C++ doesn't have properties like C#, but you can achieve a similar effect using getter and setter methods in a class to encapsulate the attributes.
class Sample {
private:
int value;
public:
// Getter
int getValue() const { return value; }
// Setter
void setValue(int v) { value = v; }
};
Understanding Properties in C#
Properties in C# provide a flexible mechanism to access and manipulate object data in a controlled manner. In essence, a property can be thought of as a combination of a private variable (field) and a pair of accessor methods (getters and setters) that control access to that variable.
The primary benefits of using properties over traditional public fields include:
- Encapsulation: Properties allow you to control how fields are accessed and modified.
- Validation: You can incorporate logic in setters to validate data or trigger other actions when the property value changes.
- Read-Only or Write-Only Access: You can define properties that are only readable or writable, enhancing data integrity.
Here is an example demonstrating a property in C#:
public class Person
{
private string name;
public string Name
{
get { return name; }
set { name = value; }
}
}
In this example, the property `Name` controls access to the private variable `name`. The `get` accessor returns the value of `name`, while the `set` accessor allows it to be modified.

Why C++ Lacks Native Property Support
C++ was designed with a focus on system programming and high performance. Unlike C#, which emphasizes ease of use and abstraction, C++ often leans towards providing granular control over system resources.
As a consequence of this design philosophy, C++ lacks native support for properties. While the absence of direct property functionality may seem limiting, it leads to alternative strategies programmers can utilize for similar benefits. Emulating C#-style properties in C++ involves getting creative with traditional principles.
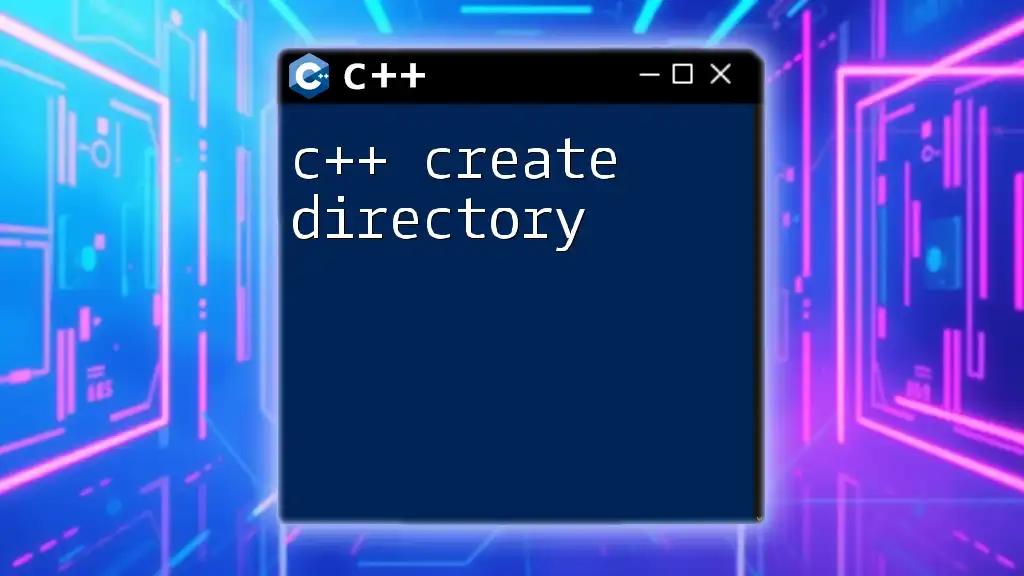
Implementing Properties in C++
In C++, the closest alternative to properties are getter and setter methods. This traditional design pattern provides a clean interface for accessing private members of a class.
Basic Syntax of Getters and Setters in C++
Here is a fundamental implementation of getters and setters in C++:
class Person {
private:
std::string name;
public:
std::string getName() const {
return name;
}
void setName(const std::string& newName) {
name = newName;
}
};
In this example, the `getName` method retrieves the name, while `setName` allows you to modify it. As with C#, this ensures encapsulation, allowing you to implement more intricate logic if needed.
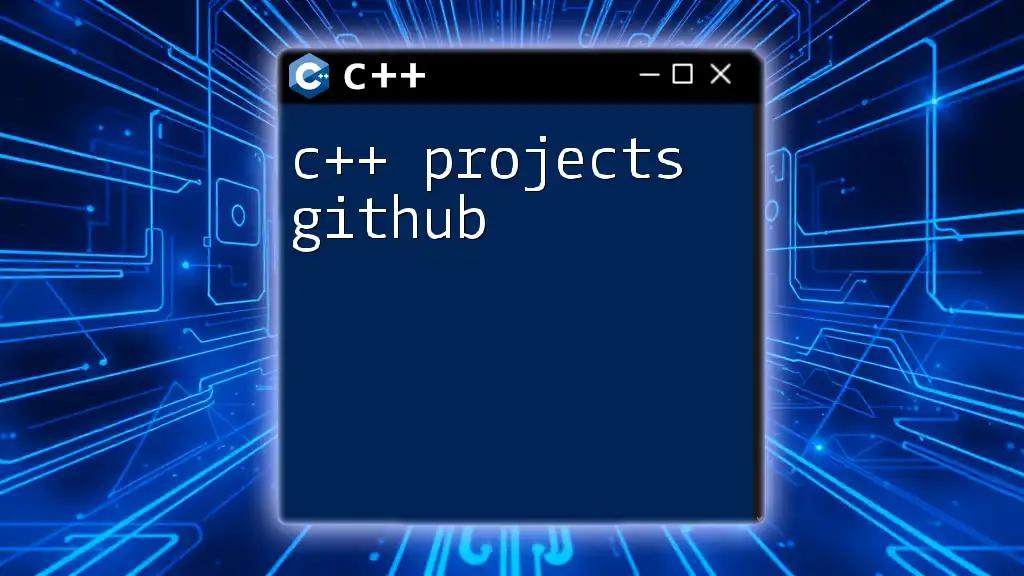
Using Accessor Functions as C++ Properties
Accessor functions serve as C++'s equivalent to properties. They enable controlled access to private variables and can encapsulate additional logic such as validation.
Example of Accessor Functions
Consider this code snippet:
class Person {
private:
std::string name;
public:
std::string getName() const {
return name;
}
void setName(const std::string& newName) {
name = newName;
}
};
Here, `getName()` provides a straightforward way to access the `name` attribute, and `setName()` enables modification. By adopting this approach, you maintain encapsulation and can later expand on the logic within these methods without altering the class interface.
Best Practices in Accessor Design
When designing your getters and setters in C++, consider these best practices:
- Keep getter methods simple and efficient.
- Implement validation in setter methods to ensure data integrity.
- Prefer `const` methods for getters to indicate the intent that they will not modify the object state.
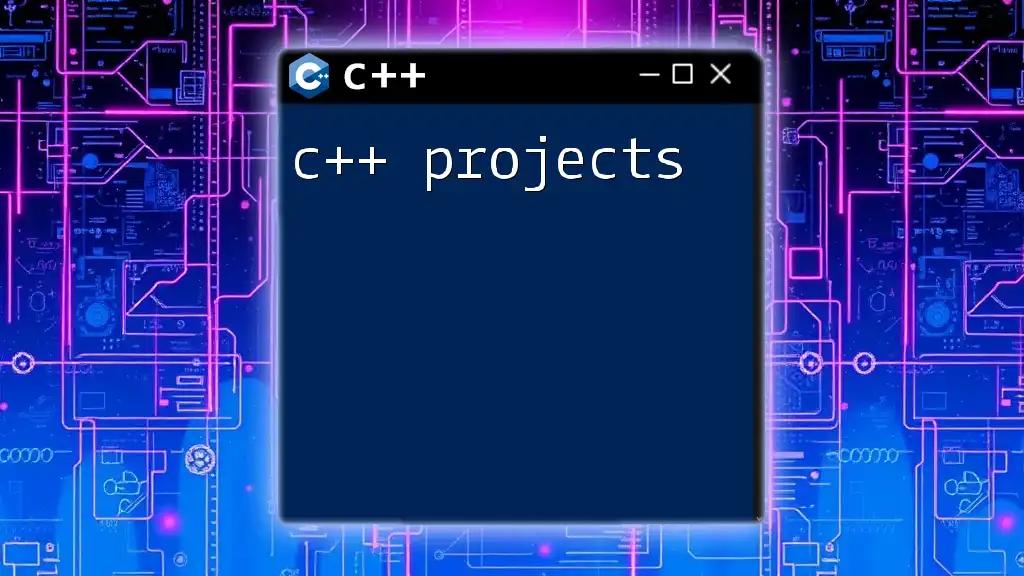
Leveraging C++11 Features for Property-like Syntax
With the introduction of C++11, new features have provided programmers greater flexibility. One of these is the ability to use `std::function` in combination with lambdas to create property-like functionality. This approach allows for a more succinct syntax.
Example Using Lambdas
Let’s illustrate this concept with the following example:
class Person {
private:
std::string name;
public:
std::function<std::string()> GetName = [&]() { return name; };
std::function<void(const std::string&)> SetName = [&](const std::string& newName) { name = newName; };
};
In this example, we define the property-like behavior with two lambda expressions. `GetName()` retrieves the name, and `SetName()` allows modification. This technique can streamline the syntax when using C++ in more modern contexts.

Use of Macros to Simplify Property Implementation
C++ allows the use of macros to simplify the boilerplate code needed to implement getters and setters, emulating a property-like syntax conveniently.
Example Code Illustrating Macro Usage
Consider the following macro implementation:
#define PROPERTY(type, name) \
private: type name; \
public: type get##name() const { return name; } \
void set##name(type value) { name = value; }
class Person {
PROPERTY(std::string, Name);
};
The `PROPERTY` macro allows you to declare a member variable and automatically generates the accompanying getter and setter. This way, you can focus more on business logic rather than repetitive code.
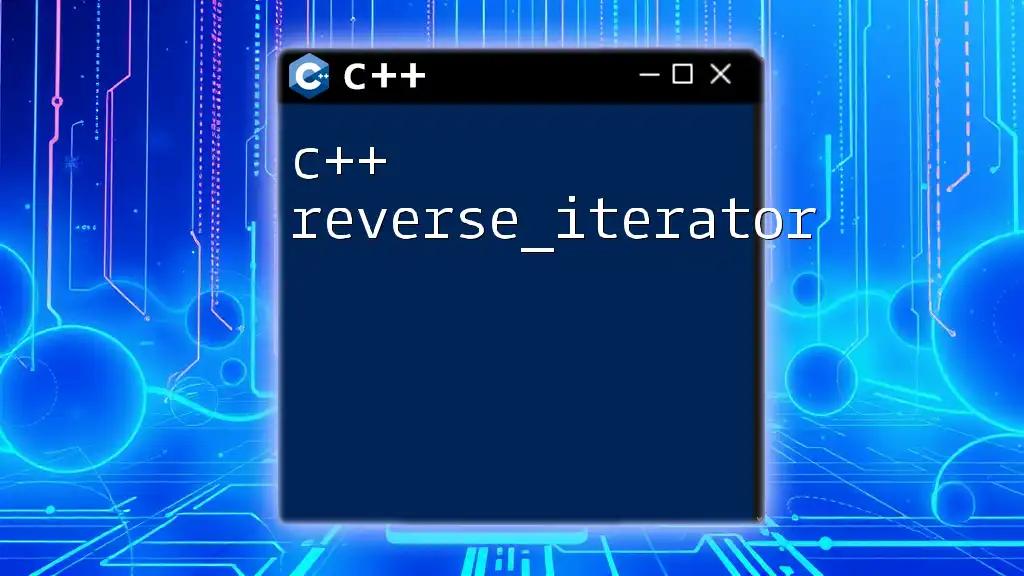
Exploring Third-Party Libraries for Properties in C++
To fully harness property-like behavior in C++, several libraries are available that offer more robust features akin to C# properties.
Introduction to Popular Libraries
One prominent library is Boost.Property, which provides tools for managing properties effectively. Using libraries enables a broader range of functionalities and eases some of the manual implementations required when utilizing standard C++.
Example Code Using Boost
#include <boost/property_tree/ptree.hpp>
Though this simple inclusion does little on its own, it opens doors to function-rich components designed for property management in various applications—from GUI frameworks to data transmission protocols.

Conclusion
In summary, while C++ does not offer properties in the same fashion as C#, it provides various ways to achieve similar functionality through getters, setters, and modern language constructs. By leveraging these mechanisms, you gain the advantages of encapsulation and data integrity, which are vital in robust software design.
As the C++ landscape evolves, programmers are encouraged to explore these methods and consider the practical implementation of property-like features within their work. Embracing innovations such as C++11 can help bridge the gap between C++ and C# designs, enhancing flexibility and efficiency in your programming endeavors.

Additional Resources
For further learning, consider exploring the following:
- The official C++ documentation for advanced features.
- C# resources for comparing language paradigms.
- Community forums and platforms like Stack Overflow for engaging discussions on implementing properties and other advanced concepts in C++.