In C++, the `end()` function of a vector returns an iterator pointing to the position just past the last element of the vector, which can be used in various algorithms to denote the end of the container.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = vec.end(); // Iterator pointing to the end of the vector
std::cout << "End iterator points to element: " << *(it - 1) << std::endl; // outputs 5
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can resize automatically when elements are inserted or removed. It is part of the C++ Standard Library and provides a way to manage collections of elements efficiently. Key characteristics of vectors include:
-
Dynamic Sizing: Unlike traditional arrays, which have a fixed size, vectors can change their size automatically. This makes them perfect for scenarios where the number of elements is not known in advance.
-
Random Access Capability: Vectors provide fast access to elements using indices, much like arrays. You can access any element using the `[]` operator, making it intuitive and straightforward to use.
Why Use Vectors?
Vectors come with several advantages:
-
Flexibility and Ease of Use: They simplify memory management and provide a variety of built-in functions to make common tasks easier.
-
Memory Management Advantages: Vectors handle their own memory, automatically allocating and deallocating space as needed.
-
Built-in Functions: Functions like `push_back()`, `pop_back()`, and `size()` allow developers to manipulate vectors without worrying about underlying memory complexities.
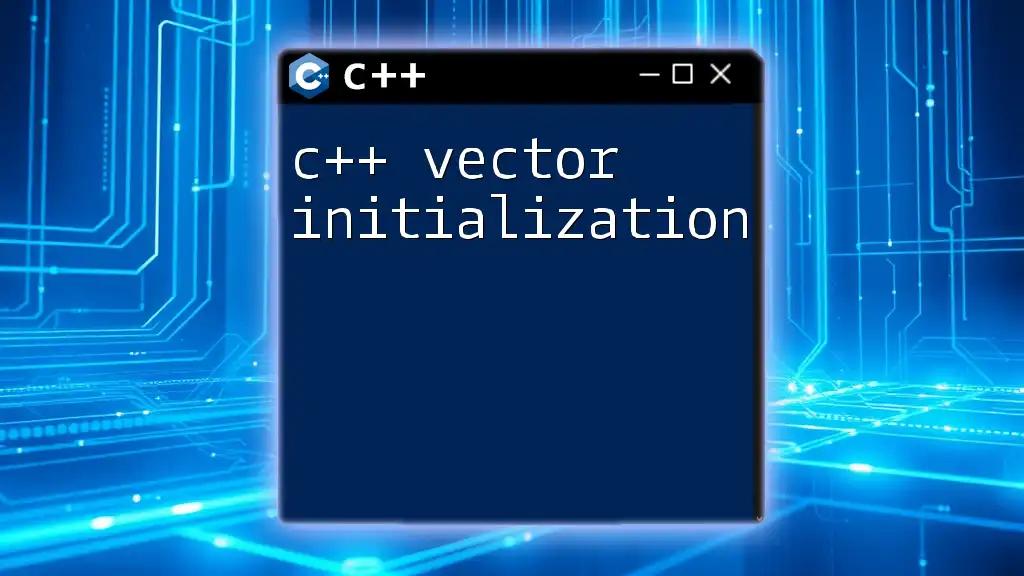
The `end` Function: An Overview
Definition of `end`
The `end` function of a C++ vector returns an iterator pointing to the position just past the last element of the vector. An important point to note is that the iterator returned by `end` does not point to an actual element in the vector; instead, it provides a convenient way to mark the end of the vector.
Key Characteristics of `end`
Understanding iterators is crucial for using the C++ Standard Library effectively. An iterator is an object that enables traversal of the elements in a container (in this case, a vector). The `end` function plays a vital role by providing a sentinel—the iterator that helps determine when to stop the iteration.
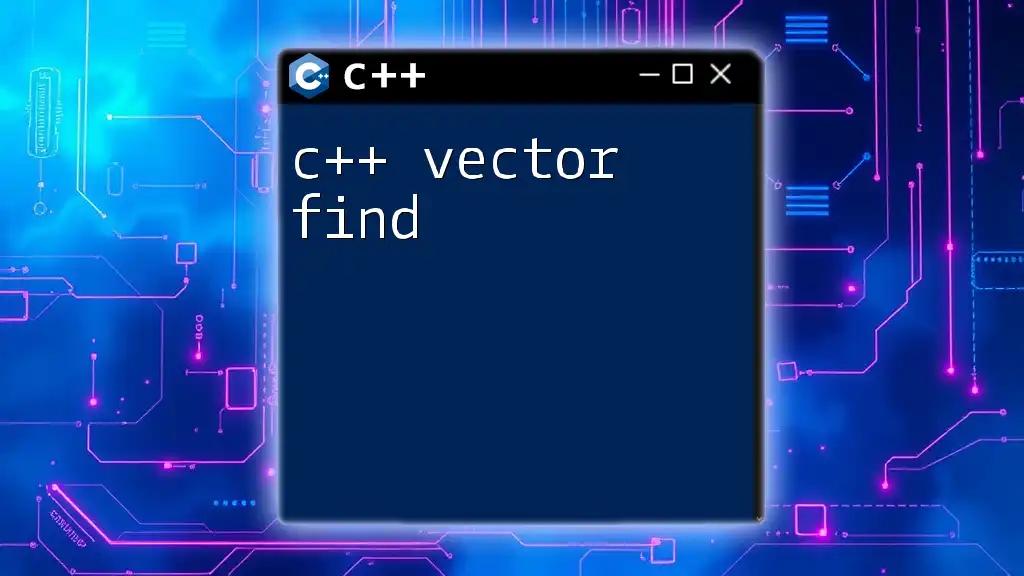
How to Use the `end` Function
Basic Syntax
The basic syntax of the `end` function is as follows:
vector<T>::iterator end();
In this syntax:
- `vector<T>` is the type of the vector.
- `iterator` is the return type of the function.
Example of Using `end`
Here’s a simple demonstration of how to use the `end` function effectively:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::vector<int>::iterator it = numbers.end();
std::cout << "The last element is: " << *(it - 1) << std::endl; // Accessing the last element
return 0;
}
In this example, we create a vector containing integers. The line `std::vector<int>::iterator it = numbers.end();` retrieves an iterator to the position just past the last element of the vector. To access the actual last element, we decrement this iterator by one.
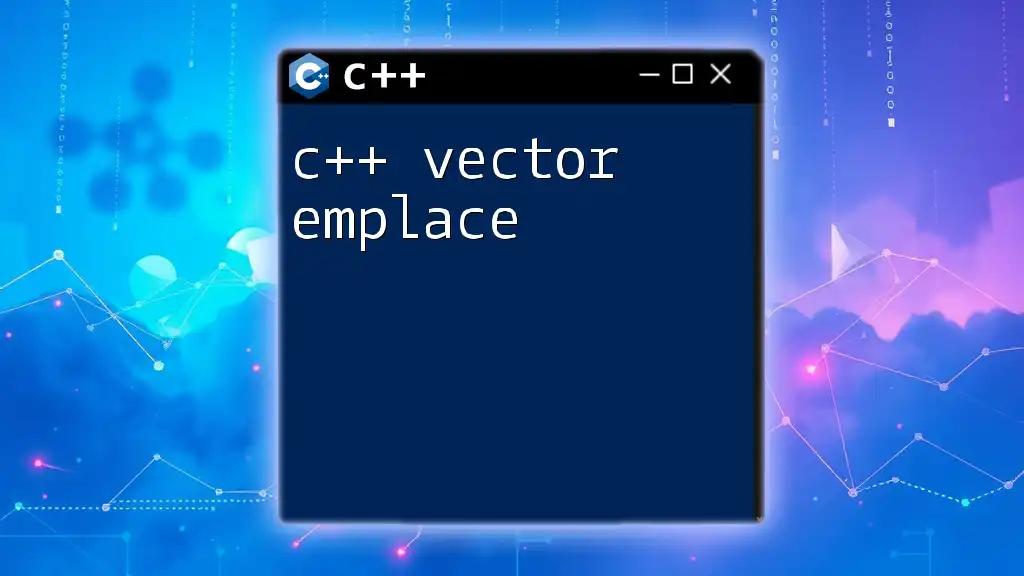
Practical Applications of `end`
Iterating Over Vectors Using `end`
Using a For Loop
One of the primary uses of the `end` function is during iteration. Here is how to iterate through a vector using a traditional for loop:
for (std::vector<int>::iterator it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
In this loop, we start from the beginning of the vector and continue until we reach the iterator returned by `end`. The condition `it != numbers.end()` prevents going beyond the last element, ensuring safe access to the vector’s contents.
Using Range-Based For Loops
C++11 introduced range-based for loops, which can simplify iteration even more. By using range-based for loops, the need for explicit iterators is eliminated:
for (auto& number : numbers) {
std::cout << number << " ";
}
The range-based loop abstracts away the iterator management, making the code cleaner and reducing the chances of errors.
Modifying Elements with `end`
The `end` function also plays a key role in modifying elements within a vector. For instance, if you need to remove elements, you can do so by using the following command:
numbers.pop_back(); // Remove the last element
After running this command, the vector's size decreases by one, and the iterator returned by `end` reflects this new size. You can verify the changes using `end`, helping ensure that your operations maintain the integrity of the vector.
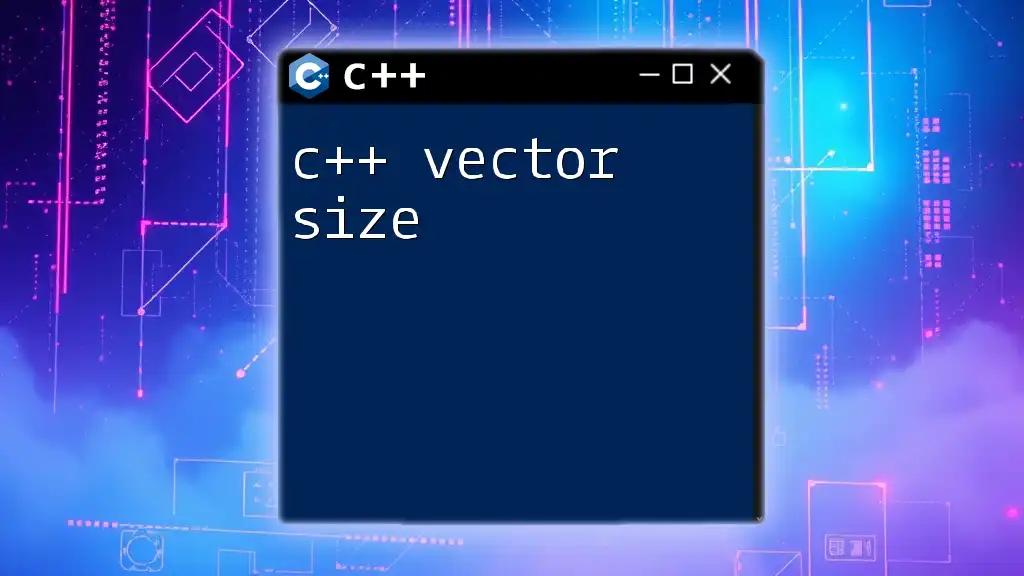
Common Errors When Using `end`
Off-By-One Errors
One of the most common mistakes when working with the `end` function is off-by-one errors. For example, consider the following incorrect loop:
// Wrong usage
for (std::vector<int>::iterator it = numbers.begin(); it <= numbers.end(); ++it) {
std::cout << *it << " "; // This is incorrect, will lead to undefined behavior
}
In this case, the loop condition should use `it != numbers.end()` instead of `it <= numbers.end()`. Using `<=` can lead to dereferencing an invalid memory location, causing undefined behavior.
Dereferencing the `end` Iterator
It's crucial to recognize that you're never supposed to dereference the iterator returned by `end`. Attempting to do so can yield unpredictable results:
std::cout << *numbers.end(); // Undefined behavior
Instead, stick to using the iterator returned by `end` only for comparisons and not for accessing values.
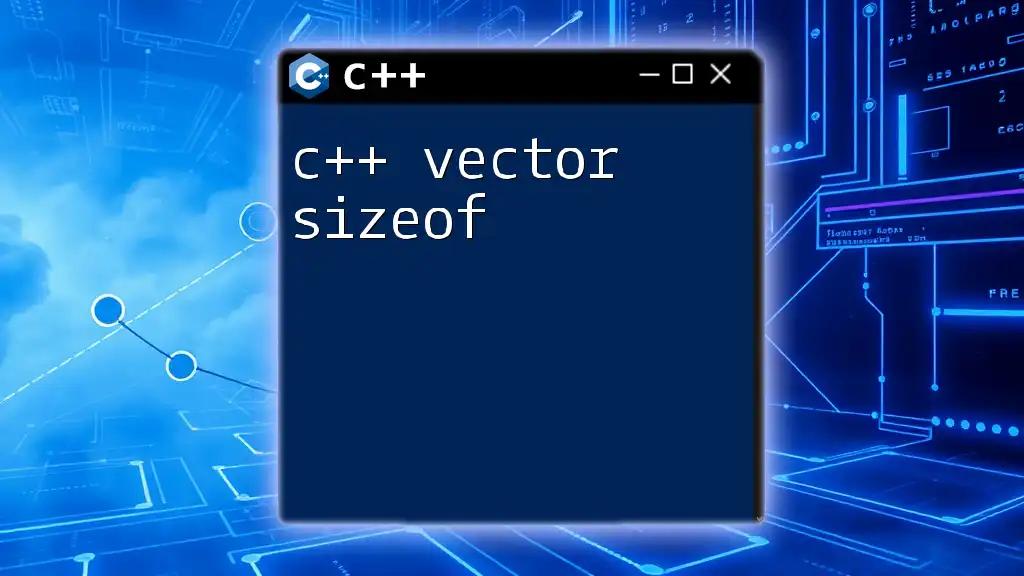
Conclusion
In summary, the c++ vector end function is an invaluable tool for managing collections of data. It helps ensure accurate and efficient iteration and manipulation of vector elements. Mastering its use can significantly enhance your programming skills and improve code quality. As you progress in your C++ journey, practicing with vectors, including methods like `end`, will provide you with a strong foundation for more complex data structures and algorithms.
Additional Resources
To further deepen your understanding, consider exploring books or online resources on C++ and the Standard Template Library (STL). The more you practice and experiment with these concepts, the more proficient you will become. Also, keep an eye out for expert tips and tricks that can help streamline your C++ coding experience.