In C++, you can set the size of a vector using the `resize` function, which adjusts the container to contain the specified number of elements.
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec;
vec.resize(5); // Resizes the vector to contain 5 elements
std::cout << "Size of vector: " << vec.size() << std::endl; // Outputs: Size of vector: 5
return 0;
}
Understanding C++ Vectors
What is a Vector in C++?
C++ vectors are dynamic arrays that can change size automatically as elements are added or removed. Unlike traditional arrays, which have a fixed size, vectors are part of the Standard Template Library (STL) and provide a flexible way to manage collections of data in a program.
Vectors are important because they simplify many programming tasks, allowing developers to manage sequences of elements without worrying about memory management and array limits. Compared to arrays, vectors offer numerous advantages, including:
- Dynamic sizing: Automatically grow and shrink as needed.
- Easier memory management: Vectors handle memory allocation behind the scenes.
- Built-in functionalities: Provide various member functions like `push_back()`, `pop_back()`, and `resize()`.
Basic Properties of Vectors
Vectors possess unique properties that make them an excellent choice for data handling in C++:
- Dynamic Sizing: Unlike arrays, vectors can grow or shrink their size dynamically based on the data being processed.
- Memory Management: The C++ engine automatically manages memory allocation, reducing the burden on developers.
- Automatic Resizing: If you attempt to add an element beyond its current size, vectors automatically reallocate memory to accommodate new elements.
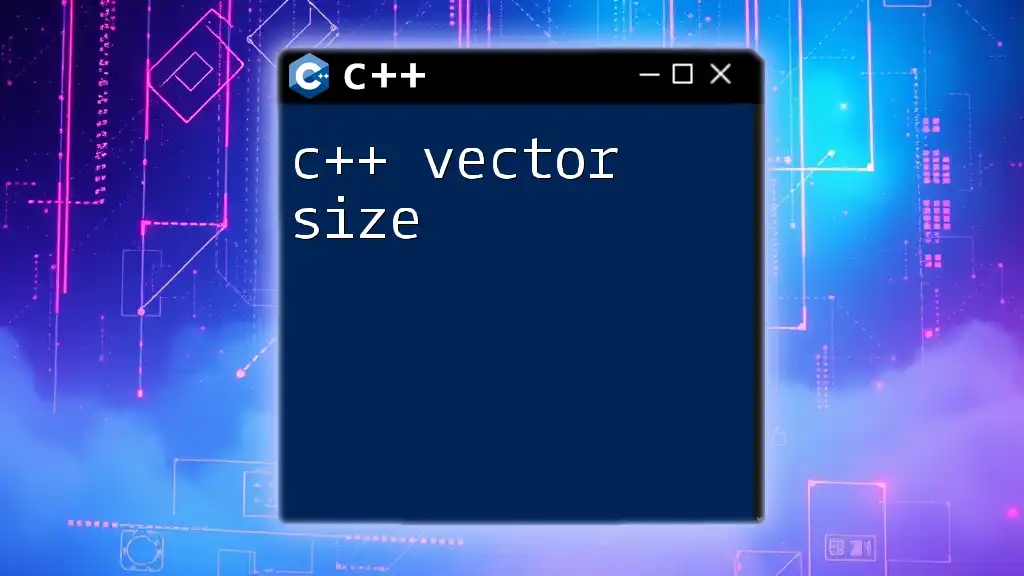
Setting the Size of a Vector in C++
Why Set the Size of a Vector?
Setting the size of a vector is essential for optimal performance in various scenarios. Here are some reasons to set the size:
- Use Cases: Knowing the expected number of elements upfront allows for more efficient memory use.
- Performance Considerations: Setting a vector size beforehand can help reduce the number of memory reallocations that occur when elements are dynamically added.
- Pre-Allocating Memory: By explicitly setting a vector size, you prevent unnecessary overhead and can optimize memory access patterns in your applications.
Syntax for Setting the Vector Size
To set the size of a vector in C++, you primarily use the `resize()` member function. The basic syntax is as follows:
std::vector<int> myVector;
myVector.resize(10);
This command initializes `myVector` to have a size of 10. Any newly allocated elements will be initialized with the default value for the specified type.
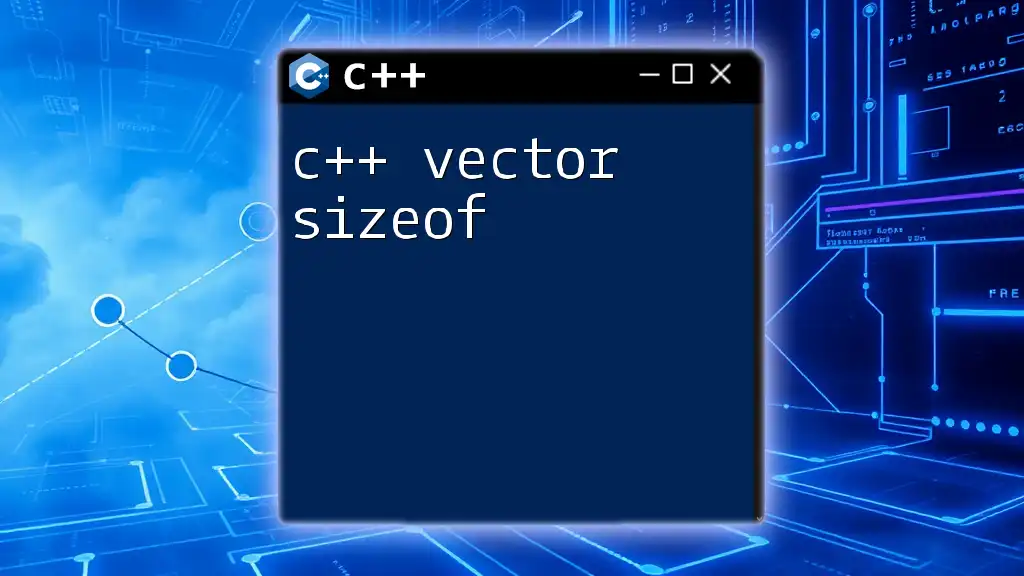
How to Set Vector Size in C++
Using `resize()`
The `resize()` function is crucial for setting the size of a vector. This function allows you to specify a new size for the vector and can also initialize newly added elements with a specified value.
For example:
std::vector<int> myVector;
myVector.resize(10, 1); // Fills with 1
In this snippet, `myVector` is resized to 10 elements, with each new element initialized to `1`. This feature is particularly useful when you want to ensure that all elements have a known initial value.
Using `reserve()`
While `resize()` changes the actual size of the vector, the `reserve()` function is used to request memory allocation without altering the size. This provides a way to avoid costly reallocations if the final size is known in advance:
std::vector<int> myVector;
myVector.reserve(10); // Allocates space for 10 elements
In this case, `myVector` has its reserved capacity increased to 10, but its size remains 0 until elements are added. This distinction is vital when you're concerned about performance and memory efficiency.
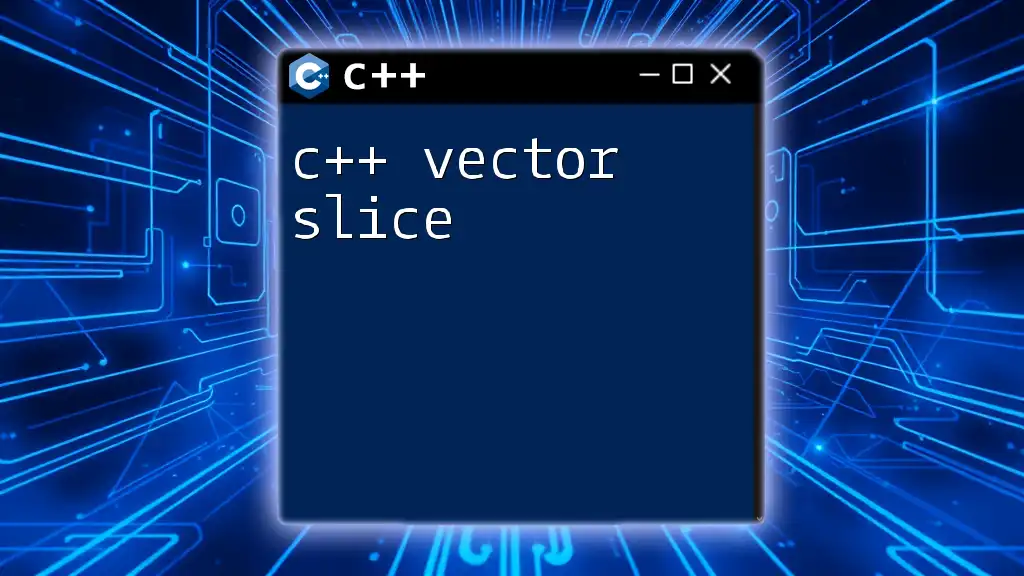
Modifying the Size of a Vector
Increasing Vector Size
To increase the size of a vector, you can utilize the `resize()` method:
std::vector<int> myVector = {1, 2, 3};
myVector.resize(5); // New size is 5, additional elements will be default initialized
In the above example, `myVector` originally contains three elements. After resizing it to five, the newly added elements will be default initialized, in this case, set to `0` (the default value for integers).
Decreasing Vector Size
Similarly, you can also decrease the vector size using `resize()`:
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.resize(3); // New size is 3, truncating elements
In this example, only the first three elements are retained, and the remaining elements are effectively removed, demonstrating how `resize()` can truncate the vector.
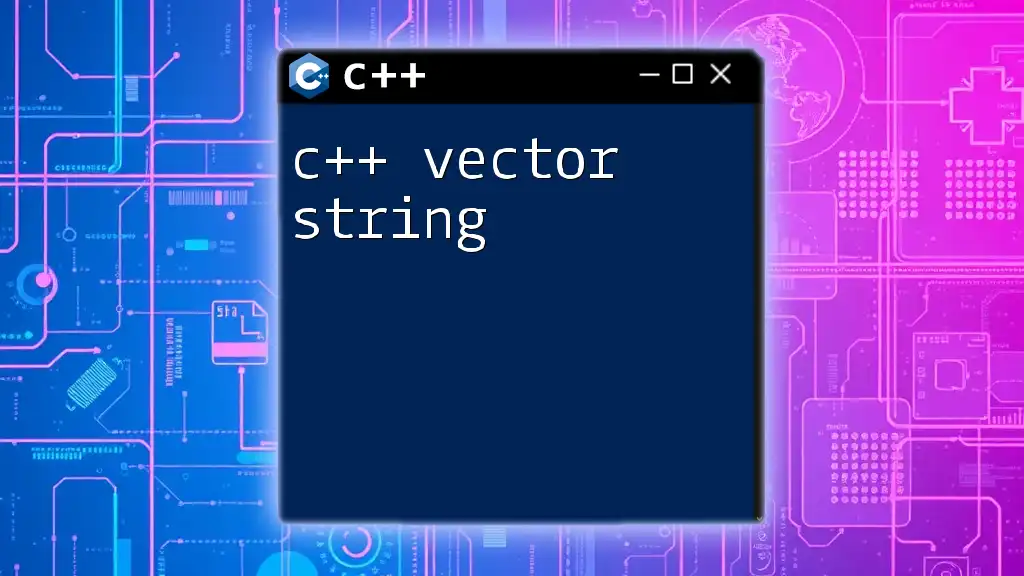
Best Practices for Setting Vector Size
When to Use `resize()` vs. `reserve()`
Understanding when to use `resize()` and `reserve()` is crucial for efficient memory management. Typically:
- Use `resize()` when you need to change the vector's size and want to assign new values to the new elements.
- Use `reserve()` when you know the final size but want to avoid overhead from resizing and reallocating memory multiple times.
Avoiding Common Pitfalls
One common mistake is failing to set the size correctly, leading to uninitialized values in the vector. Always be cautious when increasing size; if you don’t specify a value, newly added elements may contain garbage values.
Understanding memory allocations is also vital. When a vector exceeds its reserved capacity, it reallocates, which may lead to performance issues if not managed carefully.
Performance Tips
To ensure optimal performance, consider these tips:
- Always anticipate your data size and preallocate memory using `reserve()` when applicable.
- Refrain from unnecessary calls to `resize()` if you're just adding elements; use `push_back()` instead.
- When resizing, prefer initializing elements with a value when it makes sense in your application context.
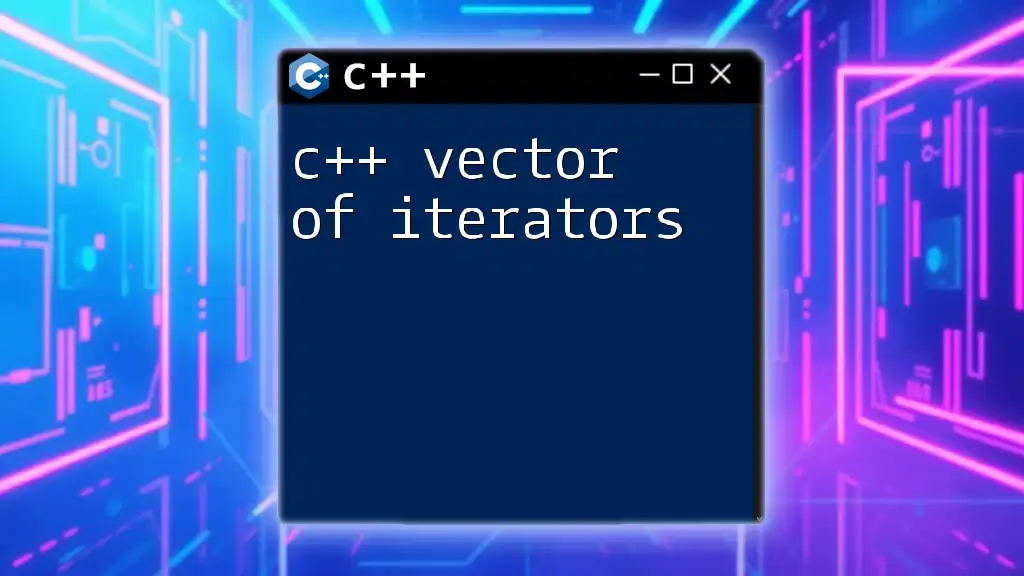
Conclusion
Recap of Key Points
Throughout this guide, we explored the significance of setting the size of a vector in C++. We discussed the different approaches available, including `resize()` and `reserve()`, along with practical examples to illustrate their use.
Encouragement for Further Learning
For those eager to learn more about vectors in C++, numerous resources are available online that delve deeper into STL components. Exploring more about iterators, algorithms, and other container types will broaden your understanding of C++ programming.
Call to Action
We encourage you to put your knowledge to the test with practical coding exercises. Engage in projects that require dynamic data handling to gain experience with C++ vectors and discover their full potential.