A semaphore in C++ is a synchronization primitive that controls access to a shared resource by maintaining a set number of permits, ensuring that only a limited number of threads can access the resource concurrently.
Here's a simple example using the C++ standard library:
#include <iostream>
#include <thread>
#include <semaphore.h> // Ensure you have support for <semaphore>
std::binary_semaphore semaphore(2); // Allows up to 2 threads to access the resource
void accessResource(int id) {
semaphore.acquire(); // Wait for a permit
std::cout << "Thread " << id << " is accessing the resource.\n";
std::this_thread::sleep_for(std::chrono::seconds(1)); // Simulate resource access
std::cout << "Thread " << id << " is releasing the resource.\n";
semaphore.release(); // Release the permit
}
int main() {
std::thread threads[5];
for (int i = 0; i < 5; ++i) {
threads[i] = std::thread(accessResource, i);
}
for (auto& th : threads) {
th.join();
}
return 0;
}
Understanding Semaphores in C++
What is a Semaphore?
A semaphore is a synchronization primitive that restricts access to shared resources in multi-threaded applications. It is a signaling mechanism primarily used to avoid race conditions, where two or more threads attempt to modify shared data simultaneously. Semaphores help effectively manage resource allocation among threads.
Historical Background of Semaphores
Semaphores were first introduced by Edsger Dijkstra in the 1960s and have since become a critical part of concurrent programming. They serve as a key component in process synchronization and have been widely implemented across various programming languages and operating systems.
Why Use Semaphores?
In concurrent programming scenarios, especially in C++, threads run simultaneously, which can lead to unpredictable behavior if they access shared resources without proper synchronization. Semaphores solve this problem by providing a way to control access to these resources.
Utilizing semaphores provides several advantages:
- Concurrency control: They help manage the level of concurrency in an application.
- Prevent resource conflicts: By controlling access to shared resources, semaphores help avoid data corruption and inconsistencies.
- Efficient resource utilization: Semaphores enable optimal use of system resources by regulating how many threads can access a resource concurrently.
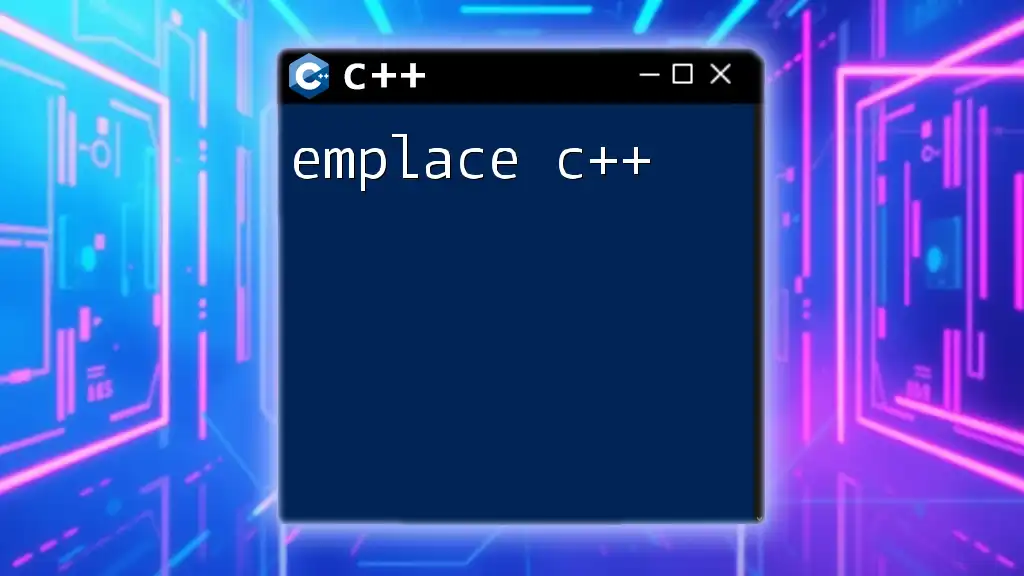
Types of Semaphores
Binary Semaphores
A binary semaphore is the simplest form of semaphore, only capable of taking two values: 0 and 1. It primarily acts as a simple lock, effectively controlling access to a single resource. Binary semaphores are useful in cases where a single instance of a resource needs exclusive access.
Counting Semaphores
Counting semaphores, on the other hand, can hold multiple values (non-negative integers). They allow multiple threads to access a specific number of instances of a resource simultaneously. This type of semaphore is particularly useful when managing a limited pool of resources, like database connections or threads in a thread pool.
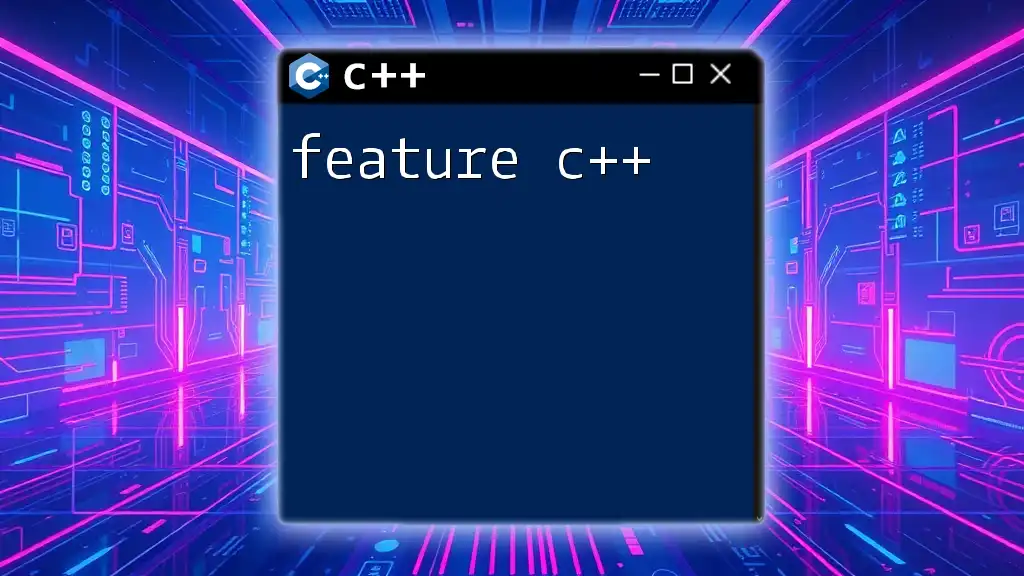
Implementing Semaphores in C++
Including Required Libraries
To use semaphores in your C++ program, you must include the relevant libraries. For C++20 and later, use the `<semaphore>` header, while for POSIX semaphores, use `<semaphore.h>`.
Here's how you include those libraries:
#include <semaphore.h> // Use for POSIX semaphores
#include <semaphore> // Use for C++20 and onward
Creating a Semaphore
Binary Semaphore Example
Creating a binary semaphore is straightforward. You can initialize it with a value of either 0 or 1:
std::binary_semaphore sem(1); // Initialize a binary semaphore with a value of 1
This line of code indicates that the semaphore is available for use.
Counting Semaphore Example
For counting semaphores, the syntax is quite similar. Here’s how you can initialize a counting semaphore:
std::counting_semaphore<1> sem(3); // Initialize a counting semaphore with a value of 3
This declaration allows up to three threads to access the shared resource concurrently.
Basic Operations on Semaphores
Wait (P Operation)
The Wait operation, often referred to as the P operation or acquire, is crucial in semaphore usage. When a thread calls this operation, it attempts to decrease the semaphore's value. If the value is 0, the thread blocks until the semaphore becomes available.
Here’s how you use the wait operation:
sem.acquire(); // Decreases the semaphore value
Signal (V Operation)
Conversely, the Signal operation – also known as the V operation or release – increases the semaphore's value, signaling that a thread has completed its work with the shared resource and is making it available for other threads to use.
Example code for the signal operation:
sem.release(); // Increases the semaphore value
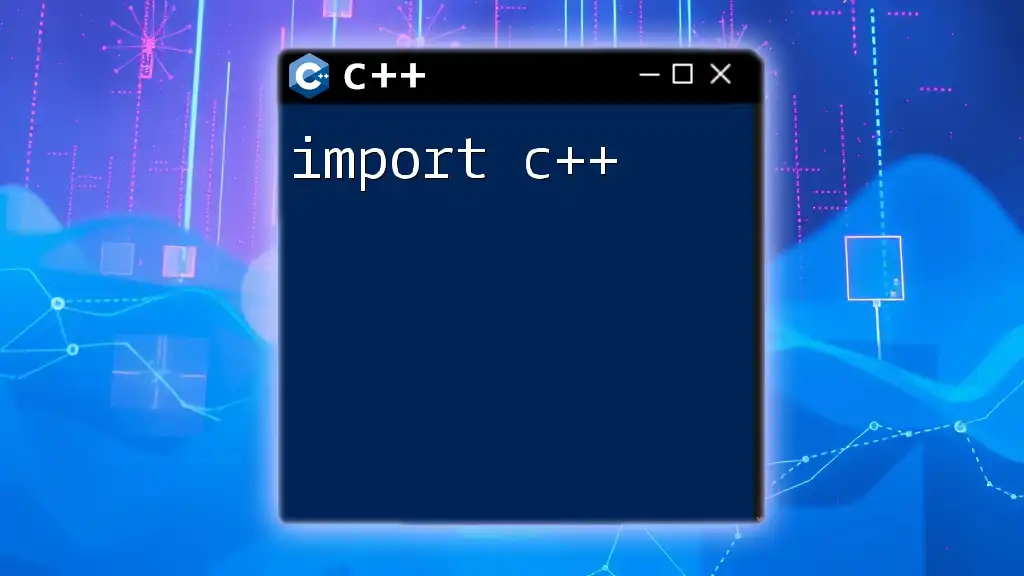
Using Semaphores in a Multithreaded Program
Creating Threads
In C++, you can create threads using `std::thread`. Threads allow you to run functions concurrently. Here's a basic example of how to create threads:
#include <thread>
#include <iostream>
#include <semaphore>
std::binary_semaphore sem(1); // A binary semaphore
void accessResource() {
sem.acquire(); // Wait for the semaphore
// Critical Section
// Here, you can access shared resources safely.
std::cout << "Accessing shared resource.\n";
sem.release(); // Signal the semaphore
}
int main() {
std::thread t1(accessResource);
std::thread t2(accessResource);
t1.join();
t2.join();
return 0;
}
Synchronizing Threads with Semaphores
In the above example, the `accessResource` function uses semaphores to synchronize access between two threads. When one thread enters the critical section and accesses the shared resource, the semaphore value decrements. The other thread is blocked until the semaphore is released.
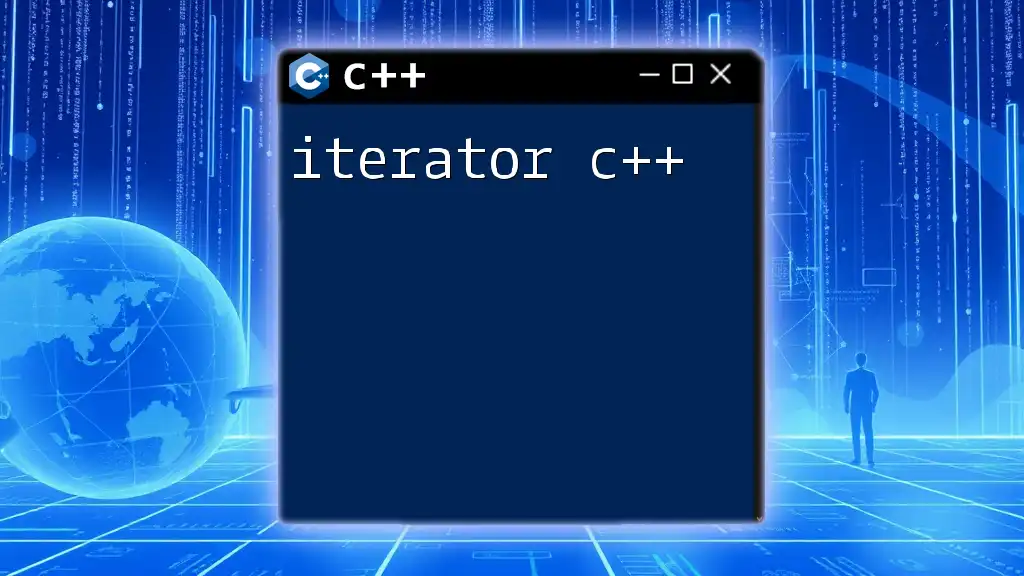
Best Practices for Using Semaphores
Avoiding Deadlocks
Deadlocks can occur when two or more threads are waiting indefinitely for each other to release resources. To prevent deadlocks, ensure that all threads acquire semaphores in a consistent order and consider implementing timeout mechanisms to avoid indefinite waiting situations.
Choosing the Right Semaphore
Selecting the appropriate semaphore type is crucial based on your project's requirements. Use binary semaphores for exclusive access scenarios, while counting semaphores are suited for limiting access to a defined number of shared resources.
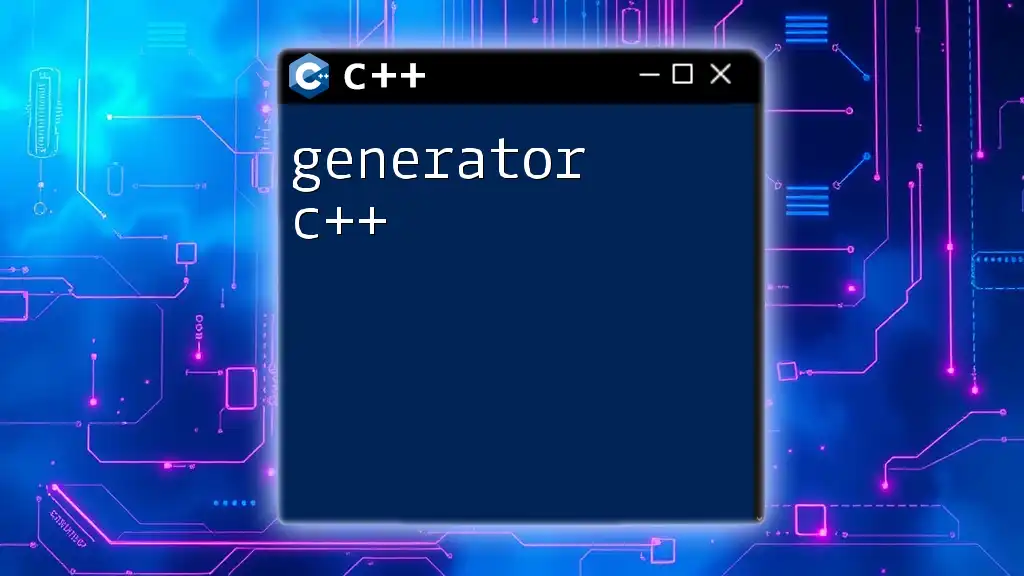
Common Pitfalls and How to Avoid Them
Misusing Semaphores
Common mistakes when using semaphores include not properly releasing semaphores after use or applying operations to undeclared semaphores. Such actions can lead to application crashes or undefined behavior. Always ensure that every acquire operation has a corresponding release.
Debugging Semaphore Issues
Debugging synchronization issues effectively can be challenging. When you encounter problems, consider logging semaphore values and thread statuses to track the flow of execution. Tools specifically designed for detecting deadlocks can also be invaluable.
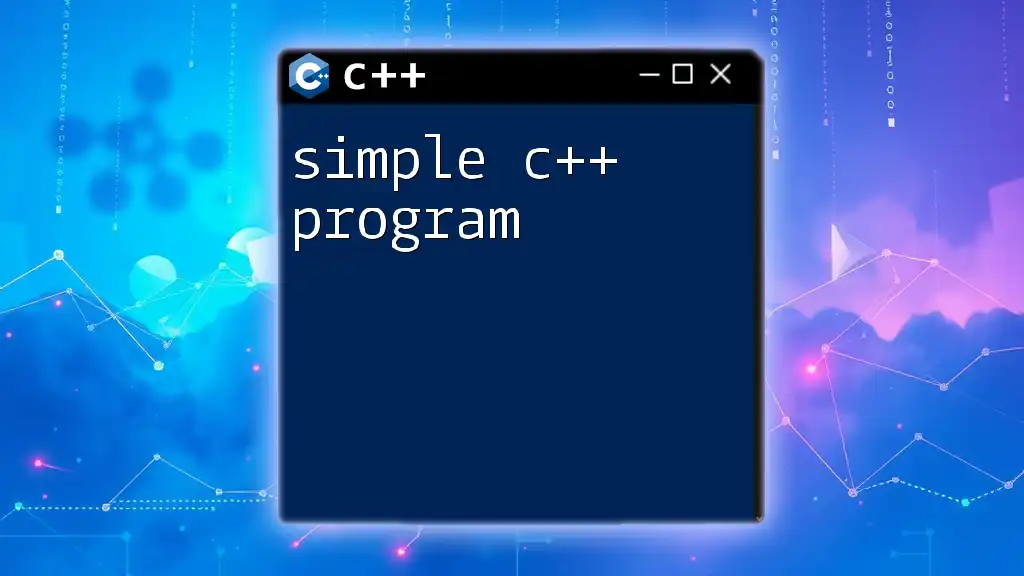
Conclusion
In conclusion, semaphores in C++ are powerful tools for managing resource access in multi-threaded applications. By understanding their types and applications, and adhering to best practices, you can avoid common pitfalls and create robust, concurrent applications. With this guide, you have the foundational knowledge necessary to implement semaphores effectively and efficiently in your projects.