In C++, `emplace` allows you to construct an object in place within a vector, optimizing memory usage and improving performance by avoiding unnecessary copies.
Here's an example code snippet demonstrating the use of `emplace` with a `std::vector`:
#include <vector>
#include <iostream>
struct Point {
int x, y;
Point(int x, int y) : x(x), y(y) {}
};
int main() {
std::vector<Point> points;
points.emplace_back(1, 2); // Constructs Point(1, 2) in the vector
points.emplace_back(3, 4); // Constructs Point(3, 4) in the vector
for (const auto& point : points) {
std::cout << "Point(" << point.x << ", " << point.y << ")\n";
}
return 0;
}
What is a C++ Vector?
A C++ vector is a sequence container that encapsulates dynamic size arrays. Vectors are part of the Standard Template Library (STL) and offer several advantages over traditional arrays:
- Dynamic Sizing: Vectors can grow and shrink in size automatically, making them more flexible.
- Ease of Use: They provide a rich set of member functions to manipulate the underlying array.
- Performance: Generally faster than linked lists for accessing elements since vectors are stored in contiguous memory.
A C++ vector manages dynamic memory automatically and handles the resizing process internally. When the size of a vector exceeds its current capacity, it automatically allocates a new larger block of memory, copies the elements, and deallocates the old memory block.
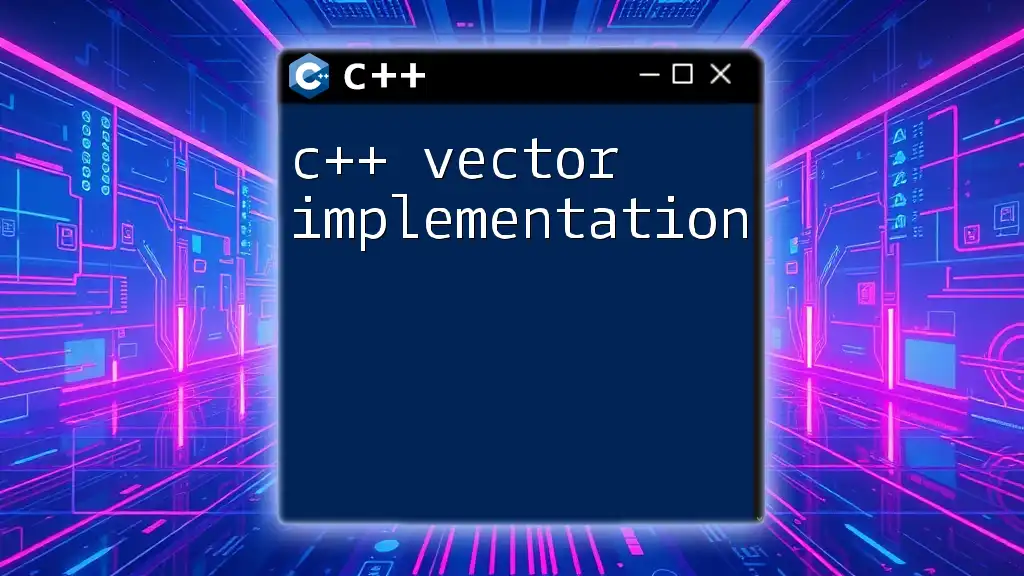
The Emplace Family of Functions
Emplace vs. Push_Back: What's the Difference?
Both `emplace` and `push_back` are used to add elements to a vector, but they operate differently:
-
push_back: This function modifies an object before storing it in the vector. The element is copied (or moved) into the vector, which may involve additional overhead, especially if the class has expensive copy constructors.
-
emplace: It constructs the object in place, which means no temporary object is created. This can lead to improved performance, especially for complex objects.
When to Use Emplace
Use `emplace` when you want to:
- Minimize overhead: Using `emplace` avoids unnecessary copies or moves.
- Construct complex objects: It allows for passing constructor arguments directly to the object that is being added.
In scenarios where object construction is costly or you're dealing with many objects, `emplace` can significantly improve performance.
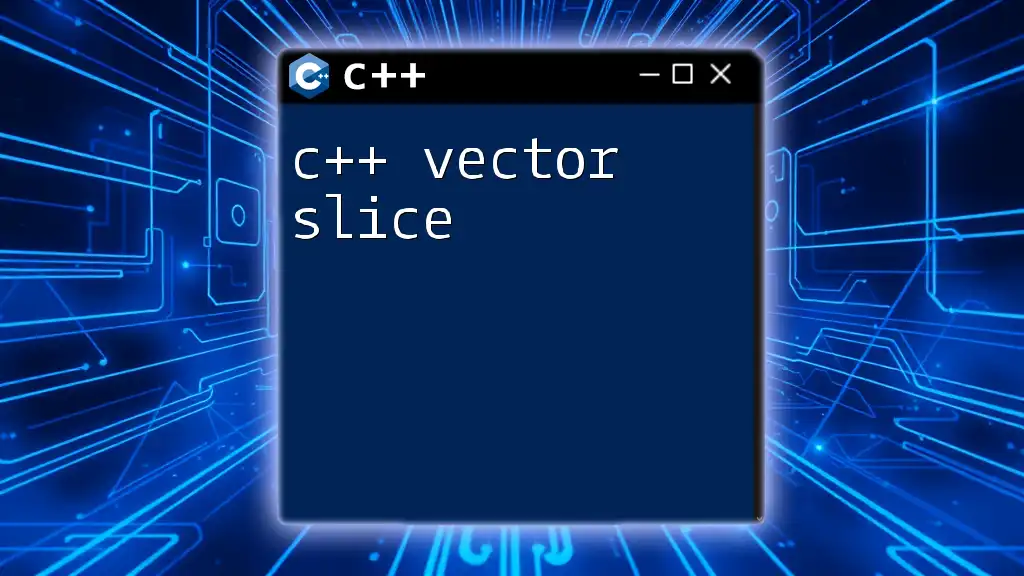
Deep Dive Into C++ Vector Emplace
Using emplace_back in Vectors
The `emplace_back` method constructs an element in place at the end of a vector. The syntax is straightforward, allowing you to pass the constructor arguments directly.
Example of emplace_back
#include <iostream>
#include <vector>
class Person {
public:
Person(const std::string& name, int age) : name(name), age(age) {}
void display() const { std::cout << name << ", " << age << std::endl; }
private:
std::string name;
int age;
};
int main() {
std::vector<Person> people;
people.emplace_back("John Doe", 30); // Creates an object in place
for (const auto& person : people) {
person.display(); // Output: John Doe, 30
}
return 0;
}
In this example, the `Person` object is created directly in the vector's memory, resulting in efficient use of both time and space.
Emplace vs. Alternative Approaches
Performance Benchmarking
When comparing `emplace_back` with `push_back`, testing performance with a complex object can highlight the advantages.
#include <vector>
#include <iostream>
#include <chrono>
class LargeObject {
public:
LargeObject(int value) : value(value) {}
// A typical expensive operation
int value;
};
// Measure performance
void test_push_back() {
std::vector<LargeObject> vec;
for (int i = 0; i < 100000; ++i) {
vec.push_back(LargeObject(i)); // Temporary object created
}
}
void test_emplace_back() {
std::vector<LargeObject> vec;
for (int i = 0; i < 100000; ++i) {
vec.emplace_back(i); // Constructed in place
}
}
While the `push_back` method requires an object to be created and then copied into the vector, `emplace_back` constructs the object directly in the storage allocated by the vector.
Best Practices for Using emplace Back
To utilize `emplace_back` effectively:
- Favor it over `push_back` when working with objects that are expensive to copy.
- Use it when you need to pass multiple constructor parameters, which can lead to cleaner code.
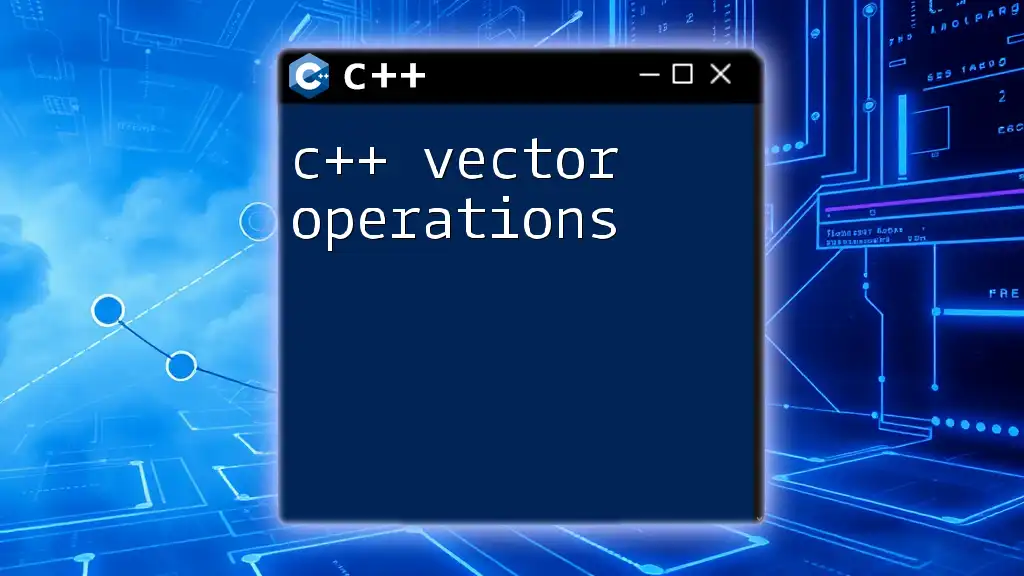
Other Emplace Methods in C++
Emplace in Context
While `emplace_back` is primarily used with vectors, it's important to understand its utility in other containers as well.
Emplacing Elements in Other Containers
Containers such as `std::map` and `std::set` provide `emplace` and `emplace_hint` methods, which allow you to insert values or keys directly, avoiding unnecessary copies.
Differences Between Emplace and Insert
-
Insert: Generally involves copying an object into the container, which can be expensive.
-
Emplace: Constructs the object in the container directly, yielding better performance.
Knowing when to use `emplace` over `insert` can be particularly useful for improving efficiency in performance-critical applications.
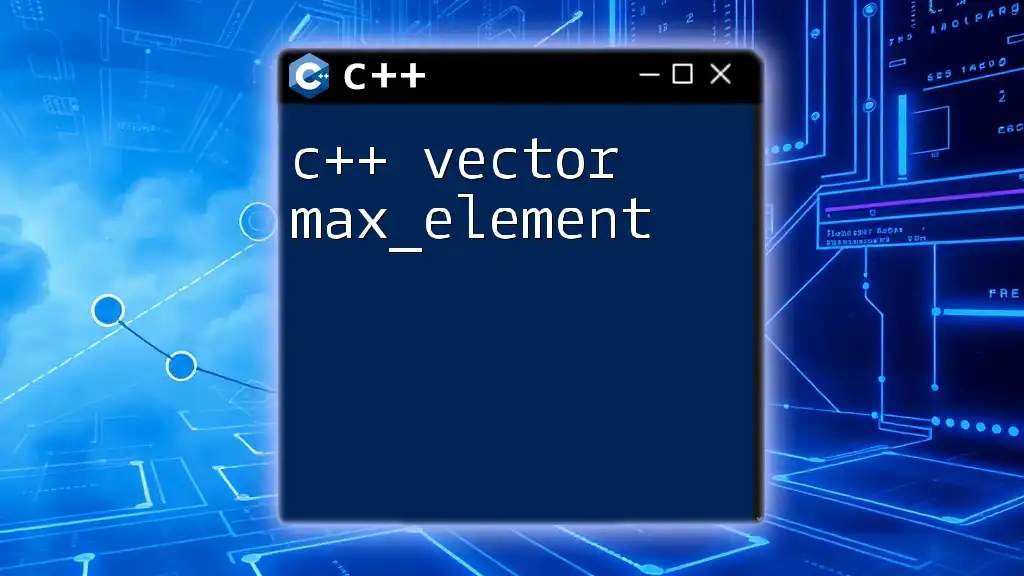
Common Pitfalls with Emplace
Misunderstandings and Errors
While `emplace` offers numerous benefits, there are potential pitfalls:
- Incorrectly passing parameters can lead to compilation errors or unexpected behavior.
- Using emplace with types that are not move-constructible can lead to issues.
Guidelines to Avoid Issues
- Provide necessary constructor arguments: Always ensure that the arguments you provide to `emplace` match those expected by the constructor.
- Test thoroughly: Conduct performance tests to validate your use of `emplace` in production scenarios.
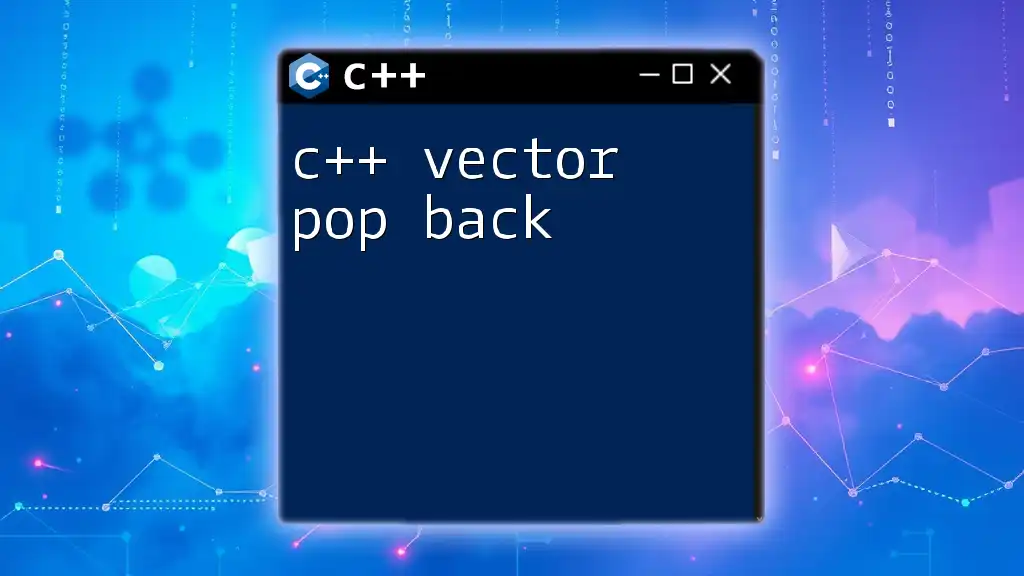
Conclusion
In summary, C++ vector emplace offers a powerful tool for efficient memory and performance optimization when working with C++ vectors. Emplace methods, particularly `emplace_back`, allow for seamless construction and insertion of complex objects, significantly improving the efficiency of your code. Understanding the differences between emplace and traditional methods ensures you leverage the full power of C++ vectors in your applications.
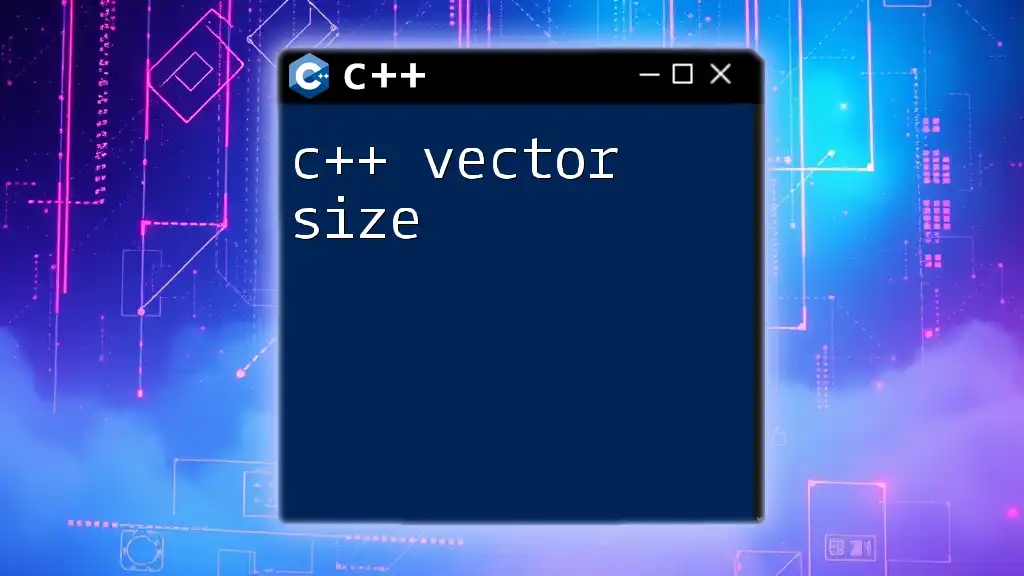
Additional Resources
Recommended Books and Online Courses
To deepen your knowledge of C++, consider exploring books like "Effective Modern C++" by Scott Meyers or online courses such as those found on platforms like Coursera and Udemy that focus specifically on STL and advanced C++ concepts.
Community and Support
Engaging with online communities and forums such as Stack Overflow, Reddit, or C++ user groups can provide you with additional support and insights as you continue your journey in mastering C++.