In C++, the `extern template` declaration is used to explicitly suppress the instantiation of a template in a translation unit, helping to reduce compile-time and binary size when the template will be instantiated elsewhere.
Here's a code snippet demonstrating its use:
// Example of extern template usage
#include <iostream>
#include <vector>
// A simple template function
template <typename T>
void printVector(const std::vector<T>& v) {
for (const auto& element : v) {
std::cout << element << " ";
}
std::cout << std::endl;
}
// Declare extern template to suppress instantiation
extern template void printVector<int>(const std::vector<int>&);
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
printVector(vec); // This will result in a linker error without the proper instantiation elsewhere
}
In this example, the `extern template` declaration tells the compiler not to instantiate `printVector<int>` in this translation unit, assuming it will be provided in a different one.
Understanding Templates in C++
What are Templates?
Templates in C++ allow developers to create functions or classes that operate with generic types. This powerful feature helps increase code reusability and reduces code duplication. By defining a function or class template, you can write code that works with any data type, which is especially useful for designing libraries or frameworks.
For instance, consider a basic function template that prints any type of variable:
template <typename T>
void print(const T& value) {
std::cout << value << std::endl;
}
// Usage
print(42); // Prints an integer
print(3.14); // Prints a double
In this example, the `print` function can accept any type, making it versatile and reusable across different types.
Template Instantiation
When a template is used with a specific type, the compiler generates a concrete instance of the template, known as template instantiation. The instantiation can occur in several ways:
- Implicit Instantiation: This happens automatically when a template is used.
- Explicit Instantiation: This is when the programmer specifically instructs the compiler to instantiate a template with a certain type.
However, every time a template is instantiated, it can lead to code bloat if the same template is used frequently with multiple types. Understanding the implications of instantiation is crucial for optimizing compile times and binary sizes.
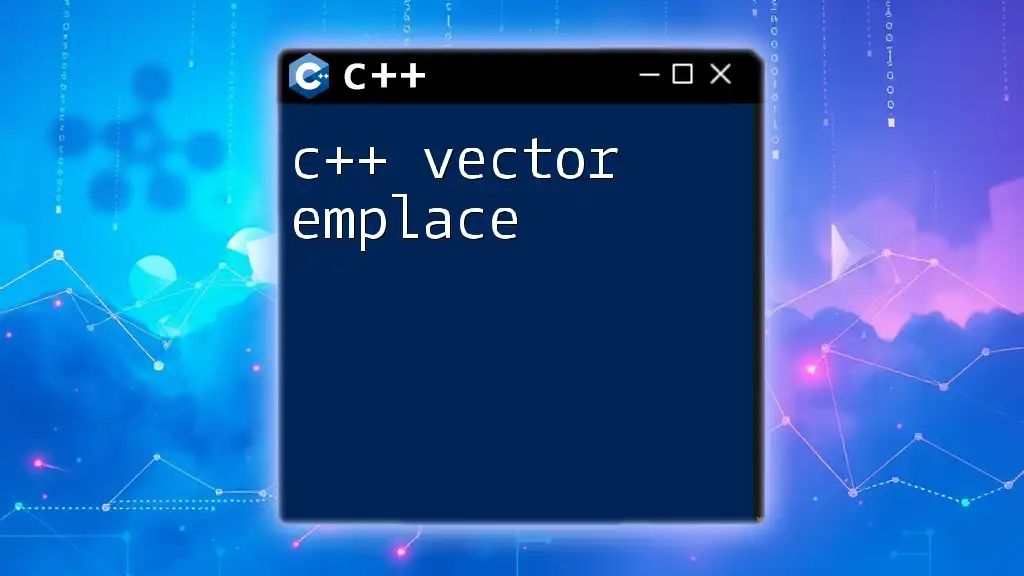
The Extern Template Keyword
What is the Extern Template?
The `extern template` keyword comes into play when you want to prevent the compiler from generating multiple instances of a template. When you declare a template as `extern`, you’re telling the compiler that it should not instantiate this template in the current translation unit; instead, it will be instantiated elsewhere.
How Extern Template Works
By default, if you include a header that defines a template class or function in multiple translation units, the compiler will instantiate them each time the header is included. This can significantly increase compilation time and binary sizes.
Consider the following code that demonstrates standard instantiation behavior:
template class MyTemplate<int>; // Compiled every time this is included
template class MyTemplate<double>; // Same here
extern template class MyTemplate<int>; // Avoids duplicate instantiation
In the code above, while the first two lines will generate new instances of `MyTemplate<int>` and `MyTemplate<double>` each time, the `extern template` declaration tells the compiler to skip instantiating `MyTemplate<int>` in this file.
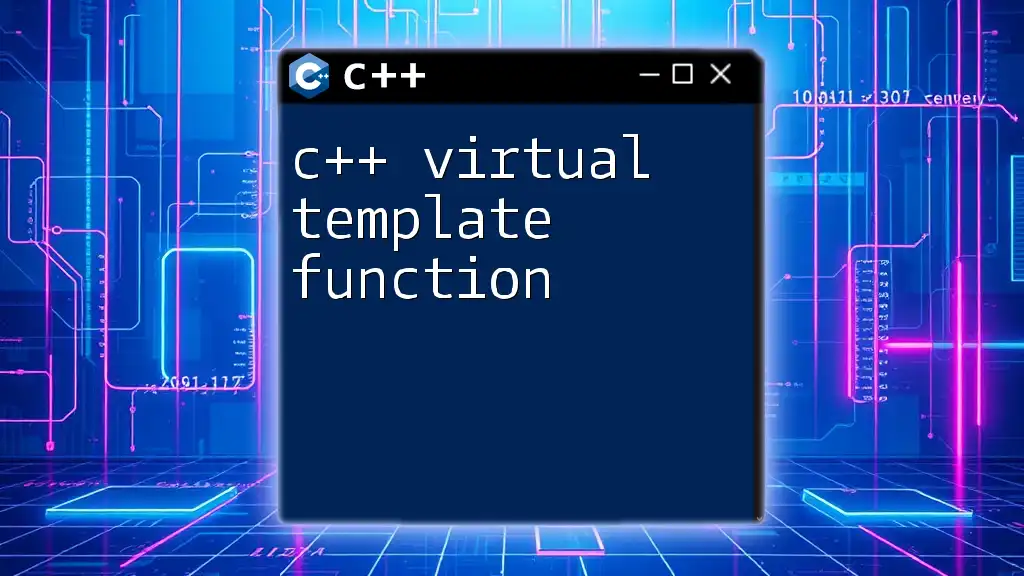
Benefits of Using Extern Templates
Improved Compilation Times
Using `extern template` can lead to marked reductions in compile times, especially for large projects. By preventing multiple instantiations, you streamline the compilation process. This is particularly advantageous in projects where templates are widely used.
Reduced Binary Sizes
Another significant benefit is the decrease in binary sizes. When templates are instantiated multiple times, they contribute to larger executable files. By using `extern template`, you can keep the binary size minimal, which enhances performance due to reduced load times and memory usage.
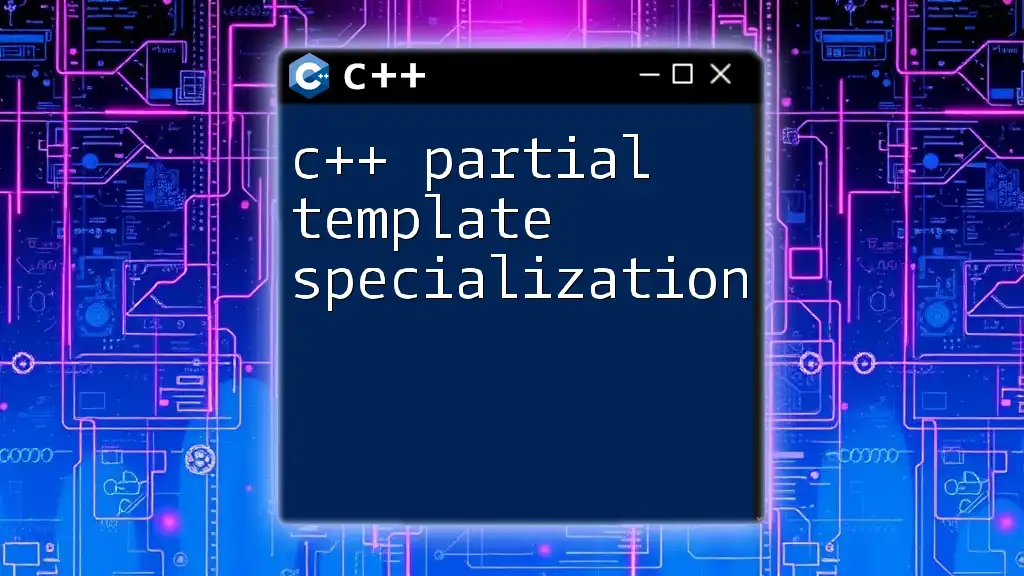
Implementing Extern Templates
Step-by-Step Implementation
To implement `extern template`, you need to follow a few straightforward steps:
- Define your template class or function in a header file.
- Provide the implementation in a corresponding source file.
- Use `extern` declarations for prototypes in other files to avoid multiple instantiations.
Code Snippet Example
Here is an example that illustrates how to effectively use the `extern template` keyword in your codebase:
// MyTemplate.h
template <typename T>
class MyTemplate {
public:
void display();
};
// MyTemplate.cpp
#include "MyTemplate.h"
#include <iostream>
template <typename T>
void MyTemplate<T>::display() {
std::cout << "Display: " << typeid(T).name() << std::endl;
}
// main.cpp
#include "MyTemplate.h"
extern template class MyTemplate<int>; // Declare extern template
int main() {
MyTemplate<int> obj; // No instantiation here
obj.display(); // Will use the existing instantiation
}
In this example, `MyTemplate<int>` will not be instantiated again in `main.cpp`, as the extern declaration informs the compiler that the instantiation already exists, thus optimizing compilation.
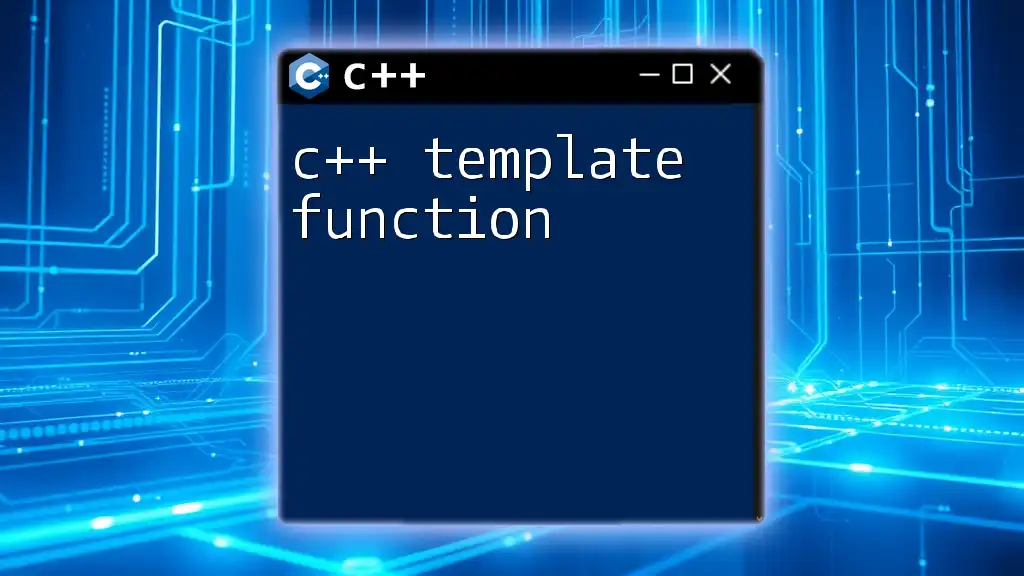
Common Pitfalls and How to Avoid Them
Incomplete Implementations
One common mistake is not providing the complete definition of a template in the appropriate file. If you declare an `extern template`, but the definition is missing in your implementation, it leads to linker errors. Always ensure that the complete template definition is available in exactly one translation unit.
Compiler-Specific Behaviors
Different compilers may interpret templates differently. While most modern compilers adhere to the C++ standard, quirks exist. It’s advisable to test your code across different compilers to ensure consistent behavior, especially when using advanced template features like `extern template`.
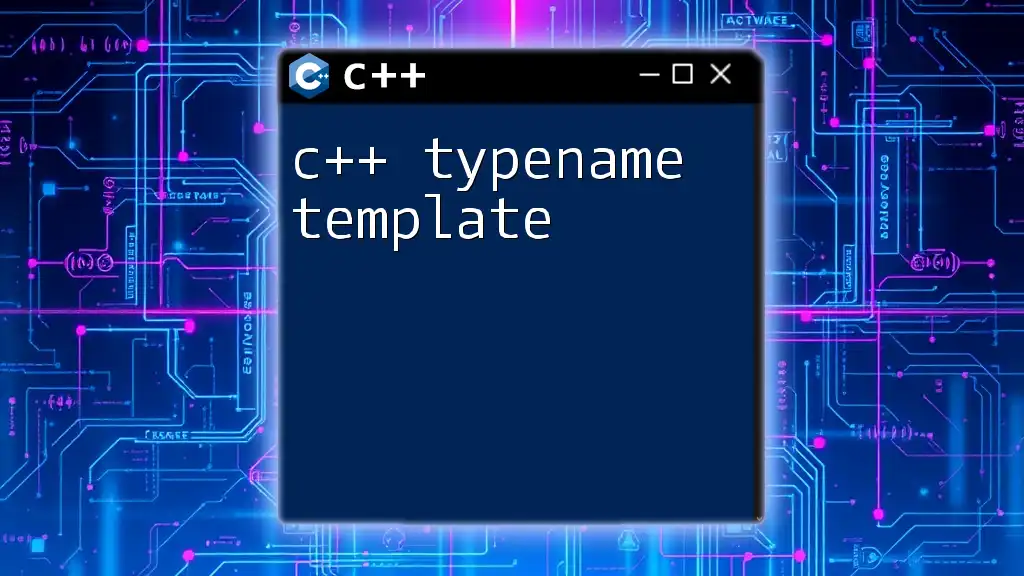
Best Practices for Using Extern Templates
When to Use Extern Templates
The `extern template` keyword should be employed judiciously. It is perfect for highly used templates that may lead to significant code bloat and for libraries where reducing compilation time is crucial. Monitor where templates are instantiated multiple times and consider using `extern template` where it makes sense.
Performance Considerations
While `extern template` reduces code bloat and compilation time, be mindful of the performance implications. Profiling your code can reveal whether the benefits of reducing instantiation outweigh the added complexity. Always aim for a balance between maintainability and performance.
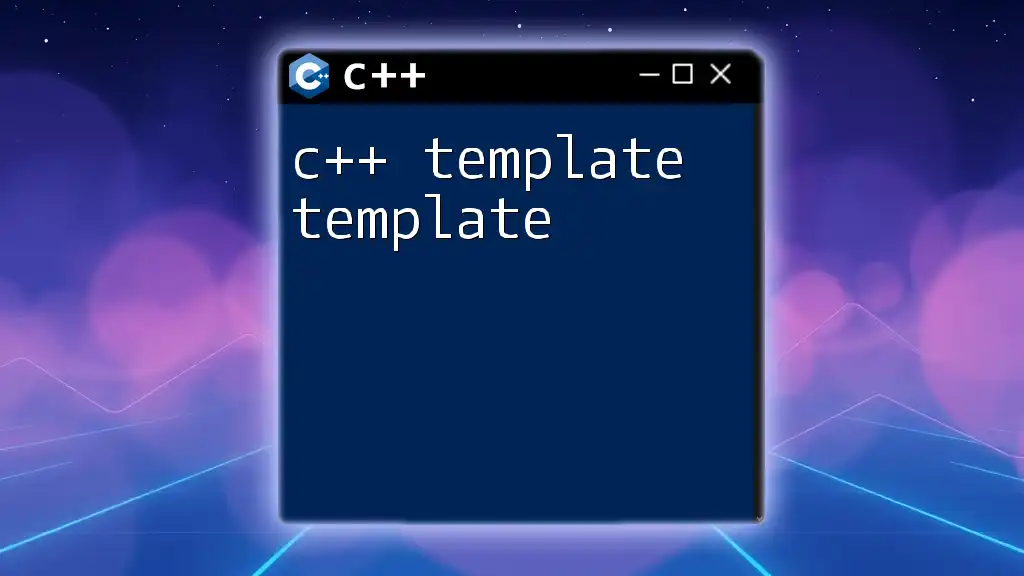
Conclusion
The C++ extern template keyword is a powerful feature that can help optimize your code’s compilation and binary size by minimizing redundant instantiations. By understanding when and how to use it effectively, you can significantly improve the performance of your C++ projects. Make sure to continually assess your use of templates, leverage tools for profiling, and stay informed on best practices to harness the full potential of C++ templates effectively.
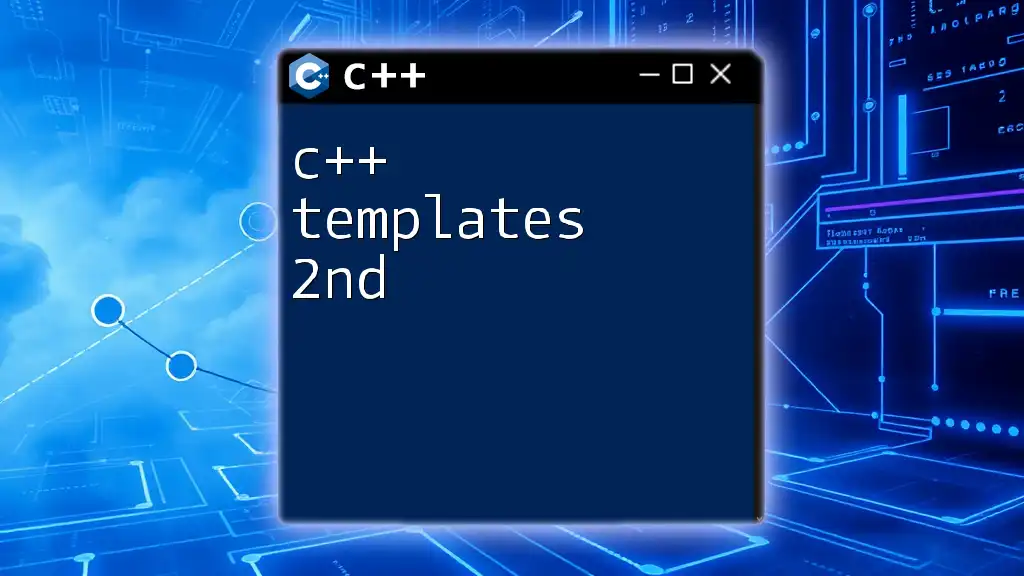
Additional Resources
For further exploration of this topic, consider consulting the official C++ documentation and authoritative texts on C++ programming that delve into templates, performance optimization, and advanced techniques. Engaging with these resources can enrich your understanding and application of the `extern template` functionality in C++.