"Free C++" refers to the use and access to the C++ programming language and its resources without cost, allowing learners to harness its capabilities for development and programming projects.
Here's a simple example of a C++ code snippet that demonstrates how to declare and use a variable:
#include <iostream>
int main() {
int number = 5; // Declare and initialize an integer variable
std::cout << "The number is: " << number << std::endl; // Output the variable
return 0;
}
What is Free in C++?
In the context of C++, the term free primarily relates to how memory is managed during a program’s execution. Proper understanding and handling of memory allocation and deallocation are crucial for creating efficient programs without memory leaks and crashes.
Dynamic memory allocation allows programmers to request memory at runtime. It is essential to distinguish between dynamic and static memory, as the inappropriate use of memory can lead to program inefficiencies and bugs.
Memory Management in C++
C++ offers powerful mechanisms for memory management, which can be divided into two main types: dynamic memory and static memory.
Dynamic memory is allocated during runtime using operators like `new` and functions like `malloc`. This provides greater flexibility and efficient resource utilization, particularly for programs that handle varying amounts of data.
Static memory, on the other hand, is allocated at compile time and lasts for the duration of the program execution. While static memory allocation is simpler and generally less error-prone, it's essential to use dynamic memory when the size of the data cannot be determined until runtime.
Introduction to `malloc` and `free`
To manage dynamic memory in C++, the standard library provides functions such as `malloc` and `free`.
-
`malloc` is used for allocating a specific amount of raw memory and returns a pointer to the allocated memory block. An example of using `malloc` is:
int *arr = (int*) malloc(5 * sizeof(int));
-
It's essential to note that `malloc` does not call constructors for class objects. Therefore, ensure you are aware of the implications if you're working with class types.
After memory allocation, you must use `free` to release it. Failing to do so results in memory leaks, which can consume an application’s available memory significantly over time. Here is how you can use `free`:
free(arr);
Using `free` liberates the allocated memory, allowing the program to reuse this memory for future allocation requests.
Understanding `new` and `delete`
In C++, the `new` operator provides a more robust way to allocate memory, tailored specifically for C++ objects. Unlike `malloc`, `new` initializes memory and calls the constructor for class objects, providing a seamless experience for managing C++ objects.
Here’s a sample of using `new`:
int *arr = new int[5];
Similarly, to deallocate memory allocated by `new`, you use the `delete` operator. For array allocation, make sure to use `delete[]` to properly clean up all allocated memory:
delete[] arr;
As a general practice, for every `new`, there should be a corresponding `delete`, helping to maintain efficient memory usage and prevent memory leaks.
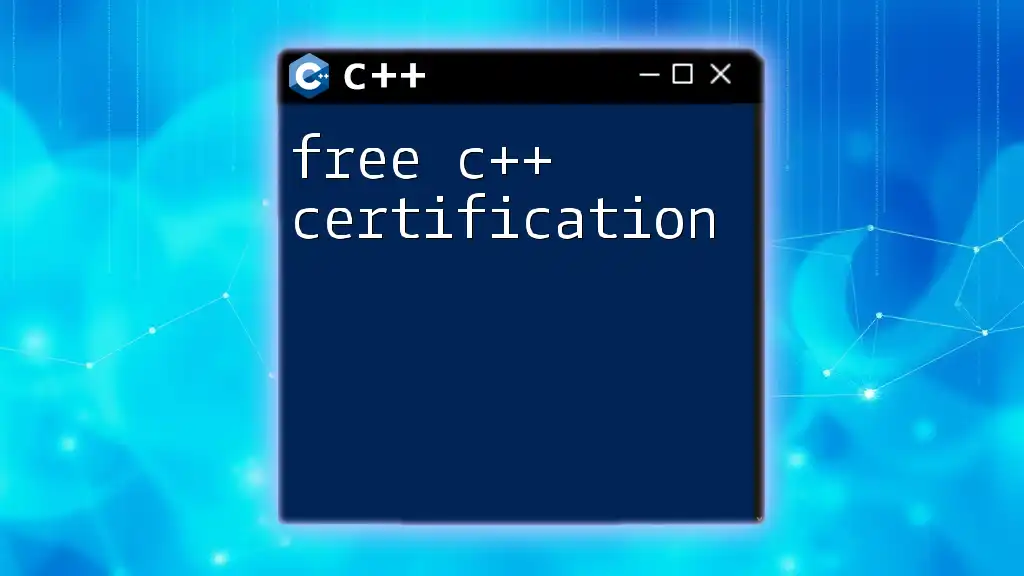
Strategies for Efficient Memory Management
Smart Pointers
C++11 introduced smart pointers, which automate memory management and prevent resource leaks. The three common types are:
-
`unique_ptr`: Represents sole ownership of an object. When it goes out of scope, the associated memory is automatically freed.
std::unique_ptr<int> ptr(new int(10));
-
`shared_ptr`: Allows multiple pointers to share ownership of an object. The memory will be freed only when the last owner exits its scope.
std::shared_ptr<int> sptr(new int(20));
-
`weak_ptr`: Does not affect the reference count of the object managed by `shared_ptr`. It is primarily used to break circular references that could lead to memory leaks.
Using smart pointers simplifies memory management and minimizes the risk of forgetting to release memory.
RAII (Resource Acquisition Is Initialization)
RAII is a programming idiom central to resource management in C++. The principle ensures that resources are tied to object lifetimes. When an object goes out of scope, its destructor releases any allocated resources.
Consider the following class example:
class Resource {
public:
Resource() { /* acquire resource */ }
~Resource() { /* release resource */ }
};
In this case, as soon as a `Resource` object goes out of scope, its destructor is automatically called, releasing the resource without additional effort from the programmer.
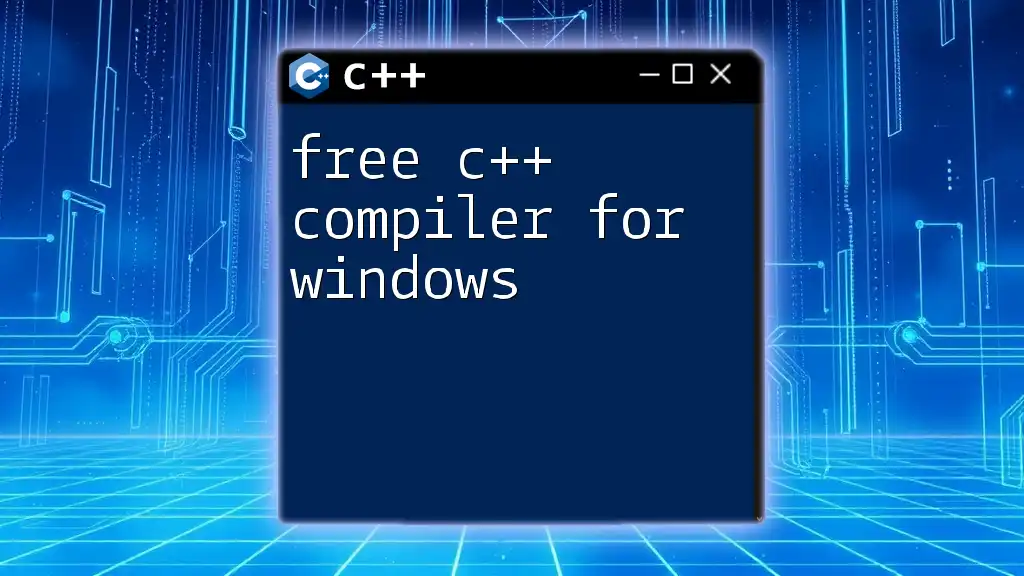
Free Resources to Learn C++
To delve deeper into free C++ knowledge, consider the following resources:
Online Tutorials and Courses
There are numerous platforms offering comprehensive and free C++ tutorials:
- Codecademy: Interactive and beginner-friendly lessons.
- Coursera: Free courses from universities covering various C++ aspects.
- edX: Offers courses from reputed institutions focusing on memory management and efficient programming practices.
Open-source C++ Libraries
Leveraging open-source libraries can enhance your learning and development experience in C++. Some notable libraries include:
- Boost: Provides free libraries that extend the functionality of C++, including smart pointers and memory management tools.
- Qt: A robust framework with free modules for building cross-platform applications that emphasize effective resource handling.
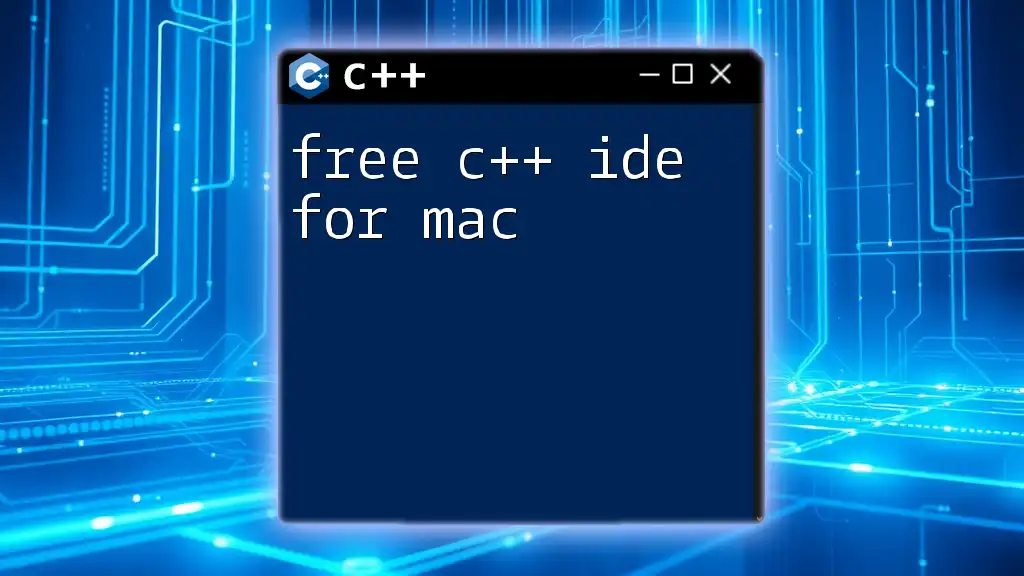
Conclusion
Understanding memory management is crucial for writing efficient and reliable C++ applications. Grasping concepts like dynamic memory, the difference between `malloc` and `new`, and employing smart pointers can aid in program stability and performance. Follow the strategies outlined, and don’t hesitate to explore the plethora of resources available for enhancing your C++ knowledge.
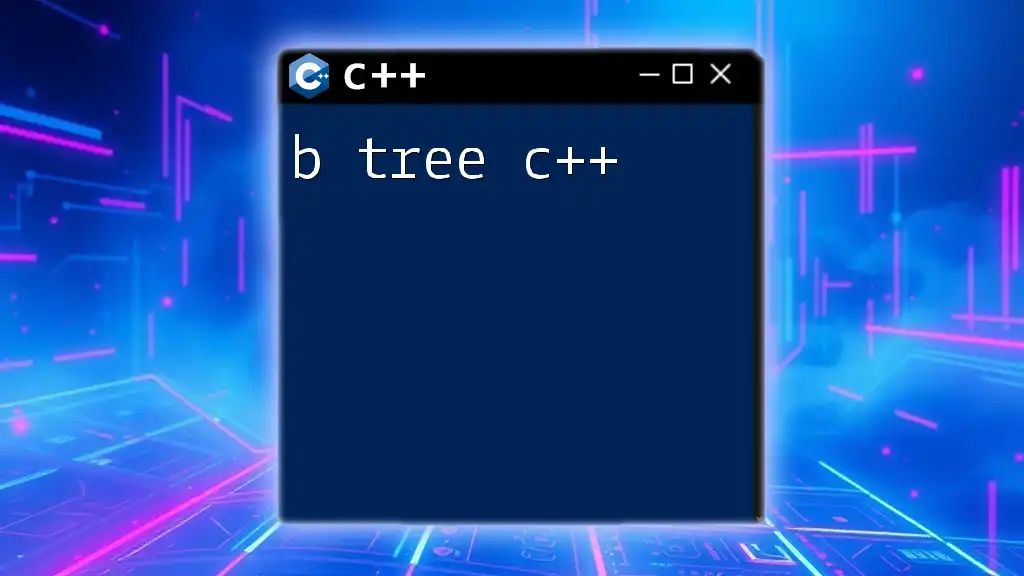
Frequently Asked Questions (FAQs)
-
What does free memory mean in C++?
It refers to the deallocation of previously allocated memory, which is essential to prevent memory leaks. -
How do I prevent memory leaks in C++?
Use smart pointers and ensure every call to `new` or `malloc` is matched with a corresponding `delete` or `free`. -
Can I use `malloc` with C++ objects?
Yes, but it does not call constructors. Prefer `new` for C++ objects to correctly initialize them.