C++ typically offers superior performance compared to C# due to its lower-level memory management and optimization capabilities, making it a preferred choice for high-performance applications.
#include <iostream>
#include <vector>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
std::vector<int> numbers;
for (int i = 0; i < 1000000; ++i) {
numbers.push_back(i);
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Time taken: "
<< std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count()
<< " ms" << std::endl;
return 0;
}
Understanding Performance
What is Performance in Programming?
Performance in programming is critical to the efficiency and effectiveness of applications. It encompasses various aspects, such as CPU usage, memory management, and execution time. Developers often find themselves in situations where the performance of their code can significantly impact user experience, scalability, and resource allocation. Thus, understanding performance is essential for making informed language choices and coding techniques.
Factors Affecting Performance
Several factors contribute to the overall performance of a programming language.
- Algorithm Efficiency: The choice of algorithm and data structures has a profound impact on performance.
- Compiler Optimizations: How well a compiler can optimize code can lead to significant differences in execution speed.
- Runtime Environments: The underlying platform and environment in which the code runs also play a crucial role in determining performance.
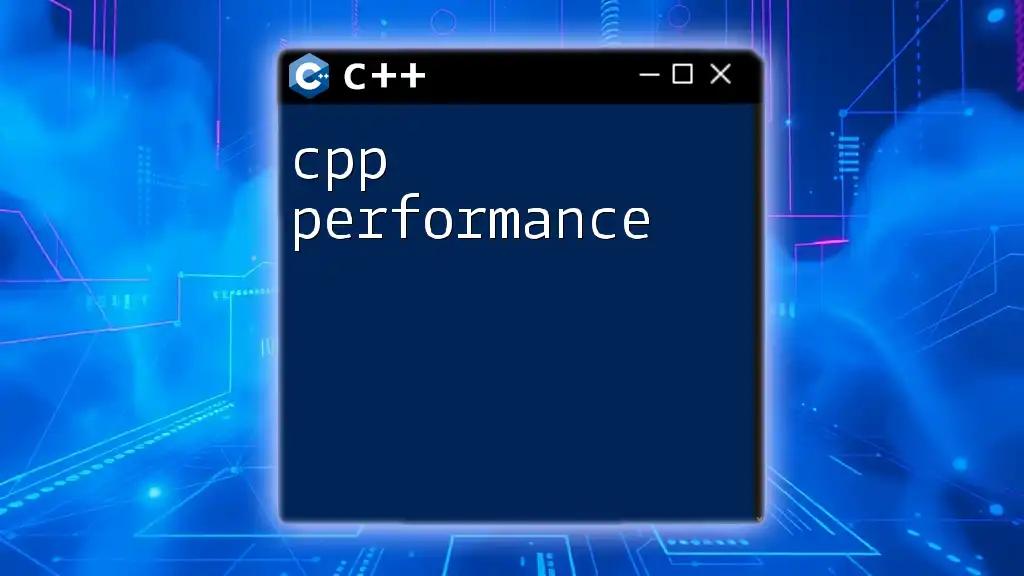
C# Performance Overview
C# Characteristics
C# is a high-level, managed language that makes it easy to develop applications rapidly. It abstracts many low-level details, allowing developers to focus on application logic rather than memory management. While this can enhance development speed, it can also impose limitations on performance due to its managed nature.
- Managed Code vs Unmanaged Code: C# runs on the Common Language Runtime (CLR), which provides automatic memory management and garbage collection, leading to safer but at times slightly slower execution compared to unmanaged languages like C++.
Garbage Collection in C#
Garbage collection (GC) is a process that automatically manages memory, reclaiming it when objects are no longer in use. While this can simplify development, it also comes with performance implications.
- Advantages: The primary benefit of garbage collection is reduced memory leaks, as developers are not manually responsible for freeing memory.
- Drawbacks: However, the GC introduces overhead during execution. When the garbage collector runs, it can pause application execution, leading to inconsistent performance, especially in time-sensitive applications.
JIT Compilation
C# uses Just-In-Time (JIT) compilation to convert Intermediate Language (IL) code into machine code at runtime. This method allows for optimizations based on the current execution environment.
- Effects on Performance: While JIT provides flexibility and optimizations, it can lead to slower startup times. The initial execution is often slower than precompiled languages since the code must be translated at runtime, affecting overall performance metrics.

C++ Performance Overview
C++ Characteristics
C++ is a powerful low-level programming language that allows direct manipulation of memory and system resources. This control enables developers to optimize applications extensively for performance.
- Direct Memory Access and Manipulation: Developers can use pointers and references to manipulate memory directly, often resulting in faster execution times. However, this flexibility comes with added responsibility, as improper memory management can lead to leaks or crashes.
Manual Memory Management
C++ provides developers with the capability (and duty) to manage memory manually. This includes allocating and deallocating memory when needed.
- Performance Implications: Although this allows for optimized memory usage, it can also lead to complex code that is harder to maintain. If managed correctly, manual memory management can significantly improve performance in high-demand scenarios.
Compile-Time Optimizations
C++ compilers perform various optimizations at compile time, including inlining functions, loop unrolling, and dead code elimination.
- Examples of Optimizations: Methods that might benefit from inlining can be defined with the `inline` keyword, which can enhance performance by eliminating function call overhead.
inline int square(int x) {
return x * x;
}
These compile-time optimizations can significantly improve the performance of applications, particularly those requiring high throughput.

C# vs C++ Performance on Common Use Cases
Desktop Applications
For desktop applications, C# provides a faster development cycle with tools and libraries like Windows Forms and WPF, which enhance UI development. However, C++ boasts superior performance, especially in computation-heavy applications.
Example code in C# for a simple desktop application:
using System;
using System.Windows.Forms;
public class MyForm : Form {
public MyForm() {
Text = "Hello World";
}
static void Main() {
Application.Run(new MyForm());
}
}
Conversely, a basic C++ application can be as follows:
#include <iostream>
int main() {
std::cout << "Hello World" << std::endl;
return 0;
}
Game Development
In game development, performance is paramount. C++ is the favored choice due to its capability to manage resources effectively and optimize for real-time requirements. Much of the game industry's architecture relies on the performance-driven features of C++. Unity, using C#, finds its place in more casual or indie game development, where speed may be exchanged for ease of use.
Web Applications
When it comes to web applications, frameworks like ASP.NET (C#) offer rapid development and a wealth of features. C++ can be utilized in backend services that require intense computational power or rapid data processing.
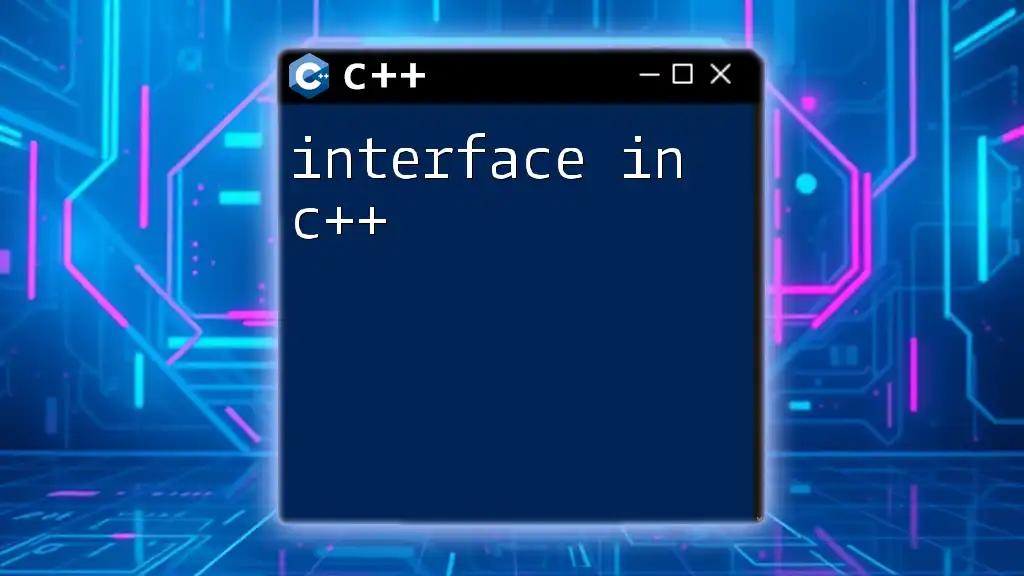
Performance Benchmarks
Key Performance Metrics
When evaluating performance, it is crucial to consider important metrics such as execution time, memory usage, and responsiveness.
Popular Benchmark Results
Multiple studies have showcased benchmarks comparing C# and C++. Typically, C# performs better in development speed, while C++, when optimized correctly, demonstrates superior execution speeds and less memory overhead.
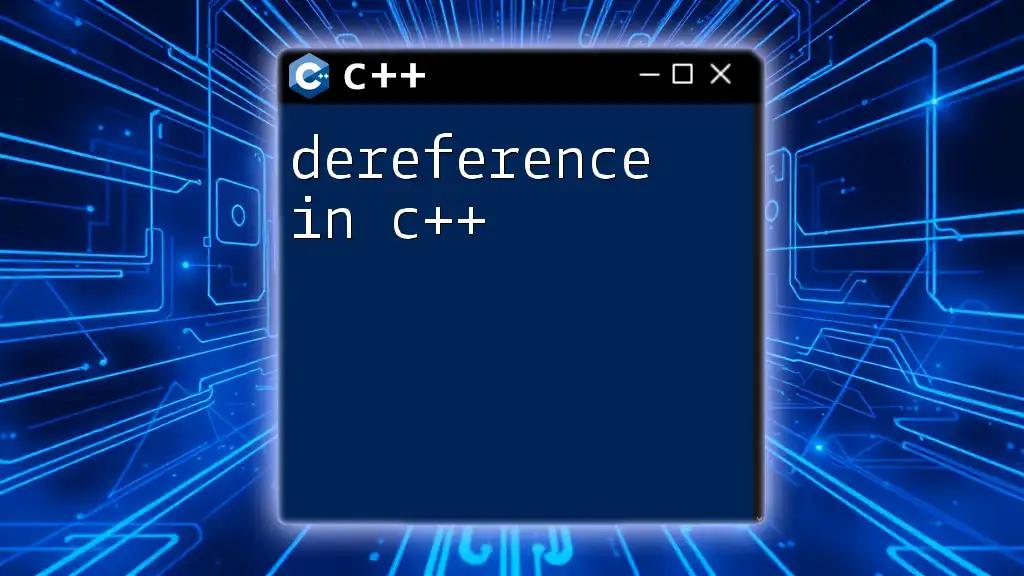
When to Choose C# Over C++
Situations Favoring C#
- Development Speed and Ease of Use: For business applications, C# allows for rapid prototyping and deployment.
- Rapid Application Prototyping: The managed environment enables faster iterations, making it ideal for projects with changing requirements.
Trade-offs for Performance
While C# may not always be as fast as C++, it can still serve most applications adequately, particularly in less demanding scenarios.
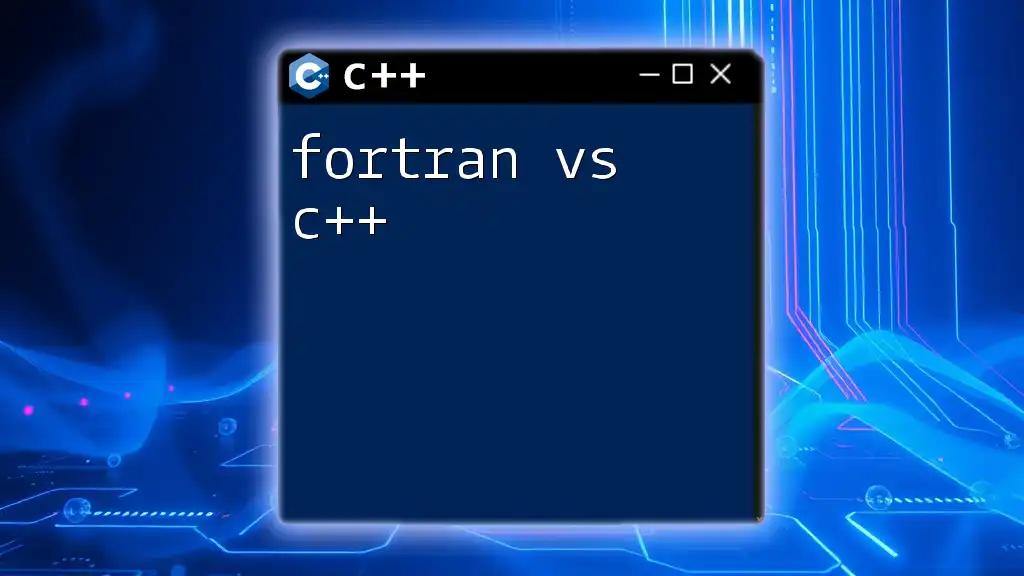
When to Choose C++ Over C#
Situations Favoring C++
- Performance-Critical Applications: C++ shines in real-time systems, game engines, and applications requiring direct hardware communication.
- Low-Level Hardware Programming Needs: If you need to optimize for specific hardware scenarios, C++ provides necessary tools.
Trade-offs for Usability
Using C++ may lead to more complex and less maintainable code, requiring experienced developers to manage the intricacies of manual memory allocation and potential errors effectively.

Conclusion
In the ongoing discussion of C# performance vs C++, it is essential to highlight the inherent strengths and weaknesses of both languages. C# provides ease of use and rapid development but may fall short in high-performance scenarios. C++, while challenging, offers the control and efficiency required for demanding applications. Ultimately, the choice depends on the specific needs of a project and the trade-offs developers are willing to navigate.