.
- Memory Usage: The amount of memory consumed during execution.
- Efficiency: A broader concept that includes the interplay between speed and resource utilization.
Understanding these metrics is essential for choosing the right tool for the job, especially when comparing C vs C++ performance.
Factors Influencing Performance
Several factors influence the performance of programs written in C and C++:
-
Algorithm Complexity: An algorithm's complexity (often expressed using Big O notation) plays a significant role in performance. A poorly chosen algorithm may offset any advantages offered by the programming language.
-
Compiler Optimizations: Modern compilers perform numerous optimizations that can dramatically improve performance. For example, C++ compilers may implement techniques like inlining functions to reduce overhead.
-
Hardware Interactions: The efficiency of how a programming language interacts with the hardware (CPU, memory, etc.) can heavily influence performance. Understanding this helps in making optimal choices.
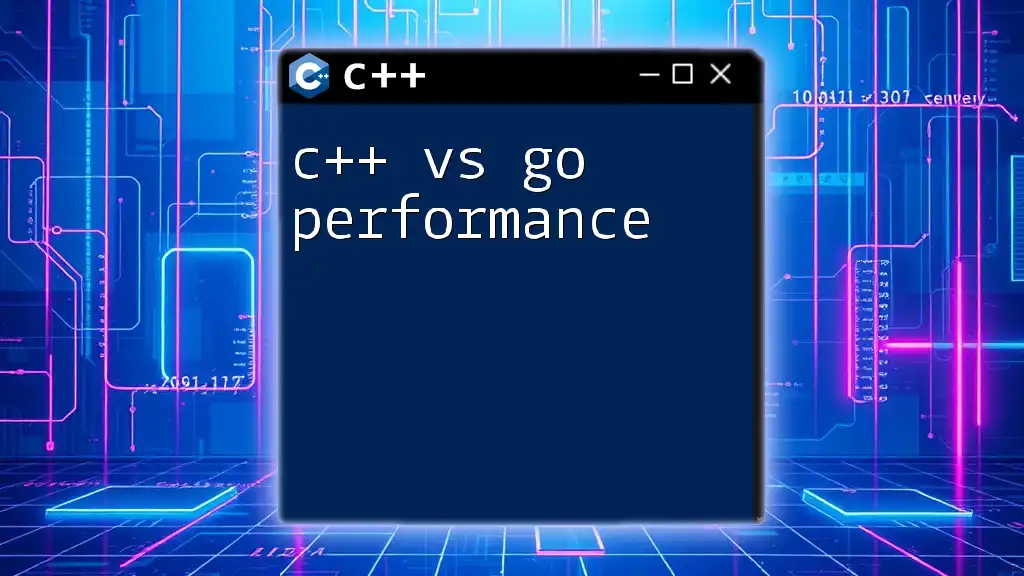
Differences in Language Features
Procedural vs Object-Oriented Programming
C is fundamentally a procedural programming language, which means it focuses on functions and the sequential flow of execution. C++, on the other hand, provides object-oriented programming (OOP) features, enabling greater abstraction and code reuse.
Here's a simple example to illustrate the difference:
// Procedural C example
#include <stdio.h>
void greet() {
printf("Hello, World!\n");
}
int main() {
greet();
return 0;
}
// Object-Oriented C++ example
#include <iostream>
class Greeter {
public:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
Greeter greeter;
greeter.greet();
return 0;
}
The procedural approach may be faster due to its simplicity, while C++ offers OOP benefits, which can lead to code maintainability and reusability even if there is a slight performance hit.
Memory Management
Memory management practices also differ dramatically between the two languages. In C, developers are responsible for manual memory management using functions like `malloc()` and `free()`. Failure to manage memory correctly can lead to memory leaks or segmentation faults.
In C++, memory management is somewhat simplified with the use of constructors and destructors for automatic initialization and cleanup of resources. For example:
// C dynamic memory allocation
#include <stdlib.h>
int main() {
int *array = (int*)malloc(10 * sizeof(int));
// ... use the array ...
free(array);
return 0;
}
// C++ dynamic memory allocation
#include <iostream>
class MyArray {
public:
MyArray(int size) {
array = new int[size];
}
~MyArray() {
delete[] array;
}
private:
int *array;
};
int main() {
MyArray myArray(10);
return 0;
}
In this example, C++ simplifies memory management with its object-oriented features, which can help reduce errors. However, improper use of these features can also lead to overhead, affecting performance.
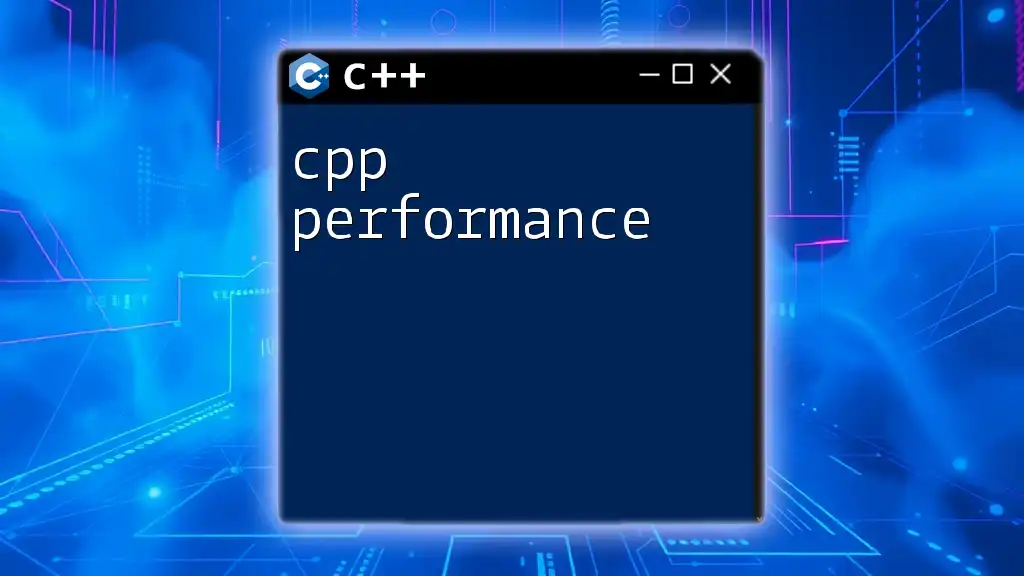
Performance Comparisons
Compilation Speed
When comparing C vs C++ performance, compilation speed can be crucial, especially in large projects. Generally, C compiles faster than C++ due to its simpler syntax and fewer complex features. This speed can accelerate the development cycle, particularly during testing and debugging.
Execution Speed
Execution speed, or runtime performance, can vary between C and C++ depending on the application. C is often faster for small, simple programs due to its lack of complex features. However, C++ can outperform C in larger, more complex applications, mainly due to optimizations like inlining and template metaprogramming.
Here’s a performance benchmark example where C may have the edge:
// C - simple loop
#include <stdio.h>
int main() {
for (int i = 0; i < 1000000; ++i) {
// Simulated task
}
return 0;
}
And a C++ equivalent:
// C++ - using templates
#include <iostream>
template <class T>
void simulatedTask(T value) {
for (int i = 0; i < value; ++i) {
// Simulated task
}
}
int main() {
simulatedTask(1000000);
return 0;
}
While the C version may execute faster for this simple task, C++ can shine in more complex scenarios that require abstraction and reusability.
Memory Usage
Memory usage is another critical factor. C generally has lower memory overhead due to its straightforward constructs and lack of features such as inheritance or polymorphism. C++ introduces additional memory costs for dynamic features, particularly when using classes or inheritance.
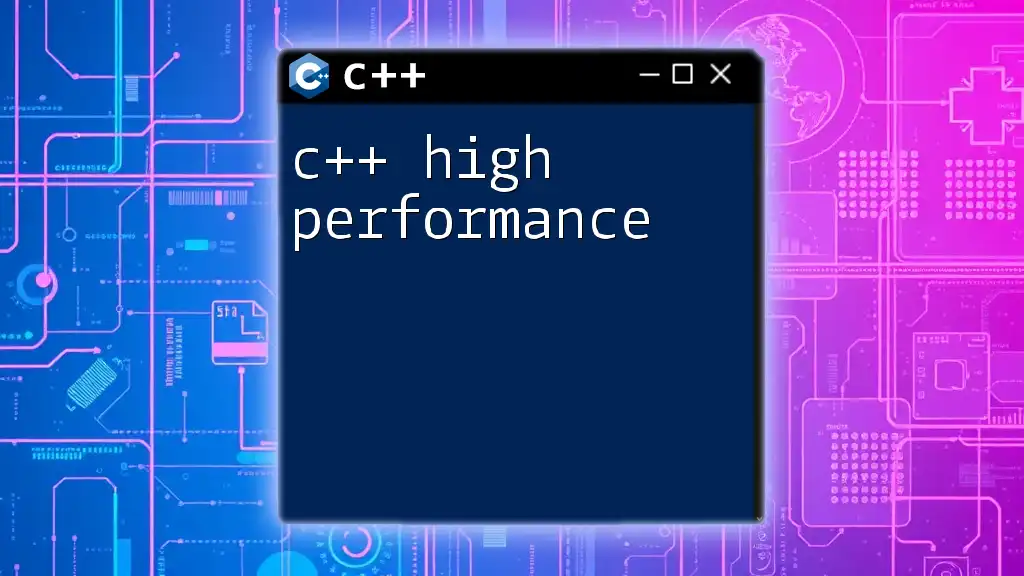
Real-World Use Cases
When to Use C
C excels in systems programming, where performance and hardware interactions are paramount. Examples include:
- Embedded Systems: Due to its low-level capabilities and efficient execution.
- Operating Systems: C is often chosen for operating system kernels because of its fine control over resources.
When to Use C++
C is not always the best choice, especially when project complexity increases. Scenarios where C++ shines include:
- Game Development: C++ allows for better architectural design, thanks to OOP principles.
- GUI Applications: Complex interface designs and event handling often benefit from C++'s object-oriented capabilities.
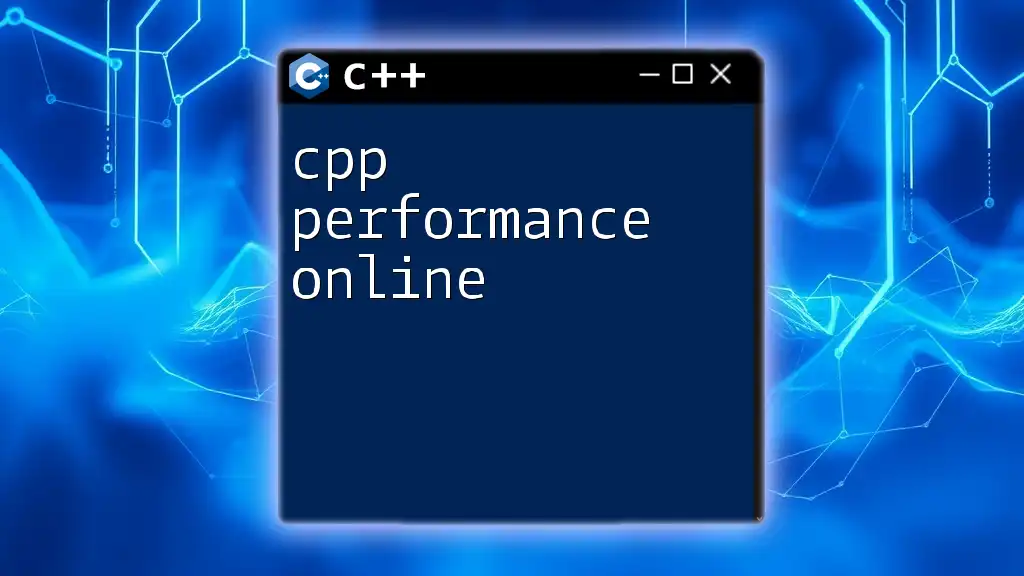
Optimization Techniques
C Optimization Strategies
Optimizing C code involves various strategies to improve performance:
- Use efficient algorithms suitable for your data.
- Minimize function calls in performance-critical parts of the code.
- Employ inline functions instead of macros where appropriate for speed.
Example of optimizing a simple function:
// C - before optimization
int addition(int a, int b) {
return a + b;
}
// C - after optimization
static inline int addition(int a, int b) {
return a + b;
}
C++ Optimization Strategies
C++ provides unique opportunities for optimization:
- Inlines: Use inline functions and member functions to reduce function call overhead.
- Templates: Utilize template metaprogramming to generate specialized code at compile time.
Here's a small optimization example:
// C++ - template for optimization
template <typename T>
T add(T a, T b) {
return a + b;
}
By using templates, you:
- Eliminate function call overhead.
- Generate code specific to the types involved.
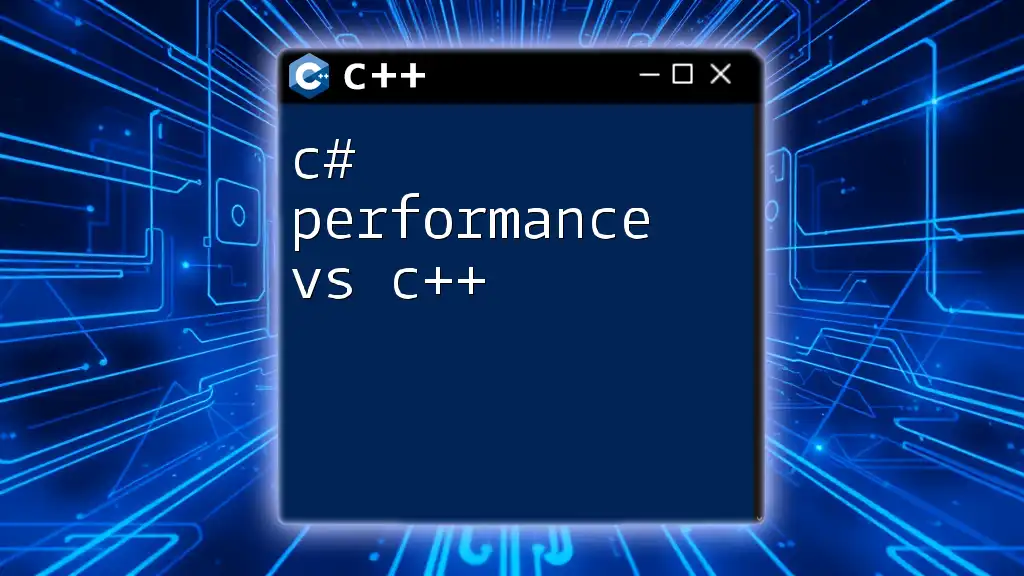
Performance Tools and Profilers
Tools for C Performance Analysis
Profiling tools are vital for optimizing C performance. Tools like gprof and Valgrind help you identify bottlenecks. By interpreting performance reports, you can focus on high-impact areas for improvement.
Tools for C++ Performance Analysis
For C++, tools such as Visual Studio Profiler and Google PerfTools provide extensive capabilities for analyzing performance. These tools help pinpoint inefficiencies, so you can fine-tune your C++ applications for optimal performance.
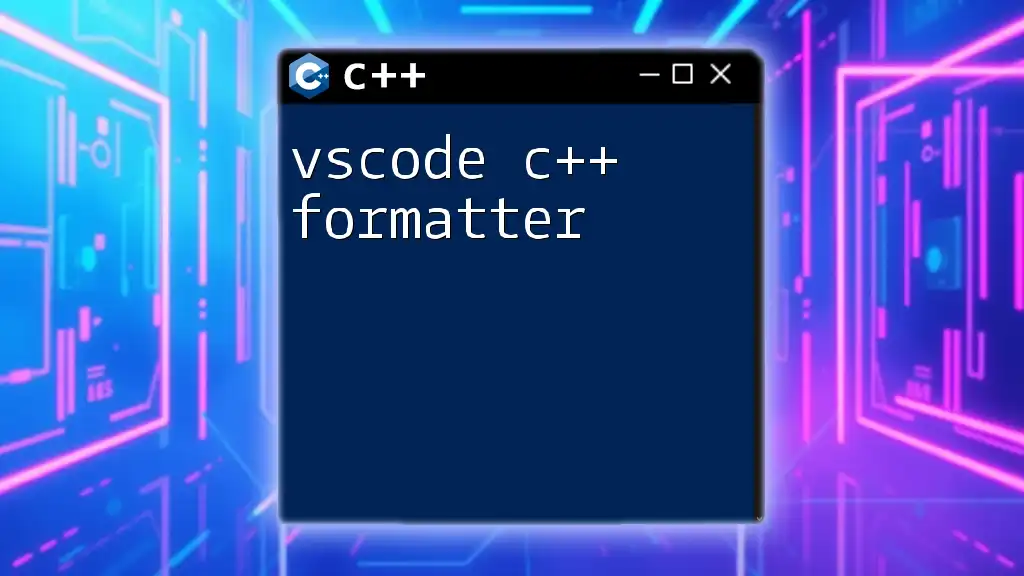
Conclusion
In comparing C vs C++ performance, it's clear that both languages offer unique strengths and weaknesses. C often provides superior performance in simpler applications, while C++ excels in more complex scenarios requiring abstraction. Understanding the fundamental differences in language features, memory management practices, and optimization techniques is essential for making informed decisions. Ultimately, the choice between C and C++ will depend on the specific needs and context of your project. Hands-on practice and continuous experimentation are encouraged to develop a deeper understanding of performance considerations in both languages.
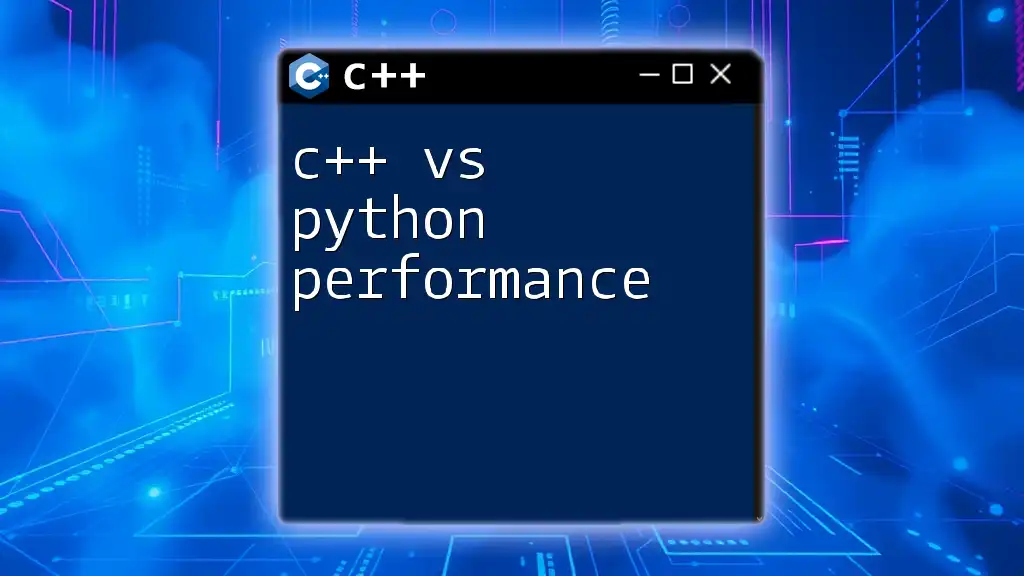
Additional Resources
Professional development and continued learning are essential for mastering performance optimization in C and C++. Consider delving into the following recommended reading materials, which offer deeper insights:
- Books: Look for comprehensive texts that explore advanced performance techniques.
- Online Communities: Engage in forums where programmers discuss optimization strategies and problems.