Boost your programming skills and enhance your resume with a free C++ certification that provides a comprehensive understanding of essential C++ commands and concepts.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Certification
What is C++ Certification?
C++ certification is a formal acknowledgment of an individual's knowledge and skills related to the C++ programming language. Unlike traditional academic degrees that focus on a broad curriculum, certification programs are designed to demonstrate specific competencies in C++. They are often regarded as reliable indicators of a candidate's capacity to handle programming tasks, making them particularly valuable in tech jobs.
Benefits of Being C++ Certified
Obtaining a C++ certification can significantly enhance your job prospects. Aside from improving your employment opportunities, certification acts as a validation of your skills to employers looking for qualified candidates. It can set you apart in a competitive job market.
Moreover, many certification programs encourage continual learning, keeping your skills sharp and up-to-date. This ongoing education is essential in the ever-evolving tech landscape.
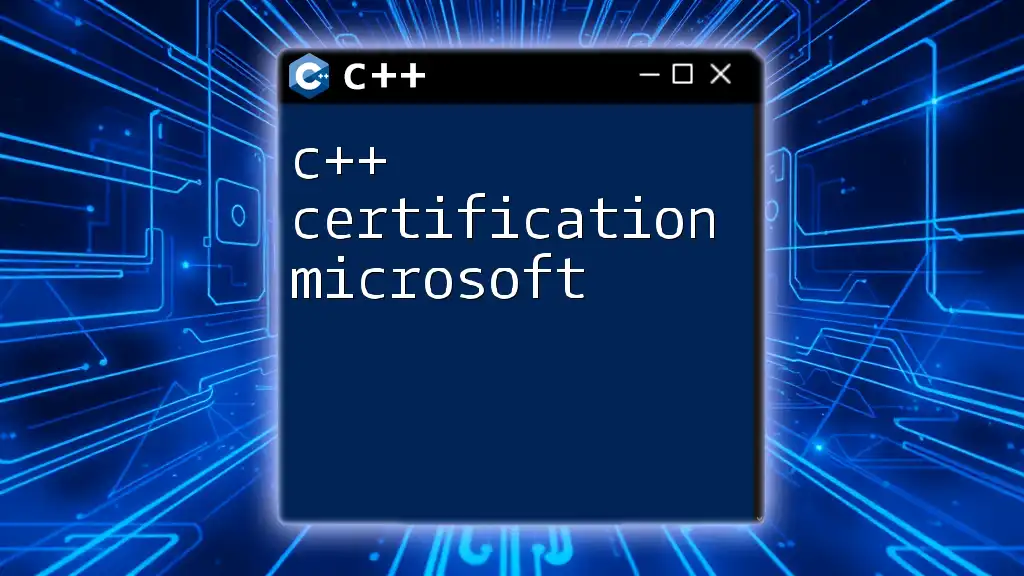
Where to Find Free C++ Certification
Online Learning Platforms
Coursera
Coursera partners with universities and organizations to offer various C++ courses. Some of these courses allow you to earn a certificate for free if you complete the program within a certain timeframe. Look for courses like "C++ for C Programmers" to advance your skills and earn a recognized certification.
edX
edX also provides C++ courses from reputable institutions such as Microsoft and Harvard. Many of these courses can be audited at no cost, opening up opportunities to gain knowledge and even obtain a certification for free. Check certificates like "Introduction to C++" offered by Columbia University, which provides valuable education irrespective of the payment option you choose.
Non-profit Organizations
FreeCodeCamp
FreeCodeCamp is a fantastic resource for those wanting to learn programming at no cost. While they primarily focus on web development, their curriculum incorporates C++ basics. Students can engage with the community and earn certification for different skill levels, including foundational data structures and algorithms, which are critical in C++.
Codecademy
Codecademy offers an interactive C++ track, allowing you to practice coding directly in your browser. While the "Pro" features require payment, you can still gain a robust understanding of C++ and earn a completion certificate through the free introductory courses, preparing you for more advanced studies.
MOOCs (Massive Open Online Courses)
Massive Open Online Courses are tailored to a global audience seeking to learn in-demand skills. For C++, platforms like Udemy and Skillshare often host relevant courses. While many are paid, you can often find free options or substantial discounts. Enroll in a course and complete it to receive a free certification. MOOCs usually encompass various topics, enabling you to learn C++ in a structured manner.
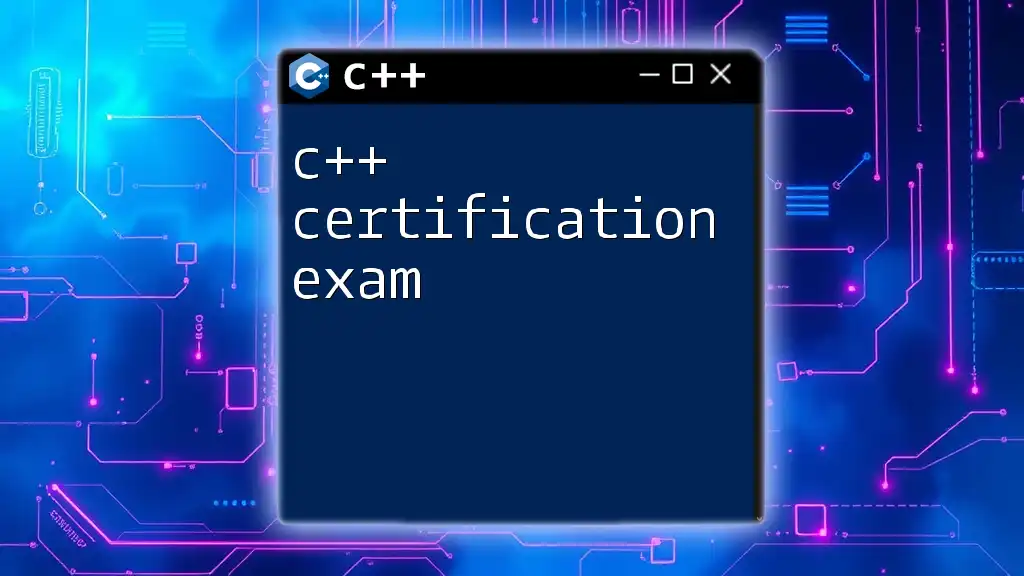
Preparing for C++ Certification Exams
Essential C++ Concepts to Master
Basic Syntax and Structure
Understanding the basic syntax and structure of C++ is foundational. It includes knowing how to form a valid code block, use semicolons, and create functions. Here’s a basic C++ program to illustrate the syntax:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple code prints "Hello, World!" to the console and forms the basis of your first C++ program.
Data Types and Variables
Familiarity with C++ data types is crucial. C++ supports several data types, including `int`, `float`, `double`, and `char`. Here’s how to declare and initialize variables:
int age = 30;
float salary = 2500.50;
char grade = 'A';
Grasping variables sets the stage for more complex programming tasks and is a fundamental component of the certification exams.
Control Structures
Control structures govern the flow of your programs. You need to master loops (like `for`, `while`) and conditional statements (`if`, `else`). Below is an example that illustrates both:
for (int i = 0; i < 5; i++) {
if (i % 2 == 0) {
cout << i << " is even." << endl;
} else {
cout << i << " is odd." << endl;
}
}
Object-Oriented Programming
Classes and Objects
Object-Oriented Programming (OOP) is a core concept in C++. It allows you to create classes that model real-world objects. Here’s a simple class example:
class Car {
public:
string brand;
string model;
int year;
void display() {
cout << brand << " " << model << ", " << year << endl;
}
};
You can create an object of the class and call its methods to understand instance variables better.
Car myCar;
myCar.brand = "Toyota";
myCar.model = "Camry";
myCar.year = 2021;
myCar.display();
Inheritance and Polymorphism
Inheritance allows new classes to inherit properties of existing classes. This maintains a hierarchical relationship, fostering code reuse. Polymorphism enables methods to do different things based on the object it is acting upon, enhancing flexibility in code.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful part of C++ that provides common data structures and algorithms. This includes vectors, lists, and maps. An example of using a vector is shown below:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << " ";
}
return 0;
}
Understanding STL is vital for efficiency and effectiveness in your programming practice.
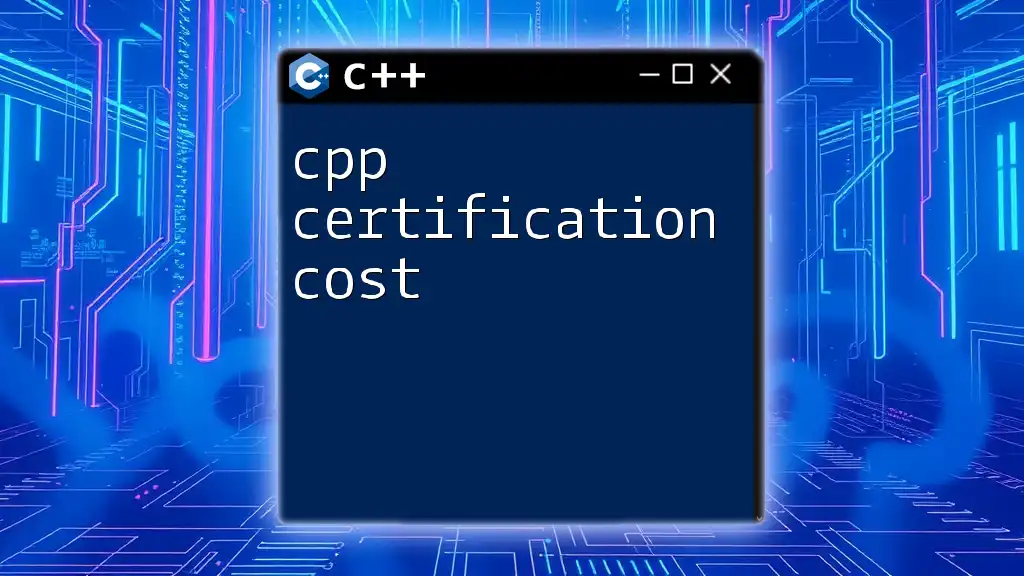
Study Materials and Resources
Books and E-books
Books are an excellent way to deepen your knowledge of C++. Look for classics like "The C++ Programming Language" by Bjarne Stroustrup, which is comprehensive and highly regarded in the programming community. Moreover, free e-books can often be found on educational websites and open-source platforms.
Online Coding Platforms
Utilizing online coding platforms such as LeetCode and HackerRank is essential for hands-on practice. These platforms not only challenge your problem-solving skills but also provide a varied assortment of C++ problems that reflect typical exam questions.
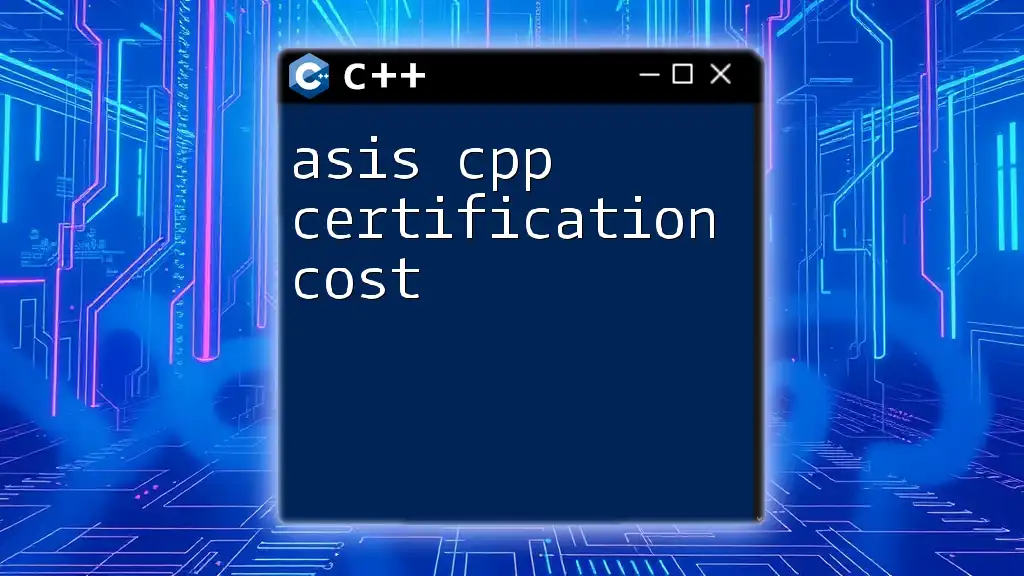
Tips for Success in C++ Certification Exams
Take Practice Tests
Practice tests are invaluable in your preparation. They help you become familiar with the exam format while highlighting areas that need improvement. Websites like ExamTopics provide free practice questions that mirror those you'd find in actual exams.
Join Online Communities
Being part of online programming communities can significantly enhance your learning curve. Engaging with peers can provide support and motivation. Platforms like Stack Overflow and Reddit's C++ subreddit are excellent places to ask questions, share knowledge, and connect with fellow learners.
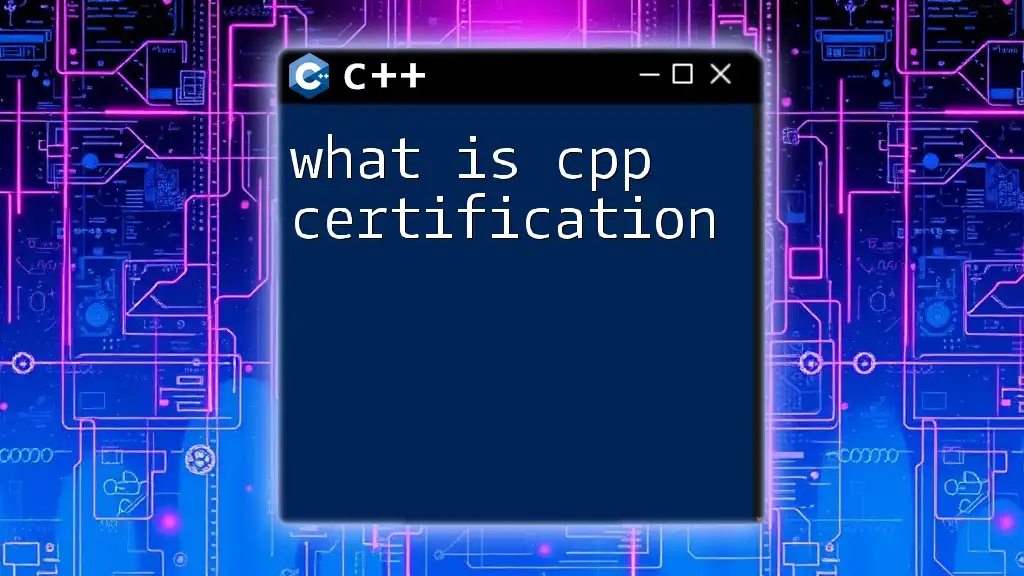
What to Do After You Get Certified
Update Your Resume
Once you earn your C++ certification, your next step is to update your resume. Highlight your certification prominently, focusing on how it validates your skills. Employers often look for computational problem-solving abilities and mastery of programming principles—ensure these elements are clear in your summary.
Networking Opportunities
Use platforms like LinkedIn to connect with other certified professionals. Networking is invaluable; it can open doors to job opportunities, collaborations, and insights into industry trends.
Continuous Learning
The tech landscape is dynamic and requires ongoing education. Staying current with new C++ standards, libraries, and frameworks is crucial. Engaging in advanced certification programs or specialized courses will help you remain competitive.
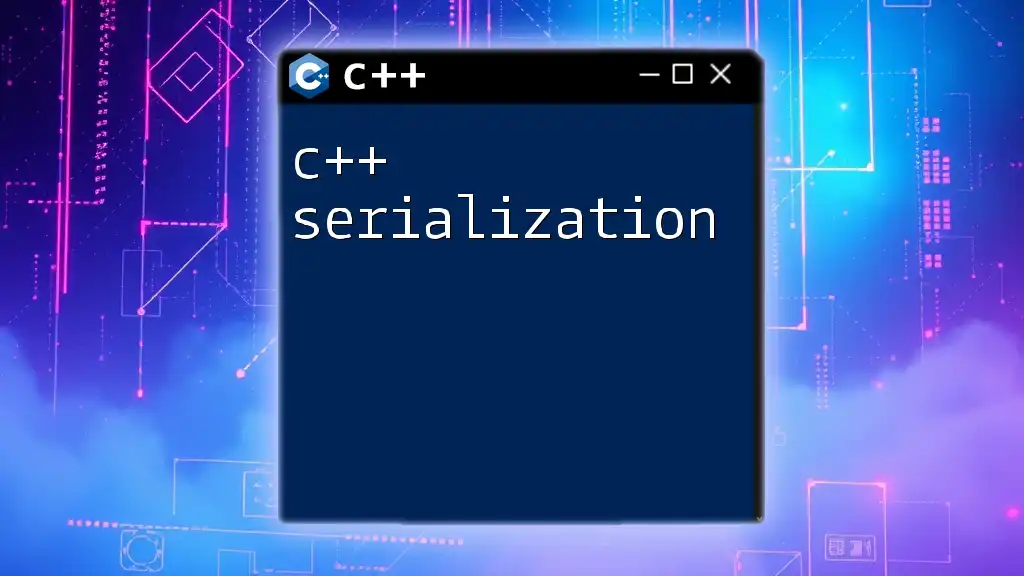
Conclusion
As demonstrated, obtaining free C++ certification could be a game changer in your programming career. With a range of resources available—platforms, books, communities—and a structured approach to preparation, you are well-equipped to succeed. Embrace this opportunity to validate your skills and take your programming journey to the next level.
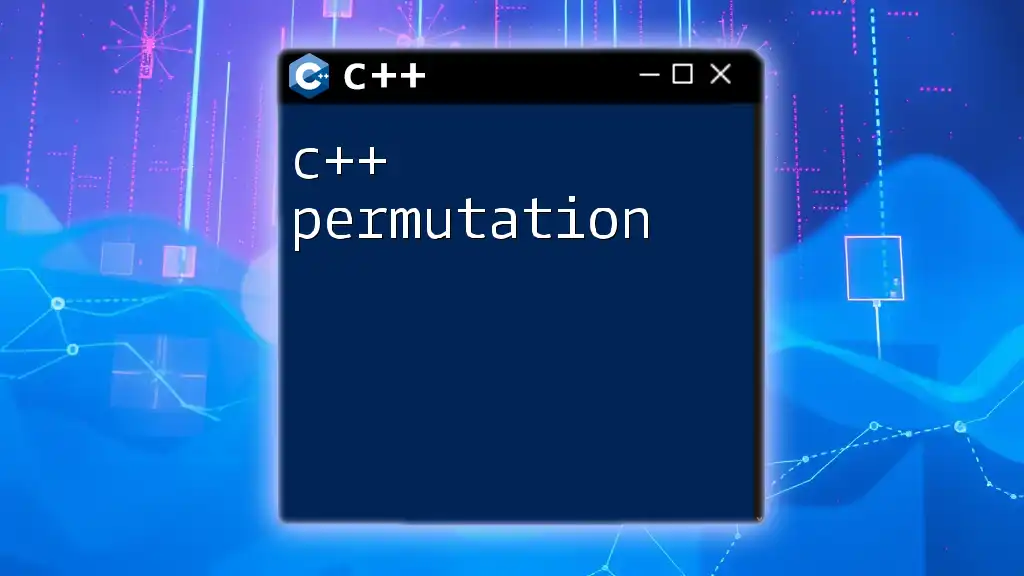
Call-to-Action
Explore the resources mentioned in this article, and don't hesitate to share your own experiences with C++ certification in the comments below. Your journey can inspire others to take their first steps toward mastery in C++.