Specialized templates in C++ allow you to define specific behaviors for a particular data type by providing an explicit implementation for that type, enabling you to customize template functions or classes for better performance or functionality.
#include <iostream>
#include <string>
// Primary template
template <typename T>
struct Calculator {
static void print() {
std::cout << "Generic calculator\n";
}
};
// Specialized template for std::string
template <>
struct Calculator<std::string> {
static void print() {
std::cout << "String calculator\n";
}
};
int main() {
Calculator<int>::print(); // Output: Generic calculator
Calculator<std::string>::print();// Output: String calculator
return 0;
}
Introduction to C++ Template Specialization
C++ template specialization is a powerful feature that allows developers to customize templates for specific data types. This technique is valuable because it enhances code flexibility and aids in optimizing specific implementations. Understanding how to effectively utilize C++ template specialization can significantly improve development efficiency and ensure precise type handling.
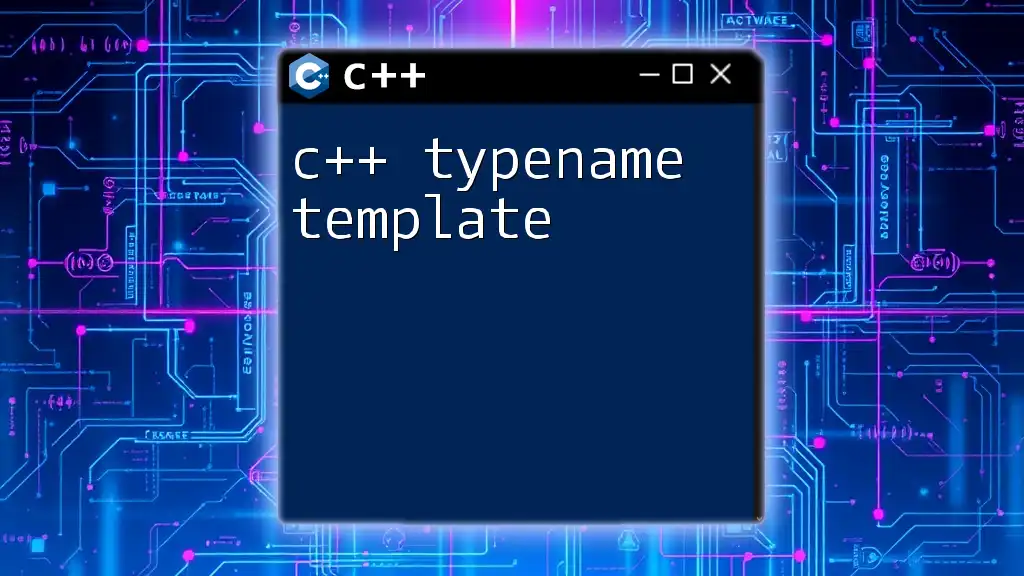
Understanding C++ Templates
What are Templates?
Templates in C++ are blueprints for creating functions or classes. They allow for writing generic and reusable code where the functionality does not change despite the varying data types. Two types of templates exist—function templates and class templates.
Function templates allow a single function definition to operate on various data types. For example:
template <typename T>
void print(T value) {
std::cout << value << std::endl;
}
Class templates provide a mechanism for creating a class that can handle various data types, similar to function templates.
Syntax of C++ Templates
The basic structure of a template is straightforward. The syntax involves the keyword `template` followed by template parameters enclosed in angle brackets. For example:
template <typename T>
class MyClass {
public:
T value;
MyClass(T val) : value(val) {}
};
In this declaration, `T` is a placeholder for any data type that can be passed as a parameter. Upon instantiation, the compiler replaces `T` with the specified type.
Understanding template parameters and arguments is crucial for mastering template specialization. Each specialization can offer customized implementations of templates depending on the types provided.
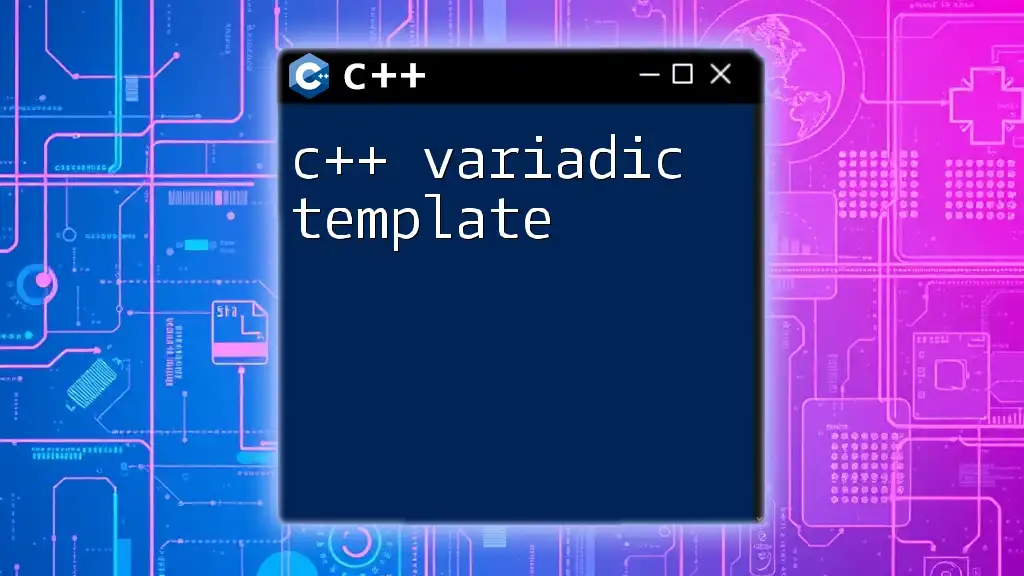
Types of Template Specialization
Full Specialization
Full specialization allows a template to be defined explicitly for a specific type. This is useful when you want to implement behavior exclusively for a certain data type without retaining the general functionality.
Example of Full Specialization:
template <typename T>
class Container {
public:
void add(const T& item) {
// General implementation
}
};
template <>
class Container<int> { // Full specialization for int
public:
void add(const int& item) {
// Specialized code for int
}
};
In this example, `Container<int>` has a distinct implementation compared to the generic `Container<T>`, illustrating the flexibility that full specialization provides.
Partial Specialization
Partial specialization allows templates to be customized for a subset of types, retaining part of the general structure. This is advantageous for providing specific behavior without losing the generic template functionality.
Example of Partial Specialization:
template <typename T, typename U>
class Pair {
public:
T first;
U second;
};
template <typename T>
class Pair<T, int> { // Partial specialization for U = int
public:
T first;
int second; // Specialized type
};
In this scenario, `Pair` is specialized for cases where the second type is an `int`, allowing us to handle that combination with particular rules.
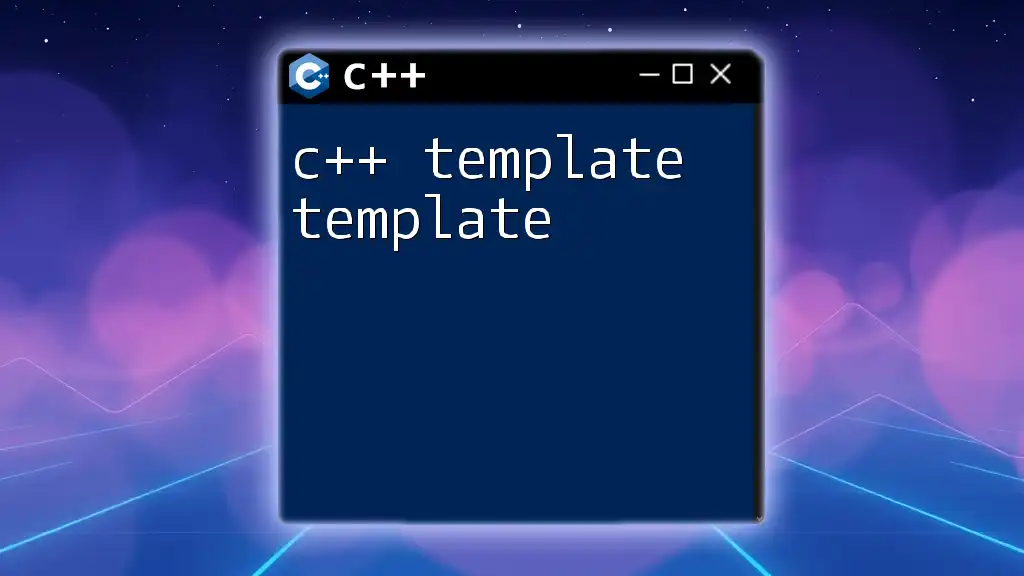
Benefits of C++ Template Specialization
Code Reusability
One of the primary benefits of using template specialization is enhanced code reusability. Specialized templates reduce redundancy by allowing developers to define specific implementations while maintaining a generic interface. This saves considerable time during development.
Increased Performance
Implementing specialized templates can lead to optimizations. By tailoring code to specific types, developers can enhance execution speed and efficiency. For instance, having a specialized template for integers can exploit their unique properties to perform faster computations.
Type Safety
Template specialization contributes to type safety by ensuring that specific implementations are bound to certain data types. This minimizes the risk of getting runtime errors due to incompatible data type operations, thereby enabling more robust application development.
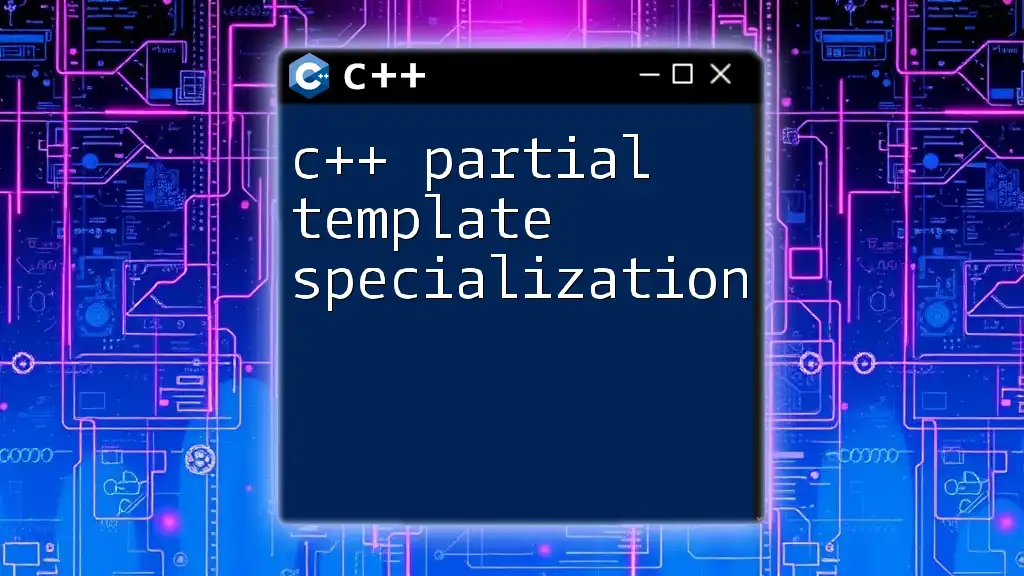
Advanced Concepts in C++ Template Specialization
SFINAE (Substitution Failure Is Not An Error)
SFINAE is a principle that allows template specialization to gracefully handle cases where template argument substitution fails. It gives developers the ability to create templates that only participate in overload resolution if certain conditions are met.
Example of SFINAE in Action:
template <typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
doSomething(T t) {
// Function only for integral types
}
In this case, `doSomething` will only be valid for integral types, preventing compilation errors for other types. This feature is invaluable for creating versatile and type-safe template behaviors.
Variadic Templates and Specialization
Variadic templates, introduced in C++11, allow templates to accept an arbitrary number of parameters. They have made implementing and specializing templates even more flexible.
Example of Variadic Template Specialization:
template <typename... Args>
class MultiContainer {
// General implementation
};
template <>
class MultiContainer<int, float> {
// Specialized implementation for int and float
};
Here, `MultiContainer<int, float>` demonstrates how variadic templates can be precisely tailored for specific combinations, providing even more robustness in code.
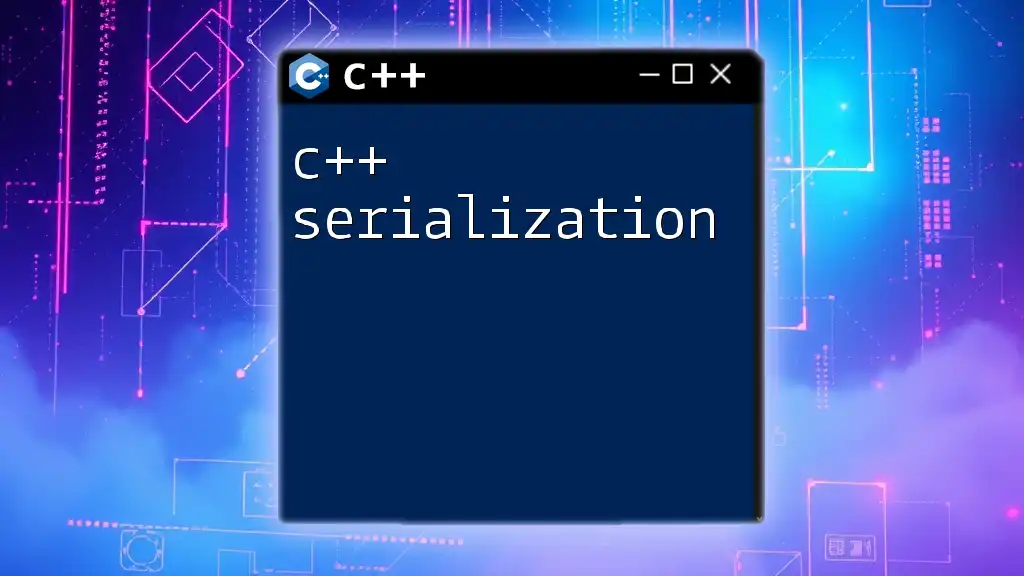
Practical Applications of C++ Template Specialization
Templates in Standard Library
The C++ Standard Library utilizes template specialization extensively. Classes like `std::vector`, `std::map`, and many others are designed using template principles. By understanding these libraries' specializations, developers can leverage built-in functionalities while ensuring efficiency and reliability.
Real-world Case Studies
Template specialization finds practical applications in various domains such as sorting algorithms or custom data structure implementations. For example, implementing a sorting function that behaves differently for different data types based on their characteristics illustrates the versatility of template specialization.
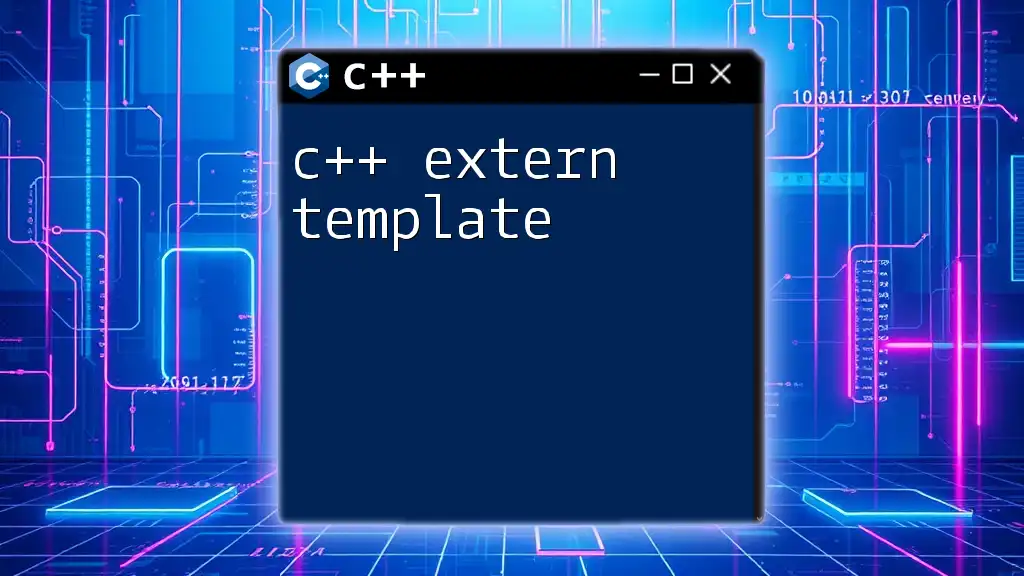
Common Issues and Troubleshooting Template Specialization
Template Deduction Failures
Template specialization can sometimes result in deduction failures due to mismatched types or incorrect argument usage. Understanding compiler errors regarding templates can improve debugging. Always ensure that the template arguments are compatible with the expected types.
Over-specialization
While specialization is powerful, excessive specialization can lead to brittle code that is difficult to maintain. Strive for a balance between generality and specificity; over-specialization can lead to an explosion of code variations that complicate debugging and enhancements.
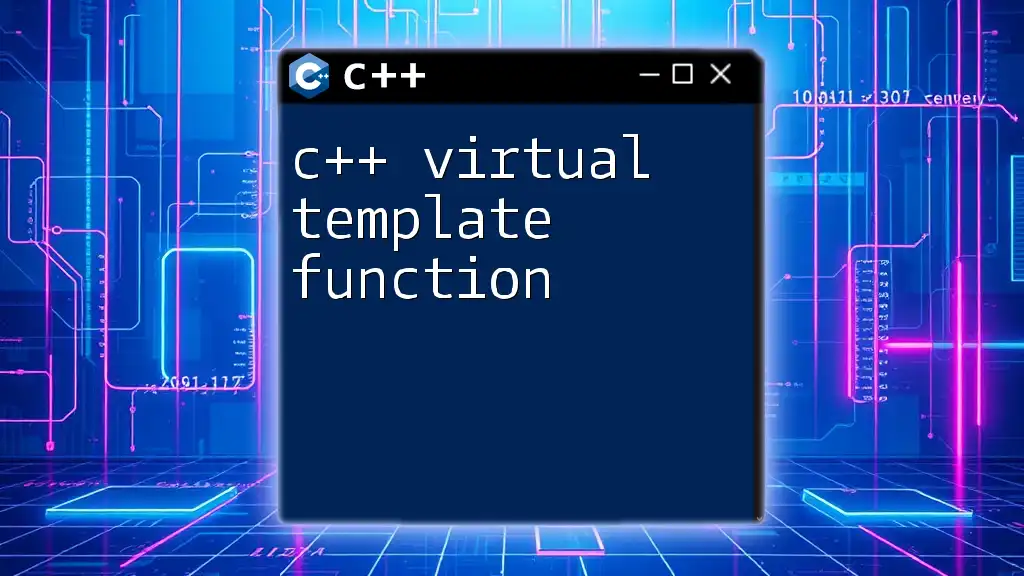
Conclusion
C++ template specialization is an essential tool for advanced programming that allows for customized, efficient, and type-safe code. By leveraging the principles of full and partial specialization, SFINAE, and variadic templates, developers can write code that handles various scenarios while optimizing performance. Embracing these concepts not only streamlines coding practices but also promotes a cleaner, more maintainable codebase. As you continue your journey with the c++ specialize template, practicing these principles will enhance your coding prowess and bolster your programming toolkit.
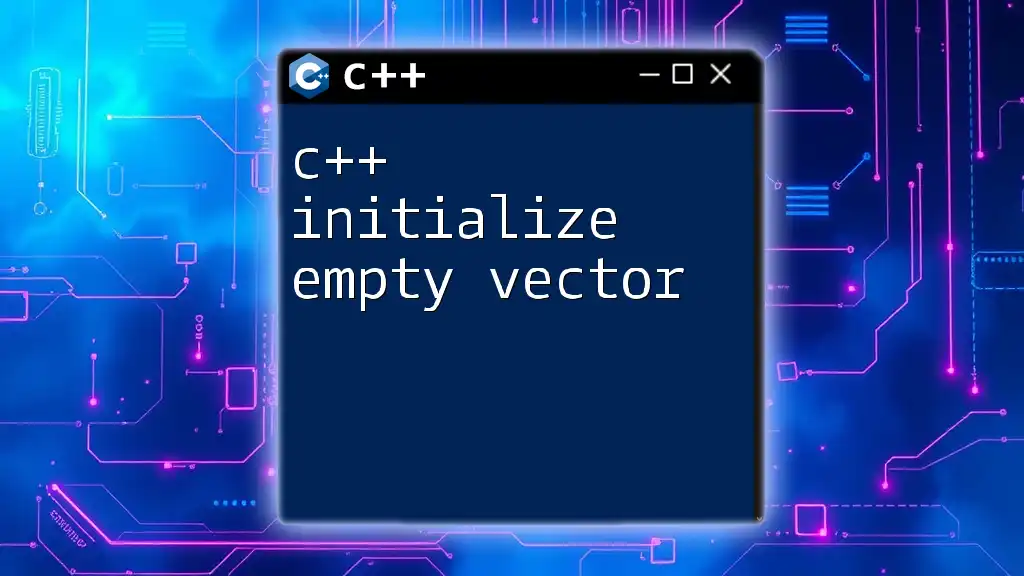
Additional Resources
For those looking to further their understanding of C++ template specialization, consider exploring recommended books, online courses, and community forums dedicated to C++. Engage with these resources to expand your knowledge and skills in this critical programming area.