A B-tree in C++ is a self-balancing tree data structure that maintains sorted data and allows for efficient insertions, deletions, and searches, commonly used in databases and filesystems.
Here's a simple code snippet demonstrating the basic structure of a B-tree node and insertion in C++:
#include <iostream>
#include <vector>
using namespace std;
class BTreeNode {
int *keys;
int t;
BTreeNode **C;
int n;
bool leaf;
public:
BTreeNode(int _t, bool _leaf);
void insertNonFull(int k);
void splitChild(int i, BTreeNode *y);
void traverse();
friend class BTree;
};
class BTree {
BTreeNode *root;
int t;
public:
BTree(int _t) { root = nullptr; t = _t; }
void traverse() { if (root != nullptr) root->traverse(); }
void insert(int k);
};
This snippet defines a class structure for a B-tree in C++, including its nodes and a basic insertion function, setting the groundwork for more complex operations.
What is a B Tree?
Definition and Characteristics
A B Tree is a balanced tree data structure that maintains sorted data and allows for efficient insertion, deletion, and searching. The primary features of a B Tree include:
- Multi-way Tree Structure: Unlike binary trees, each node may contain more than two children, making B Trees more efficient in scenarios where the data can be stored in sorted order.
- Balanced Levels: All leaf nodes are at the same depth, which ensures that the tree remains balanced, thus preventing degradation to a linear structure like in a binary search tree.
- Dynamic Size: The number of keys in a node ranges between a predefined minimum and maximum, leading to dynamic growth or shrinkage as keys are inserted or deleted.
Structure of B Trees
B Trees consist of nodes that can have multiple keys and children. Each node has the following characteristics:
- Leaf Nodes: These nodes contain only keys with no children.
- Internal Nodes: These nodes comprise both keys and child pointers.
- Order 't' of the B Tree: This defines the bounds for the number of keys a node can hold:
- Each internal node can contain at most \(2t - 1\) keys and at least \(t - 1\) keys.
- Nodes can have between \(t\) and \(2t\) children.
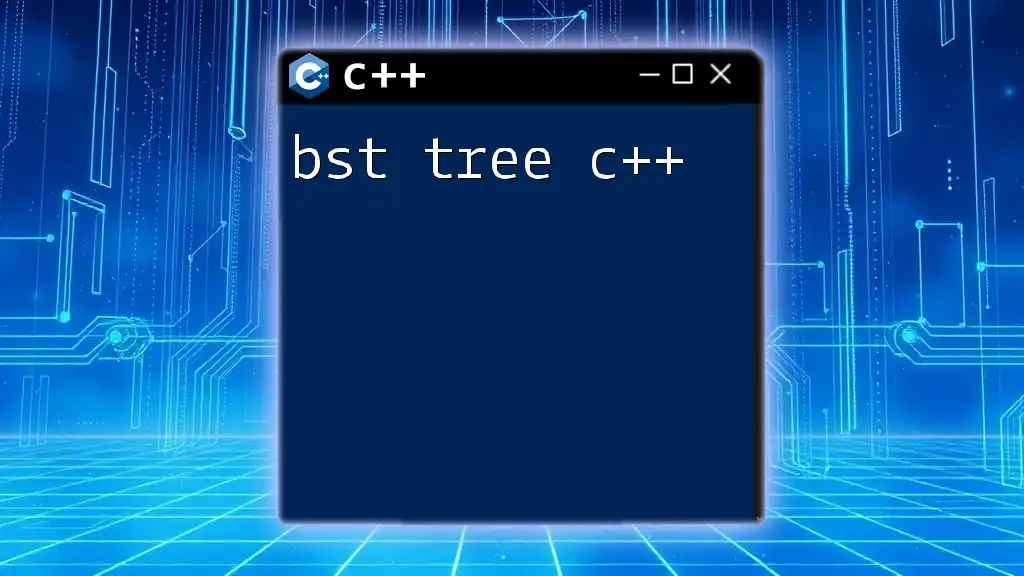
Why Use B Trees in C++?
Advantages of B Trees
B Trees are particularly advantageous in databases and filesystems, primarily due to their efficiency:
- Efficient Searching, Insertion, and Deletion: Operations typically take logarithmic time due to the balanced nature of the tree.
- Reduced Disk Access: Since B Trees are designed to minimize disk accesses, they are well-suited for systems that make frequent queries to disk storage, such as databases.
Comparison with Other Data Structures
Examining B Trees in contrast to other data structures emphasizes their unique strengths:
- B Trees vs Binary Search Trees: B Trees can maintain balance better than binary search trees, which may become unbalanced with successive insertions and deletions.
- B Trees vs Other Tree Structures: Other data structures like AVL trees also maintain balance but may not be optimized for systems where disk I/O is a performance bottleneck.
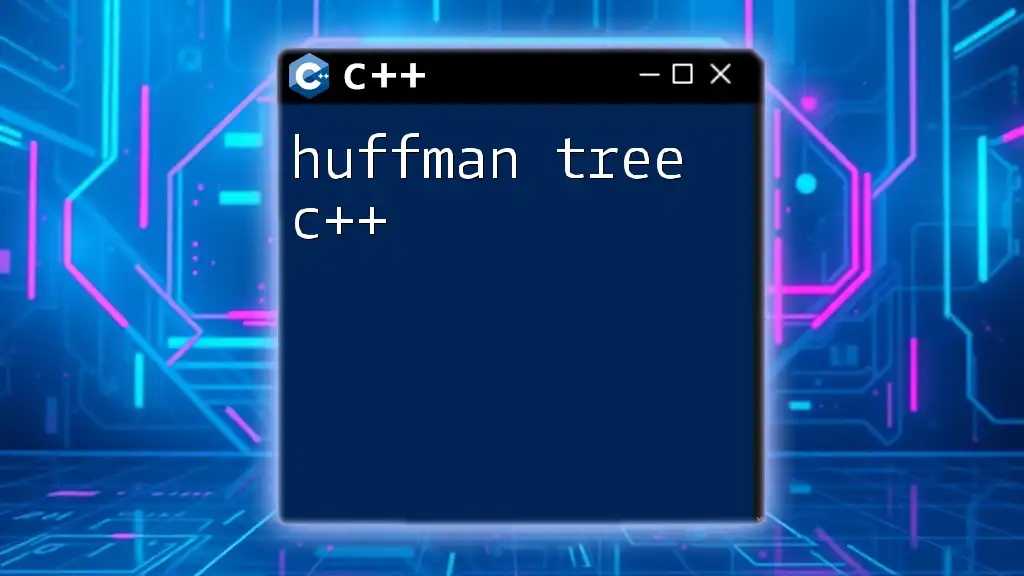
Implementing a B Tree in C++
Basic B Tree Node Structure
To start, we need to define the basic structure of a B Tree node. Here's how it looks in C++:
struct BTreeNode {
int *keys; // Array of keys
int t; // Minimum degree (defines the range for number of keys)
BTreeNode **C; // Array of child pointers
int n; // Current number of keys
bool leaf; // True if leaf node
};
This structure consists of:
- An array for keys.
- A minimum degree that governs the properties of the B Tree.
- An array for child pointers.
- The current number of keys held in the node.
- A boolean indicating if the node is a leaf.
Building the B Tree
Creating a New B Tree
To create a new B Tree, we can write a constructor that initializes it:
class BTree {
public:
BTreeNode *root;
int t; // Minimum degree
BTree(int _t) {
root = nullptr;
t = _t;
}
};
Inserting Keys into the B Tree
The heart of a B Tree lies in its insert function. Insertions can be handled through a method called `insertNonFull` which manages cases where the node is not full:
void insertNonFull(BTreeNode *node, int key);
// Node split management
void splitChild(BTreeNode *parent, int i, BTreeNode *child);
If a node is full during insertion, it will be split, and the median key will be pushed up to the parent node.
Searching in a B Tree
Searching keys in a B Tree involves traversing the tree from the root downwards. The search function can be represented as:
BTreeNode *search(BTreeNode *node, int key);
This function examines a node's keys sequentially, finding the appropriate child node or key. If a match is found, the function returns the node containing the key; otherwise, it proceeds to the appropriate child.
Deleting Keys from a B Tree
Deletion Mechanics
Deleting keys from a B Tree can be complex due to the need to maintain balance and order. The core concept involves handling three cases:
- Leaf Deletion: If the key is found in a leaf node, simply remove it.
- Internal Deletion: If the key is in an internal node, replace it with its predecessor or successor, then delete that key.
- Rebalancing: If the node becomes underflowed (holds fewer than \(t-1\) keys), it can borrow from a sibling or merge with it.
void deleteKey(int key);
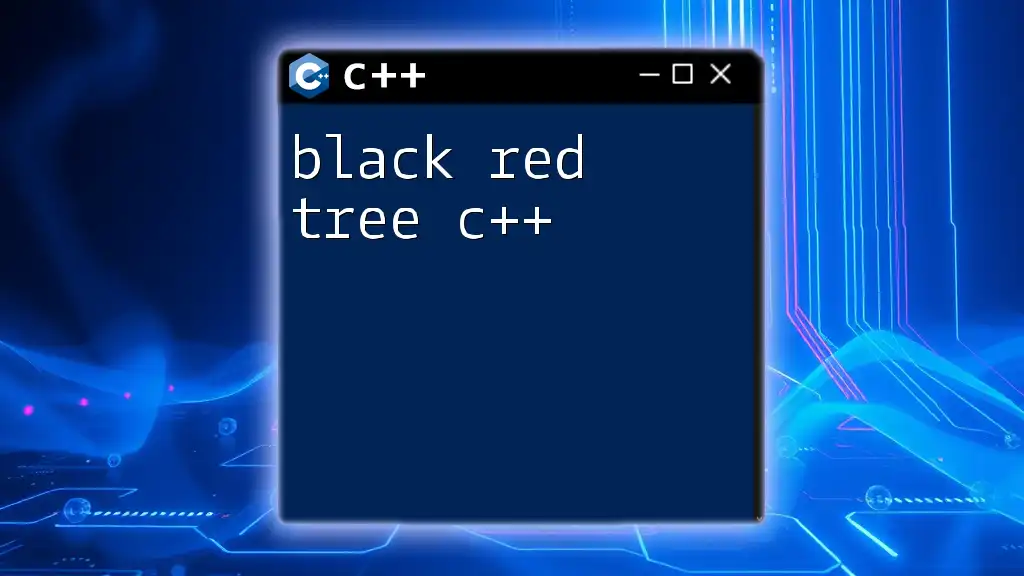
Traversing the B Tree
In-order Traversal
Traversal of a B Tree can be effectively done using in-order traversal to display keys in sorted order.
void traverse();
This method visits all nodes while ensuring keys are printed in order and may utilize recursion to handle multiple levels.
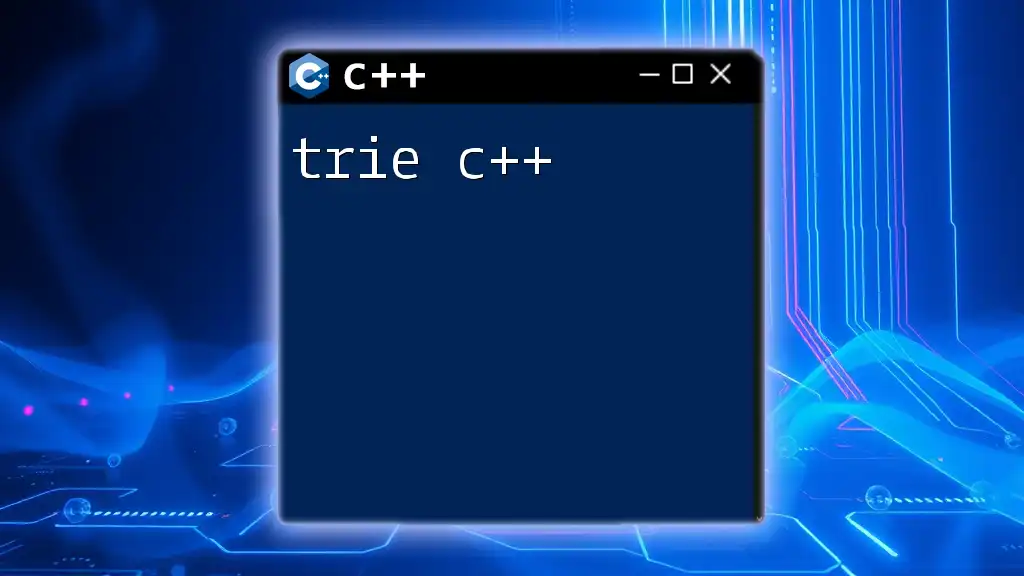
Practical Use Cases of B Trees
B Trees are widely used in scenarios where efficient data management is critical:
- Database Indexing: B Trees enable quick retrieval of records in databases, leading to faster query responses by minimizing the number of disk accesses.
- File Systems: In file systems, B Trees help organize directories and file metadata, allowing for efficient access and management of files.
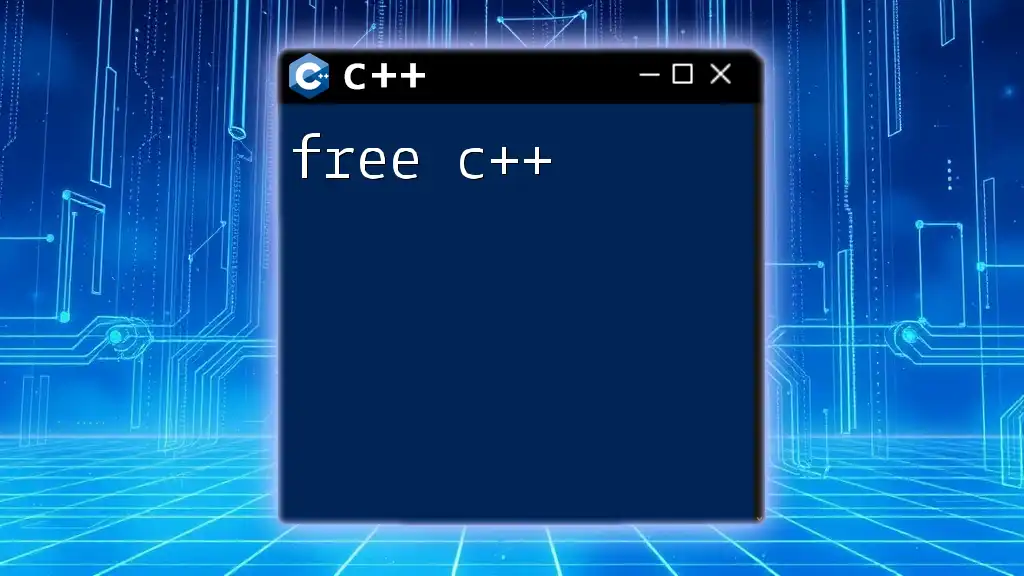
Common Challenges and Solutions in B Trees
Rebalancing the Tree
Maintaining balance in a B Tree is crucial, especially during deletions. If a node has fewer than the required number of keys after a deletion, strategies for rebalancing must be employed, such as merging nodes or transferring keys from neighboring siblings.
Debugging Tips
Common issues when implementing B Trees include improper handling during splits or forgetting to update parent pointers. Thorough testing and consistent checks for conditions upon insertion and deletion can mitigate these challenges.
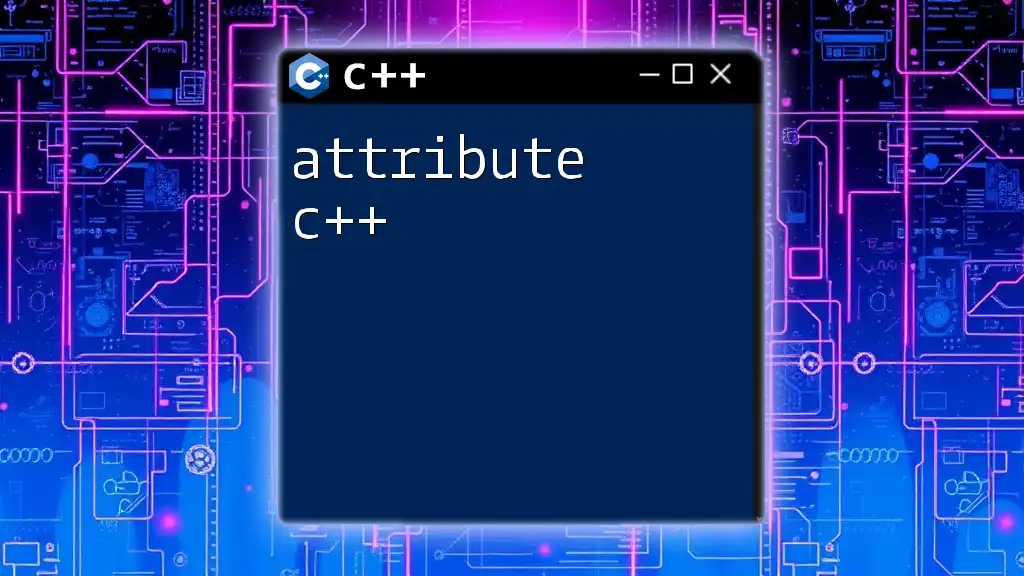
Conclusion
In summary, B Trees represent a powerful and flexible data structure for managing sorted data. Their balanced nature offers significant performance improvements in searching, insertion, and deletion operations, making them a preferred choice in various applications such as databases and file systems.
For those keen on mastering B Trees and further exploring data structures in C++, numerous resources are available, including books, online courses, and active programming communities. Engaging with such resources will deepen your understanding and proficiency in implementing and leveraging B Trees effectively in your projects.