Python is known for its simplicity and readability, making it ideal for beginners, while C++ offers more control over system resources and performance, making it suitable for high-performance applications.
Here’s a simple comparison using a code snippet that demonstrates a basic "Hello, World!" program in both languages:
Python Example:
print("Hello, World!")
C++ Example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Basics
What is Python?
Python is an interpreted, high-level programming language first released in 1991. Its design philosophy emphasizes code readability, enabling developers to express concepts in fewer lines of code compared to other languages. Here are some key features of Python:
- Dynamic Typing: Variables do not need to be declared with a type; their type is determined at runtime.
- Extensive Libraries: Python boasts a vast collection of libraries, making it ideal for various applications, from web development to data science.
- Flexibility: It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
What is C++?
C++, created by Bjarne Stroustrup and released in 1985, is a compiled, high-performance programming language that adds object-oriented features to C. This makes it both powerful and versatile. Here are its notable features:
- Static Typing: Variables must be declared with a specific type, which leads to compilation errors if mismatched.
- Memory Management: Offers low-level access to memory management, allowing for fine-tuned performance optimizations.
- Multi-Paradigm: Supports procedural, object-oriented, and generic programming styles, enabling flexibility in implementation.
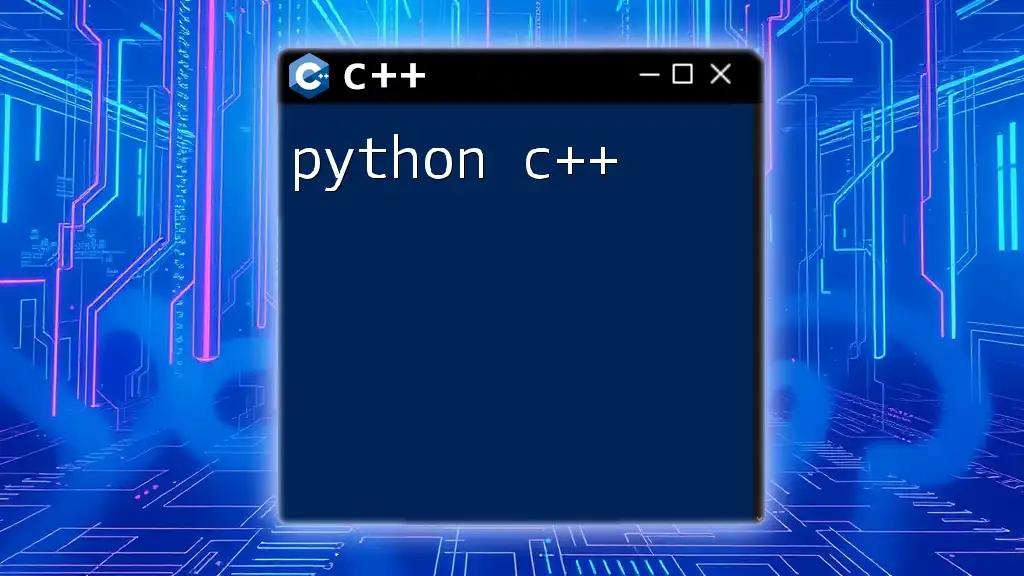
Syntax Comparison
Simplicity in Python
One of Python's most appealing aspects is its clean and readable syntax. This ease of reading fosters a faster learning curve for beginners. Consider the example below:
def greet(name):
return f"Hello, {name}!"
print(greet("World")) # Output: Hello, World!
Here, the function `greet` simply takes an input `name` and returns a formatted greeting string. It’s straightforward and intuitive, showcasing Python's ease of use. Readability is not just about aesthetics; it’s critical for maintainability and collaboration among developers.
Complexity in C++
In contrast, C++ has a more complex syntax that reflects its capabilities for performance and control. Here’s a basic C++ example:
#include <iostream>
std::string greet(std::string name) {
return "Hello, " + name + "!";
}
int main() {
std::cout << greet("World") << std::endl; // Output: Hello, World!
return 0;
}
While this example achieves the same output as the Python example, notice the verbosity required for declarations and libraries. This complexity arises from the need to explicitly manage types and memory, which can be an advantage for situations where performance is paramount.

Performance Comparison
Speed and Efficiency in C++
C++ is often preferred in situations that require high performance. Being a compiled language, C++ translates code into machine language, allowing programs to run more quickly and efficiently. For example, consider a simple performance benchmark scenario:
// C++ example
for(int i = 0; i < 100000000; ++i);
This operation executes at incredible speeds within a loop, demonstrating how C++ manages resources effectively. Performance is critical in applications like game development and real-time simulations, where lag can detract from user experience.
Suitability for Rapid Development: Python
On the other hand, Python shines in scenarios where rapid development and prototyping are required. Thanks to its extensive libraries and framework support, developers can quickly build robust applications. Python's speed of development is often seen in:
- Web Development: Frameworks like Django and Flask allow developers to create web applications rapidly.
- Data Analysis: Libraries such as Pandas and NumPy drastically simplify data manipulation and analysis tasks.
Thus, while C++ may excel in performance, Python is preferred for its agility and ease of use in development processes.
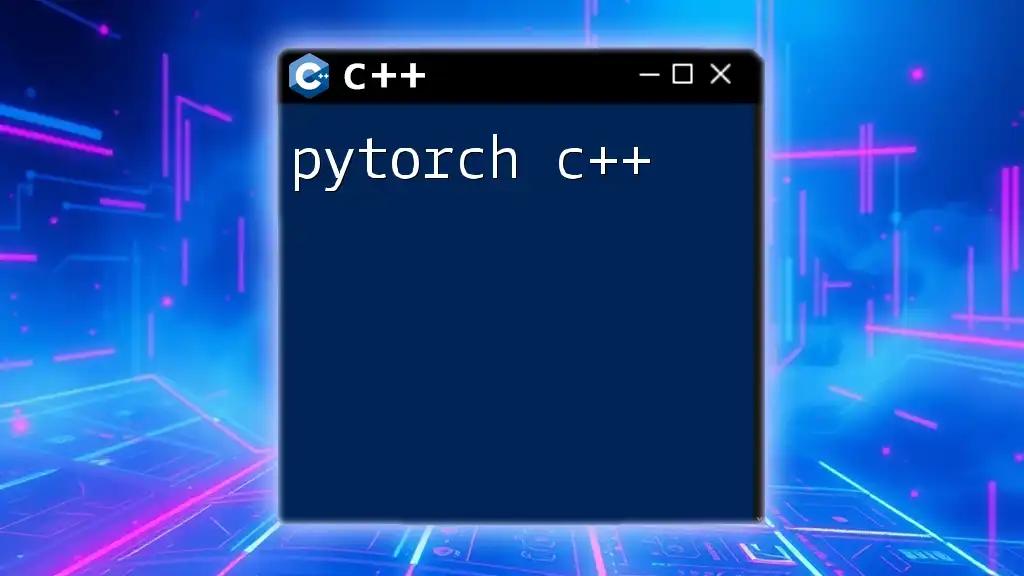
Use Cases and Applications
Where Python Shines
Python's versatility allows it to thrive in specific domains:
- Data Science and Machine Learning: With libraries like TensorFlow, SciPy, and Scikit-Learn, Python is a popular choice for researchers and data professionals.
- Web Development: Frameworks make it effortless to design interactive and powerful web applications.
- Automation and Scripting: Python scripts can automate repetitive tasks, making life easier for system administrators and developers.
C++ in Action
C++ is indispensable in performance-critical and systems-level programming. Key application areas include:
- Gaming and Real-Time Systems: Major game engines (like Unreal Engine) are built using C++, offering the performance required for high-quality graphics.
- System Programming: Operating systems and hardware-level interactions often rely on C++ for its low-level capabilities and efficiency.
- Performance-Critical Applications: Applications that require maximum efficiency, such as trading systems or scientific simulations, frequently utilize C++ for its performance strengths.
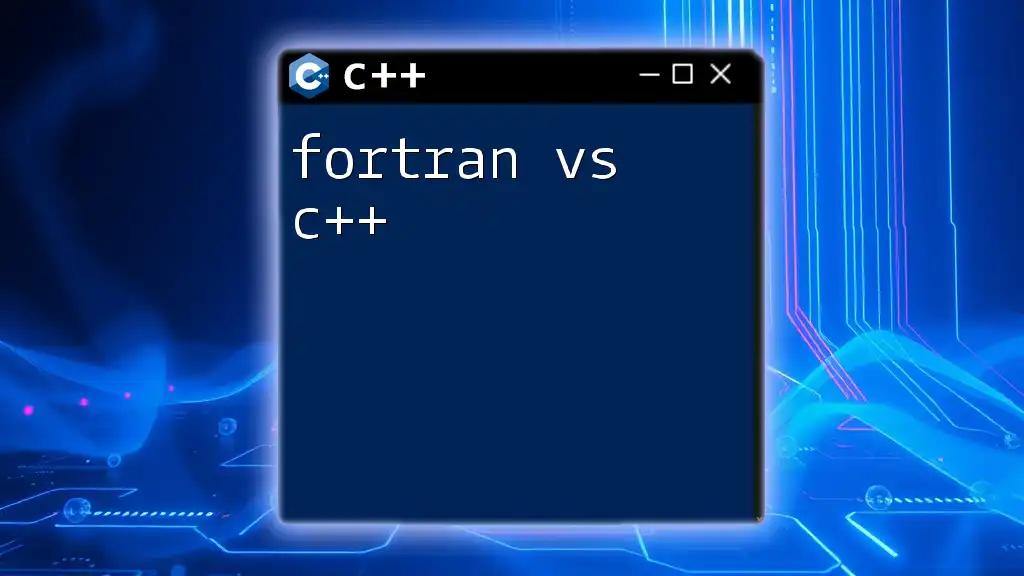
Community and Ecosystem
Python's Rich Ecosystem
Python has a vibrant ecosystem filled with resources, libraries, and active community support. Some of the most utilized libraries include:
- NumPy for numerical operations
- pandas for data manipulation
- Flask for web applications
This extensive ecosystem not only facilitates quicker development cycles but also fosters collaboration through community-driven resources, tutorials, and forums.
C++ Community Insights
The C++ community is robust, albeit with a steeper learning curve. Noteworthy libraries include:
- Boost for additional functionality
- SDL for multimedia applications
C++ is continuously evolving with new standards (like C++17 and C++20) aimed at modernizing the language while preserving its foundational principles. This ongoing development supports a vibrant community committed to pushing the language forward.

Learning Curve and Accessibility
How Easy is it to Start with Python?
Python's design makes it an excellent choice for beginners. The abundance of resources—tutorials, courses, and documentation—encourages newcomers to dive in. A simple project, like creating a web scraper, can engage beginners while providing instant feedback, enhancing the learning experience.
Challenges of Learning C++
While C++ offers tremendous capabilities, the learning curve can be daunting. Concepts like pointers, memory allocation, and template programming require a solid understanding of both programming and computer science fundamentals. However, grasping these foundational aspects equips developers with the knowledge to write efficient, robust programs.
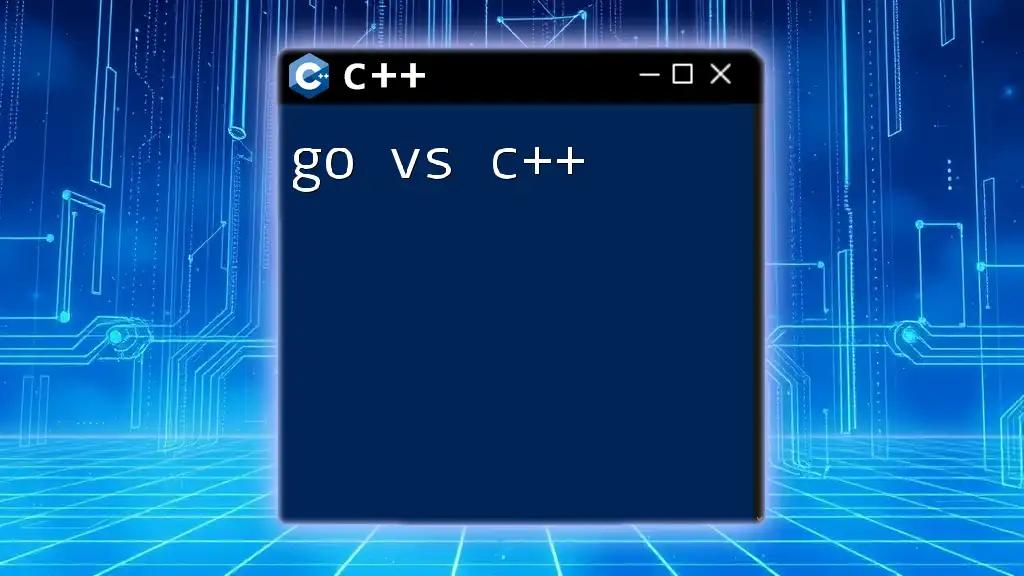
Conclusion
In summary, the debate of Python vs C++ is nuanced and highly dependent on the specific requirements of a project. C++ offers unparalleled performance and control, making it ideal for applications where resource management is critical. Python, conversely, provides rapid development capabilities and extensive libraries that simplify complex tasks, making it a go-to for many developers entering fields like web development and data science.
Ultimately, both languages have their unique strengths, and understanding their capabilities is essential for any developer. Exploring both Python and C++ can significantly broaden a programmer’s skill set, opening doors to various opportunities in the tech landscape.

Call to Action
We invite you to share your experiences in choosing between Python and C++. What projects have you undertaken using either language? How did you navigate challenges? Feel free to engage in the discussion or explore our offerings on learning C++ commands quickly and effectively.