In C++, a vector slice allows you to create a subset of a vector by specifying a range of indices, enabling easy manipulation and access to a portion of the vector's elements.
Here's an example of slicing a vector:
#include <iostream>
#include <vector>
std::vector<int> slice(const std::vector<int>& vec, int start, int end) {
return std::vector<int>(vec.begin() + start, vec.begin() + end);
}
int main() {
std::vector<int> original = {1, 2, 3, 4, 5, 6, 7};
std::vector<int> sliced = slice(original, 2, 5); // Slices elements 3 to 5
for (int num : sliced) {
std::cout << num << " "; // Output: 3 4 5
}
return 0;
}
Understanding C++ Vectors
What is a C++ Vector?
A C++ vector is a part of the Standard Template Library (STL) and offers a dynamic array implementation. Unlike regular arrays, vectors can grow or shrink in size, making them a versatile choice for a variety of programming tasks. Key characteristics of C++ vectors include:
- Dynamic Sizing: Vectors can automatically adjust their size as elements are added or removed, providing flexibility compared to static arrays.
- Random Access: You can access elements through an index, making data retrieval efficient.
- Memory Management: The STL handles memory allocation and deallocation, allowing developers to focus on application logic instead of low-level tasks.
Basic Operations with Vectors
To effectively work with C++ vectors, it is crucial to understand some basic operations:
-
Initializing a Vector: Vectors can be initialized in several ways, including:
std::vector<int> vec1; // Empty vector std::vector<int> vec2(5, 10); // Vector of size 5, with all elements initialized to 10
-
Adding and Removing Elements: You can use functions like `push_back()`, `pop_back()`, and `erase()` to manage vector contents:
vec1.push_back(20); // Adds 20 to the end of vec1 vec1.pop_back(); // Removes the last element
-
Accessing Vector Elements: Elements can be accessed using the standard indexing method:
int value = vec1[0]; // Accesses the first element
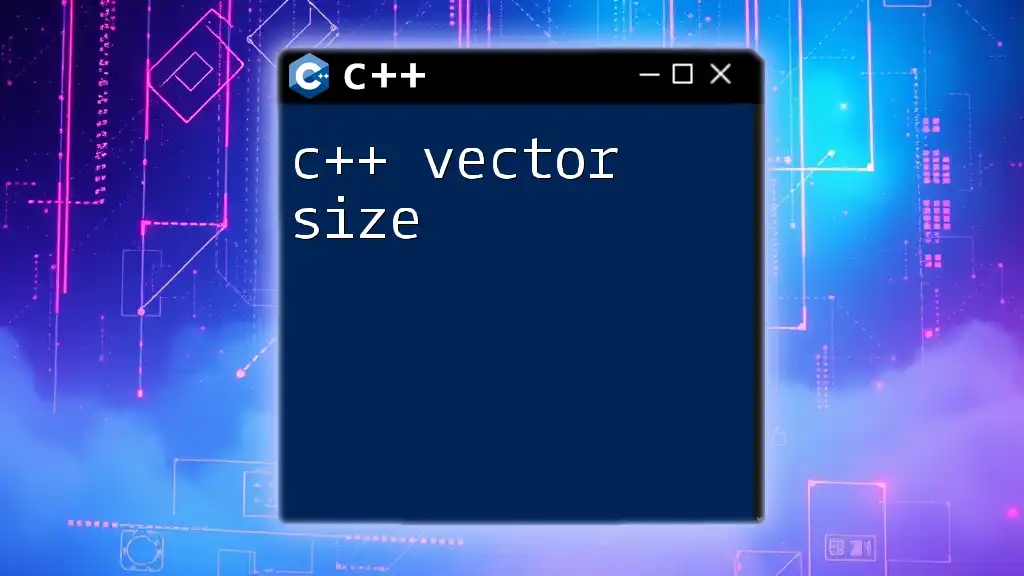
What is Vector Slicing?
Definition of Vector Slicing
Vector slicing is the process of creating a sub-vector from an existing vector. This technique allows you to extract a portion of the vector's contents, facilitating focused operations on specific data segments. Unlike traditional slicing found in dynamic languages like Python, C++ requires manual implementation of this functionality, thus enhancing comprehension of memory management.
Scenarios for Using Vector Slicing
Vector slicing becomes particularly useful in various scenarios:
- Data Analysis Use Cases: When working with large datasets, slicing allows you to analyze subsets without the overhead of copying entire vectors.
- Benefits in Algorithm Development: Many algorithms benefit from operating on sub-vectors, especially when implementing operations like search and sort on specific data portions.
- Techniques for Handling Large Datasets: Slicing can help reduce memory consumption and increase efficiency by working with smaller chunks of data.
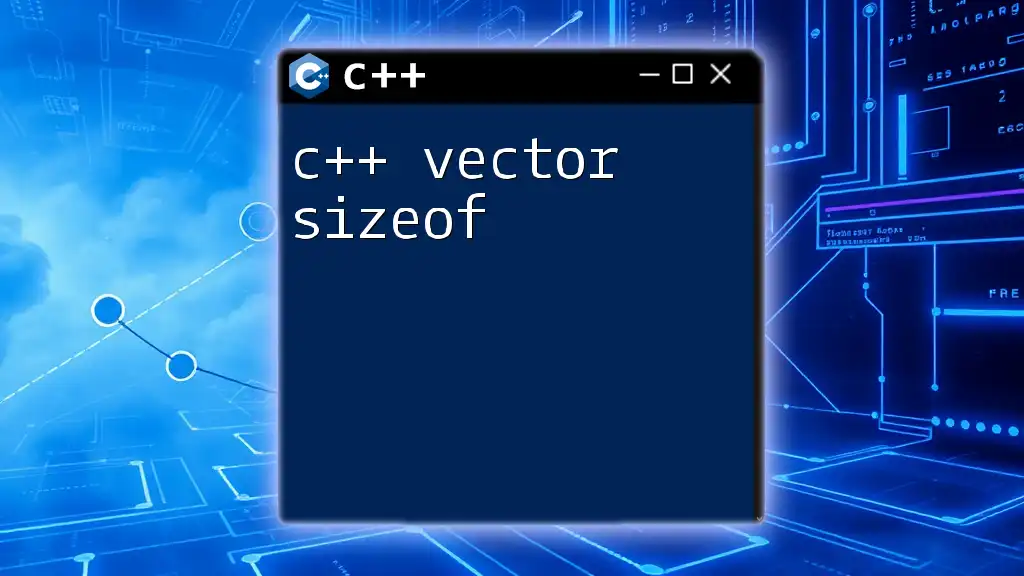
Implementing Vector Slicing in C++
Creating a Function for Slicing
To slice a vector in C++, you need to create a function that takes the original vector and the desired start and end indices as parameters. A basic implementation looks like this:
#include <iostream>
#include <vector>
std::vector<int> sliceVector(const std::vector<int>& vec, int start, int end) {
if (start < 0 || end > vec.size() || start >= end) {
throw std::out_of_range("Invalid slice range");
}
return std::vector<int>(vec.begin() + start, vec.begin() + end);
}
Explained Code
In the `sliceVector` function:
- Input Validation: The function checks for valid start and end indices to prevent accessing out-of-bounds elements. If the indices are invalid, it throws an `out_of_range` exception, providing a strong guard against potential errors.
- Slicing Logic: The function constructs a new vector from a range defined by the specified start and end indices, leveraging the functionality of iterators to navigate through the original vector.
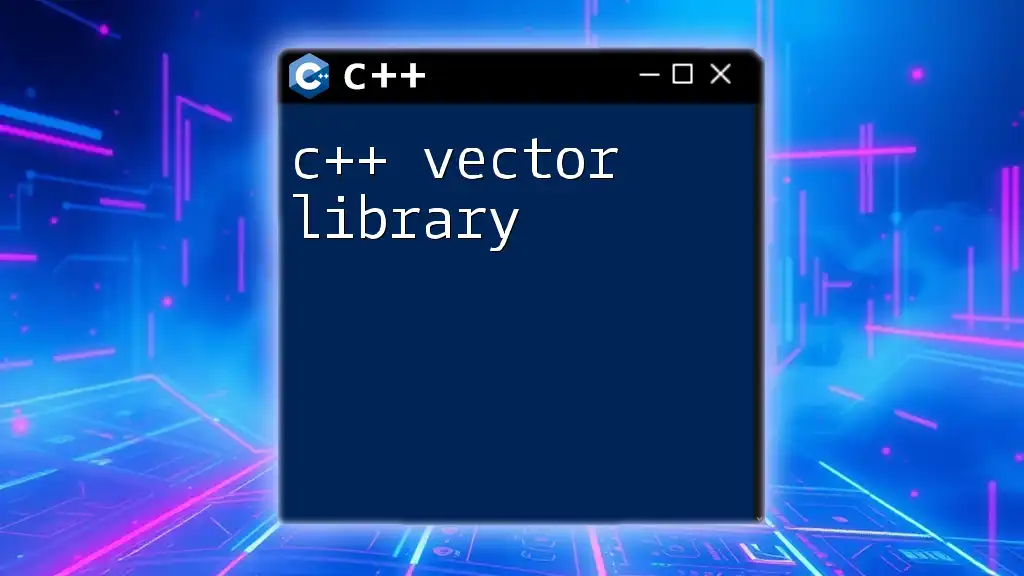
Practical Examples of Vector Slicing
Example 1: Basic Usage of Slice Function
To understand how the slice function works, let’s consider an example with a sample vector:
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5, 6, 7};
std::vector<int> sliced = sliceVector(myVector, 2, 5);
for (int num : sliced) {
std::cout << num << " "; // Output: 3 4 5
}
return 0;
}
In this example, the `sliceVector` function extracts values from indices 2 to 4, resulting in a new vector containing the elements 3, 4, and 5.
Example 2: Handling Edge Cases
When working with vectors, exceptional situations may arise. It’s crucial to handle these cases to avoid crashes:
try {
std::vector<int> invalidSlice = sliceVector(myVector, 5, 10); // Out of range
} catch (const std::out_of_range& e) {
std::cerr << e.what() << '\n'; // Output: Invalid slice range
}
In this code snippet, attempting to slice beyond the existing indices leads to an exception, which is properly caught and logged.
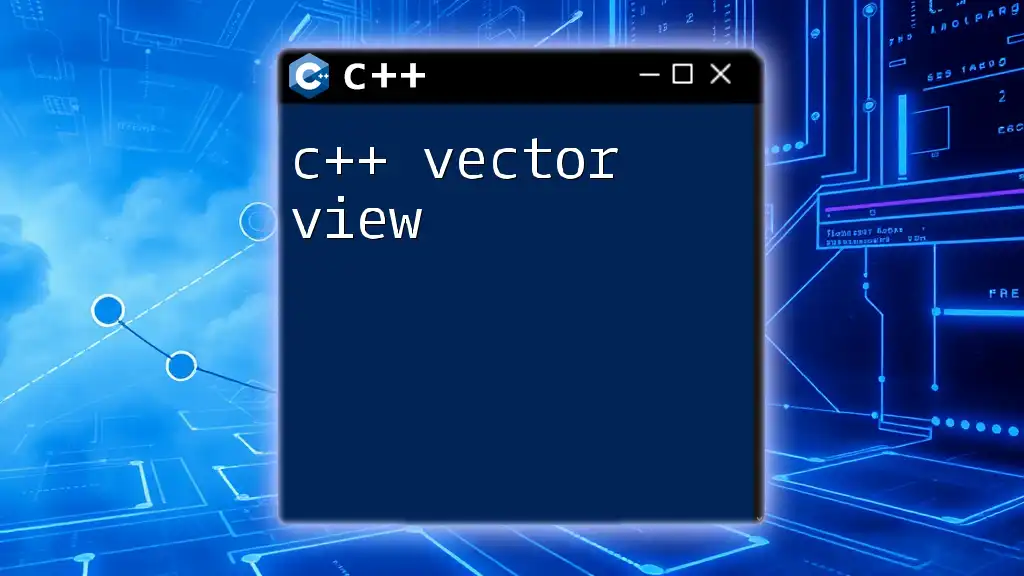
Advanced Slicing Techniques
Using Iterators for Slicing
To achieve more flexibility, you can implement the slice function using iterators:
std::vector<int> sliceVectorUsingIterators(const std::vector<int>& vec, size_t start, size_t end) {
return std::vector<int>(vec.begin() + start, vec.begin() + end);
}
Using iterators in this manner offers more control over how you access elements within the vector, making it easier to handle various data types.
Multi-dimensional Vector Slicing
Working with multi-dimensional vectors, such as matrices, involves slightly different techniques. You can extract slices from nested vectors:
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
std::vector<int> flattenSlice = sliceVector(matrix[1], 0, 2); // Slicing 4 and 5
In this example, you slice the second row of a matrix, demonstrating the versatility of slicing across different data structures.
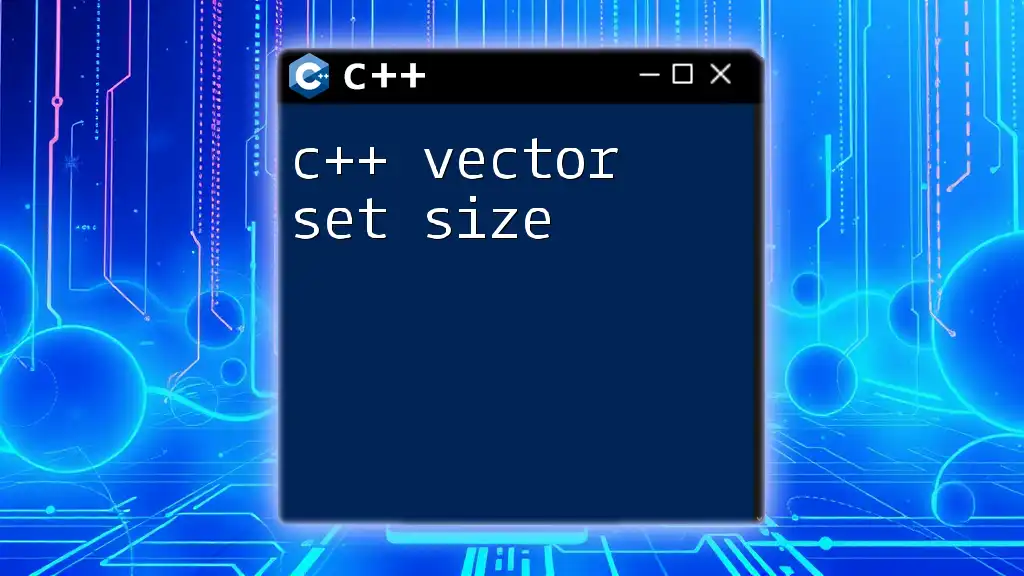
Common Pitfalls and Best Practices
Potential Pitfalls
While working with vector slicing, it's essential to be aware of common pitfalls:
- Out of Range Errors: Always ensure your indices fall within the vector's bounds, as invalid accesses can lead to runtime errors.
- Undefined Behavior: Failing to handle exceptions may result in crashes or unexpected behavior in your application.
Best Practices for Vector Slicing
To ensure safe and effective slicing, follow these best practices:
- Implement Input Validation: Always validate the start and end parameters before slicing to maintain program stability.
- Use C++ Exception Handling: Implement try-catch blocks to gracefully handle errors arising from invalid operations.
- Limit Scope of Sliced Vectors: When slicing, consider creating a limited-scope vector to minimize memory usage and enhance performance.
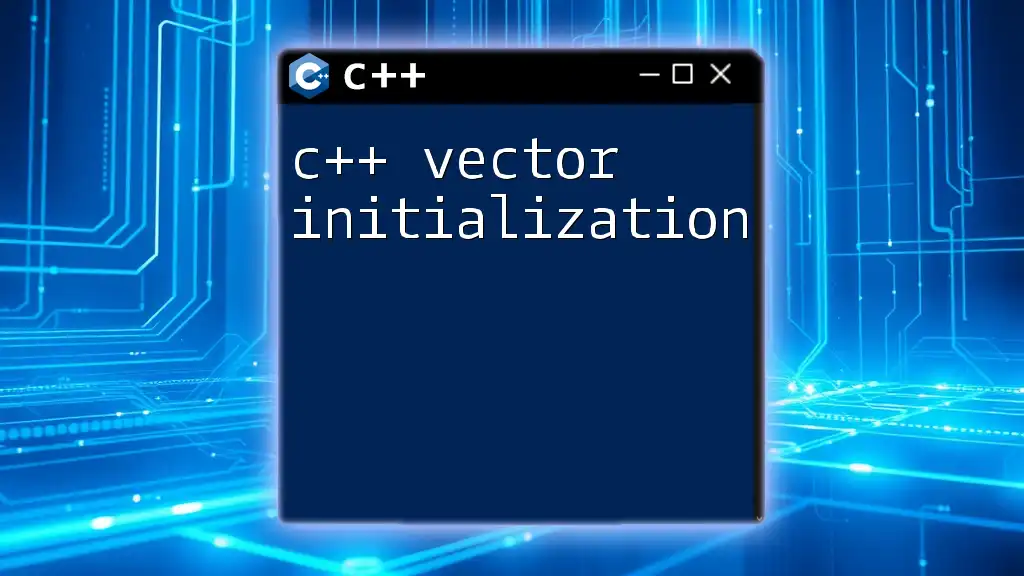
Conclusion
In summary, mastering the C++ vector slice concept is crucial for effective data manipulation within the C++ programming environment. By understanding how to implement and utilize vector slicing, you can simplify data handling, improve program efficiency, and foster better coding practices. Embrace these techniques, experiment in your projects, and continue exploring the powerful capabilities of C++ vectors.
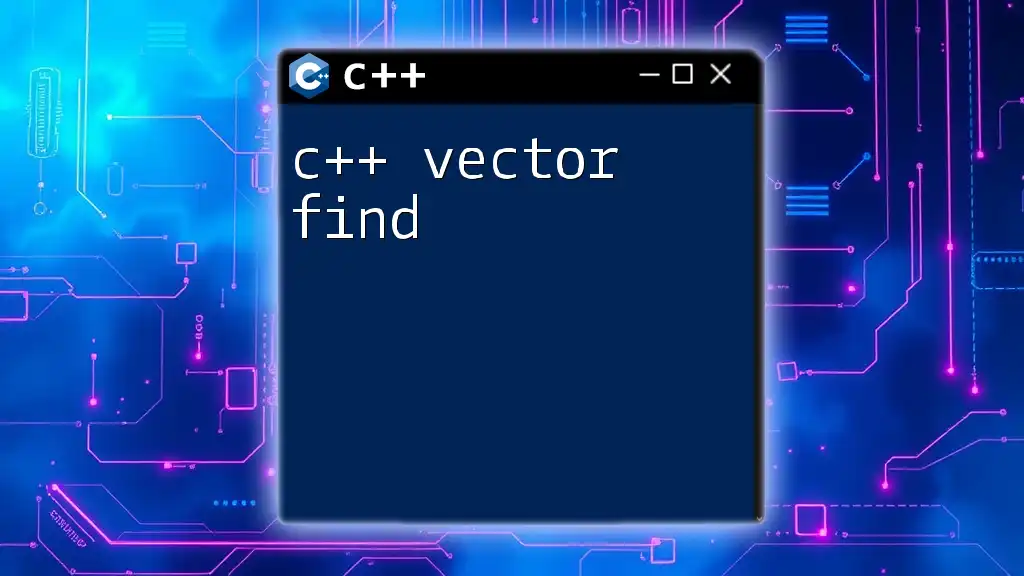
Additional Resources
Don't hesitate to explore further resources and tutorials to deepen your understanding of C++ vectors and slicing. Online platforms and community forums offer great opportunities for learning and sharing knowledge about C++.