The C++ certification exam tests candidates' proficiency in C++ programming through practical coding challenges and theoretical questions to ensure they have a solid understanding of the language's core concepts.
Here's a simple code snippet demonstrating a basic C++ program that outputs "Hello, World!":
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Understanding C++ Certification
What is C++ Certification?
C++ certification serves as a formal recognition of a programmer's ability to use the C++ programming language effectively. This certification is especially valuable in a competitive job market where employers seek verified skills. By completing a C++ certification, candidates demonstrate their commitment to mastering the language and their proficiency in applying its concepts in various scenarios.
Types of C and C++ Certifications
When it comes to C and C++ certifications, they can be categorized primarily based on their focus:
-
ISO C++ Certification: A widely recognized standard that verifies an individual's understanding and application of C++ according to international standards.
-
C++ Institute Certifications: These range from entry-level to advanced certifications, including:
- CLA (C++ Certified Associate Programmer): A foundational certification focusing on basic principles of C++.
- CLP (C++ Certified Professional Programmer): An advanced certification that dives into deeper and more complex C++ concepts.
Understanding the distinctions between these certifications can help individuals choose the right path based on their experience and career ambitions.
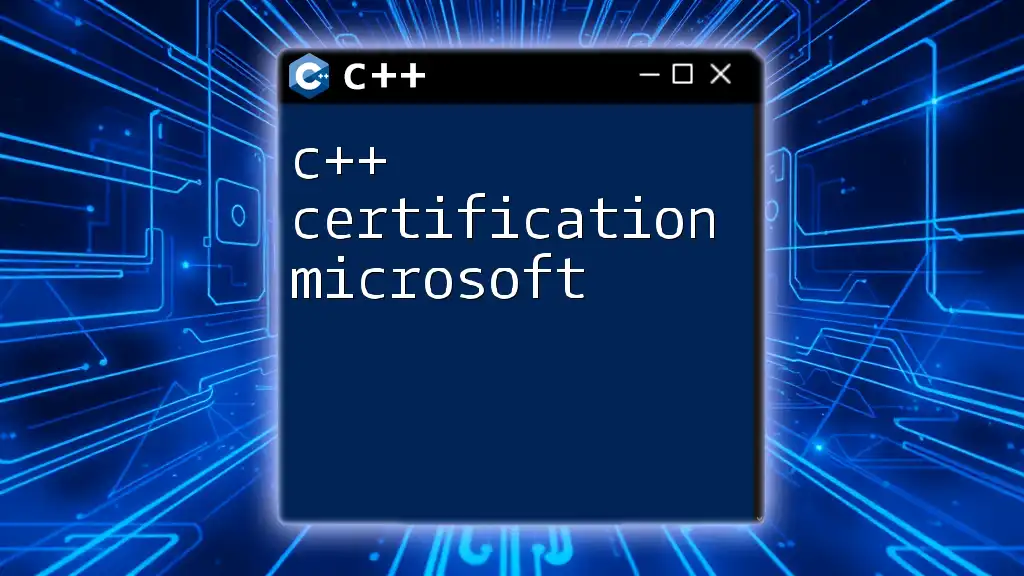
C++ Certification Exam Overview
Who Should Take the C++ Certification Exam?
The C++ certification exam is ideal for a diverse range of individuals, from newcomers to seasoned developers. If you're just starting, certification can provide a structured learning path and a tangible representation of your skills. For experienced programmers, obtaining a certification signifies a commitment to excellence and can open doors to advanced roles and responsibilities.
Benefits of Getting Certified in C++
Getting certified in C++ brings numerous advantages, such as:
-
Recognition of skills and knowledge: Certification clearly shows employers that you possess specific, validated competencies, making you a more attractive candidate.
-
Enhanced job prospects and earning potential: Research shows that certified professionals often earn a higher salary and have better job placement opportunities due to their recognized qualifications.
-
Networking opportunities: Many certification programs offer access to exclusive forums and communities, allowing participants to interact with peers and industry professionals, which can lead to collaborative projects and job opportunities.
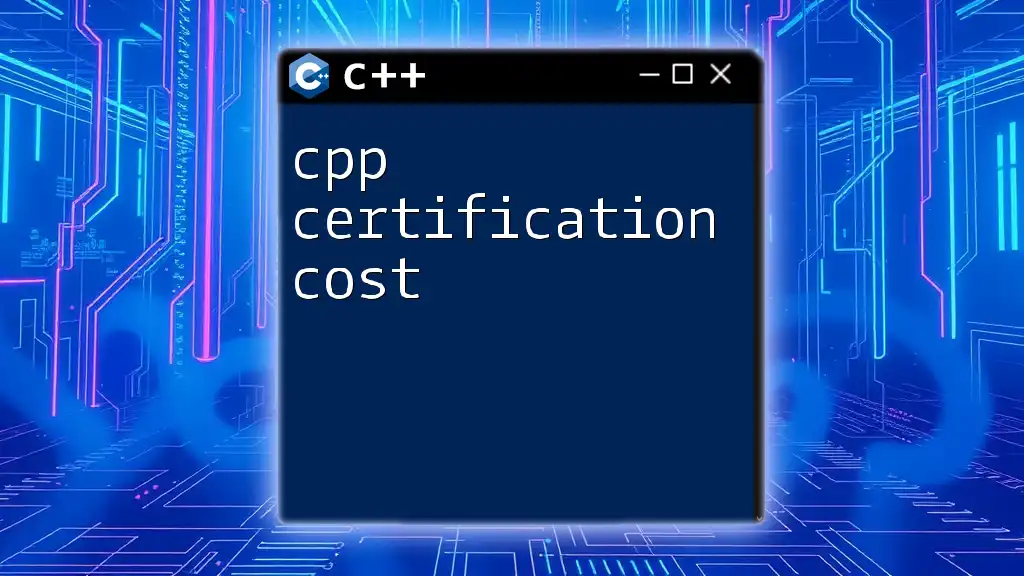
Preparing for the C++ Certification Exam
Prerequisites and Recommended Knowledge
Before taking the C++ certification exam, ensure you have a solid understanding of foundational concepts. Key areas to review include:
-
Object-Oriented Programming (OOP): Master the principles of encapsulation, inheritance, and polymorphism.
-
Data Structures and Algorithms: Familiarize yourself with standard libraries and data manipulation techniques, which form the backbone of effective C++ programming.
A good grasp of these concepts not only boosts your confidence but also equips you for the practical challenges you will face during the exam.
Study Resources for C++ Certification
To prepare for the C++ certification exam effectively, consider utilizing various study materials:
-
Textbooks and Online Courses: Books such as The C++ Programming Language by Bjarne Stroustrup can provide in-depth knowledge. Online platforms like Coursera, Udemy, and Codecademy also offer specialized C++ courses.
-
C++ Documentation and References: The official C++ documentation is an invaluable resource for understanding standard libraries and syntax.
-
Practice Exams and Coding Challenges: Websites like LeetCode, HackerRank, and Coderbyte provide a wealth of coding problems that simulate exam conditions and help reinforce your learning.
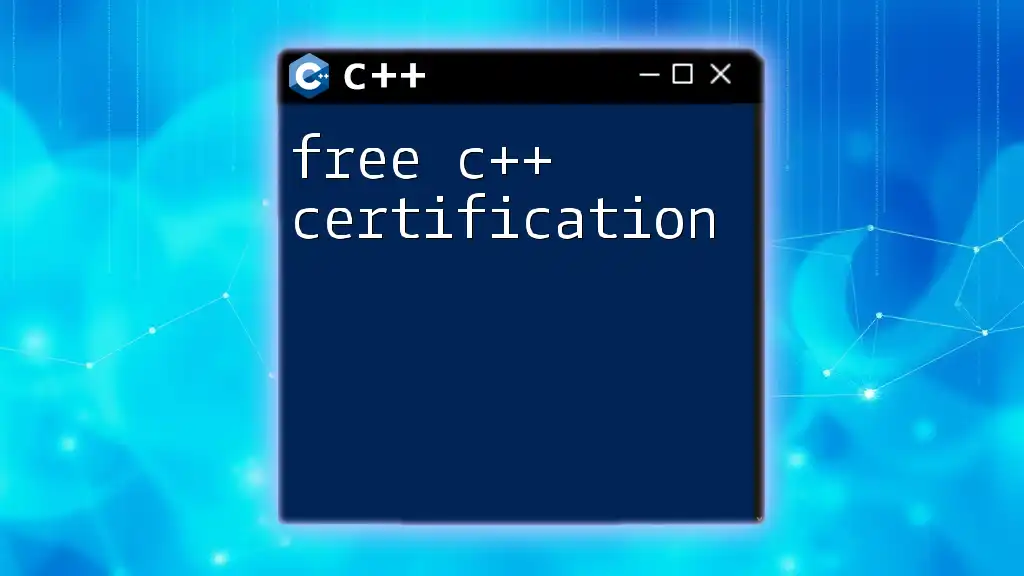
C++ Certification Exam Structure
Format of the Exam
The format of the C++ certification exam typically includes a mix of question types designed to test both theoretical knowledge and practical skills. Expect to encounter:
-
Multiple-choice questions: These often assess foundational concepts and syntax rules.
-
Coding tasks: You'll be required to write efficient and correct code snippets that solve specific problems.
The duration of the exam varies depending on the certification body, so it's essential to manage your time effectively during the test.
Sample Exam Questions
To provide a clearer picture, consider these example questions:
-
Syntax and Semantics Question: What is the output of the following code snippet?
#include <iostream> int main() { int a = 5; int b = 10; std::cout << "Sum: " << a + b << std::endl; return 0; }
Answer: The output will be "Sum: 15".
-
Coding Challenge: Write a function that returns the factorial of a number using recursion.
int factorial(int n) { if (n <= 1) return 1; return n * factorial(n - 1); }
These examples emphasize the need for both theoretical knowledge and coding skills.
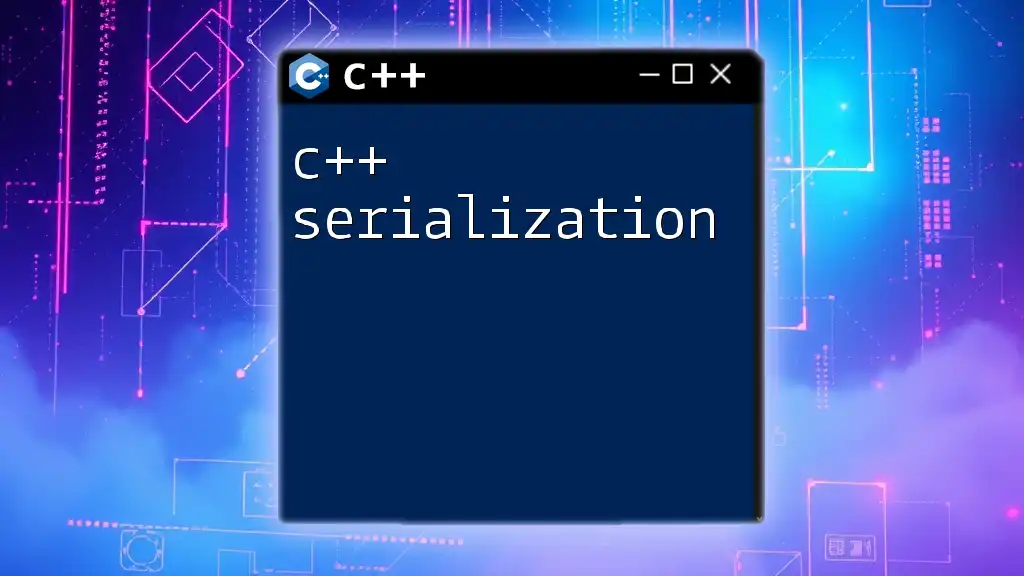
C++ Certification Cost
Understanding the Cost of C++ Certifications
Certification costs can differ significantly based on the specific certification path chosen. Generally, you might encounter:
-
Exam fees: These range from $150 to $500, depending on the certification level.
-
Additional expenses: Consider costs for preparation materials, online courses, and potential retake fees if necessary.
Financial Assistance and Scholarships
Several organizations offer financial assistance or scholarships for prospective candidates:
-
Government assistance programs: Check for local or national initiatives aimed at supporting technical education.
-
Scholarships from institutions: Many educational institutions and coding bootcamps provide scholarships to help cover certification exams.
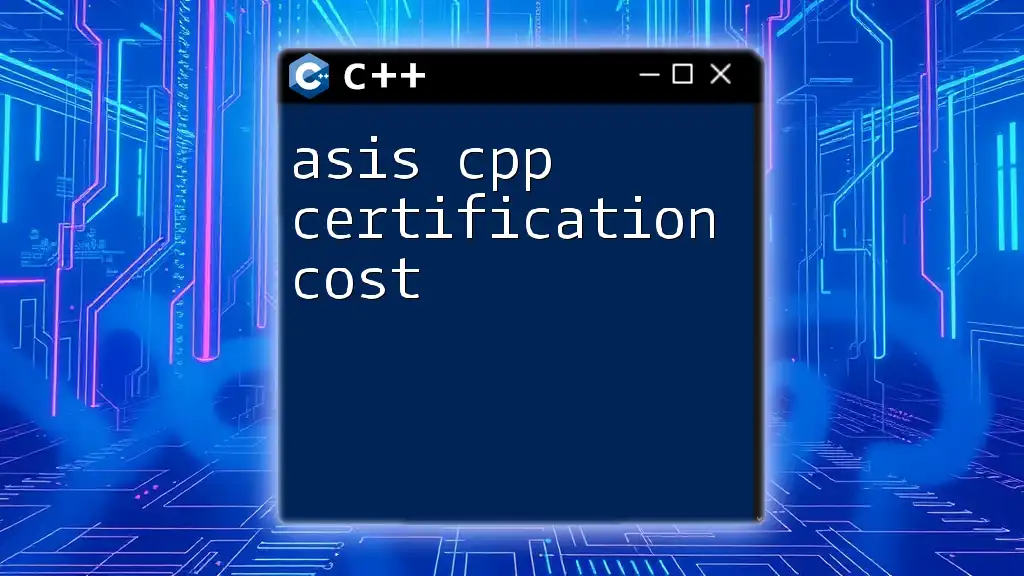
Maintaining Your C++ Certification
Renewal and Continuing Education
Once you achieve your C++ certification, be aware of necessary steps to maintain it. Most certifications require renewal every few years, which can include:
-
Additional coursework: Staying current with evolving industry standards through continual learning.
-
Professional development: Engaging in projects that allow you to apply new skills and knowledge.
Continuing Education Resources
Continuing education is vital for career growth. Look for:
-
Advanced courses: Pursue specialized training in areas like advanced algorithms or design patterns.
-
Programming communities and forums: Joining communities like Stack Overflow or GitHub offers valuable insights, collaboration opportunities, and a space to ask questions.
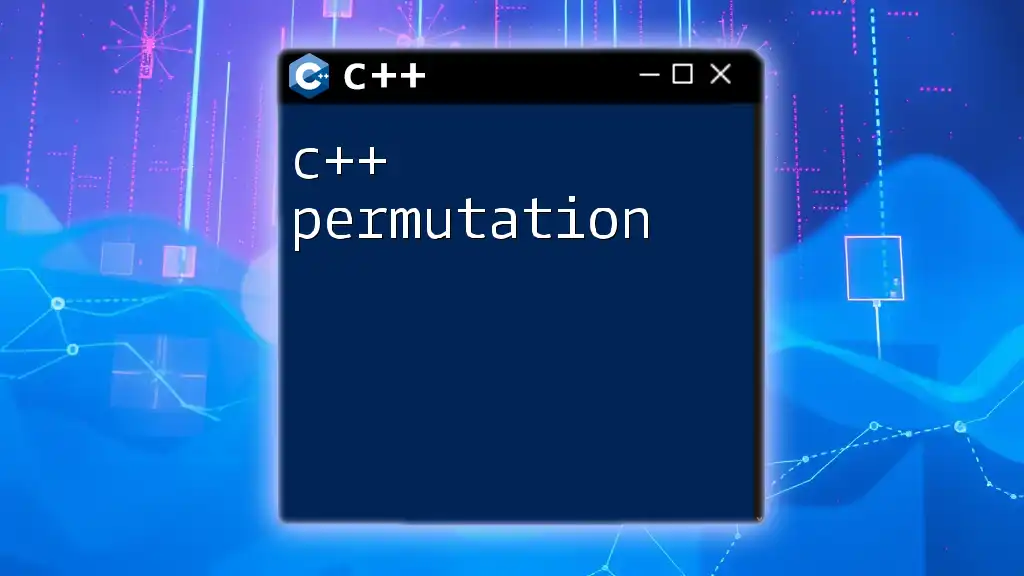
Conclusion
Is C++ Certification Worth It?
In summary, the decision to pursue a C++ certification can significantly impact your career trajectory. The structured learning that a certification provides, coupled with recognition from potential employers, makes it an attractive option for those serious about their programming careers.
Final Thoughts and Next Steps
If you're considering taking the C++ certification exam, start by gathering resources, mapping out a study plan, and engaging in practice exercises. Your commitment to this journey can propel you into a successful career in software development, where proficiency in C++ is a valuable asset. Begin your C++ certification journey today and unlock new opportunities!