C++ coroutines are a feature that allows functions to pause execution and resume later, enabling writing asynchronous code more intuitively and efficiently.
Here's a simple example of a coroutine that generates a sequence of numbers:
#include <iostream>
#include <coroutine>
struct Generator {
struct promise_type {
int current_value;
auto get_return_object() { return Generator{this}; }
auto yield_value(int value) {
current_value = value;
return std::suspend_always{};
}
auto return_void() { return; }
auto unhandled_exception() {}
};
promise_type *promise;
Generator(promise_type *p) : promise(p) {}
bool next() {
return promise->current_value != 0;
}
int value() { return promise->current_value; }
};
Generator numbers() {
for (int i = 1; i <= 5; ++i) {
co_yield i;
}
}
int main() {
auto gen = numbers();
while (gen.next()) {
std::cout << gen.value() << ' ';
}
return 0;
}
Introduction to C++ Coroutines
What are Coroutines?
Coroutines are a type of control structure that generalizes subroutines for non-preemptive cooperative multitasking. In simpler terms, coroutines allow functions to pause execution and yield control back to the caller, allowing the state of the function to be preserved and resumed later. This ability makes them extremely useful for asynchronous programming, where operations such as I/O can be handled without blocking the entire program.
Comparison with Threads
Coroutines differ from threads in that they provide a more lightweight way to manage concurrency. Threads operate in a preemptive manner, where the operating system schedules them, potentially leading to complexity due to context switching. In contrast, coroutines are user-controlled; they yield execution voluntarily, and the costs of managing them are generally lower.
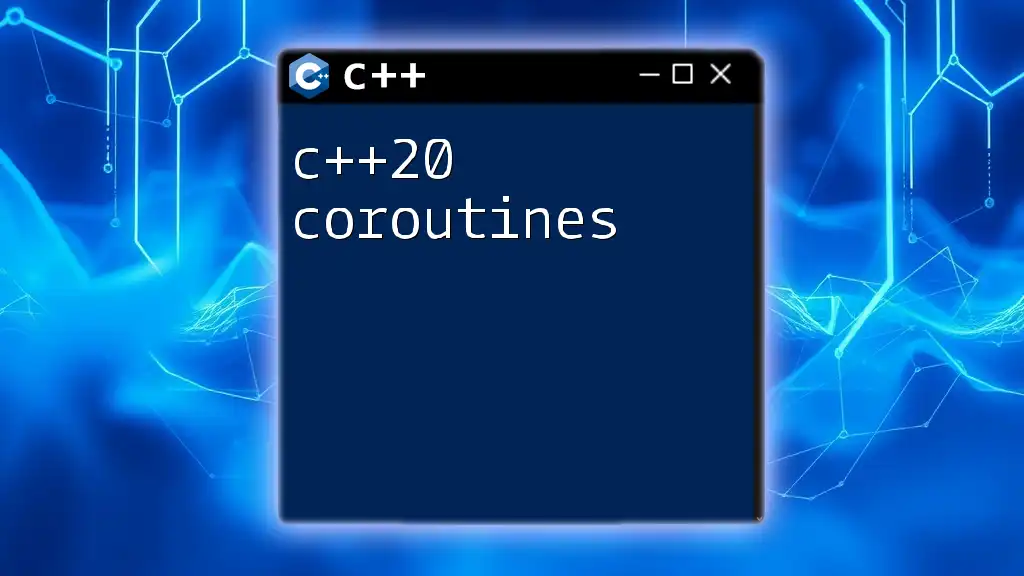
The Basics of C++ Coroutines
C++ Coroutine Syntax
With the introduction of C++20, a new and simplified syntax for creating coroutines was introduced. C++ coroutines leverage three key keywords:
- `co_await`: Used to suspend the coroutine execution until the awaited operation is complete.
- `co_yield`: Used to produce a value from the coroutine and suspend its state until resumed.
- `co_return`: Signals the end of the coroutine and returns a value, if applicable.
How Coroutines Work
Execution Context
Every coroutine maintains its own state, stored in an execution context. This context includes local variables and where the coroutine paused its execution. Unlike threads that may have a lot of overhead, coroutines keep state management lightweight, leading to improved performance.
Lifecycle of a Coroutine
A coroutine has a defined lifecycle, which can be broken down into:
- Suspension: A coroutine can suspend execution by yielding control back to the calling context, typically via `co_await` or `co_yield`.
- Resumption: The execution can be resumed later using coroutine handles, allowing continuation from the exact point it was suspended.
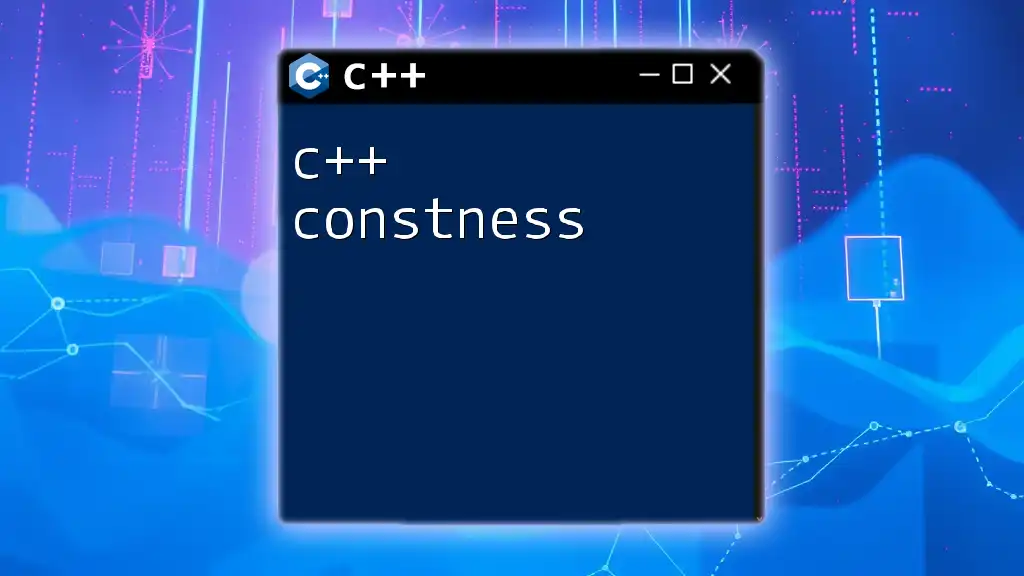
Setting Up Your Environment for C++ Coroutines
C++20 Support
To work with C++ coroutines, you need to ensure that your development environment supports C++20 features. Major compilers like GCC, Clang, and MSVC have incorporated these changes. For instance, you can enable C++20 support in your IDE by adjusting the compiler settings, ensuring the flag `-std=c++20` is included for GCC and Clang.
Basic Example of a Coroutine in C++
Here’s a simple example of a coroutine that counts numbers and yields back to the caller:
#include <iostream>
#include <coroutine>
struct Counter {
struct promise_type {
Counter get_return_object() {
return {};
}
std::suspend_always yield_value(int value) {
std::cout << "Yield: " << value << '\n';
return {};
}
void return_void() {}
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
};
using handle_type = std::coroutine_handle<promise_type>;
};
Counter count() {
for (int i = 0; i < 5; ++i) {
co_yield i;
}
}
int main() {
auto counter = count();
return 0;
}
In this example, the `count` function is a coroutine. When it is called, it returns an object that interacts with the coroutine's lifecycle. Each time `co_yield` is reached, the coroutine pauses and yields the current loop index. Upon resumption, it continues from where it left off.
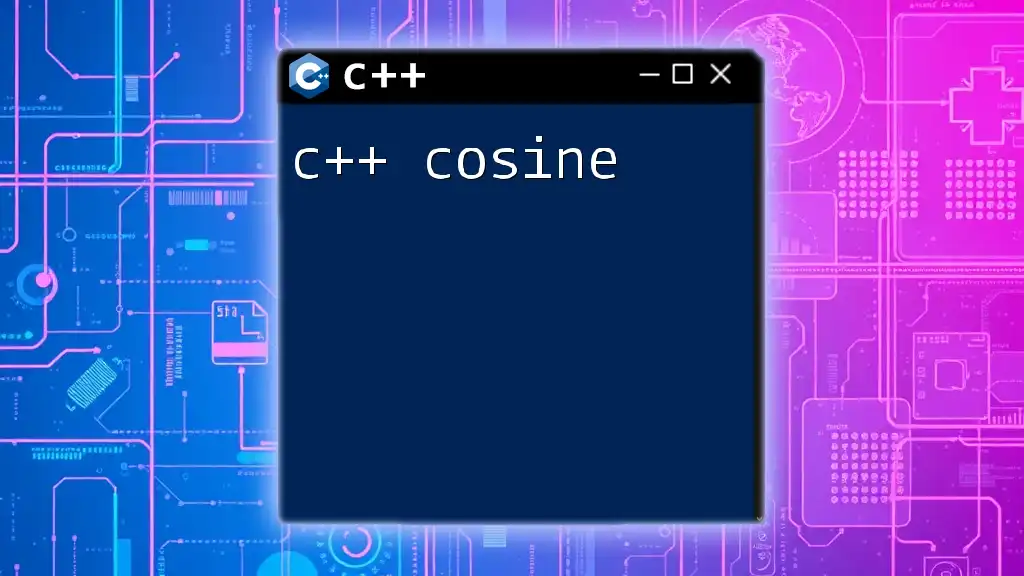
Advanced Coroutine Concepts
Awaitables and Awaiters
What is an Awaitable?
An awaitable is an object that can be used with `co_await`. When you await an operation, the coroutine can suspend until the operation is completed. An awaitable type must implement two functions that define its behavior: `await_ready`, `await_suspend`, and `await_resume`.
Creating Custom Awaitables
Creating your own awaitable type can be powerful, especially in projects that require custom asynchronous behavior. Here’s an example:
#include <iostream>
#include <coroutine>
struct MyAwaitable {
struct awaiter {
bool await_ready() { return false; }
void await_suspend(std::coroutine_handle<>) {}
void await_resume() { std::cout << "Operation complete!\n"; }
};
awaiter operator co_await() { return {}; }
};
struct MyCoroutine {
struct promise_type {
MyCoroutine get_return_object() {
return {};
}
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
void unhandled_exception() {}
};
};
MyCoroutine example() {
co_await MyAwaitable{};
}
int main() {
example();
return 0;
}
In this snippet, `MyAwaitable` creates an awaitable object that can be used in a coroutine. It merely simulates an asynchronous operation that resumes and outputs a message.
Coroutine Return Types
Standard Return Types vs. Custom Return Types
With C++ coroutines, you can use standard types like `void`, and `int`, but you can also create custom return types that employ `std::coroutine_handle`. This versatility allows for complex control flows and state management that can be tailored to project needs.
Here’s an example of a coroutine utilizing a custom return type:
#include <iostream>
#include <coroutine>
struct CoroutineHandle {
struct promise_type {
CoroutineHandle get_return_object() { return {}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
};
};
CoroutineHandle example() {
std::cout << "Coroutine started\n";
co_return;
std::cout << "Coroutine resumed\n";
}
int main() {
example();
return 0;
}
In this code, the coroutine logs a message upon starting. After it yields and resumes, a second log shows that it continued from where it left off.
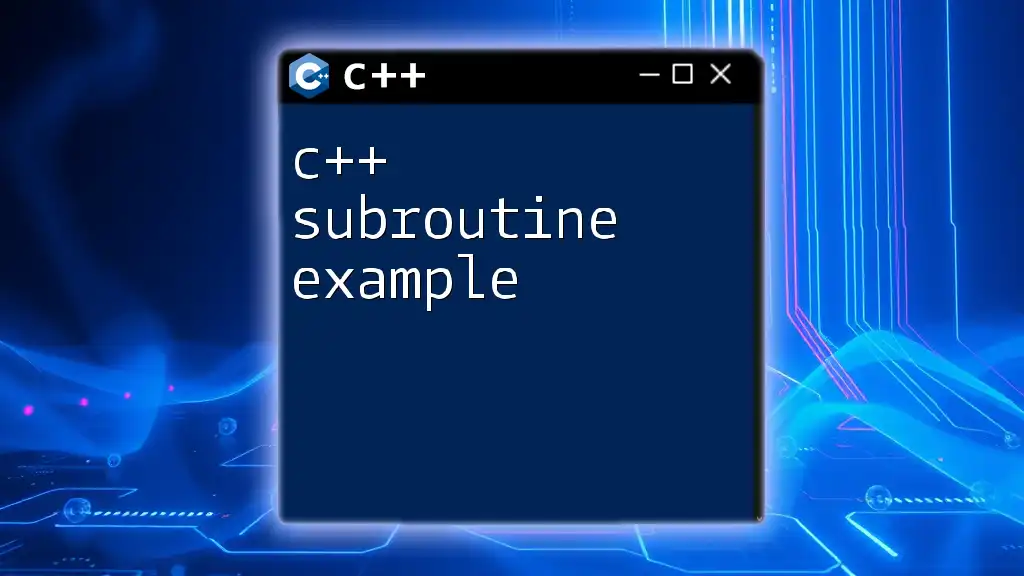
Practical Use Cases for C++ Coroutines
Asynchronous Programming
C++ coroutines can make asynchronous programming drastically more manageable. When you have operations that rely on waiting for responses (like network calls), you can instead use `co_await` to pause until the work is done, thus improving code readability and maintainability.
Game Development
In game development, managing numerous simultaneous tasks—like physics updates, rendering, and AI behaviors—can be cumbersome. Coroutines allow for an elegant solution in managing these continuations, maintaining the game's performance and responsiveness while handling complex game logic.
Networking
Network programming often involves many asynchronous tasks, such as sending and receiving data. Using coroutines eliminates the complexity of callbacks and simplifies error handling. Implementing a coroutine-based network client can look something like this:
#include <iostream>
#include <coroutine>
struct NetworkAwaitable {
struct awaiter {
bool await_ready() { return false; }
void await_suspend(std::coroutine_handle<>) { /* initiate async operation */ }
void await_resume() { std::cout << "Data received!\n"; }
};
awaiter operator co_await() { return {}; }
};
struct NetworkCoroutine {
struct promise_type {
NetworkCoroutine get_return_object() { return {}; }
std::suspend_always initial_suspend() { return {}; }
std::suspend_always final_suspend() noexcept { return {}; }
void return_void() {}
};
};
NetworkCoroutine async_network_request() {
co_await NetworkAwaitable{};
}
int main() {
async_network_request();
return 0;
}
Here, `async_network_request` demonstrates awaiting a network operation, making handling network calls more straightforward.
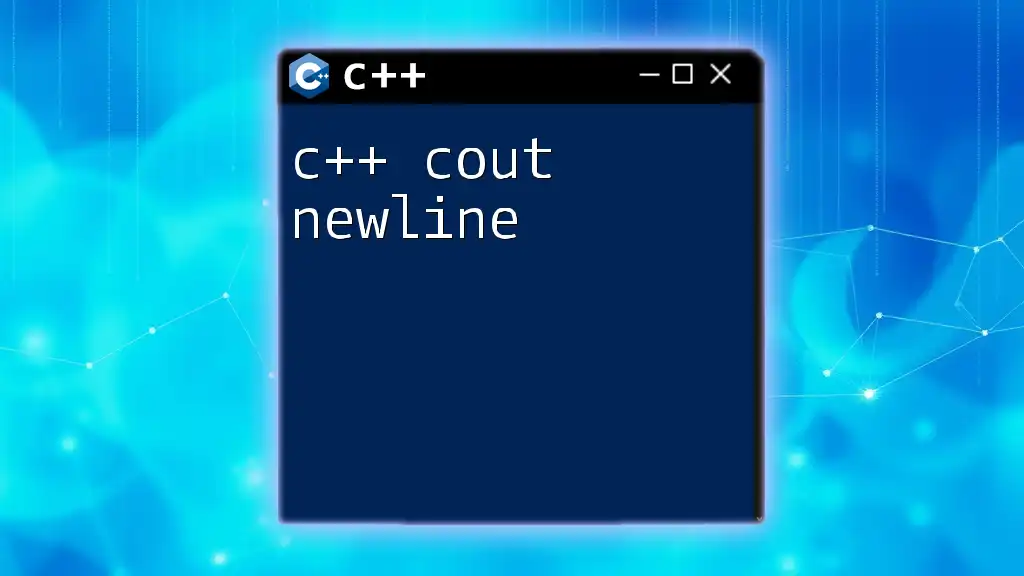
Best Practices for Using C++ Coroutines
Error Handling in Coroutines
When using C++ coroutines, it's essential to implement robust error handling to manage exceptions effectively. Often, exceptions propagated within a coroutine need to be caught and handled appropriately to maintain the program's integrity. Custom awaiters should also incorporate exception safety for a smoother experience.
Performance Considerations
While C++ coroutines bring several advantages, overusing them can introduce overhead. It is critical to optimize coroutine design, ensuring that memory allocation and suspension costs are minimal wherever possible. Profiling tools can help evaluate coroutine performance in your application, allowing you to make informed decisions.
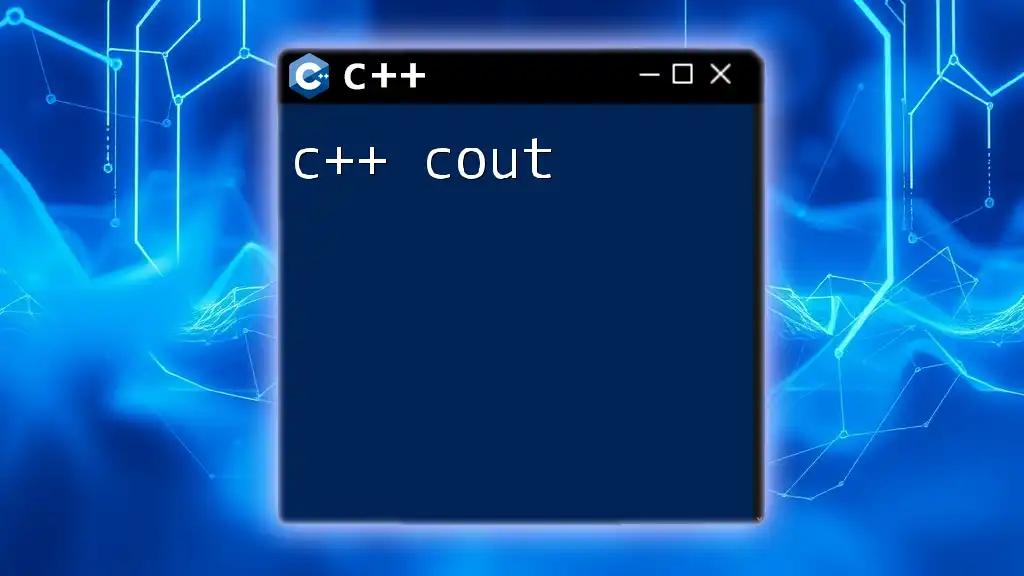
Troubleshooting Common Issues with C++ Coroutines
Debugging Coroutines
Debugging coroutines can present unique challenges due to their asynchronous nature. Utilizing modern IDE features, such as breakpoints and step-into capabilities, can help track the execution flow. Introducing logging within coroutines can also provide insight into their behavior during development.
Compatibility Issues
As C++20 features may not be uniformly supported in all environments, compatibility issues can arise. Always ensure the latest version of your compiler is utilized, and consider consulting the documentation for a feature’s compatibility. Workarounds, such as backporting functionalities or using libraries, may also be viable solutions.
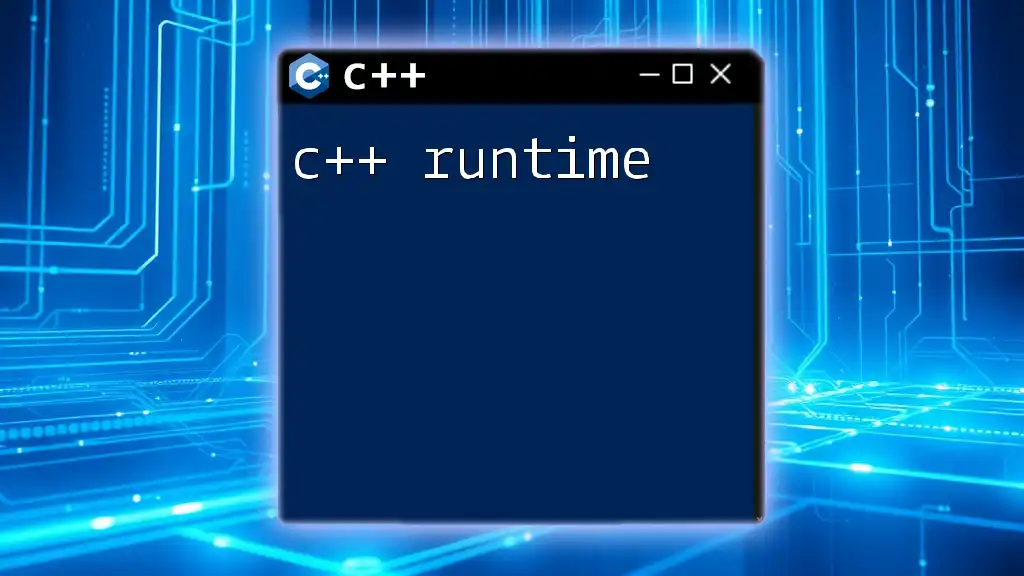
Conclusion
C++ coroutines are a powerful addition to the C++ language, enabling asynchronous programming with ease and efficiency. Their ability to create lightweight, maintainable code can greatly enhance software design, particularly in performance-sensitive applications like games and networked systems. As the language evolves, coroutines will continue to play a vital role in simplifying the complexity of concurrent programming.
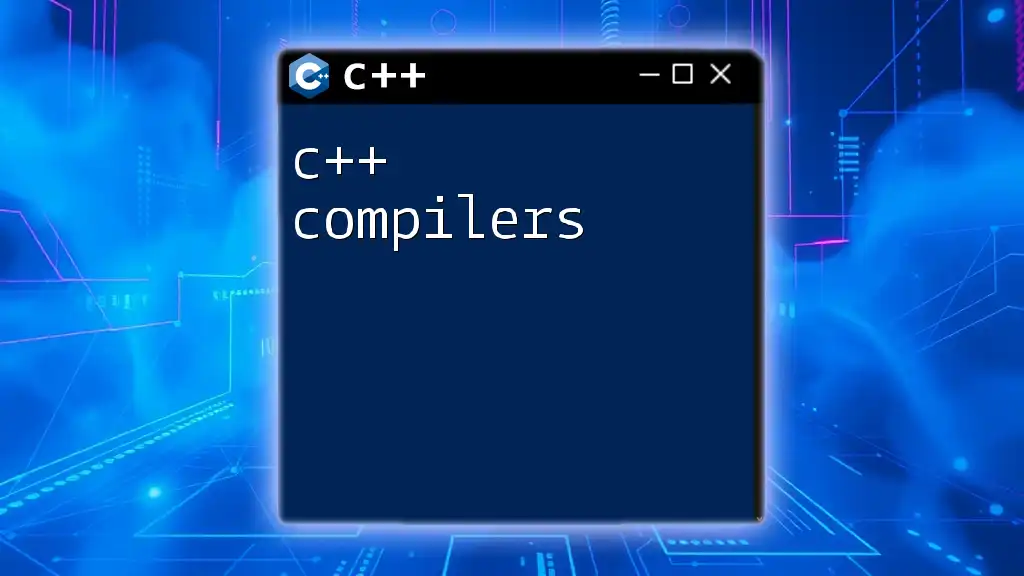
Additional Resources
For those looking to deepen their understanding of C++ coroutines, consider exploring specialized books and online communities dedicated to C++ features. Engaging with fellow developers can provide insights and foster a shared learning experience.