`emplace` in C++ is a function that constructs an object in-place within a container, allowing for more efficient memory management by eliminating unnecessary copies.
Here’s a simple code snippet demonstrating the use of `emplace` in a `std::vector`:
#include <iostream>
#include <vector>
struct Point {
int x;
int y;
Point(int x, int y) : x(x), y(y) {}
};
int main() {
std::vector<Point> points;
points.emplace_back(1, 2); // Constructs Point(1, 2) in-place
points.emplace_back(3, 4); // Constructs Point(3, 4) in-place
for (const auto& point : points) {
std::cout << "(" << point.x << ", " << point.y << ")\n";
}
return 0;
}
What is `emplace` in C++?
`emplace` is a C++11 feature that allows for the direct construction of objects in containers, thus enabling better performance through in-place object creation. Unlike `insert` or `push_back`, which may create a temporary object and then move or copy it to the container, `emplace` constructs the object directly in the container's memory. This not only saves on unnecessary copy or move operations but also leads to improved performance, especially with complex objects.
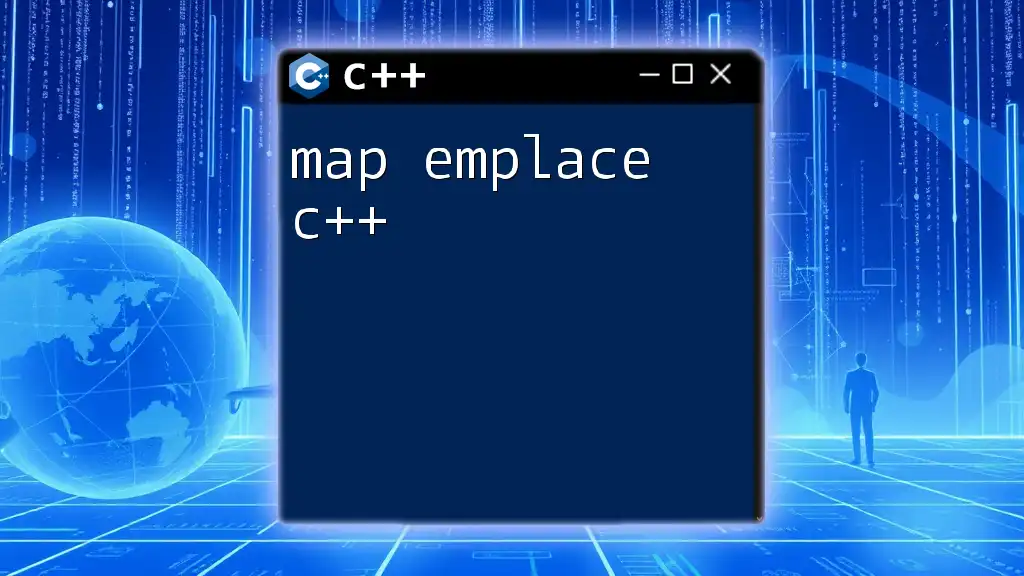
Why Use `emplace`?
The advantages of using `emplace` over traditional insertion methods are significant. By constructing the object in the place it will reside in the container, you minimize the overhead associated with moving or copying objects. This is especially beneficial in scenarios where:
- Performance matters: When working with large or complex objects, avoiding unnecessary moves and copies can yield noticeable performance improvements.
- Memory efficiency is key: Directly constructing objects in the container can reduce memory fragmentation and optimize allocation patterns.
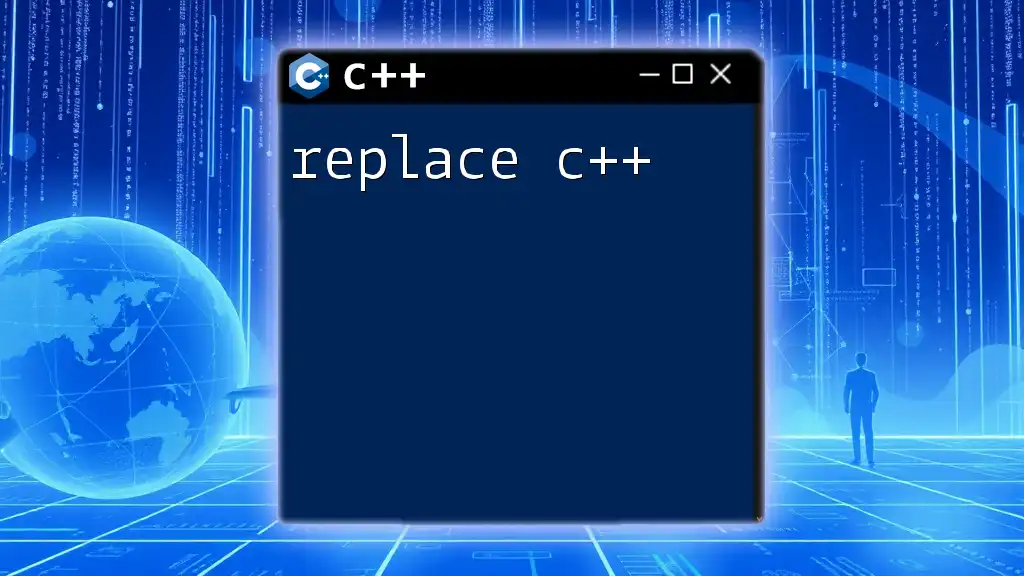
Understanding `emplace` in Different Containers
Using `emplace_back` with `std::vector`
`std::vector` utilizes `emplace_back` to construct an object at the end of the vector. This method allows the programmer to create an object in-place by passing the constructor arguments directly.
std::vector<MyClass> vec;
vec.emplace_back(constructor_args);
When you call `emplace_back`, the vector allocates memory and constructs the `MyClass` object with the provided arguments directly inside the vector. This eliminates the need for a temporary object, thus optimizing performance.
Using `emplace` for Key-Value Pairs in `std::map`
In `std::map`, the `emplace` function is particularly useful for inserting key-value pairs:
std::map<int, std::string> myMap;
myMap.emplace(1, "One");
myMap.emplace(std::piecewise_construct, std::make_tuple(2), std::make_tuple("Two"));
The first `emplace` directly constructs the key-value pair in the map without creating a temporary object. The second `emplace` uses `std::piecewise_construct`, allowing you to create the key and value using tuples. This is particularly advantageous when you want to construct complex objects.
Using `emplace` with `std::unordered_map`
Similar to `std::map`, `std::unordered_map` also supports the `emplace` method, providing an efficient way to add key-value pairs:
std::unordered_map<int, std::string> myUnorderedMap;
myUnorderedMap.emplace(3, "Three");
Using `emplace` ensures that the key-value pair is constructed directly in the unordered map, offering the same performance benefits as seen in `std::map`.
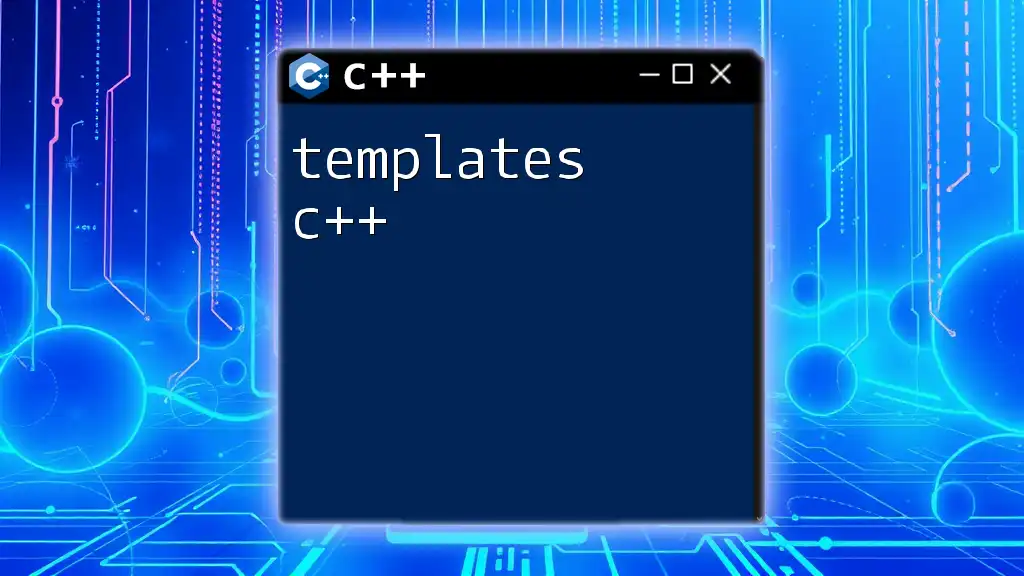
Detailed Syntax and Parameters of `emplace`
The syntax for `emplace` can vary slightly between container types but generally maintains a similar structure. Its primary signature can be summarized as:
void emplace(Args&&... args);
This allows for perfect forwarding of constructor arguments. The return type is often the iterator to the newly constructed element, depending on the container’s type.
Explaining Perfect Forwarding
Perfect forwarding is a powerful mechanism enabled by `emplace`. When you use `std::forward` within `emplace`, it allows the function to take advantage of the exact value category (lvalue, rvalue) of the passed arguments. This ensures that performance optimizations, like move semantics, are maintained.
template<typename... Args>
void emplace(Args&&... args) {
container.emplace(std::forward<Args>(args)...);
}
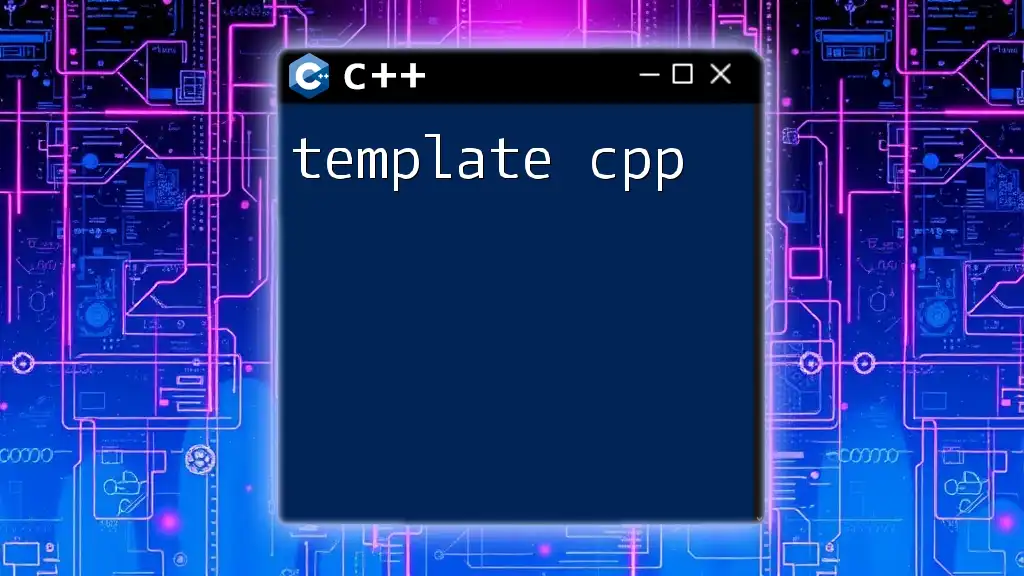
Common Use Cases for `emplace`
Construction of Complex Objects
When you need to construct complex objects with multiple data members, `emplace` makes life significantly easier. For instance, when using `std::vector` with `unique_ptr` objects:
std::vector<std::unique_ptr<MyClass>> vec;
vec.emplace_back(std::make_unique<MyClass>(constructor_args));
In this scenario, the unique pointer is created and stored in the vector directly, avoiding any copying of the object.
Using `emplace` in Custom Containers
Implementing `emplace` in your own data structures can enhance user experience by mimicking the behavior of standard containers. Imagine creating a simple linked list where each node can be emplaced:
class Node {
public:
Node(int value) : data(value), next(nullptr) {}
int data;
Node* next;
};
class LinkedList {
public:
void emplace(int value) {
head = new Node(value); // Direct construction
}
private:
Node* head = nullptr;
};
In this example, the custom container allows you to construct new nodes directly, similar to how standard containers work.
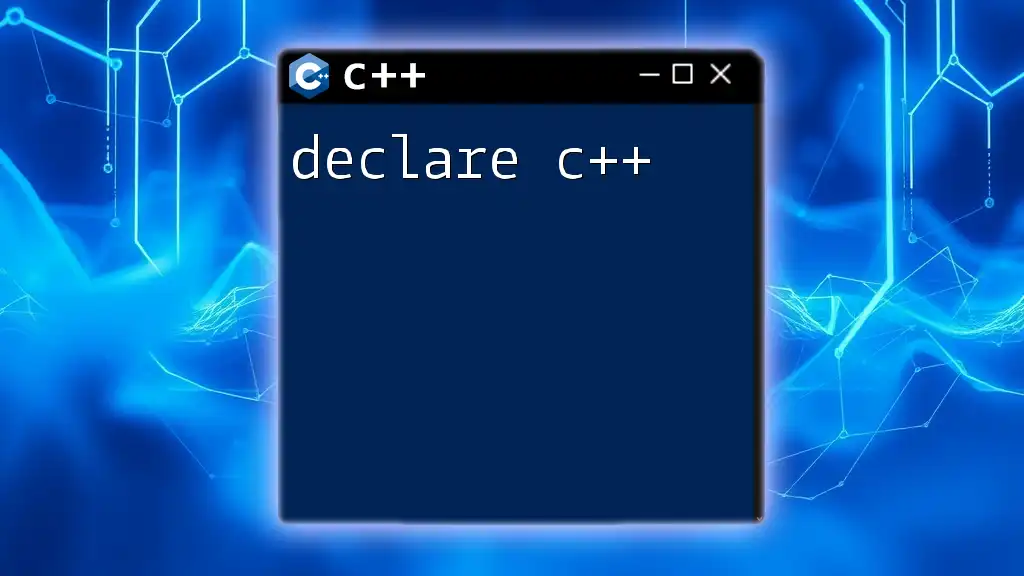
Performance Considerations
Performance Impact of Using `emplace` vs. `insert`
When considering whether to use `emplace` or `insert`, it’s vital to evaluate the specific use case. In many instances, `emplace` can significantly outperform `insert`, especially in contexts involving complex objects that would otherwise incur move or copy costs. Benchmarks often show notable discrepancies in execution time under heavy load conditions.
Memory Efficiency
Memory management is crucial in performance-sensitive applications. By employing `emplace`, you can minimize memory overhead and reduce the likelihood of fragmentation. Particularly when constructing large numbers of objects, observing the pattern of allocation becomes essential, and `emplace` can help streamline this process.
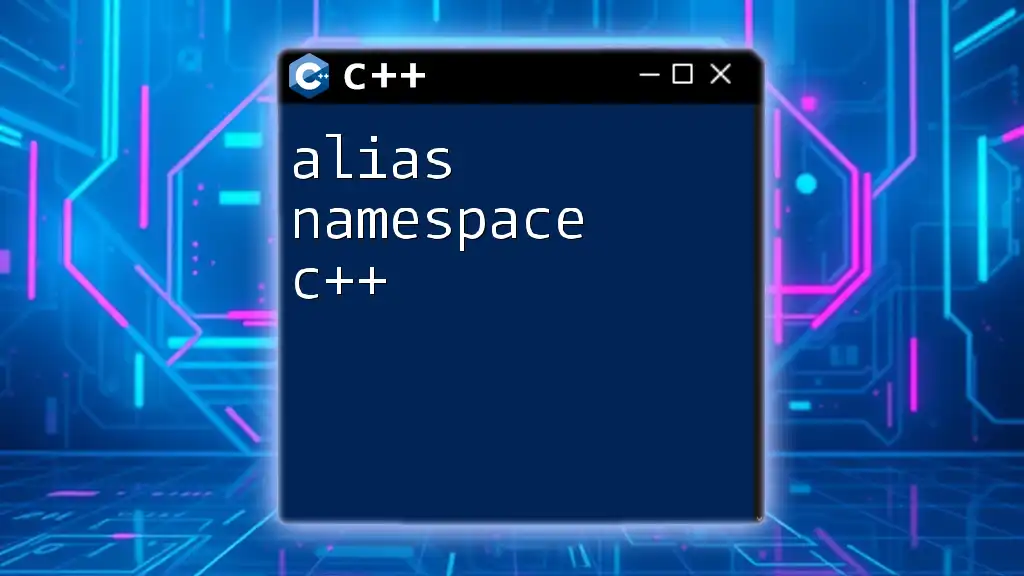
Common Pitfalls and Misconceptions
Common Errors with `emplace`
Despite its advantages, developers can easily misuse `emplace`. A common mistake involves attempting to pass objects that do not match the expected constructor signature, leading to unexpected outcomes or compilation errors. Always ensure that the arguments used with `emplace` align with the constructors of the objects being created.
How to Debug Common Problems
Debugging issues that arise with `emplace` can be challenging. If you encounter runtime errors, check that the correct types and numbers of arguments are provided. Compiler error messages will often guide you toward mismatched types, so pay close attention to the messages generated.
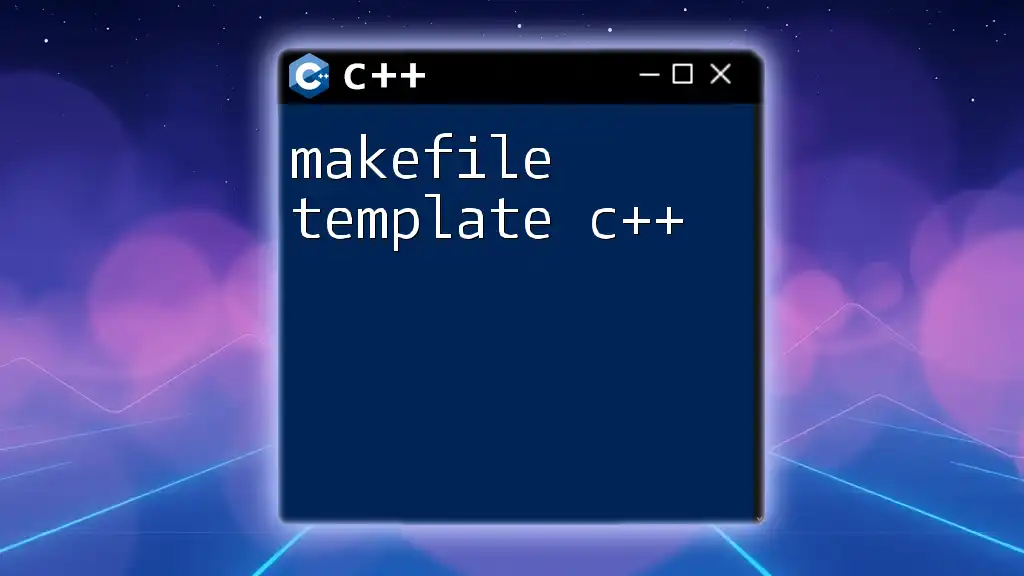
Recap of Key Points
The use of `emplace` in C++ provides considerable benefits, including improved performance and memory efficiency. By allowing in-place construction of objects within containers, `emplace` empowers developers to write cleaner, faster, and more resource-efficient code.
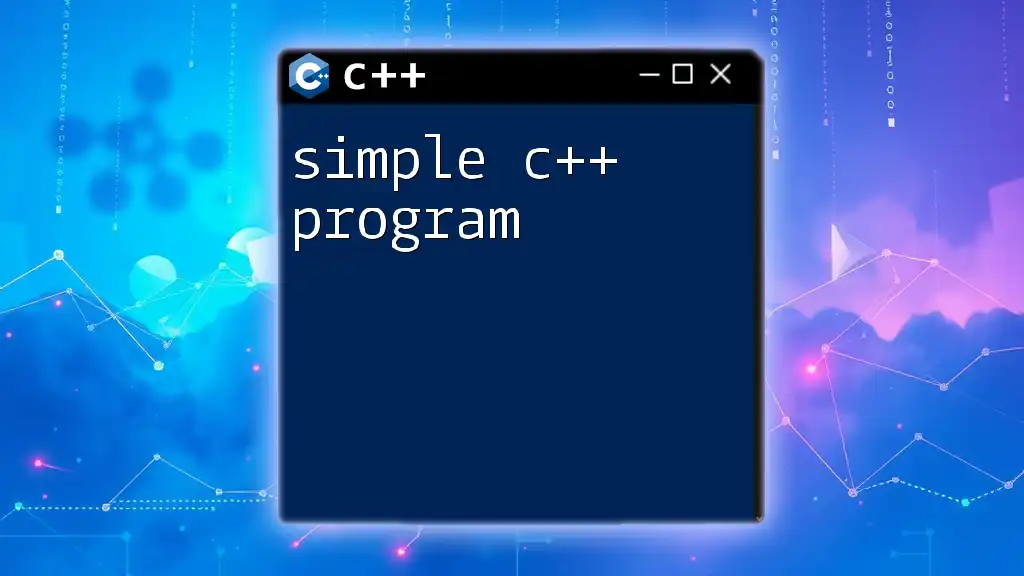
Encouragement to Experiment
I invite you to practice using `emplace` with various C++ standard containers. By experimenting with real-world scenarios, you will deepen your understanding and appreciation for this essential feature of modern C++. Don't hesitate to try it out with different object constructions to see its powerful implications in action!
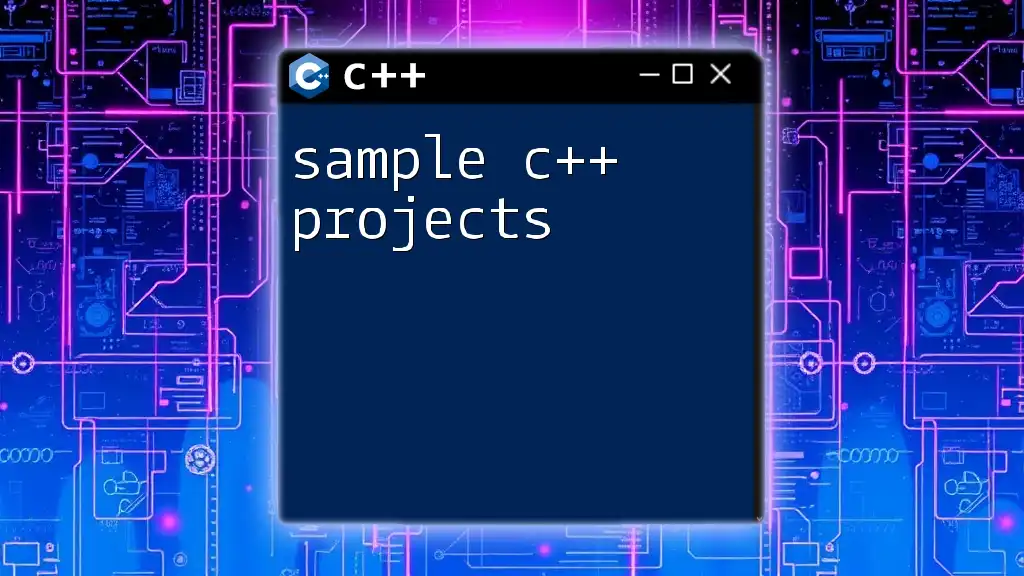
Additional Resources
To further your understanding of `emplace`, consider checking out the official C++ documentation, which provides detailed explanations and examples. Additionally, engaging with community forums can offer deeper insights and discussions about best practices when utilizing `emplace` in your C++ projects.