A trie, or prefix tree, is a specialized tree data structure used to efficiently store and retrieve keys in a dataset of strings, where nodes represent characters of the keys.
#include <iostream>
#include <unordered_map>
#include <vector>
using namespace std;
class TrieNode {
public:
unordered_map<char, TrieNode*> children;
bool isEndOfWord;
TrieNode() : isEndOfWord(false) {}
};
class Trie {
private:
TrieNode* root;
public:
Trie() {
root = new TrieNode();
}
void insert(const string& word) {
TrieNode* node = root;
for (char ch : word) {
if (!node->children.count(ch)) {
node->children[ch] = new TrieNode();
}
node = node->children[ch];
}
node->isEndOfWord = true;
}
bool search(const string& word) {
TrieNode* node = root;
for (char ch : word) {
if (!node->children.count(ch)) {
return false;
}
node = node->children[ch];
}
return node->isEndOfWord;
}
};
What is a Trie?
A trie is a specialized tree-like data structure that stores a dynamic set of strings, typically used for retrieval. Unlike binary search trees and other similar structures, a trie connects characters by their prefixes: the path from the root to a node represents a prefix of some words. This unique design allows for efficient insertions and queries, making tries particularly useful in various applications, such as auto-completion and spell checking.
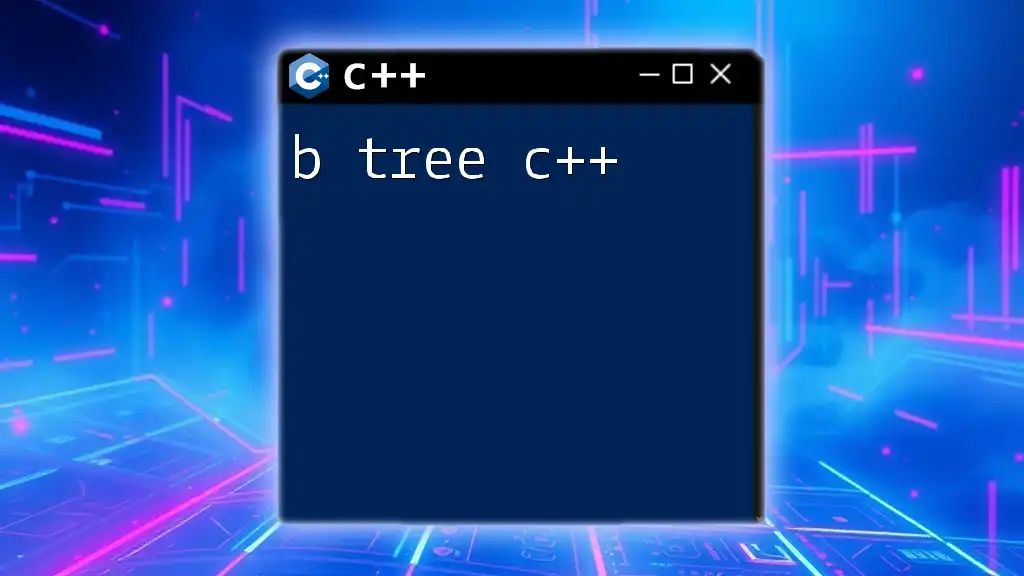
Common Use Cases for Tries
Tries are widely applicable in numerous domains:
- Auto-completion: When a user starts typing, a trie can quickly suggest possible completions from a large dictionary.
- Spell checking: Tries can efficiently store and verify words, identifying possible corrections for input errors.
- Storing dictionaries: Tries offer a compact representation of the word set, minimizing the memory footprint especially when dealing with large datasets.
- IP routing: In networking, tries are used to manage IP address storage and routing decisions.
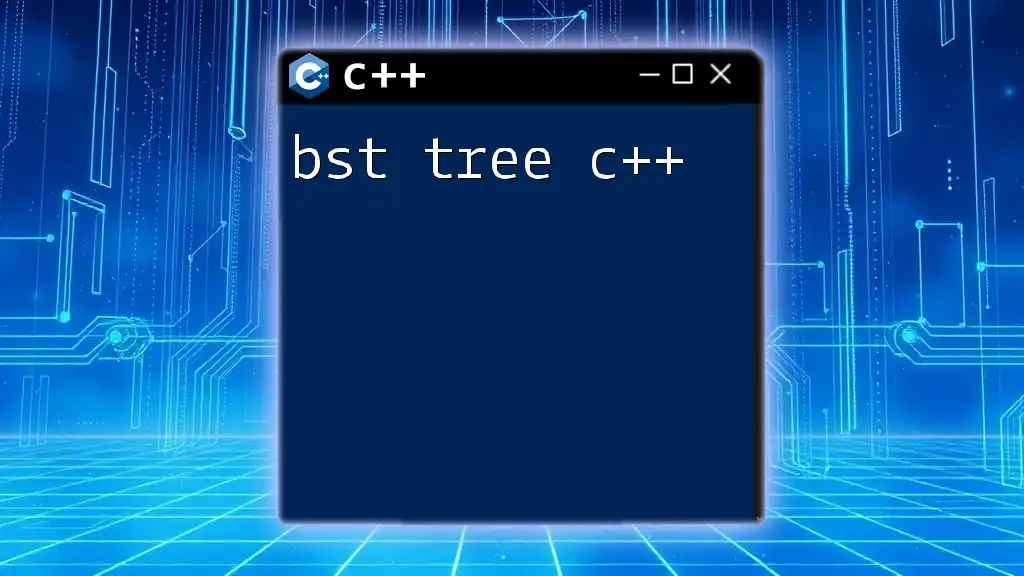
Understanding the Structure of a Trie
Basic Components of a Trie
A trie is made up of nodes, where each node contains:
- Child pointers: Each node can point to multiple children, representing subsequent characters in the stored words.
- End of Word flag: A boolean flag indicating whether a complete word ends at that node.
Properties of a Trie
Tries have several fundamental properties:
- Memory efficiency: Although tries can consume more memory than other structures for small datasets, they excel with larger collections of strings due to their prefix-sharing nature.
- Time complexity: For insertions, deletions, and searches, tries provide an average time complexity of O(m), where m is the length of the word being processed.
Visual Representation of a Trie
Visualizing a trie may help in understanding its structure. Each node can be represented as a character, and the path taken from the root node represents the stored strings.
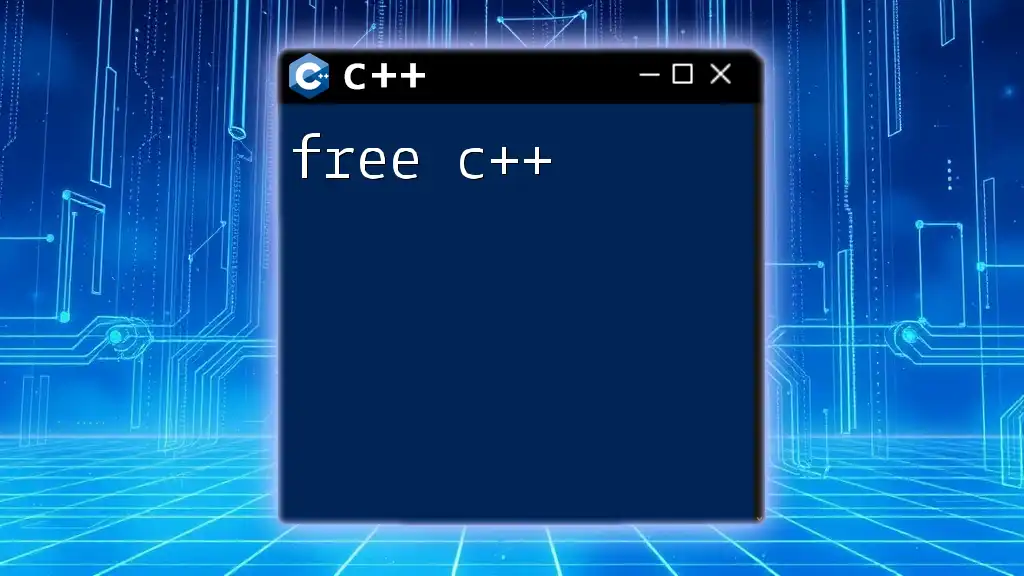
Implementing a Trie in C++
Setting Up the Trie Node Structure
Before creating the trie class, we need to define the structure for the trie nodes:
struct TrieNode {
TrieNode *children[26]; // Assuming only lowercase letters
bool isEndOfWord; // Flags if a word ends at this node
};
Creating the Trie Class
Next, we define a trie class that includes essential methods for interaction:
class Trie {
private:
TrieNode *root;
public:
Trie(); // Constructor
~Trie(); // Destructor
void insert(const std::string &word);
bool search(const std::string &word);
bool startsWith(const std::string &prefix);
void remove(const std::string &word);
};
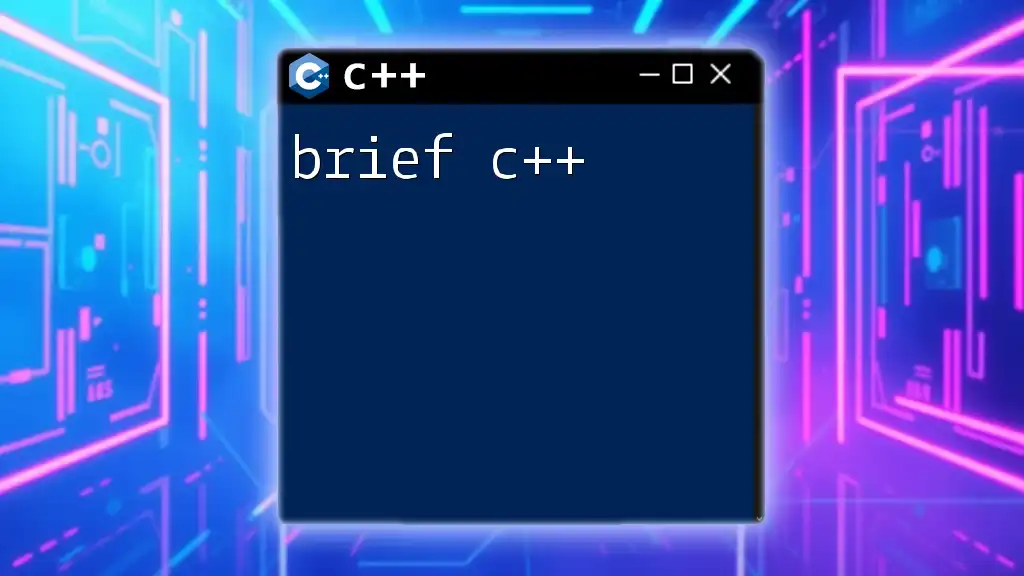
Inserting Words into a Trie
To insert a word into a trie, the process involves navigating through each character and creating a new node when necessary. Here's how it can be done:
void Trie::insert(const std::string &word) {
TrieNode *node = root;
for (char ch : word) {
int index = ch - 'a'; // Calculate index for 'a' to 'z'
if (!node->children[index]) {
node->children[index] = new TrieNode(); // Create new node if it doesn't exist
}
node = node->children[index];
}
node->isEndOfWord = true; // Mark the end of the word
}
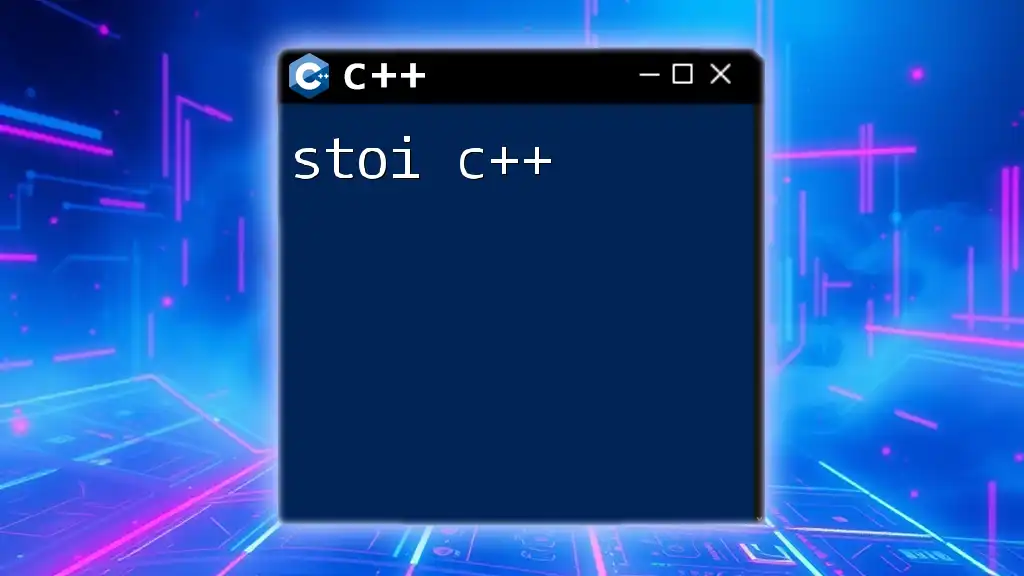
Searching for Words in a Trie
Two types of searches can be performed in a trie: searching for an exact match and checking for a prefix.
Searching for Exact Matches
When searching for a complete word, we must navigate through each character and check if it exists in the trie:
bool Trie::search(const std::string &word) {
TrieNode *node = root;
for (char ch : word) {
int index = ch - 'a';
if (!node->children[index]) {
return false; // Not found
}
node = node->children[index];
}
return node->isEndOfWord; // True if it's an exact word
}
Searching for Prefix Matches
To check if a prefix exists, we can use a similar approach, terminating the search process before reaching the end of the word:
bool Trie::startsWith(const std::string &prefix) {
TrieNode *node = root;
for (char ch : prefix) {
int index = ch - 'a';
if (!node->children[index]) {
return false; // Prefix not found
}
node = node->children[index];
}
return true; // Found prefix
}
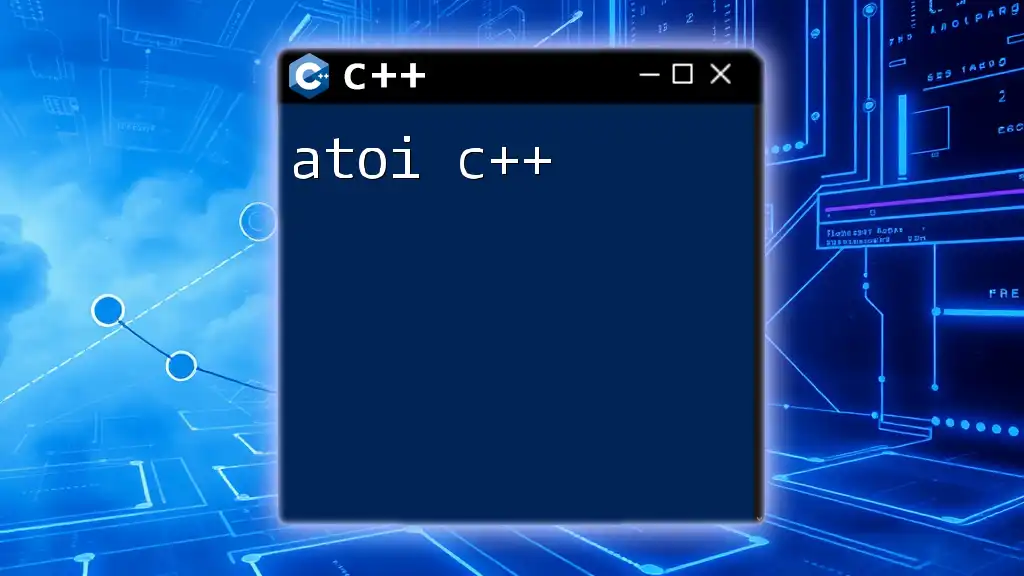
Deleting Words from a Trie
Removing a word from a trie can be tricky because we have to ensure that the structure remains intact and children of nodes are preserved correctly. Using recursion can simplify this process:
bool Trie::remove(TrieNode *node, const std::string &word, int depth) {
if (!node) return false; // Base case: node does not exist
if (depth == word.size()) {
if (node->isEndOfWord) {
node->isEndOfWord = false; // Unmark the end of the word
return node->isLeaf(); // Return true if no children
}
}
return remove(node->children[word[depth]-'a'], word, depth + 1);
}
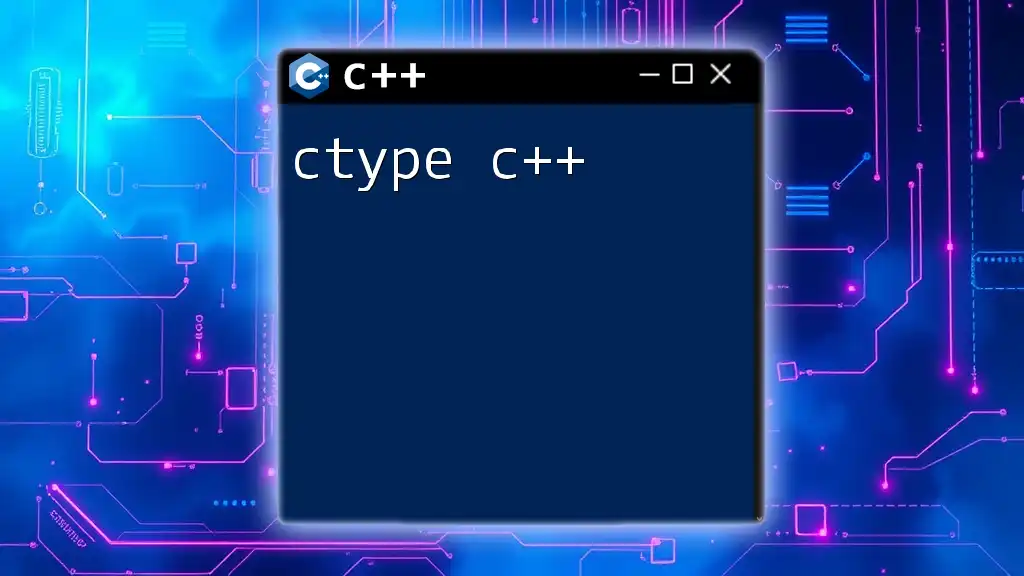
Optimizing Trie Operations
Space Optimization Techniques
To optimize space, you can explore various techniques, such as:
- Using arrays vs. hashmaps for child pointers: Arrays offer faster access but can waste memory, while hashmaps are more space-efficient but slightly slower.
- Implementing a compressed trie (Patricia Trie) can further reduce memory usage by combining common prefixes.
Time Complexity Considerations
The average time complexity for each operation (insert, delete, search) in a trie is O(m), where m is the length of the word. This is generally efficient, especially compared to hash tables in worst-case scenarios. However, it's essential to consider cases where tries may become inefficient, such as storing many long strings that share little prefix similarity.
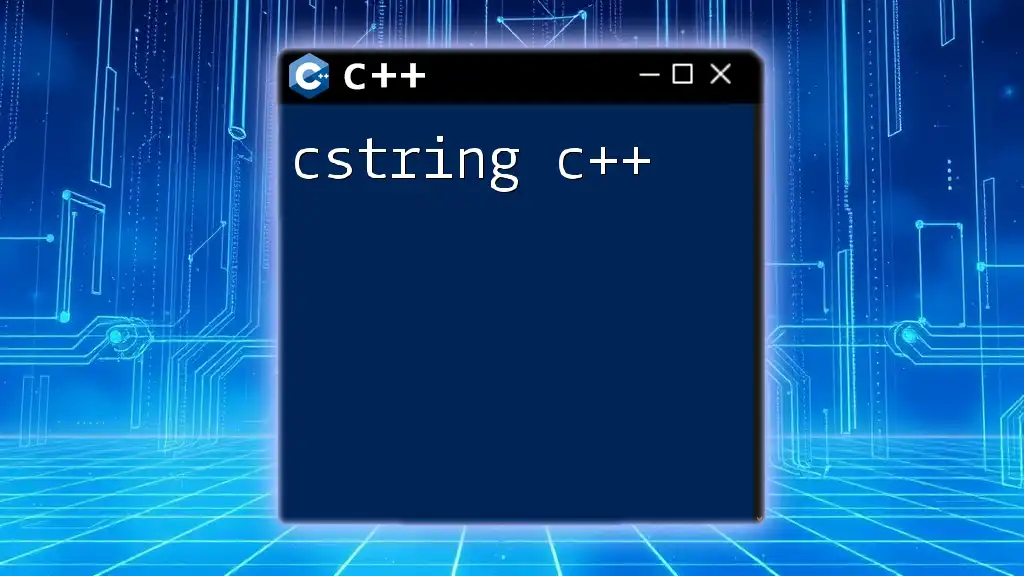
Conclusion
In summary, the trie in C++ is a powerful data structure for managing sets of words with efficient insert, search, and delete operations. Given its specialized nature, tries shine in applications where prefixes are vital, such as auto-completion and spell checking. Exploring further into advanced structures like compressed tries can lead to even greater efficiency. Tries stand out among traditional data structures, particularly for handling string-based data, offering developers a streamlined way to manage and retrieve textual information effectively.
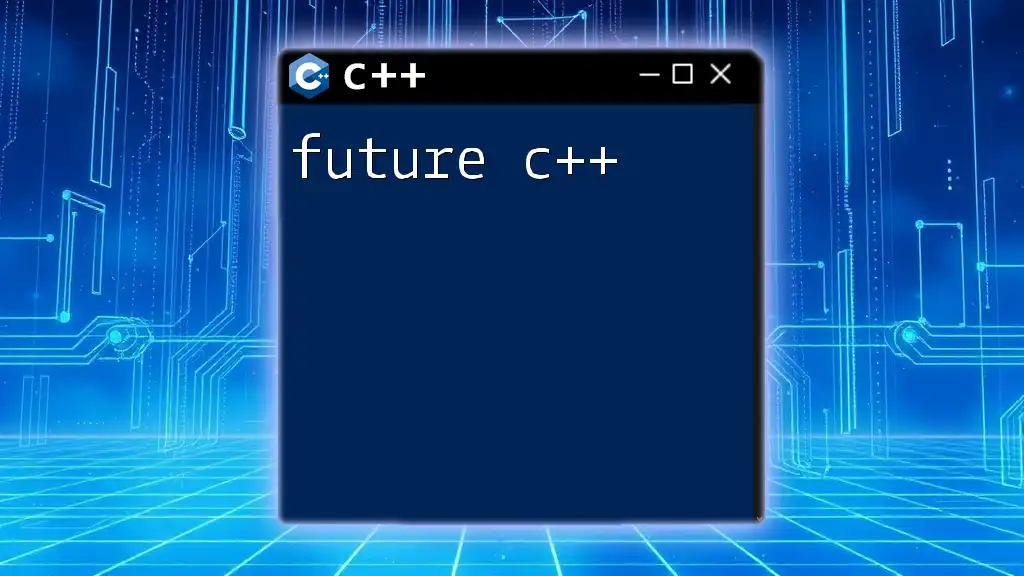
Frequently Asked Questions About Tries
What are the advantages of using a Trie over other data structures?
Tries provide rapid searches and prefix management, making them more suitable for certain applications compared to hash tables or binary trees, especially when dealing with strings.
Can Tries be used for languages other than English?
Yes, tries can be adapted to any language as long as you adjust the character set in the node structure to accommodate language-specific alphabets.
How do Tries compare to Suffix Trees or other advanced data structures?
While tries excel in prefix-based applications, suffix trees (which hold all suffixes of a string) can be more complex and memory-intensive but offer powerful mechanisms for substring search and matching. Depending on the specific use case, each structure has its strengths and weaknesses.