In C++, a vector constructor is used to create a vector, allowing you to specify its size and initial values, as demonstrated in the following example:
#include <vector>
#include <iostream>
int main() {
// Creates a vector of size 5, initialized with the value 10
std::vector<int> vec(5, 10);
for(int val : vec) {
std::cout << val << " "; // Outputs: 10 10 10 10 10
}
return 0;
}
What is a Vector in C++?
A vector in C++ is a dynamic array offered by the Standard Template Library (STL). Unlike traditional arrays, vectors can change in size, allowing programmers to manage collections of data more flexibly. This dynamism makes vectors an essential tool for C++ developers, especially when working with collections of unknown size that can grow or shrink at runtime.
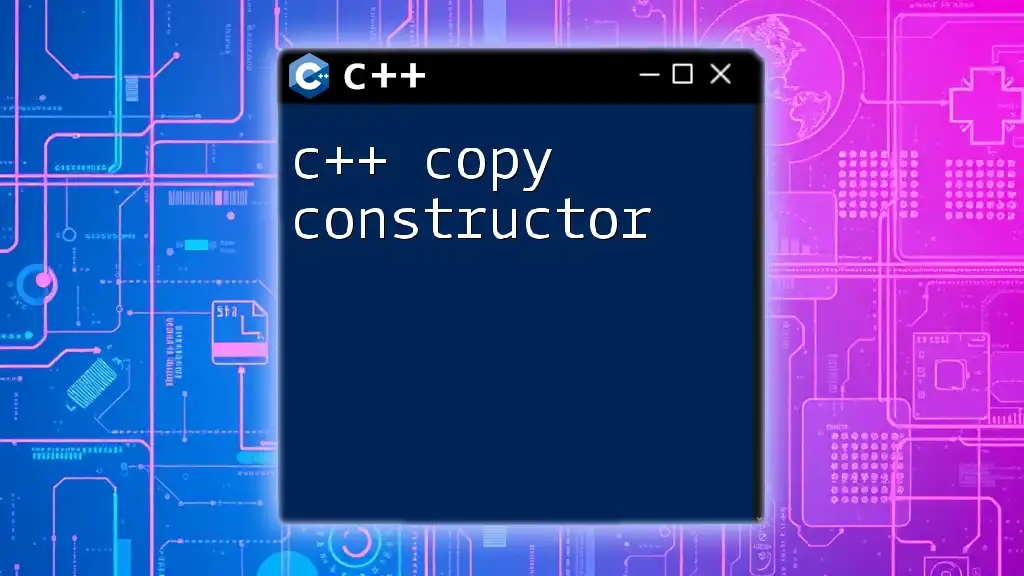
Why Use Vector Constructors?
Vector constructors come into play when you want to create and initialize vectors efficiently. They allow you to customize the initialization of your vector, providing versatility and ease of use. Vectors handle memory management automatically, which helps avoid common pitfalls associated with manual memory management, such as memory leaks or buffer overflows.
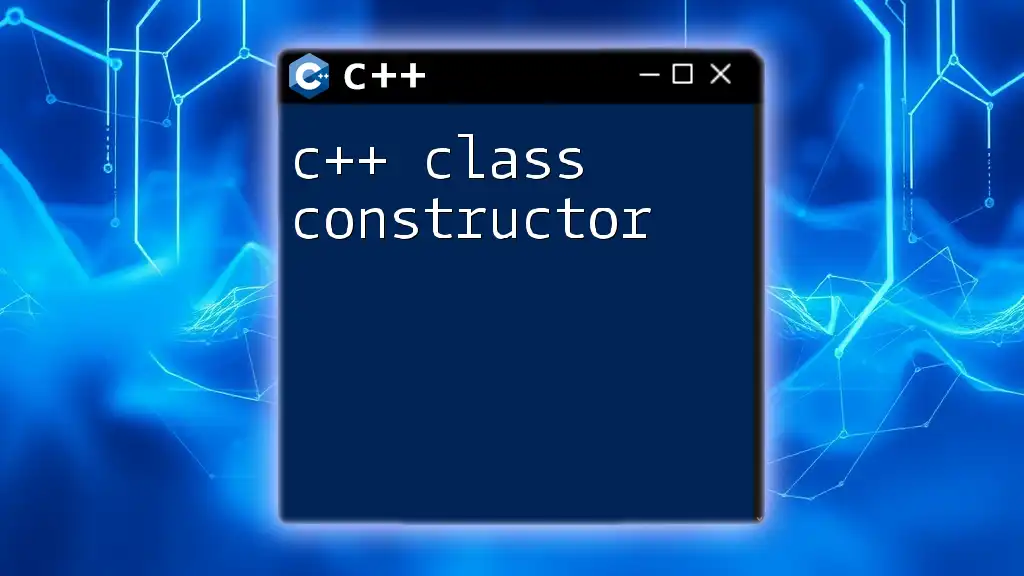
Understanding Vector Constructors in C++
What is a Constructor?
Constructors are special member functions that automatically get called when an object of a class is instantiated. They prepare the object for use, initializing member variables and allocating resources. For vectors, constructors enable you to define how a vector should behave upon creation.
Types of Vector Constructors
Default Constructor
The default constructor initializes an empty vector with zero elements. This is useful when you know that you'll be populating the vector later.
std::vector<int> myVector;
In this example, `myVector` is created but contains no elements initially. You can then append elements to it using methods like `push_back()`.
Fill Constructor
The fill constructor allows you to create a vector of a specified size, with each element set to a specific value. This is particularly useful for initializing a vector when you have a predetermined number of identical elements.
std::vector<int> myVector(5, 10); // Creates a vector of size 5, initialized with 10
In this example, `myVector` consists of five elements, all set to 10. Such explicit initialization simplifies the code.
Range Constructor
The range constructor initializes a vector based on a range of elements from another container, like another vector or an array. This is highly beneficial for copying or merging datasets.
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> myVector(source.begin(), source.end());
Here, `myVector` is filled with elements from the `source` vector, providing a convenient way to replicate existing data.
Copy Constructor
The copy constructor creates a new vector as a copy of an existing one, allowing you to duplicate its size and contents. It's crucial to understand the difference between shallow and deep copies, especially with dynamic data.
std::vector<int> myVector2(myVector); // Creates a copy of myVector
In this example, `myVector2` is an exact duplicate of `myVector`. Using the copy constructor ensures that you have a distinct instance with its own memory allocation.
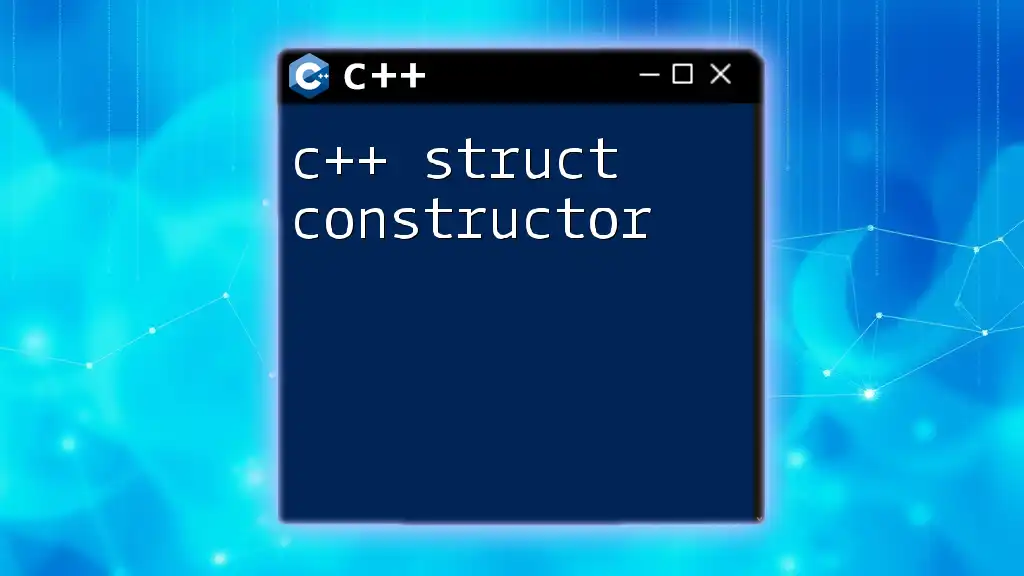
Deep Dive into Each Constructor Type
Default Constructor
When to Use: Utilize the default constructor when the immediate size of the vector is unknown or unnecessary. This is especially relevant in algorithms where elements are added progressively based on conditions.
std::vector<std::string> names; // Start with an empty vector
names.push_back("Alice");
names.push_back("Bob");
In the example above, names can grow dynamically as needed, allowing for efficiency and adaptability in your code.
Fill Constructor
Use Cases: Situations where a specific number of identical elements is required make the fill constructor exceptionally practical. This can come in handy for initializing states in simulations or creating constant data buffers.
std::vector<char> charVector(10, 'A'); // Vector of 10 'A's
In this instance, creating a vector filled with the same character simplifies future manipulations.
Range Constructor
Use Cases: Leverage the range constructor when you wish to copy and transform elements from existing containers. This can lead to cleaner code and reduce the chance of errors when managing datasets.
std::vector<int> evenNumbers = {2, 4, 6, 8, 10};
std::vector<int> myVector(evenNumbers.begin(), evenNumbers.end());
By initializing `myVector` with the elements from `evenNumbers`, you maintain an effective and efficient method of data handling.
Copy Constructor
Understanding Ownership in C++: The copy constructor is essential in C++ to handle instances where deep copies may be necessary. It ensures that if the original vector is modified, the copy remains unaffected, thus keeping data integrity intact.
std::vector<int> original {1, 2, 3};
std::vector<int> copyVector(original); // New vector with a copy of original
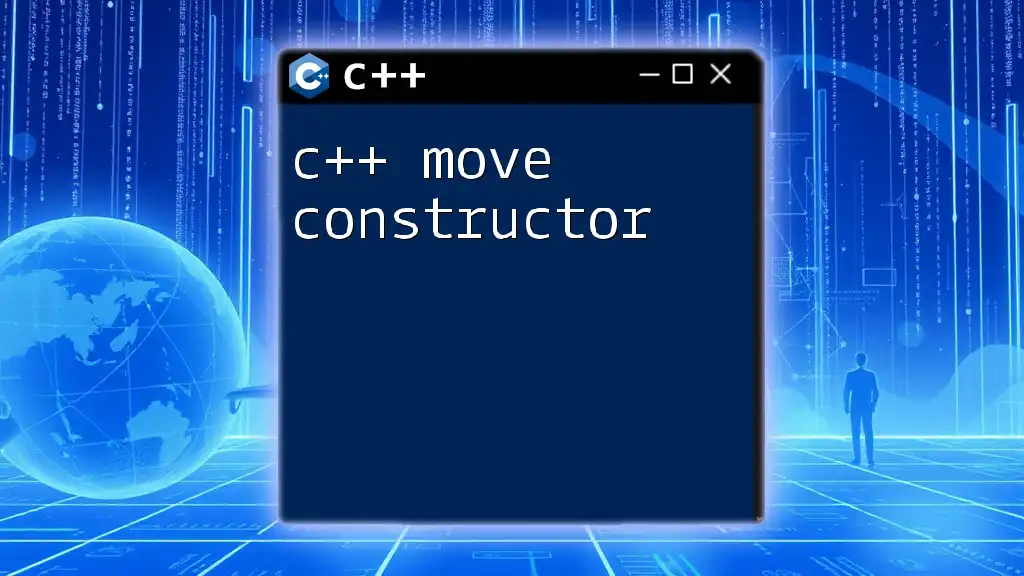
Important Characteristics of C++ Vectors
Memory Management
Vectors manage memory automatically, growing and shrinking as needed. When a vector's current size exceeds its capacity, it reallocates memory to accommodate additional elements. This internal resizing mechanism ensures that vectors maintain performance while providing easier memory management.
Performance Considerations
Vectors offer varying time complexities for different operations, such as:
- O(1) for access by index.
- O(n) for insertion and deletion at arbitrary positions.
Being aware of these complexities helps with optimizing the code and enhances performance.
Capacity vs Size
Understanding the capacity (the amount of space allocated) versus the size (the current number of elements) is crucial when managing vectors. Using `reserve()` can help allocate enough memory beforehand, minimizing the need for repeated reallocations.
std::vector<int> myVector;
myVector.reserve(100); // Pre-allocate memory for 100 elements
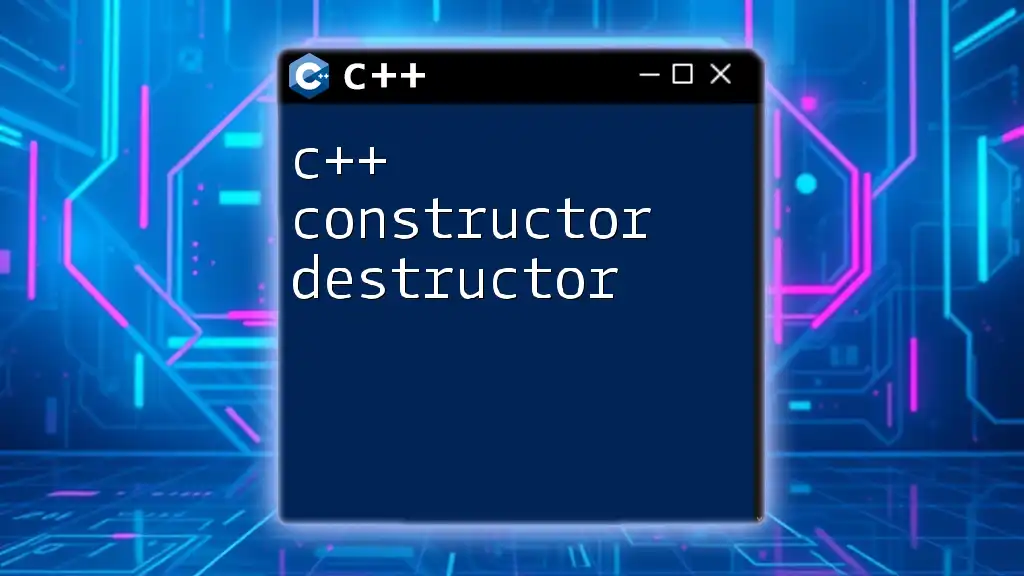
Common Pitfalls and Best Practices
Avoiding Unintentional Copies
While deep copying is sometimes necessary, it can also lead to performance issues. Utilize references or pointers when passing vectors to functions to avoid unintended copies.
Vector Initialization
Always initialize vectors properly using appropriate constructors to avoid undefined behaviors. This helps prevent runtime errors and ensures that your data structures are in a known state.
Always Think About Capacity
When working with large datasets, it’s good practice to manage capacity efficiently. Regularly check and set capacities using `reserve()` to enhance performance when you know the expected size of your vectors in advance.
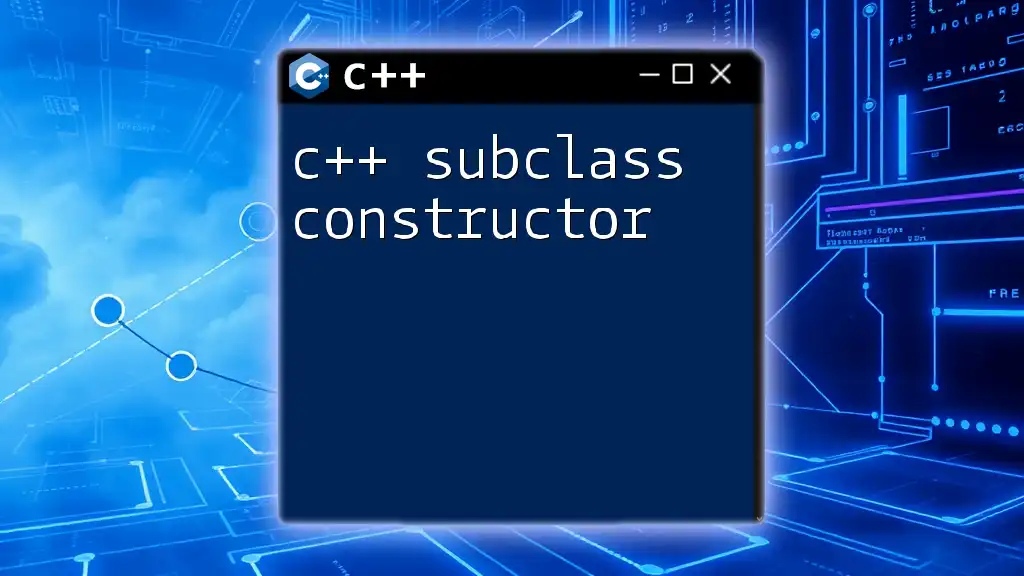
Conclusion
Understanding the nuances of the C++ vector constructor is vital for any developer dealing with dynamic data management. Utilizing the various constructors effectively can significantly improve code performance and readability. Experimenting with these constructors in practical applications will solidify your grasp of C++ vectors and empower you to write cleaner and more efficient C++ code.
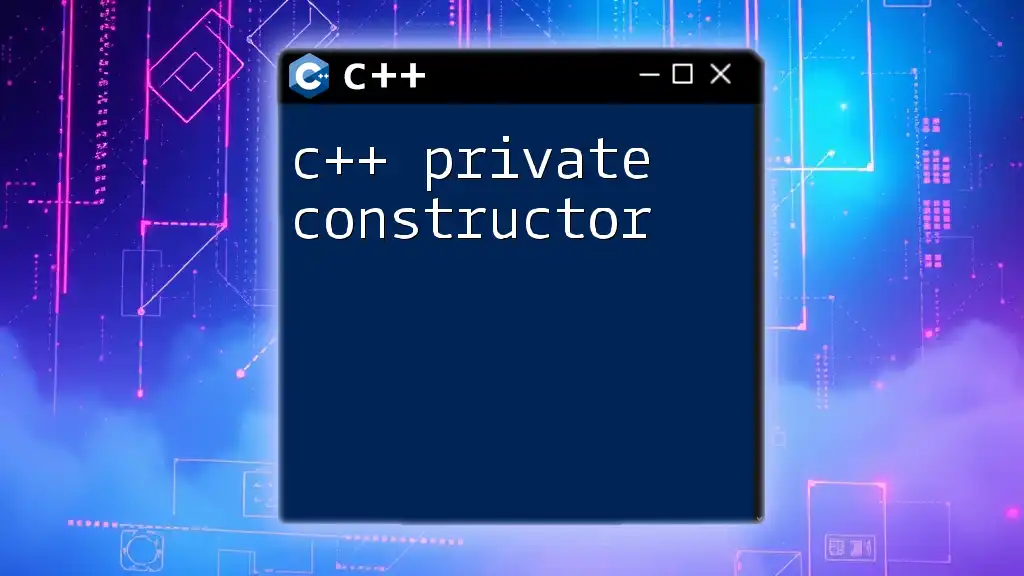
Additional Resources
For a deeper understanding of C++ STL vectors, explore tutorials, documentation, and community forums that discuss both basic usage and advanced techniques.
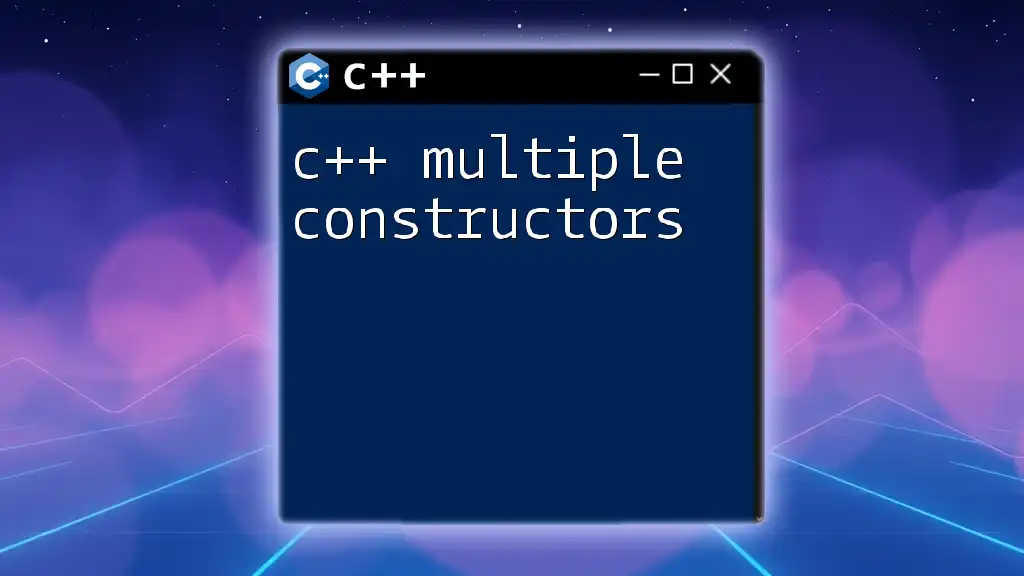
FAQs
What is the default initial value of a vector?
When initialized using the default constructor, vectors contain no elements; their size is zero.
Can a vector store different data types?
Vectors in C++ are designed to hold elements of a single data type. However, utilizing a `std::variant` or `std::any` can allow for heterogeneous collections.
What happens when a vector exceeds its allocated capacity?
When a vector’s size surpasses its capacity, it automatically reallocates memory to accommodate additional elements, ensuring that the vector continues to function properly.