C++ vector assignment allows you to assign new values to a vector, either by directly initializing it with a list of elements or by assigning the contents of one vector to another.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <vector>
int main() {
// Method 1: Direct initialization
std::vector<int> vec1 = {1, 2, 3, 4, 5};
// Method 2: Assignment from another vector
std::vector<int> vec2;
vec2 = vec1;
// Output the contents of vec2
for (int v : vec2) {
std::cout << v << " ";
}
return 0;
}
Understanding C++ Vectors
What is a Vector?
In C++, a vector is a dynamic array that can change its size during runtime. Unlike traditional arrays, which have a fixed size, vectors can grow and shrink as needed, providing flexibility in data management. A vector can store elements of the same data type and offers convenient methods for accessing and manipulating these elements.
Vectors have several characteristics:
- They manage their own memory.
- They are part of the Standard Template Library (STL), offering a rich set of functionalities.
- They can store elements that may or may not be initialized, providing control over how you manage your data.
When compared to arrays, vectors have several advantages:
- Dynamic sizing: You can easily add or remove elements.
- Built-in functions: Vectors come with a multitude of member functions that simplify operations like insertion, deletion, and search.
- Memory management: The `std::vector` automatically handles memory allocation and deallocation for you.
Why Use Vectors?
Choosing vectors over arrays is beneficial for several reasons. Their ability to resize dynamically makes them ideal for scenarios where you do not know the number of elements in advance. Moreover, their member functions—such as `.push_back()`, `.pop_back()`, and `.size()`—enable you to perform complex operations with minimal code. Vectors allow for safer programming by handling boundaries and reducing the chance of overflow errors commonly associated with static arrays.
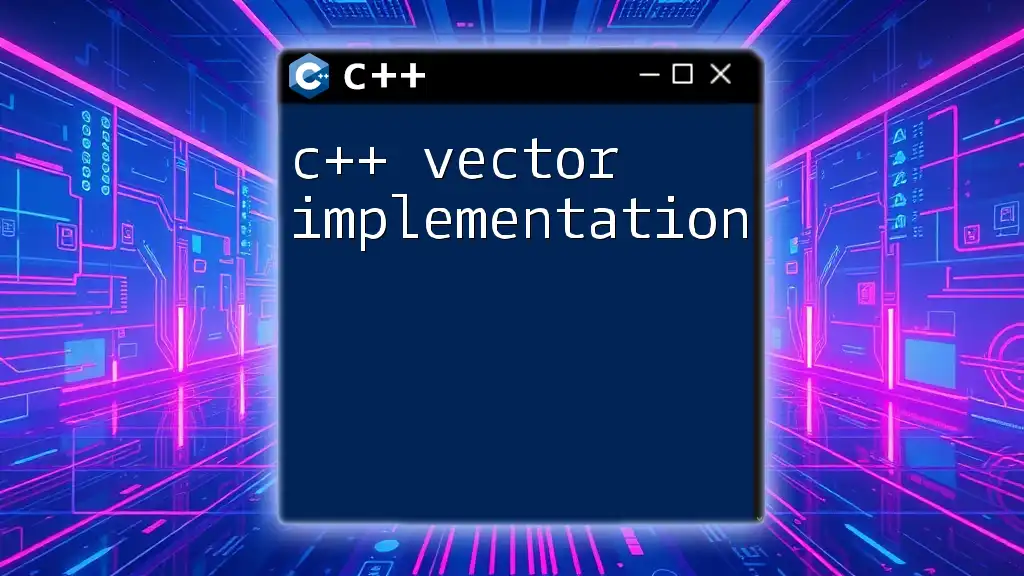
Basics of C++ Vector Assignment
What is Vector Assignment?
C++ vector assignment refers to the process of assigning values to a vector or copying one vector's data into another. This is crucial for managing collections of data efficiently. Through vector assignment, you can overwrite existing values, initialize a vector, or create copies of vectors seamlessly.
Syntax of Vector Assignment
The syntax for vector assignment is straightforward and involves either using the `assign()` member function or the assignment operator (`=`). Understanding these two methods will help you leverage the full potential of vectors in your C++ programs.
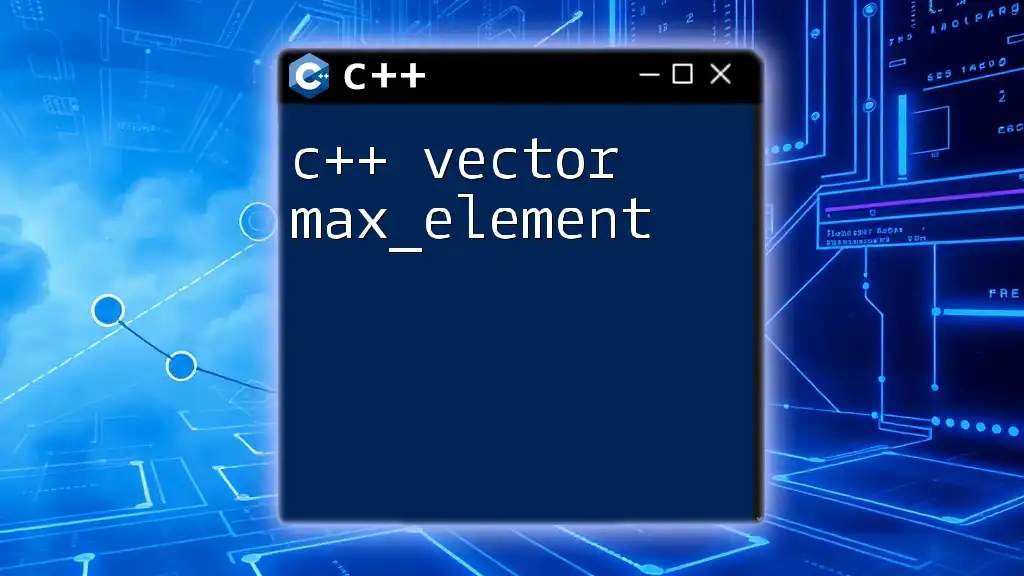
Assigning Vectors
Using the `assign` Method
The `assign()` method is specifically designed for assigning new values to a vector. It takes two parameters: the number of elements to assign and the value to be assigned.
Its syntax looks like this:
vectorName.assign(n, value);
Where `n` is the number of times the value should be assigned to the vector. Here is a practical example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v;
v.assign(5, 10); // Assigns 10 to vector v 5 times
for (auto i : v) {
std::cout << i << " ";
}
return 0;
}
In this example, we declare a vector `v`, then use the `assign()` method to fill it with the value `10`, repeated `5` times. The output will be `10 10 10 10 10`, clearly demonstrating how `assign()` establishes the vector's values.
Using the Assignment Operator
Another way to assign elements to a vector is by using the assignment operator (`=`). This approach is often used to copy elements from one vector to another.
The syntax looks like this:
vectorName2 = vectorName1;
Here’s an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v1 = {1, 2, 3};
std::vector<int> v2;
v2 = v1; // Assigns contents of v1 to v2
for (auto i : v2) {
std::cout << i << " ";
}
return 0;
}
As shown, the vector `v2` is assigned the contents of `v1`. The elements of `v1` are copied to `v2`, so the output will be `1 2 3`.
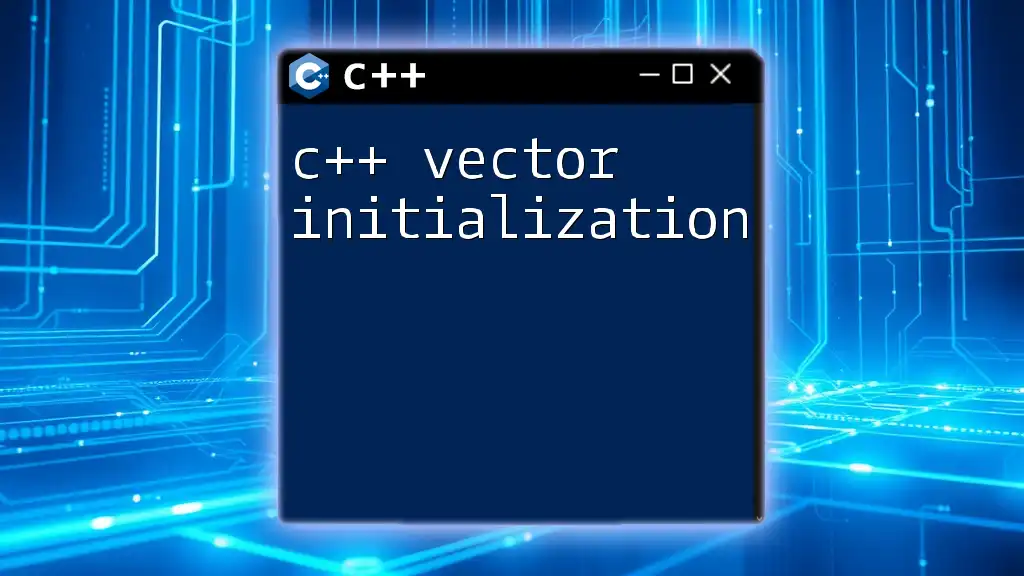
Overwriting Existing Data
Assigning New Values to an Existing Vector
Vectors can be reassigned to hold entirely new datasets. This overwriting is a vital feature when you need to change the data contained within a vector without creating an entirely new instance.
For example, consider the following code snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3, 4, 5};
v.assign(3, 7); // New values are assigned, discarding previous ones
for (auto i : v) {
std::cout << i << " ";
}
return 0;
}
In this code, the vector `v`, initially containing the numbers `1` to `5`, is reassigned using the `assign()` method. The new value `7` is assigned, repeated `3` times, and hence the output becomes `7 7 7`.
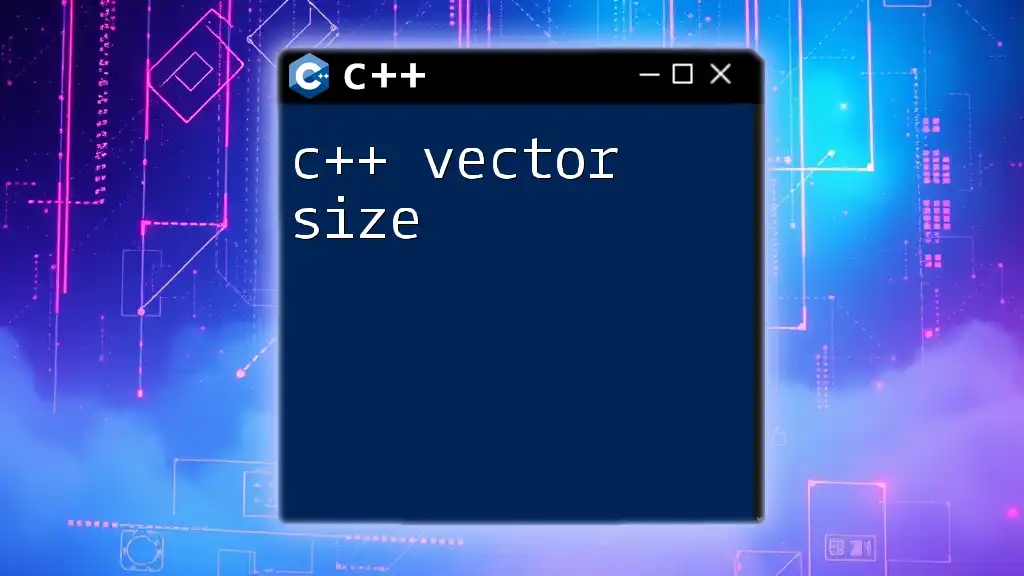
Copying One Vector to Another
Shallow vs Deep Copy
In C++, copying a vector can either be a shallow copy or a deep copy. A shallow copy means that both the original and copied vector refer to the same memory locations for their elements. In contrast, a deep copy means that a completely new set of elements is created.
The assignment operator performs a shallow copy: the copies point to the same data, making it crucial to understand this behavior when working with data that might change.
Example of Copying Vectors
Here's how you can use the assignment operator to copy one vector to another:
#include <iostream>
#include <vector>
int main() {
std::vector<int> original = {1, 2, 3};
std::vector<int> copy = original; // Shallow copy occurs
copy[0] = 10; // Change in copy does not affect original
for (auto i : original) {
std::cout << i << " "; // Outputs: 1 2 3
}
return 0;
}
In this example, modifying the `copy` vector does not affect the `original`, showcasing the safe nature of vector copying when using the assignment operator.
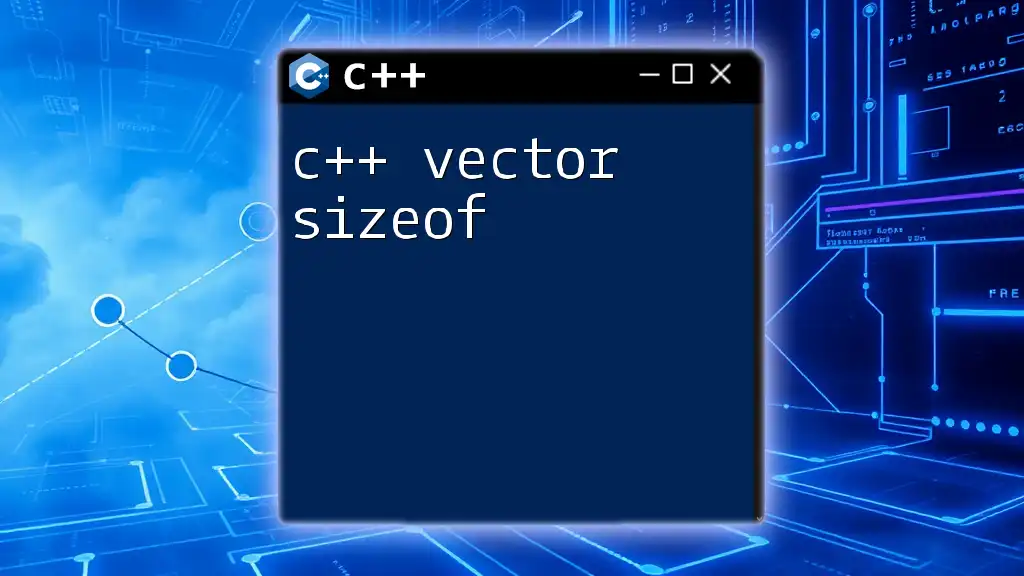
Best Practices for Vector Assignment
Efficient Use of Vectors
When working with vectors, it's essential to choose the right method for assignment based on your needs. Using the `assign()` method is perfect for initializing a new vector or changing all contents, while the assignment operator should be used for copying or replacing existing vectors.
Performance Considerations
Performance can also be impacted by how you manage your vectors. Avoid unnecessary copies, and in scenarios where you know the size of the vector in advance, consider using the `reserve()` method to preallocate memory. This can help avoid multiple allocations and improve performance.
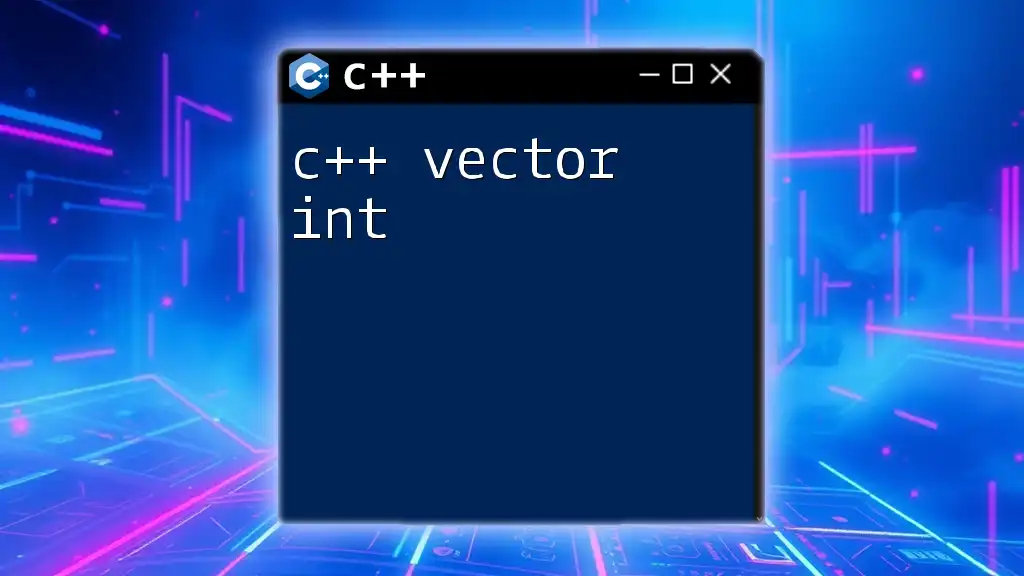
Common Errors and Troubleshooting
Common Errors in Vector Assignment
One common error is attempting to access elements of an uninitialized vector. For instance:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v;
v[0] = 10; // Error: vector is not initialized
return 0;
}
This will lead to undefined behavior since there are no elements in `v` yet. Always ensure that a vector has been initialized before trying to access its elements.
Tips for Effective Debugging
Thoroughly test your code in small blocks to isolate issues related to vector assignments. Utilize debugging techniques such as print statements or debugging tools to ensure that vectors contain the expected values after assignment.
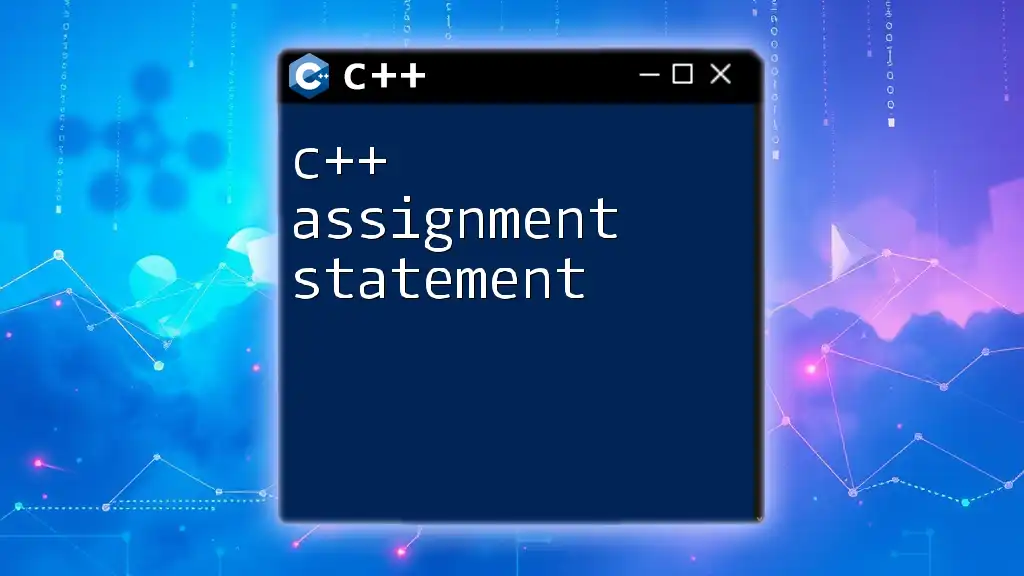
Conclusion
Recap of Key Concepts
In summary, understanding C++ vector assignment is key to effective data manipulation. This guide has covered the syntax, methods, and nuances associated with vector assignment, such as using the `assign()` method compared to the assignment operator.
Encouragement for Continued Learning
Continue exploring the STL and practice further on vector operations. As you become more familiar with these concepts, you'll find vectors to be a powerful tool in your C++ arsenal. Embrace the learning journey ahead!