In C++, the `swap` function exchanges the contents of two vectors, allowing their values to be quickly swapped without needing to copy data.
Here's a code snippet demonstrating how to use `swap` with C++ vectors:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
// Swapping the vectors
vec1.swap(vec2);
// Output to verify the swap
for (int i : vec1) std::cout << i << " "; // Output: 4 5 6
std::cout << std::endl;
for (int i : vec2) std::cout << i << " "; // Output: 1 2 3
return 0;
}
What are C++ Vectors?
Definition and Characteristics
C++ vectors are dynamic arrays provided by the Standard Template Library (STL). They offer a flexible, high-level approach to template-based programming. Unlike traditional arrays, vectors can resize automatically when elements are added or removed. This dynamic nature means vectors manage their own memory allocation and deallocation, significantly simplifying coding.
In terms of characteristics:
- Dynamic Sizing: Vectors can grow and shrink as needed, accommodating varying workloads and data sizes.
- Automatic Memory Management: They handle memory allocation internally, eliminating concerns about memory leaks that are common with raw pointers.
- Type-Safe: By using templates, vectors ensure that only elements of a specific type are stored, enhancing type safety.
When to Use Vectors
Vectors are suitable for scenarios where you need a collection of items that can change in size, such as:
- When the number of elements is not known at compile time.
- Situations requiring frequent additions or deletions from the middle of the sequence.
- Cases where factors like easy access to elements via indices and efficient iteration are needed.
Advantages over traditional arrays include:
- Ease of use due to member functions like `push_back()` and `pop_back()`.
- Enhanced functionality for sorting and searching through STL algorithms.
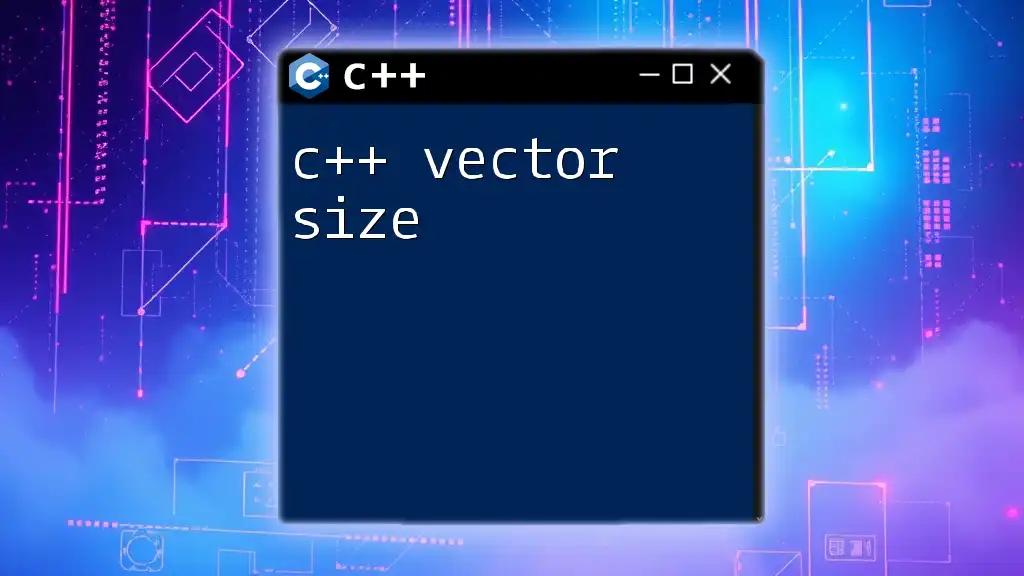
Understanding the Swap Function
Definition of Swap in C++
In C++, the `swap` function is a powerful utility that allows two objects to exchange their values easily and efficiently. The `swap` function for vectors is a member function that directly swaps the contents of two vectors, ensuring that their data is exchanged without a large overhead.
Why Use Swap?
Using `swap` provides substantial benefits:
- Performance: Swapping typically involves fewer operations than copying, particularly for large datasets. Instead of copying each element, `swap` merely exchanges the internal pointers or metadata of the vectors.
- Resource Efficiency: With `swap`, no new memory allocation is required, which can enhance performance in resource-constrained applications.
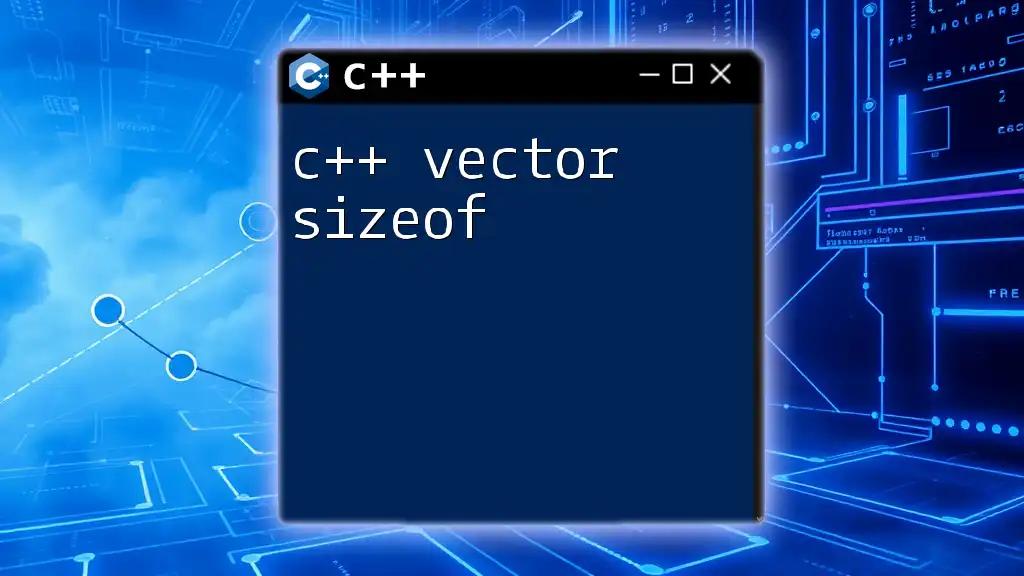
Syntax of Vector Swap
Overview of the Syntax
The syntax for using the vector's `swap` member function is quite straightforward. Here’s how you call it:
vector1.swap(vector2);
Parameters:
- `vector1`: The vector calling the swap method.
- `vector2`: The vector to swap contents with.
Basic Example
Here’s a simple code snippet that demonstrates a basic use of the `swap` function:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
vec1.swap(vec2);
std::cout << "vec1: ";
for (int n : vec1) std::cout << n << ' ';
std::cout << "\nvec2: ";
for (int n : vec2) std::cout << n << ' ';
return 0;
}
In this example, after the `swap`, `vec1` will contain the elements `{4, 5, 6}` and `vec2` will have `{1, 2, 3}`. The swap operation effectively exchanges the contents.

In-Depth Look at Vector Swap
Member Function `swap`
The `swap` method operates quickly, often in constant time (O(1)), because it swaps internal data structures rather than copying each individual element. This efficiency is particularly beneficial for large vectors, allowing applications to remain responsive under heavy data manipulation.
Swap in Action
Case Study: Performance Comparison
In systems programming, performance can often be pivotal. Here’s an example comparing the performance of copying versus swapping vectors:
#include <chrono>
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec1(1000000, 1);
std::vector<int> vec2(1000000, 2);
auto start = std::chrono::high_resolution_clock::now();
vec1 = vec2; // Copy
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Copy time: " << std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << " microseconds\n";
start = std::chrono::high_resolution_clock::now();
vec1.swap(vec2); // Swap
end = std::chrono::high_resolution_clock::now();
std::cout << "Swap time: " << std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << " microseconds\n";
return 0;
}
In this benchmark, the output will typically show that the swap operation is significantly faster than copying, especially as the size of the vectors increases. This empirical evidence underscores the efficiency of using `swap` in performance-critical applications.
Exception Safety
The vector swap function is designed to provide strong exception safety guarantees—it will not throw exceptions under normal circumstances, making it a robust choice for operations where performance and reliability are critical.
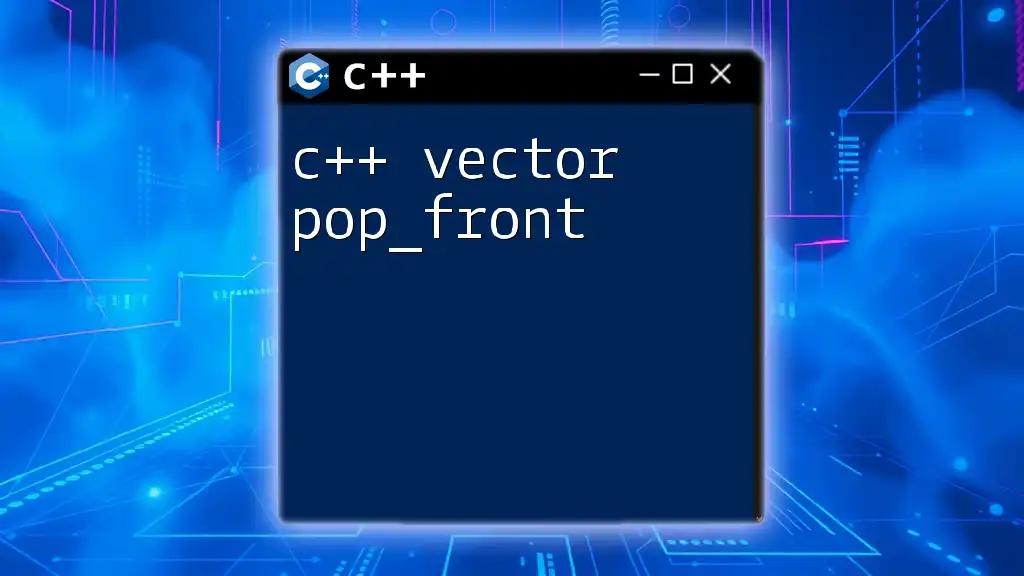
Practical Uses of Vector Swap
Use Case Scenarios
Vectors can be manipulated in various scenarios. For example, consider needing to exchange data between two arrays:
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
// Swap the contents
vec1.swap(vec2);
This approach can be particularly useful in algorithms where intermediate storage is required, such as sorting or during merges.
Tips for Effective Use
- Avoid Unnecessary Swaps: If vectors contain the same data or if you don’t need to exchange them, it’s prudent to avoid swap calls.
- Use in Functionality: Leverage `swap` to reduce complexity in algorithms, especially when working on in-place manipulations or during partitioning in sorting algorithms.
- Be Thread-Safe: Ensure both vectors are not shared across threads without protective measures as swapping is not thread-safe.
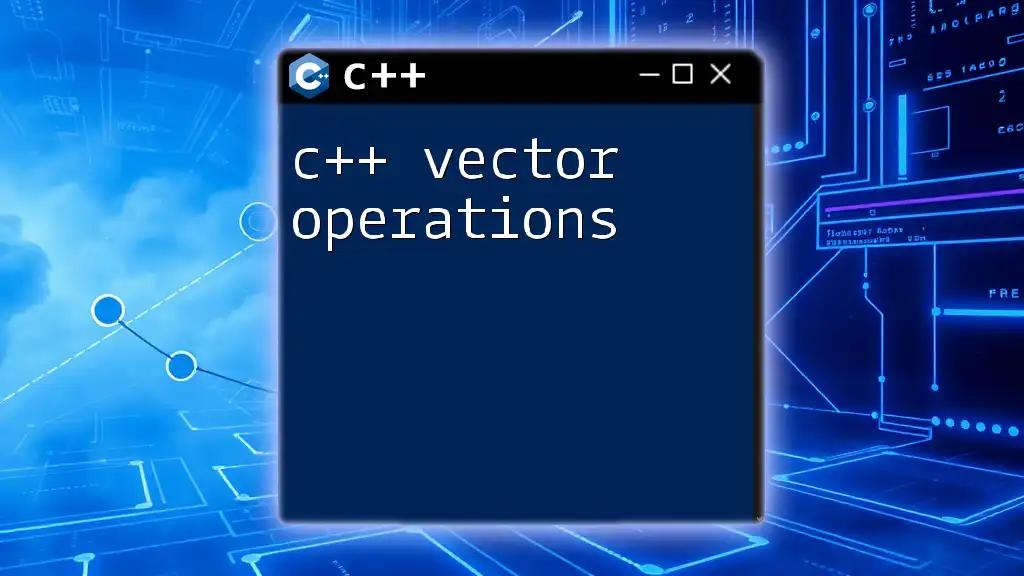
Alternative Methods to Swap Vectors
Using `std::swap`
In addition to the member function, C++'s STL provides a free function `std::swap()` that can achieve the same effect. The usage is slightly different but equally effective:
#include <algorithm>
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::swap(vec1, vec2); // Effectively swaps vec1 and vec2
return 0;
}
Comparing Swap Methods
While both `swap` and `std::swap` are efficient, using `swap` as a member function keeps context explicit and can be slightly more readable when dealing specifically with vector types. However, `std::swap` often offers better flexibility when working with different containers or data types.
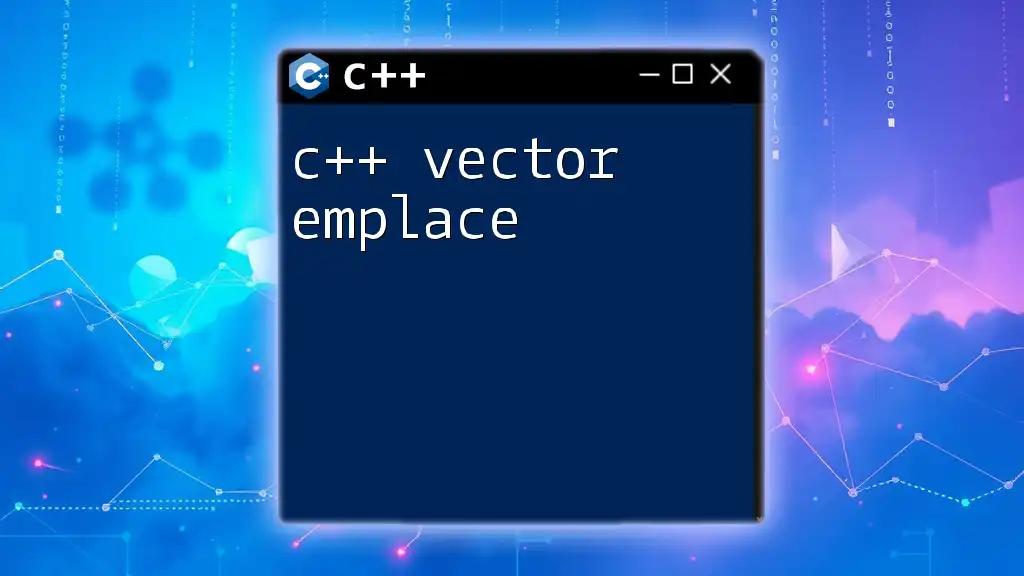
Conclusion
In summary, understanding c++ vector swap is essential for efficient vector manipulation. This powerful function simplifies code while enhancing performance in applications requiring dynamic data handling. By leveraging the features of vectors and the swap operation, developers can optimize both resource usage and execution speed effectively.

Additional Resources
To further expand your knowledge, consider exploring these resources:
- C++ Reference for [std::vector](https://en.cppreference.com/w/cpp/container/vector)
- Books on Advanced C++ Programming Techniques
- Tutorials on STL Algorithms

FAQs
Common Questions about Vector Swap
-
What happens to the original vectors after a swap? The original vectors retain their initial memory allocations, but their contents are exchanged. After a swap, the original contents of the vectors are no longer in their initial vectors.
-
Can swap be used with empty vectors? Yes, swapping empty vectors is safe and won’t raise any issues. They will remain empty after the operation.
-
Is there a performance penalty for swapping large vectors? Generally, no. Swapping is designed to be efficient, even for large vectors, as it avoids copying data and works with internal pointers instead.