In C++, the `erase` function is used to remove elements from a container, such as a vector or a list, based on their position or value.
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.erase(numbers.begin() + 2); // Erases the element at index 2 (3)
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 4 5
}
return 0;
}
Understanding C++ Containers
C++ containers are a crucial part of the standard library, designed to store collections of objects. They provide a way to manage and manipulate data efficiently. Common containers include `vector`, `list`, `set`, and `map`, each with its unique characteristics and use cases.
Using these containers allows developers to work with groups of data without having to manage the underlying memory, freeing them to focus on algorithmic solutions.
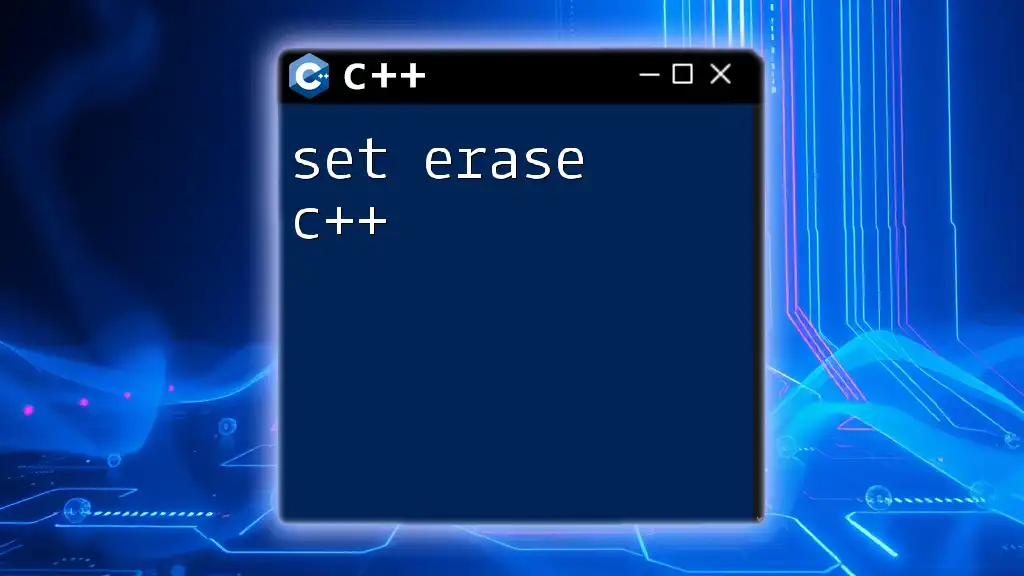
The `erase` Function: Basics
The `erase` function is employed to remove elements from containers in C++. This powerful function enhances data management and can significantly improve performance when employed correctly.
Syntax of `erase`
The syntax for `erase` varies slightly based on the type of container. The general format is:
container.erase(position);
Here, `position` can be an iterator pointing to the element to be erased or the first iterator of a range to be erased.
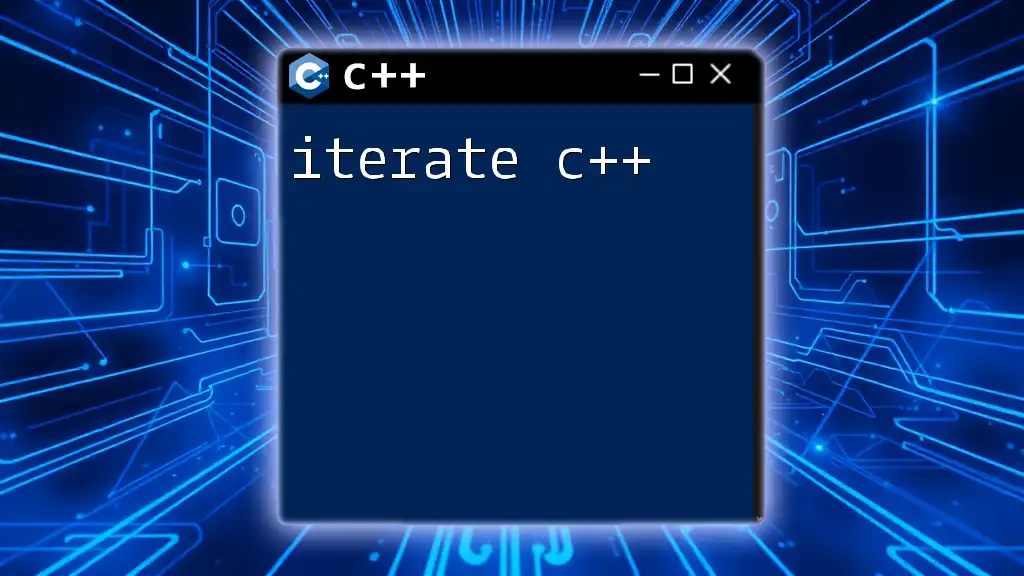
Using `erase` with Different Containers
Erasing Elements from a `vector`
The `vector` is perhaps the most commonly used container in C++. It allows dynamic sizing and efficient access to elements. The `erase` function can remove specific elements or entire ranges.
To remove a single element from a `vector`, you simply specify its index with an iterator:
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the element at index 2
In the code above, the element at index 2, which is `3`, is removed from `vec`.
To erase a range of elements, specify two iterators:
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 1, vec.begin() + 4); // Removes elements from index 1 to 3
Here, elements `2`, `3`, and `4` are erased, leaving `1` and `5`.
Erasing Elements from a `list`
A `list` is another container that allows for constant time insertions and deletions from anywhere in the list. This makes it particularly useful when dealing with large datasets.
To remove an element from a `list`, you can use:
std::list<int> lst = {1, 2, 3, 4, 5};
auto it = lst.begin();
std::advance(it, 1);
lst.erase(it); // Removes the second element
In this example, the second value `2` is removed seamlessly.
Erasing Elements from a `set`
Sets do not allow duplicate values, which makes them ideal for storing unique items. The `erase` function in a `set` accepts the value to be removed:
std::set<int> mySet = {1, 2, 3, 4, 5};
mySet.erase(3); // Removes the element 3
With this call, `3` is removed from `mySet`, showcasing the simplicity of managing unique elements.
Erasing Elements from a `map`
For mappings of keys to values, the `map` container is invaluable. The `erase` function works by specifying the key of the element to be deleted:
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}};
myMap.erase(1); // Removes the entry with key 1
In this instance, the pair with the key `1` is erased, demonstrating the ease of managing key-value associations.
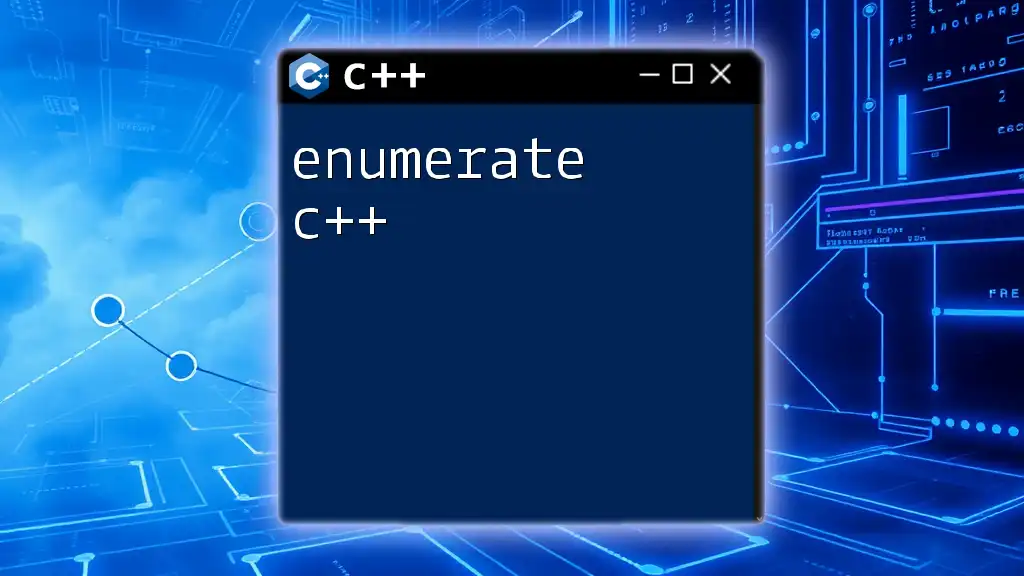
`erase` with Iterators
Iterators provide a powerful way to traverse and manipulate container elements. Notably, when using `erase`, you can pass iterators to indicate the specific element you want to remove:
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end())
vec.erase(it); // Erase using iterator
In this example, `std::find` is used to search for the value `3`, and if found, it is removed using the iterator.
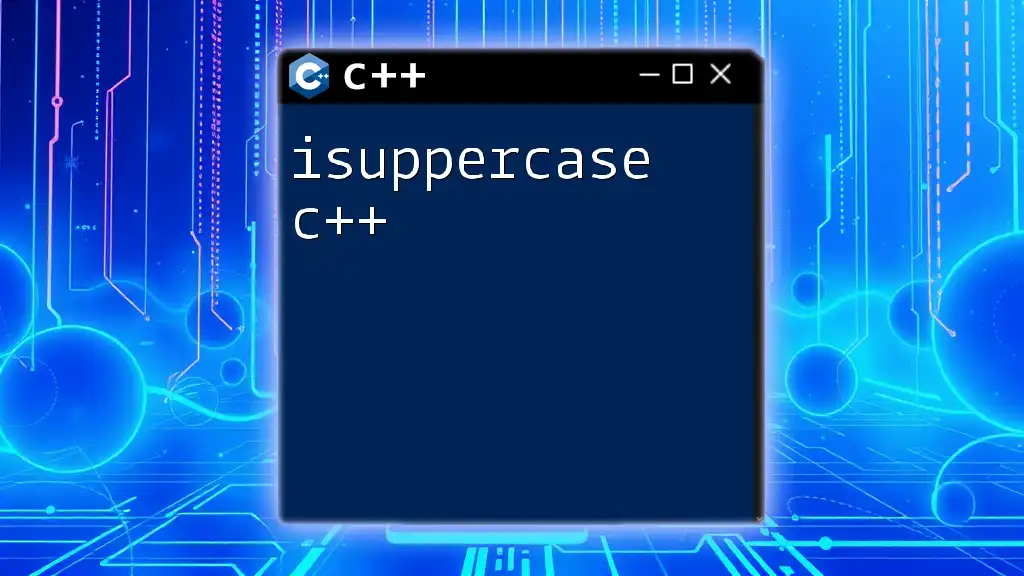
Performance Considerations
Understanding the performance impact of using `erase` is essential. The time complexity of `erase` varies based on the type of container. For instance, removing an element from a `vector` has a complexity of O(n) since it may require shifting elements. In contrast, operations on a `set` or `map` are generally O(log n) due to the underlying tree structures they use.
When using `erase`, especially in loops, it’s important to assess the efficiency of your approach. Frequent use of `erase` in `vector` can lead to performance bottlenecks as it may result in many allocations and copies.
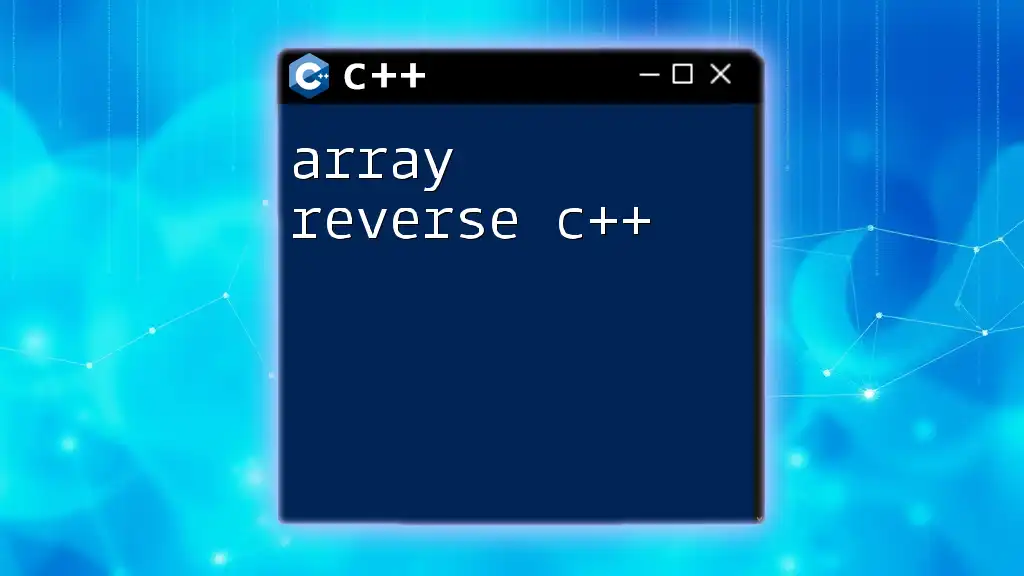
Common Pitfalls and Best Practices
When using `erase`, developers must be conscious of iterator invalidation. After erasing an element, any iterators pointing to that element become invalid. This can lead to undefined behavior if not handled properly.
Best Practices for Using `erase`
- Avoid using `erase` inside a loop over a container, as it can lead to skipped elements or out-of-bounds access. Instead, consider the combination of `remove_if` and `erase`, known as the erase-remove idiom.
Example of Using `remove_if` with `erase`
std::vector<int> vec = {1, 2, 3, 4, 5, 6};
vec.erase(std::remove_if(vec.begin(), vec.end(), [](int x) { return x % 2 == 0; }), vec.end());
In the code above, all even numbers are removed efficiently. `remove_if` reorganizes the vector so that all elements that satisfy the condition are moved to the end, and then `erase` removes them in a single operation.
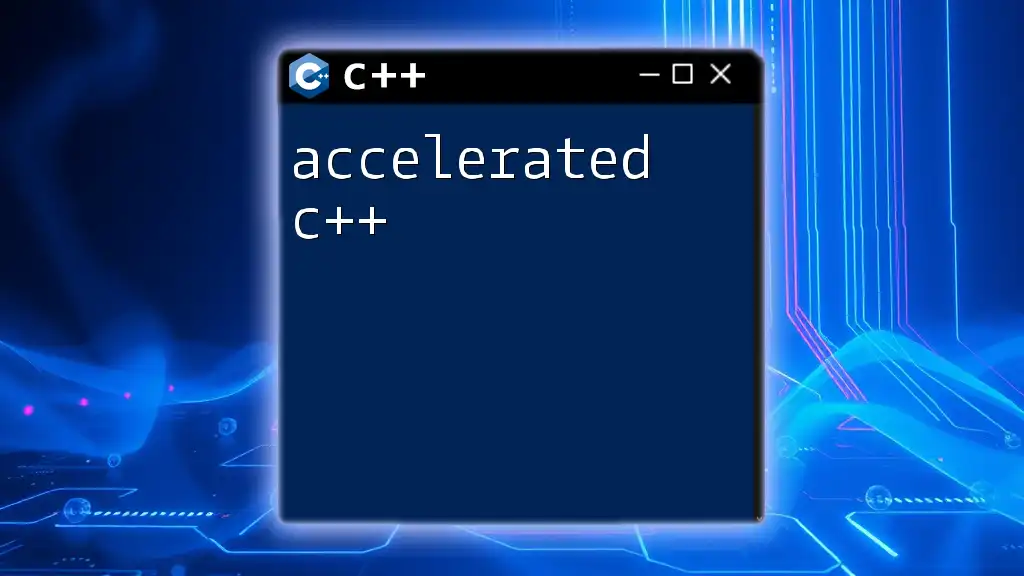
Conclusion
The `erase` function is a vital tool for managing data in C++. Understanding how to use `erase` effectively across different containers not only simplifies code but also enhances performance. By following best practices and being aware of the implications of element removal, developers can create efficient and maintainable C++ applications.
With a solid grasp of the `erase` function, you can effectively manipulate data structures and make your C++ programs more robust. To further improve your understanding, consider diving deeper into documentation and tutorials, or practice your skills through hands-on coding challenges.