The `std::max_element` function in C++ is used to find the largest element in a vector, returning an iterator to that element.
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> nums = {1, 3, 2, 5, 4};
auto max_it = std::max_element(nums.begin(), nums.end());
std::cout << "Max element: " << *max_it << std::endl;
return 0;
}
Understanding max_element in C++
C++ provides a powerful tool in its Standard Template Library (STL), particularly with its `max_element` function, which is often utilized in conjunction with vectors. But what exactly is `max_element`?
What is max_element in C++?
`max_element` is a standard library algorithm that finds the largest element in a range defined by iterators. When used with a vector, it efficiently locates the maximum value stored within it.
Header File Requirement
To make use of `max_element`, you'll need to include the appropriate header at the beginning of your program. This requirement ensures that all STL functions and models are properly recognized by the compiler. Simply add:
#include <algorithm>
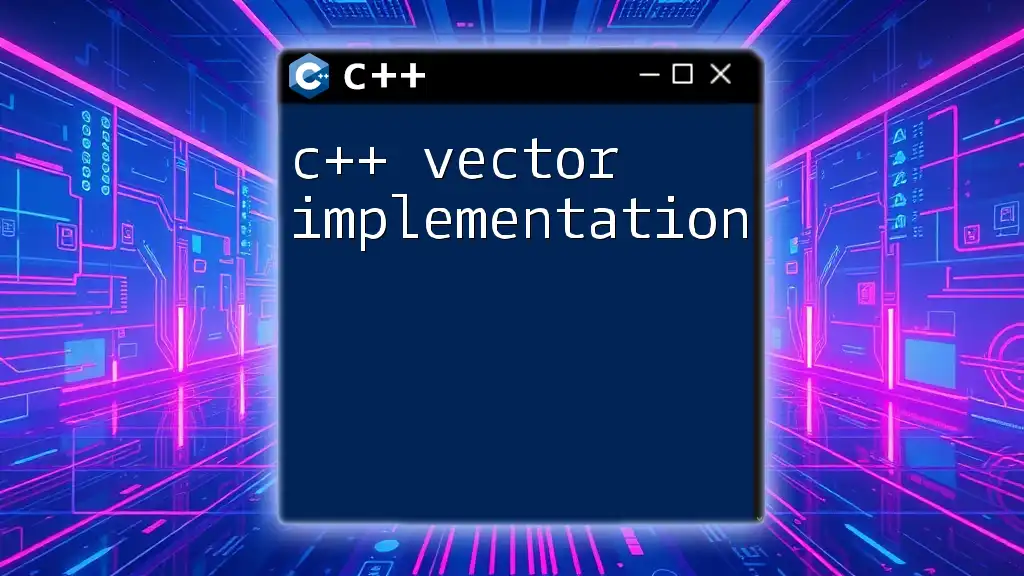
Syntax of max_element
The basic syntax for using `max_element` is as follows:
std::vector<Type>::iterator max_element(Iterator begin, Iterator end);
Here, `begin` and `end` parameters define the range of the vector where `max_element` will search for the maximum value. This function returns an iterator pointing to the maximum element in that specified range.
Using max_element with Custom Comparators
In some situations, you may want to define custom criteria for determining the maximum element. This can be achieved using a custom comparator, such as a lambda function, which you can pass as a third argument in `max_element`.
Here's a simple syntax to illustrate:
auto max_element(Iterator begin, Iterator end, Comparator comp);
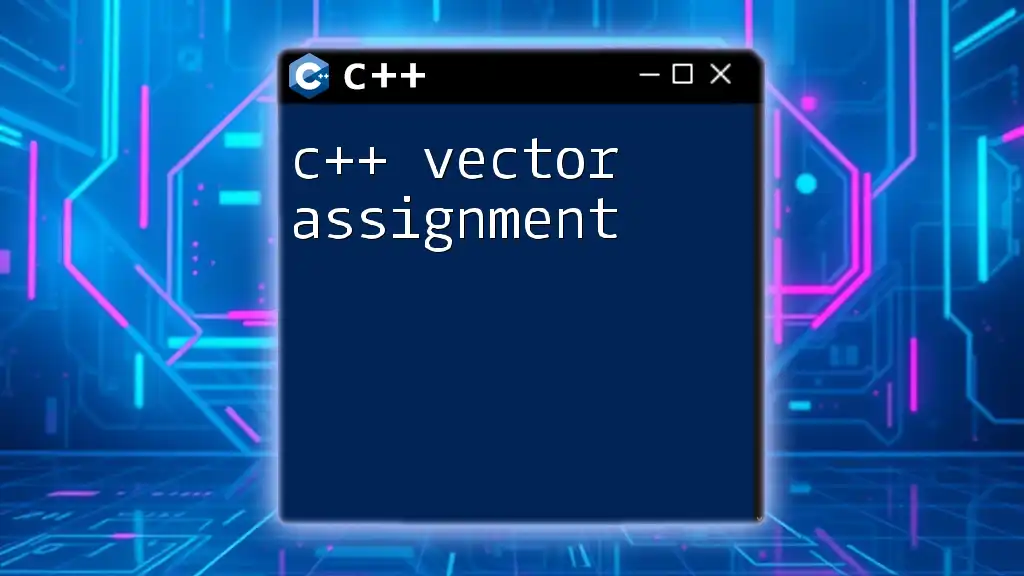
Practical Examples of max_element
Finding the Maximum Value in a Vector
Let's start with a basic example using an integer vector to find the maximum number.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 3, 5, 2, 4};
auto maxIt = std::max_element(numbers.begin(), numbers.end());
std::cout << "Maximum Element: " << *maxIt << std::endl; // Output: 5
return 0;
}
In this example:
- We declare a vector of integers named `numbers` containing some values.
- By calling `std::max_element` with `numbers.begin()` and `numbers.end()`, we retrieve an iterator pointing to the maximum value.
- The dereferencing operator `*` is used to display the maximum value found.
Using max_element with Custom Data Types
`max_element` isn't limited to simple data types; it can also be applied to structures. Here’s an example using a struct to find the student with the highest score:
#include <iostream>
#include <vector>
#include <algorithm>
struct Student {
std::string name;
int score;
};
int main() {
std::vector<Student> students = {{"Alice", 90}, {"Bob", 85}, {"Charlie", 95}};
auto topStudent = std::max_element(students.begin(), students.end(),
[](const Student &a, const Student &b) { return a.score < b.score; });
std::cout << "Top Student: " << topStudent->name << " with Score: " << topStudent->score << std::endl; // Output: Charlie 95
return 0;
}
Here:
- We define a `Student` struct with `name` and `score` attributes.
- The vector `students` holds multiple `Student` objects.
- We pass a lambda function as a comparator to `max_element`, allowing us to find the student with the highest score.
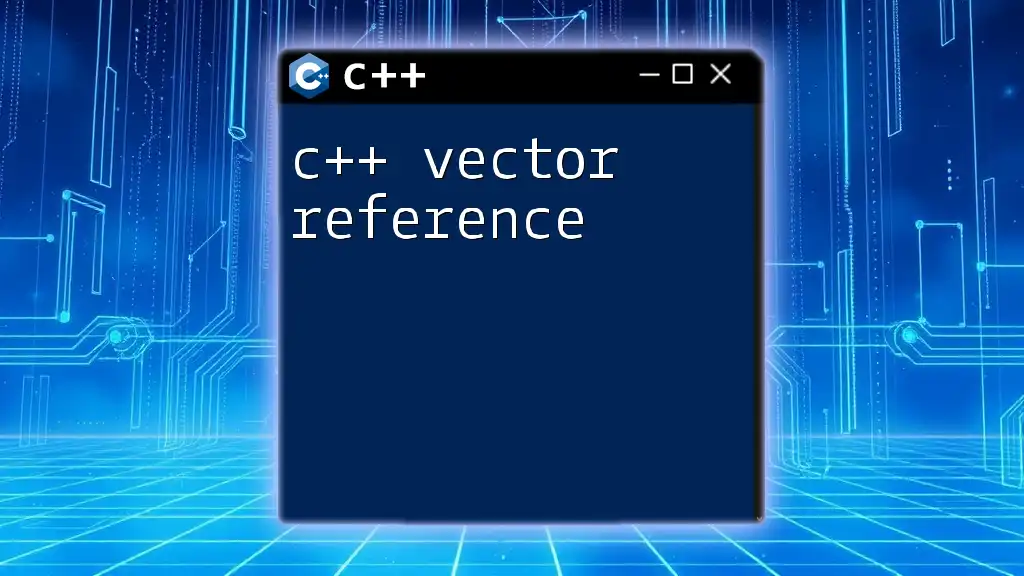
Handling Edge Cases
Understanding potential edge cases is crucial when utilizing `max_element`.
Empty Vectors
If `max_element` is called on an empty vector, the behavior is undefined, which can lead to unexpected results or crashes. Therefore, it's best practice to check if the vector is empty before invoking this function:
if (!numbers.empty()) {
auto maxIt = std::max_element(numbers.begin(), numbers.end());
// Proceed with using *maxIt
}
All Elements are Equal
When all values in the vector are identical, `max_element` will still return an iterator pointing to the first occurrence of that maximum value. While this won't cause issues, it’s good to be aware of this when interpreting results.
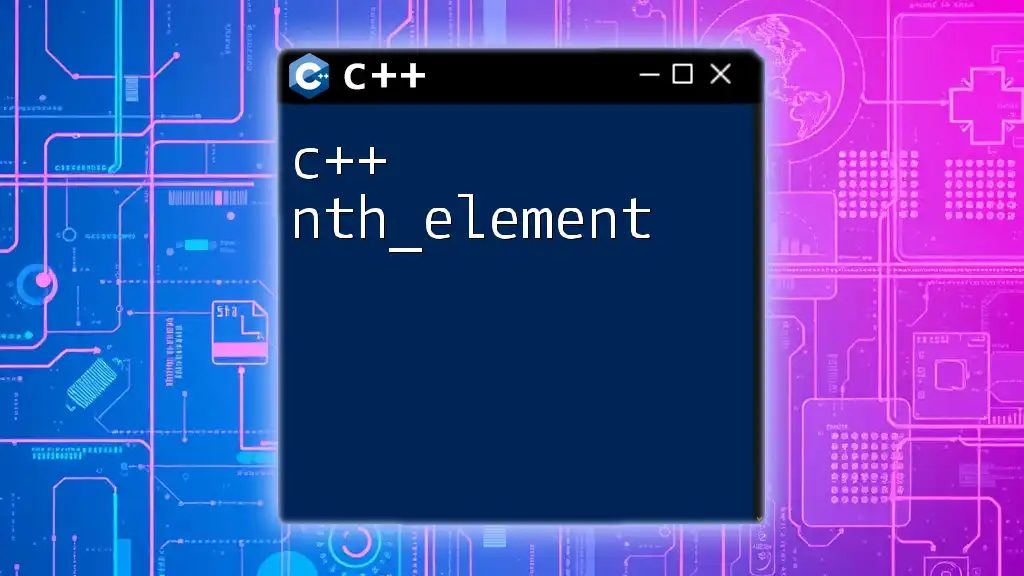
Performance Considerations
Time Complexity of max_element
The time complexity for `max_element` is O(n), where n is the number of elements in the vector. This means the function will have to scan each element in the worst-case scenario to determine the maximum, making it efficient for small to medium-sized vectors.
Space Complexity Insights
The space complexity is generally O(1) since `max_element` doesn't create additional containers; it simply operates on the given range and returns an iterator.
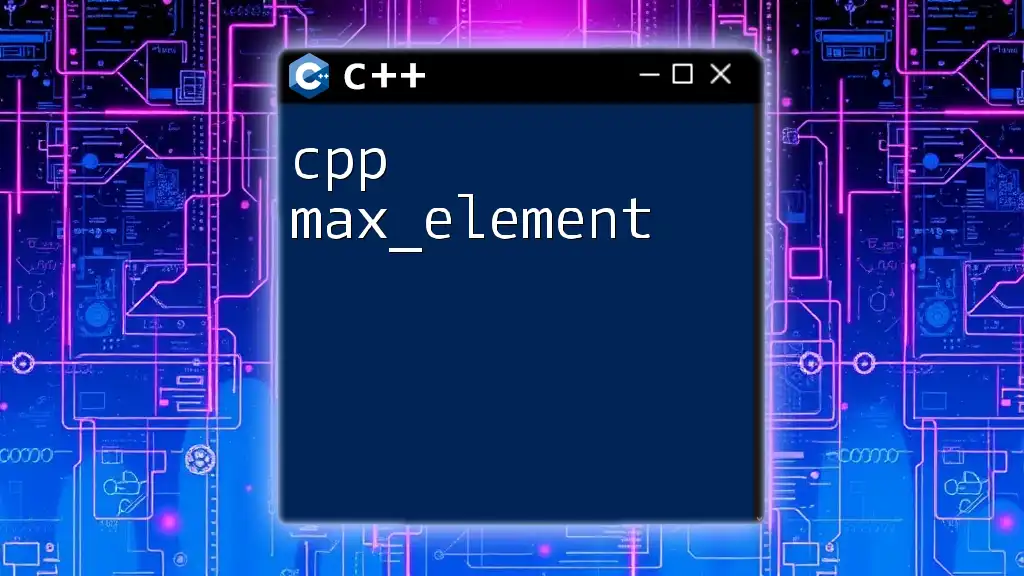
Conclusion
In summary, C++ vector `max_element` is an essential tool for anyone looking to efficiently locate the largest value in a dataset. Implementing it correctly can save time and enhance the effectiveness of your C++ programs.
Encouragement to Practice
Don't just stop here! I encourage you to experiment with `max_element` using various data types and custom comparison criteria. The more you practice, the more proficient you will become in using these powerful STL functions.
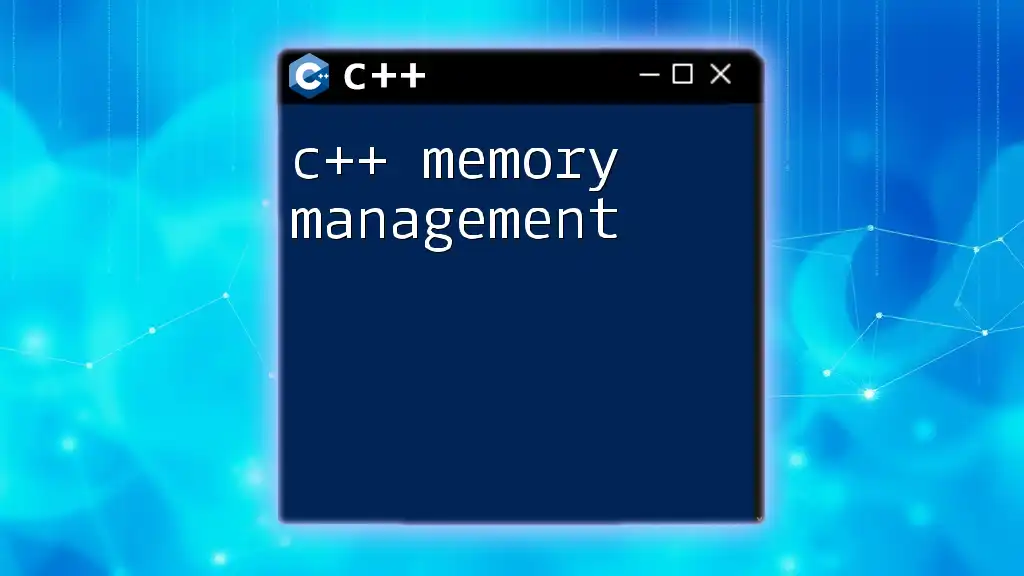
Additional Resources
For further reading and additional examples, consider exploring the wider aspects of C++'s STL and algorithms. This foundational knowledge will empower you to write more concise and efficient C++ code in your upcoming projects.
Call to Action
Feel free to share your experiences and any questions regarding C++ vector `max_element`. Engaging with the community can often lead to new insights and learning opportunities. Subscribe for more tips and tricks on mastering C++ coding!