A C++ profiler is a tool that helps developers analyze the performance of their C++ code by measuring execution time, memory usage, and other critical metrics to optimize their applications.
Here's a simple example of how you might use a profiler in your code to measure the execution time of a function:
#include <iostream>
#include <chrono>
void exampleFunction() {
// Simulate some work with a loop
for (int i = 0; i < 1000000; ++i);
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
exampleFunction();
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Elapsed time: " << elapsed.count() << " seconds\n";
return 0;
}
Understanding C++ Profiling
What is a C++ Profiler?
A C++ profiler is a powerful tool that helps developers understand the performance of their applications. By collecting data about various aspects of a program during its execution, profilers allow you to identify bottlenecks, optimize resource usage, and enhance overall efficiency. Proper profiling is crucial in software development as it enables a systematic approach to improving performance, hence making applications faster and more reliable.
Types of Profiling
When delving into C++ profiling, it's vital to recognize the different methodologies applied in this domain:
Static vs. Dynamic Profiling
-
Static Profiling involves analyzing the code without executing it. This might include code reviews, complexity analysis, or static analysis tools that inspect code structure and potential issues.
-
Dynamic Profiling takes place while the application is running, collecting real-time data on performance metrics. This type is more beneficial for understanding actual execution behavior, including memory usage and function call frequency.
Both methods are essential depending on the stage of development and specific goals, providing unique insights into different areas.
Instrumentation Profiling vs. Sampling Profiling
-
Instrumentation Profiling requires developers to insert specific code points (instrumentation) into their application to collect detailed execution information. This method, while precise, can introduce overhead, potentially skewing the application's performance.
-
Sampling Profiling, on the other hand, takes sporadic snapshots of the program state at defined intervals or triggers. It collects less detailed data but has lower performance overhead. It’s best suited for understanding trends in application performance rather than pinpointing precise issues.
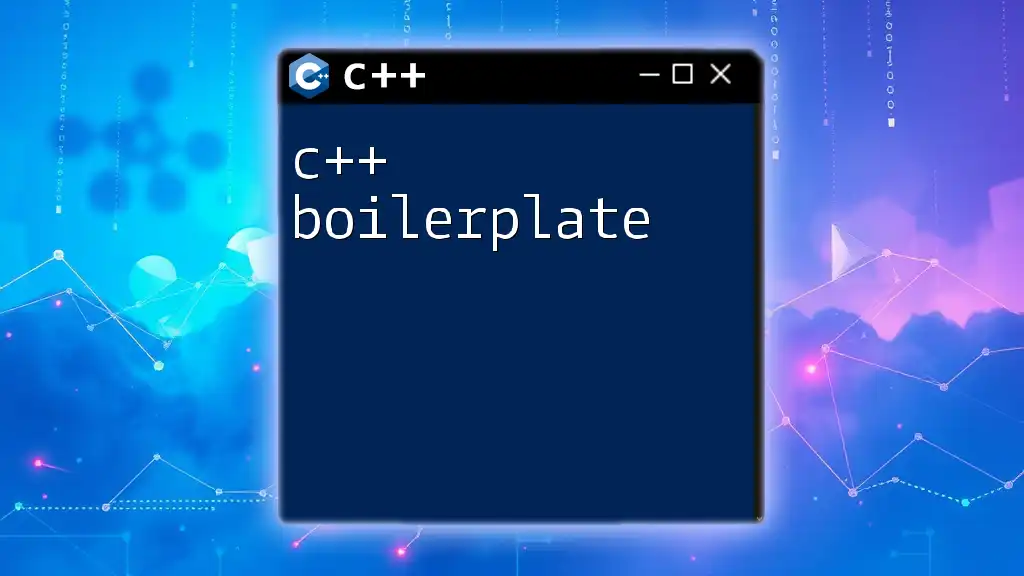
Popular C++ Profiling Tools
Gprof
Gprof is a widely-used profiler for C and C++ applications. To utilize Gprof effectively, one must install it and compile the application with the `-pg` flag:
g++ -pg -o my_application my_application.cpp
After executing the application, Gprof generates a `gmon.out` file containing profiling information. To view results, run:
gprof my_application gmon.out
Interpreting Gprof’s output involves understanding key metrics such as time spent in functions and call graphs. This information can guide your optimization efforts by revealing which functions are consuming the most resources.
Valgrind
Valgrind offers several powerful tools, among which Memcheck and Cachegrind are standout features. To run an application under Valgrind, simply prefix your command with `valgrind`:
valgrind --tool=cachegrind ./my_application
Cachegrind will generate output files that provide detailed cache usage statistics. Visualizing this data can be accomplished with additional tools like `kcachegrind`, enhancing the ease of interpretation.
Visual Studio Profiler
For developers using Visual Studio, the built-in profiler provides seamless integration. To profile a C++ application, you can access the Performance Profiler from the Analyze menu. After selecting the type of profiling you want, Visual Studio captures performance metrics during your application’s execution.
A practical example involves profiling a simple C++ console application. After reviewing the profiling data gathered, you can identify slow functions, memory allocation issues, and CPU bottlenecks with detailed graphs and charts.
Other Notable Profilers
Perf is an excellent tool for Linux systems offering system-wide profiling capabilities. It's particularly useful when analyzing the performance of kernel and hardware interaction. To use it effectively, run:
perf record ./my_application
perf report
This generates a report showing where CPU time is spent, allowing for a deeper analysis of performance issues.
Intel VTune Profiler caters to those seeking advanced profiling features tailored for Intel’s architectures. It provides highly detailed insights into CPU and GPU utilization, memory access, and threading issues. VTune is highly effective for optimizing performance on Intel hardware, making it a valuable tool for specific use cases.
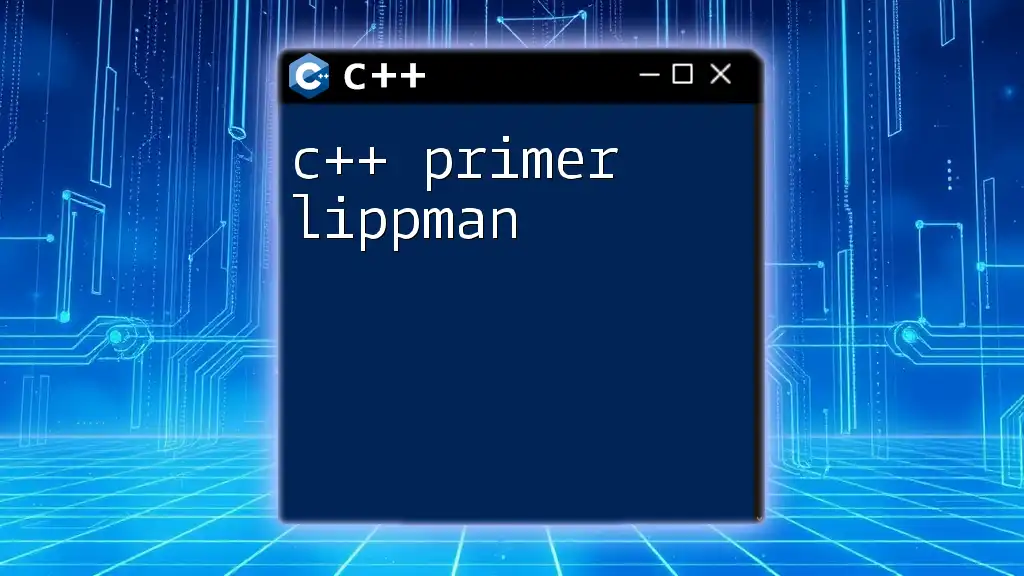
How to Profile a C++ Application
Preparing for Profiling
Effective profiling begins with preparation. Ensuring your application is set up correctly involves compiling it with specific profiling flags. For instance, in GCC you can use:
g++ -g -O2 -pg -o my_application my_application.cpp
The `-g` flag includes debugging symbols, while `-O2` (optimizations) can lead to more realistic profiling results. Always strive to replicate production-like environments to achieve meaningful insights.
Profiling Workflow
The profiling workflow is a structured approach to identifying performance bottlenecks:
Step 1: Identify Performance Bottlenecks
Before profiling, pinpoint which areas of your application need attention. This might include functions experiencing high delay or excessive memory consumption. Knowing where to focus your profiling efforts enhances efficiency.
Step 2: Run the Profiler
Launch your chosen profiler and execute your application in a monitored environment. Take note of particular scenarios that put more strain on your application, as these represent valuable learning opportunities.
Step 3: Analyze Results
The analysis phase is where you synthesize the data collected during profiling. Look for abnormal CPU usage spikes or disproportionate memory allocations. Understanding the characteristics of each bottleneck allows for targeted optimizations.
Profiling for Memory Leaks
Memory leaks can significantly degrade performance. Using Valgrind’s Memcheck tool, one can easily identify memory allocation issues.
For instance, consider a situation where your application fails to free memory. After adding Valgrind, running it on your application can produce output indicating where the leaks are occurring. An example command is:
valgrind --leak-check=full ./my_application
This output will highlight any unfreed memory blocks and guide you toward resolution.
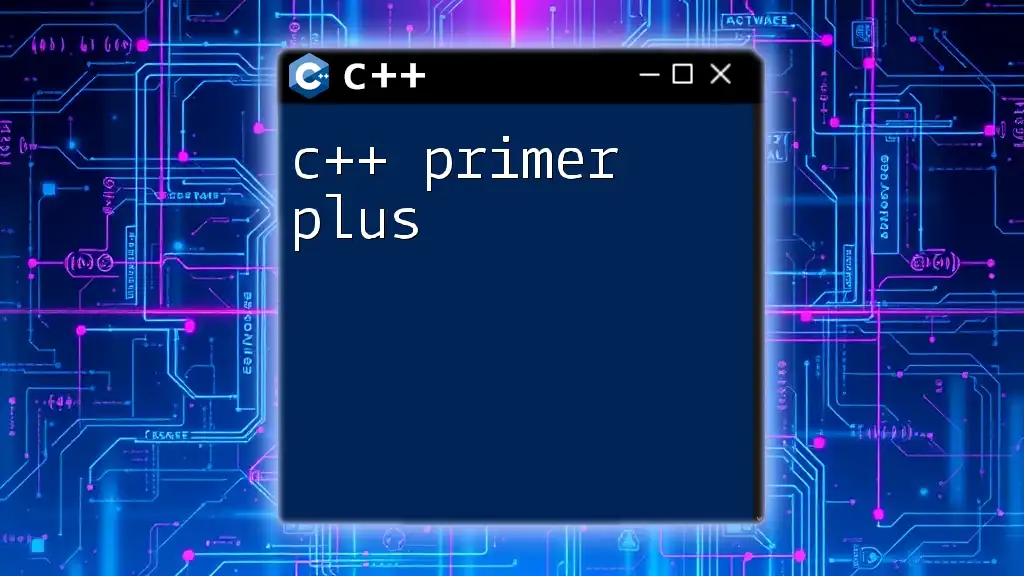
Best Practices for C++ Profiling
Keeping the Profiled Code Clean
Writing clean and organized code is imperative for effective profiling. Developing a clear structure assists both humans and tools in understanding the purpose and function of segments of code. Utilize naming conventions, comments, and modular designs to maintain clarity throughout your application.
Regular Profiling
Integrating profiling into your routine allows for ongoing performance assessment. Applying profiling as part of your Continuous Integration/Continuous Deployment (CI/CD) pipeline can help catch issues early, ensuring consistent performance levels throughout development.
Learning from Profiling Data
A fundamental aspect of profiling is translating insights into actionable improvements. Use your profiling experiences to form a feedback loop that drives adjustments in coding practices, design principles, and optimization strategies.
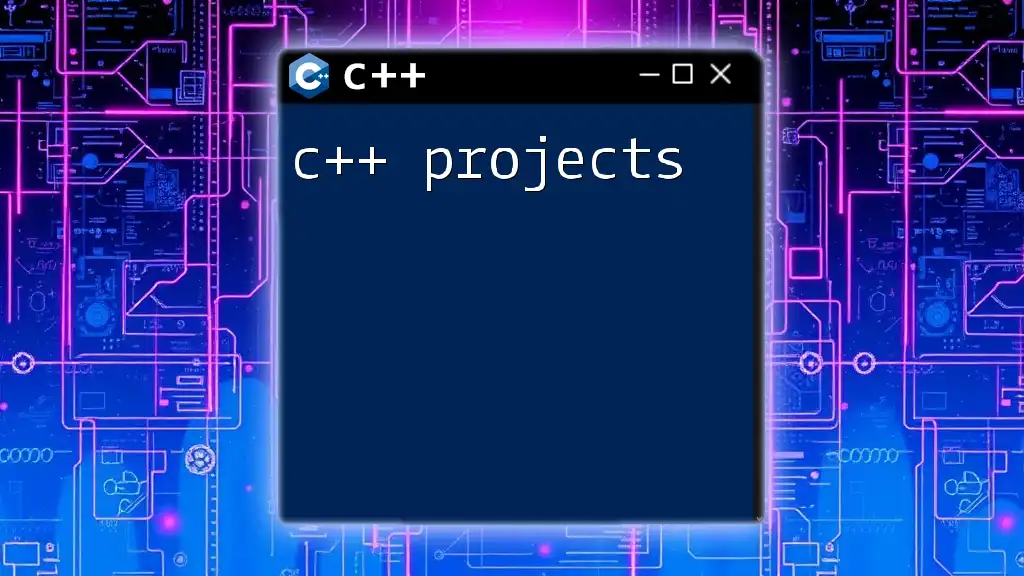
Conclusion
As technology evolves, so too does the landscape of performance profiling. Using a C++ profiler is not just beneficial; it's essential for developers dedicated to maintaining high-performance applications. Emphasizing regular evaluation and continuous refinement cultivates a deeper understanding of your software and enhances its reliability.
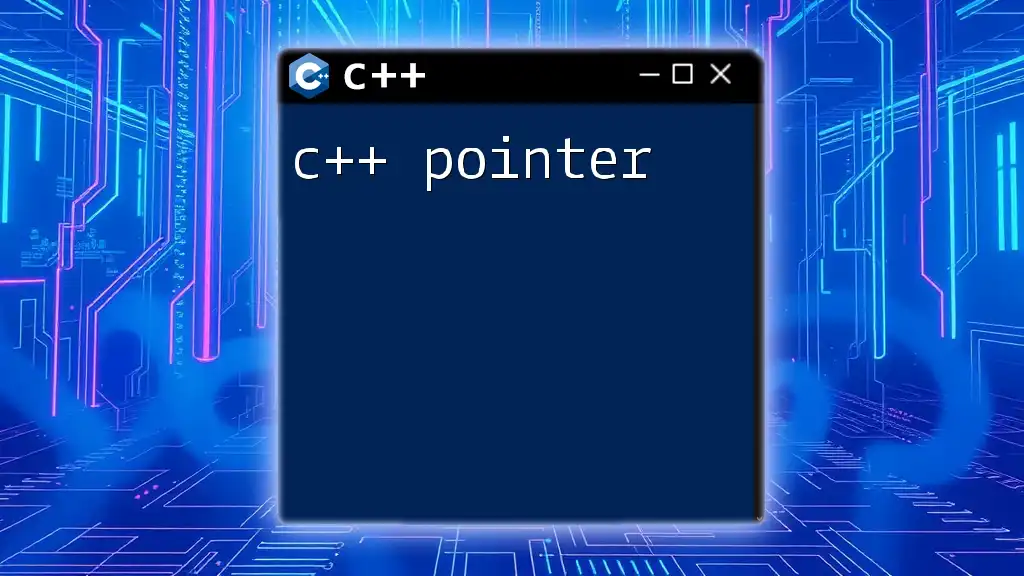
Additional Resources
Books, Blogs, and Tutorials
To further your knowledge in profiling, consider reading books and following blogs on performance optimization. Engaging with tutorials can also provide practical insights and hands-on practice.
Community Forums and Support
Participating in community forums and online discussions is invaluable. Platforms like Stack Overflow and specific C++ development forums can serve as excellent resources for troubleshooting and tips, allowing developers to share knowledge and experiences.