C++ vector view is a lightweight, non-owning interface that provides a view over a sequence of elements in a `std::vector`, allowing efficient iteration and manipulation without copying the data.
#include <vector>
#include <iostream>
#include <ranges>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto view = vec | std::views::filter([](int x) { return x % 2 == 0; });
for (int x : view) {
std::cout << x << " "; // Outputs: 2 4
}
}
Understanding Vectors
What is a Vector?
A vector in C++ is a dynamic array provided by the Standard Template Library (STL). Unlike traditional arrays, vectors can resize themselves automatically when elements are added or removed. This flexibility makes vectors a preferable choice for many applications where the size of the data set isn’t known upfront.
Vectors hold elements in contiguous memory. Consequently, they perform well in terms of access time, similar to arrays. Additionally, vectors provide various built-in functions for manipulation, making data management easier.
Benefits of Using Vectors
- Dynamic Size Adjustment: Vectors automatically expand or contract as elements are added or removed, which minimizes the need for manual memory management.
- Automatic Memory Management: The C++ Standard Library handles the allocation and deallocation of memory for vectors, reducing the chances of memory leaks or buffer overflows.
- Rich Set of Member Functions: Vectors come with numerous member functions such as `push_back()`, `pop_back()`, `insert()`, and `erase()`, which simplify data manipulation.
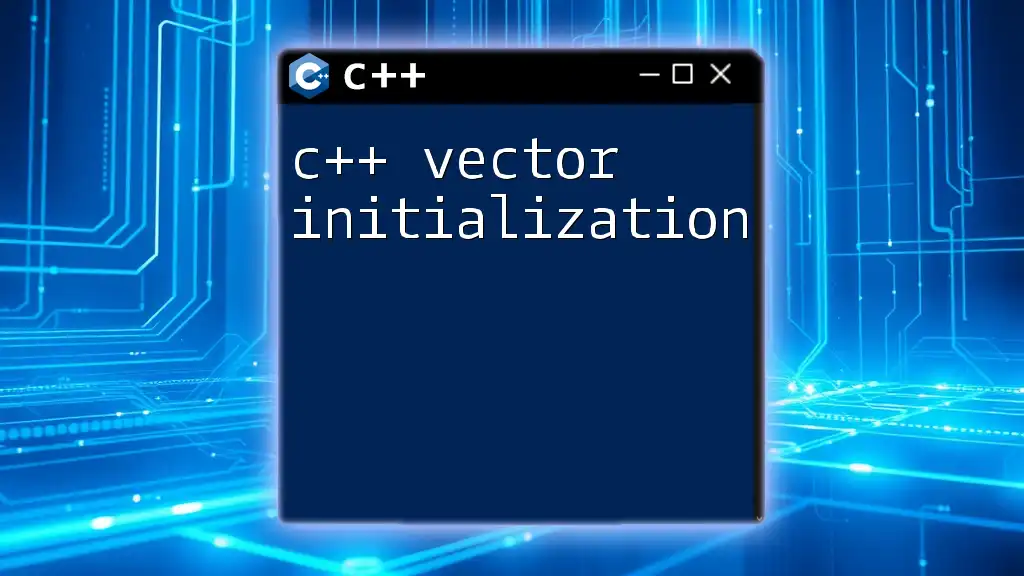
Introduction to Views
What are Views in C++?
Views allow programmers to interact with a subset of data from collections without creating copies. This means that computations can be performed lazily; elements are only processed when specifically accessed, improving performance and reducing memory usage in cases where full datasets aren't necessary.
Benefits of Using Views
- Enhanced Performance: By working with views, you can manipulate large datasets without incurring the cost of multiple memory allocations and copies. This is particularly beneficial when dealing with extensive ranges or containers.
- Memory Efficiency: Views do not duplicate data, leading to lower memory overhead.
- Simplified Code: Views can lead to more concise and readable code. Their API is cleaner, ensuring that developers can write expressive code without unnecessary complexity.
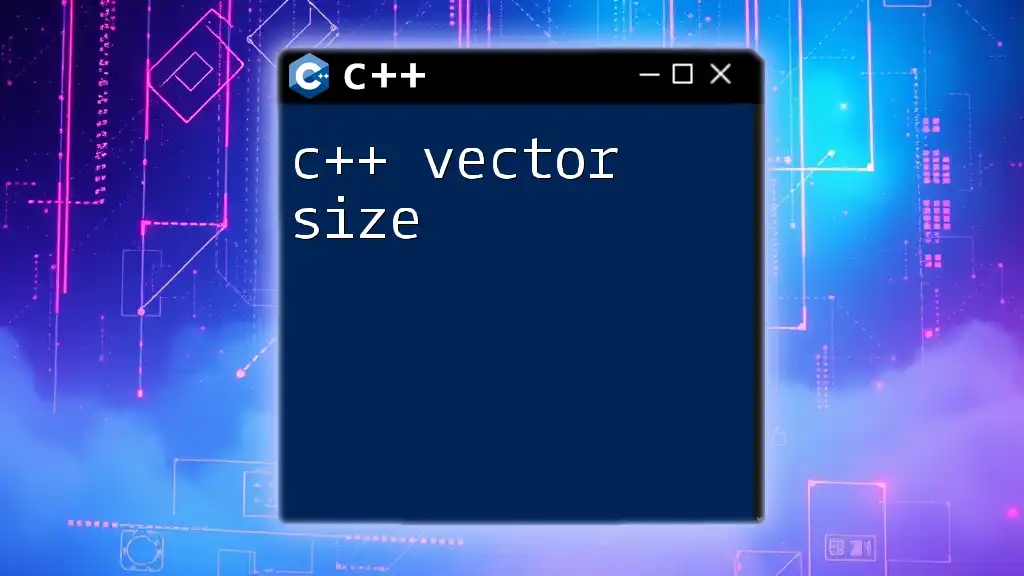
Introducing C++ Vector Views
What is a Vector View?
A vector view is essentially a lightweight, read-only wrapper around the original vector. While views do not create new containers, they allow access to specified parts of a vector, facilitating operations without consuming additional memory.
Key Features of Vector Views
- Subsequence Extraction Without Data Copying: Vector views can reference specific sections of a vector, allowing you to operate on them without making a copy.
- Read-Only vs. Read-Write Views: While many views are read-only, there are possibilities for creating modifiable views, adding flexibility in usage.
- Example Code to Demonstrate View Creation:
#include <vector>
#include <ranges>
#include <iostream>
int main() {
std::vector<int> vec{1, 2, 3, 4, 5};
auto view = std::views::take(vec, 3);
for (const auto& elem : view) {
std::cout << elem << " "; // Outputs: 1 2 3
}
}
In this example, a vector view is created that takes the first three elements of the original vector.
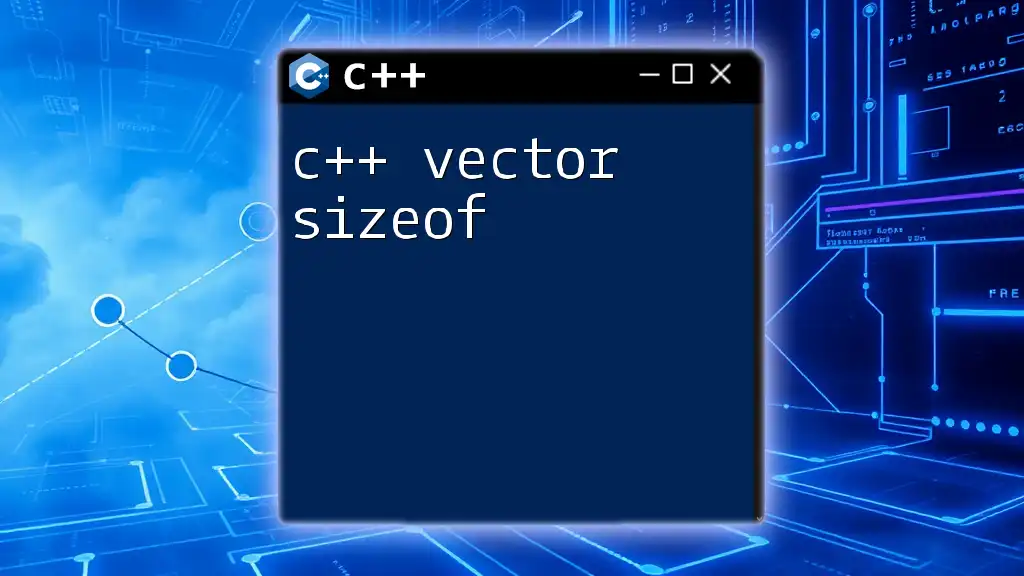
Creating Vector Views
Basic Syntax for Creating a Vector View
Creating a vector view usually employs the `std::views` library. For instance, you can generate views based on criteria such as range or transformation. The syntax is both intuitive and efficient.
Practical Examples
Subsetting Vectors
To create a view for a specific subset of a vector, you can utilize the standard range library features. Consider the following example that drops the first two elements of a vector:
auto subsetView = vec | std::views::drop(2); // Drops the first two elements
Now, `subsetView` includes only the elements `{3, 4, 5}`.
Transforming Views
You can also manipulate vectors through views, enabling transformations. For example, to double the values of a vector, you would write:
auto transformedView = vec | std::views::transform([](int n) { return n * 2; });
In this case, `transformedView` contains `{2, 4, 6, 8, 10}`.
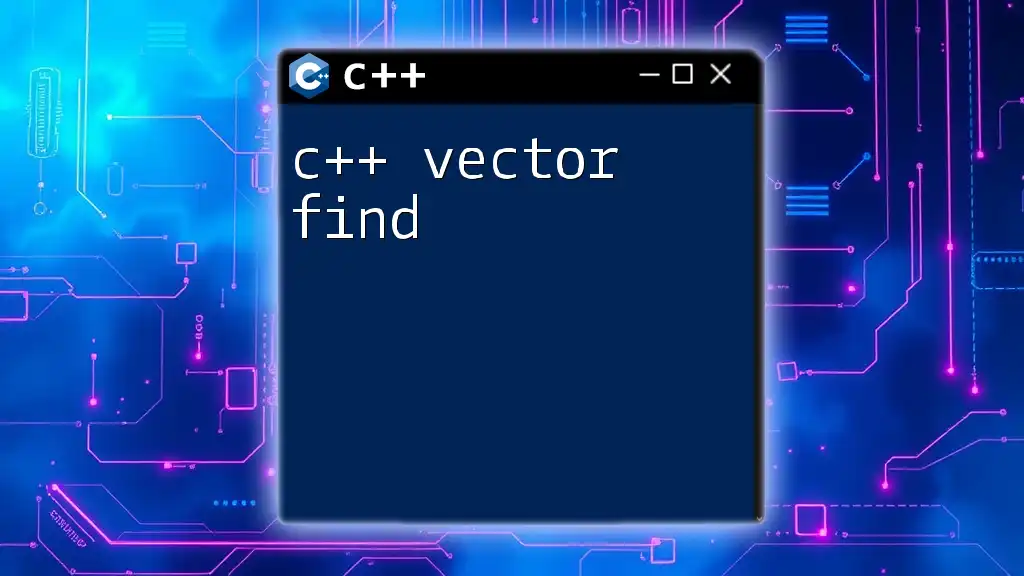
Manipulating Vector Views
Iterating through a Vector View
Iterating through a vector view remains seamless, as it integrates effortlessly with C++’s range-based for loops. This allows for a clean traversal of the elements being referenced.
Modifying Original Vectors through Views
While most views are designed to be read-only, it’s crucial to understand that some may allow for modifications to the underlying data. However, caution is advised—unintended side effects can occur if you are not careful. Here's an example:
auto modifiableView = vec | std::views::drop(1);
for (auto& elem : modifiableView) {
elem += 10; // Adds 10 to elements from index 1 onward
}
If `vec` contains `{1, 2, 3, 4, 5}`, it is updated to `{1, 12, 13, 14, 15}` by modifying the elements within the view.
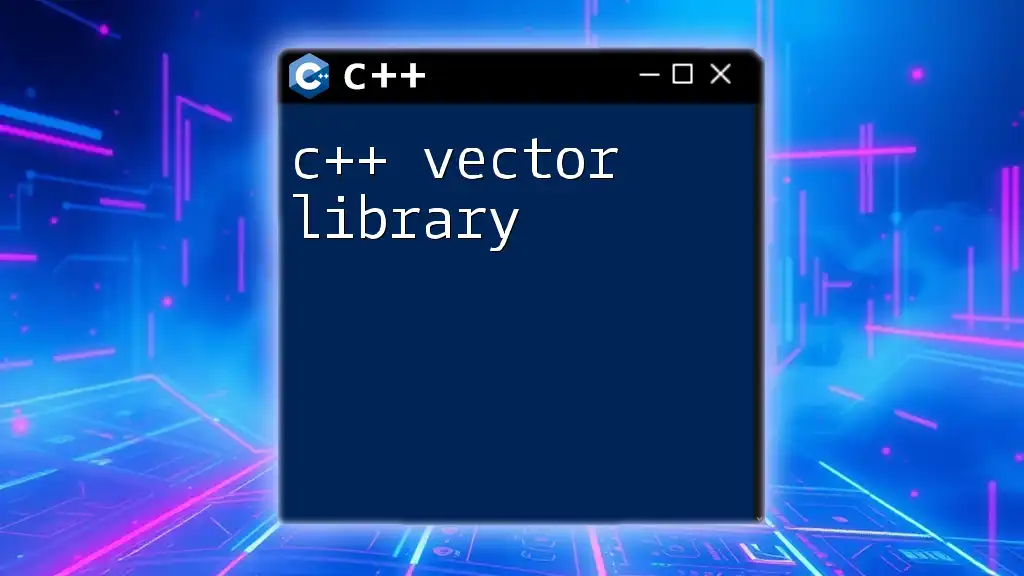
Performance Considerations
When to Use Vector Views
Vector views shine in scenarios where efficiency is paramount, such as when performing complex operations on extensive datasets or when the entire dataset is not necessary for computational tasks. Given their lazy evaluation nature, views can significantly enhance performance.
Performance Benchmarks
Comparative benchmarking can provide insight into performance differences between using views versus traditional vectors. In many scenarios, especially in large data manipulations, views demonstrate lower time complexity and memory footprint, allowing developers to handle larger datasets more effectively.
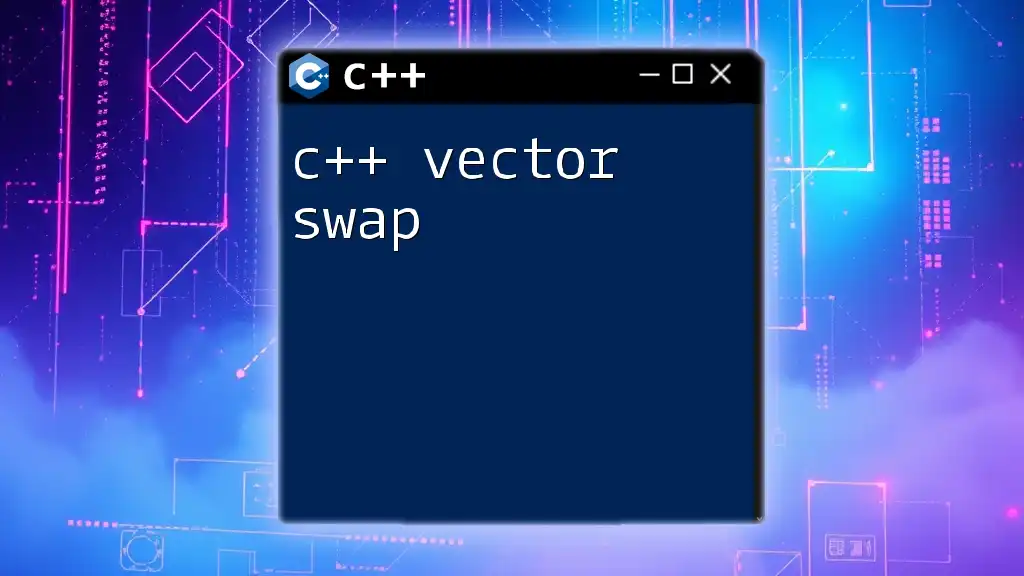
Best Practices
Writing Readable and Maintainable Code
While leveraging vector views, maintaining clarity and expressiveness in your code is crucial. Use meaningful names for your views and ensure that functions applied to them are straightforward to understand.
Avoiding Common Pitfalls
Despite their advantages, using views can lead to confusion, particularly regarding their mutability. A good practice is to remain cautious about the scope and lifetime of the underlying data, ensuring that the referenced data does not go out of scope or get modified unexpectedly.
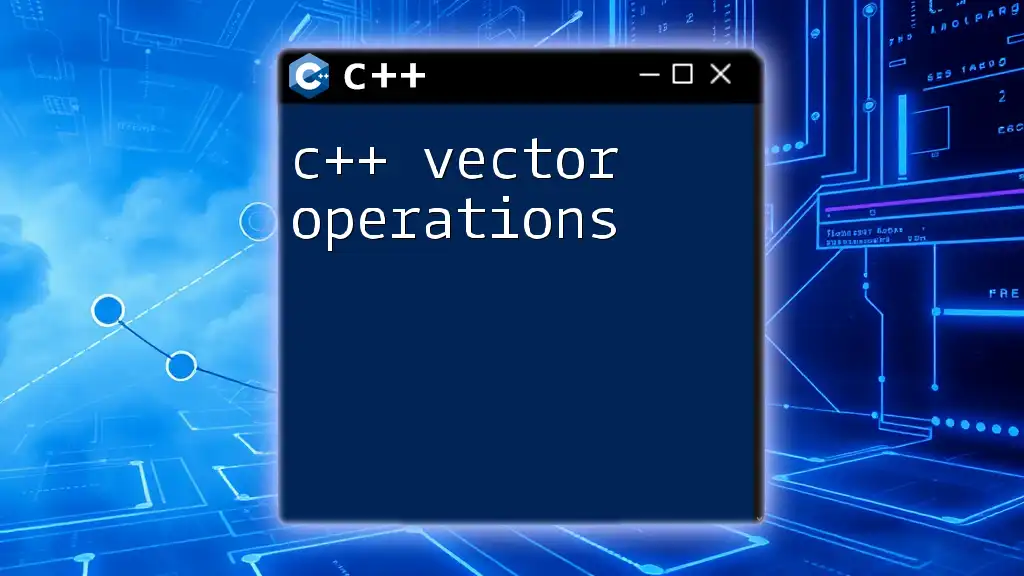
Conclusion
C++ vector views offer a powerful and flexible approach to working with collections in a memory-efficient way. They provide a clean and readable syntax for manipulating large datasets, all while ensuring high performance. As you explore the capabilities of vector views, remember that experimentation and practical application in projects will enhance your understanding and mastery of C++.