In C++, a vector of strings is a dynamic array that can store multiple string elements, allowing for easy management and manipulation of string data. Here's a simple example:
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> fruits = {"Apple", "Banana", "Cherry"};
for (const auto& fruit : fruits) {
std::cout << fruit << std::endl;
}
return 0;
}
What is a String in C++?
A string in C++ is represented by the `std::string` class, part of the Standard Template Library (STL). Unlike C-style strings, which are essentially arrays of characters terminated by a null character, `std::string` offers flexibility and a host of member functions for easier manipulation.
Characteristics of `std::string`:
- Automatically manages memory allocation for the string's content.
- Provides utility functions to compare, concatenate, search, and manipulate strings.
- Supports dynamic resizing, allowing strings to grow or shrink as needed.
Common use cases for strings include reading input from users, text processing, and constructing messages for output.
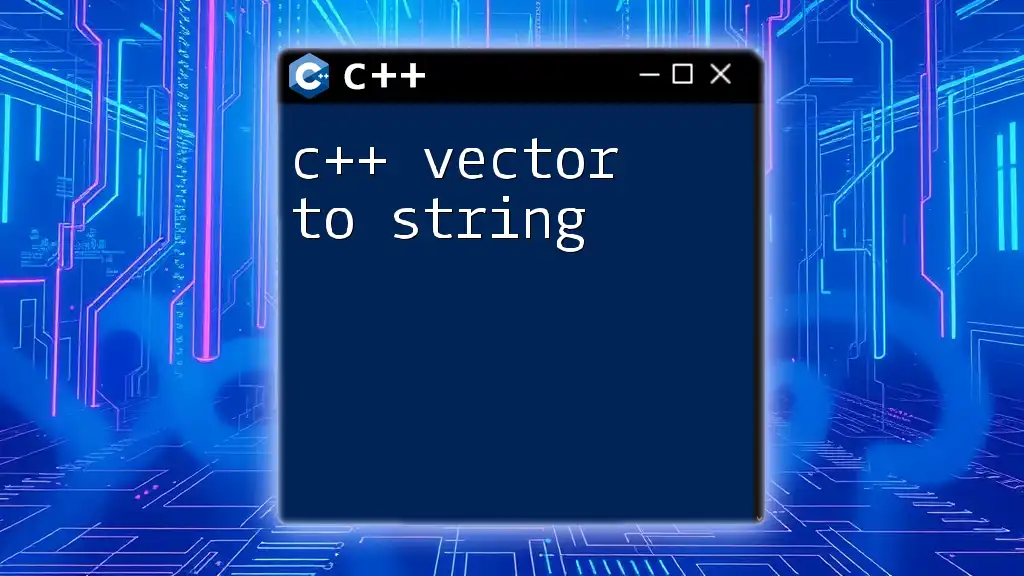
Understanding Vectors in C++
A vector in C++ is a dynamic array provided by `std::vector`, which can change its size as elements are added or removed. Unlike traditional arrays, vectors manage memory automatically, ensuring that developers do not run into the problems of buffer overflow or memory waste.
Advantages of using vectors over arrays include:
- Dynamic resizing: Vectors can grow and shrink in response to the number of elements.
- Rich member functions: Vectors come with built-in functions to manipulate data easily, such as adding, removing, and accessing elements.
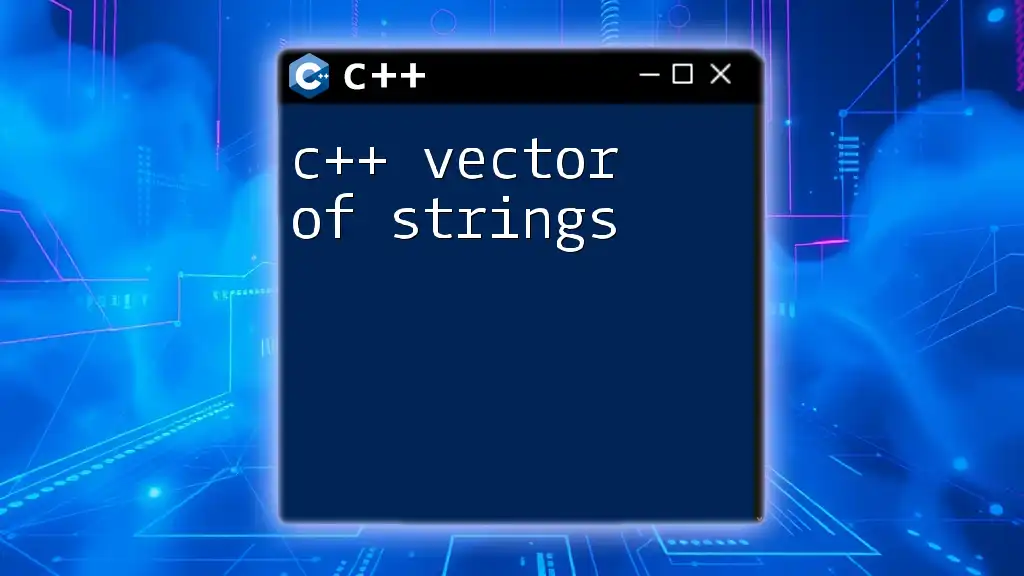
The C++ String Vector: Overview
So, what exactly is a string vector in C++? A string vector is an instantiation of `std::vector` specifically designed to store multiple strings. This powerful data structure allows developers to manage a collection of strings dynamically.
Using a string vector (`std::vector<std::string>`) can be incredibly useful in situations where the number of strings is not known beforehand, such as user inputs or reading lines of text from a file.
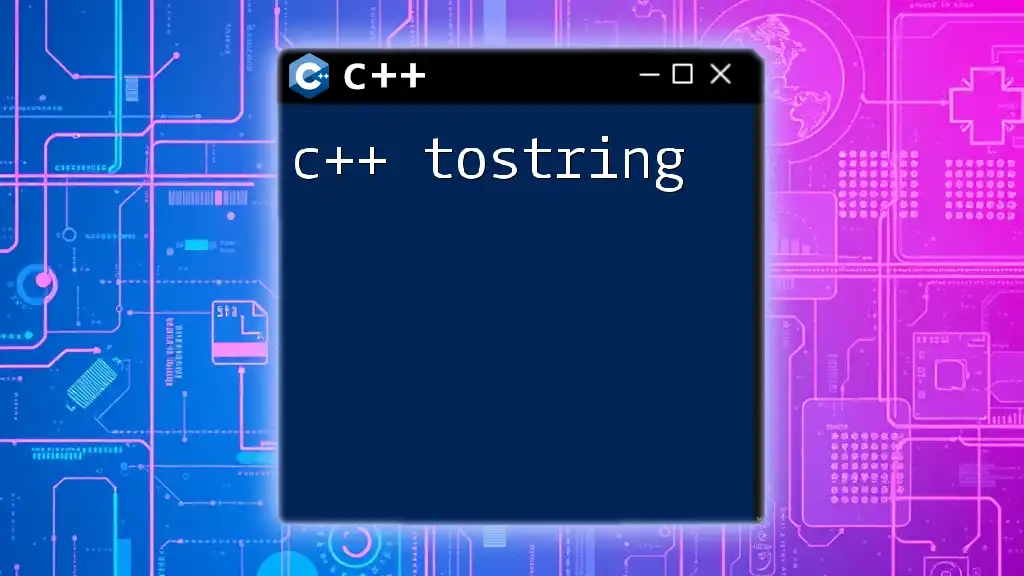
Creating a C++ String Vector
Declaring a String Vector
To declare a string vector, you can use the following syntax:
std::vector<std::string> myStringVector;
This code creates an empty string vector named `myStringVector`.
C++ allows for more versatile declarations, such as initializing a vector with pre-defined values:
std::vector<std::string> fruits = {"apple", "banana", "cherry"};
Initializing a String Vector
Initializing a string vector can be done through various methods. You can use an initializer list, which can be seen in the previous example. Additionally, you can add elements manually using the `push_back()` method:
myStringVector.push_back("grape");
This code appends the string "grape" to the end of the `myStringVector`.
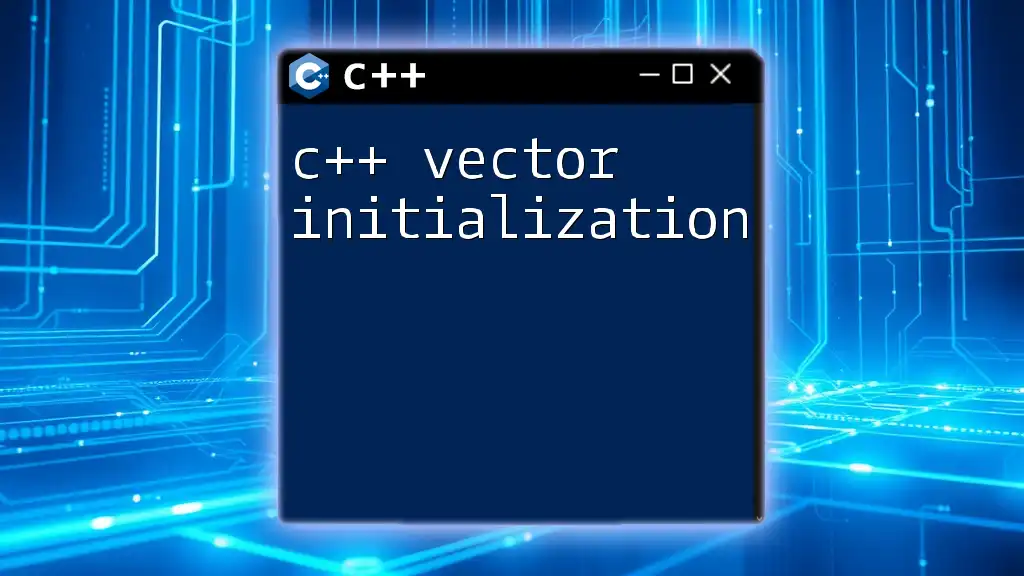
Manipulating a C++ String Vector
Adding Elements to a String Vector
To add new strings to a string vector, you can use either `push_back` or `emplace_back`.
- Using `push_back`:
myStringVector.push_back("orange");
- Using `emplace_back`:
myStringVector.emplace_back("kiwi");
While both methods effectively add elements to the vector, `emplace_back` can be more efficient in certain scenarios as it constructs the object in-place.
Accessing Elements in a String Vector
You can access elements in a string vector using the `at()` function or the subscript operator.
- Using `at()`:
std::string firstFruit = myStringVector.at(0);
- Using the subscript operator:
std::string secondFruit = myStringVector[1];
Using `at()` is preferable for safety, as it performs bounds checking, unlike the subscript operator, which may result in undefined behavior if the index is out of range.
Modifying Elements in a String Vector
Once you've populated your string vector, you may want to modify existing elements. For example:
myStringVector[1] = "blueberry"; // Changing "banana" to "blueberry"
This simple line of code updates the second element in the vector.
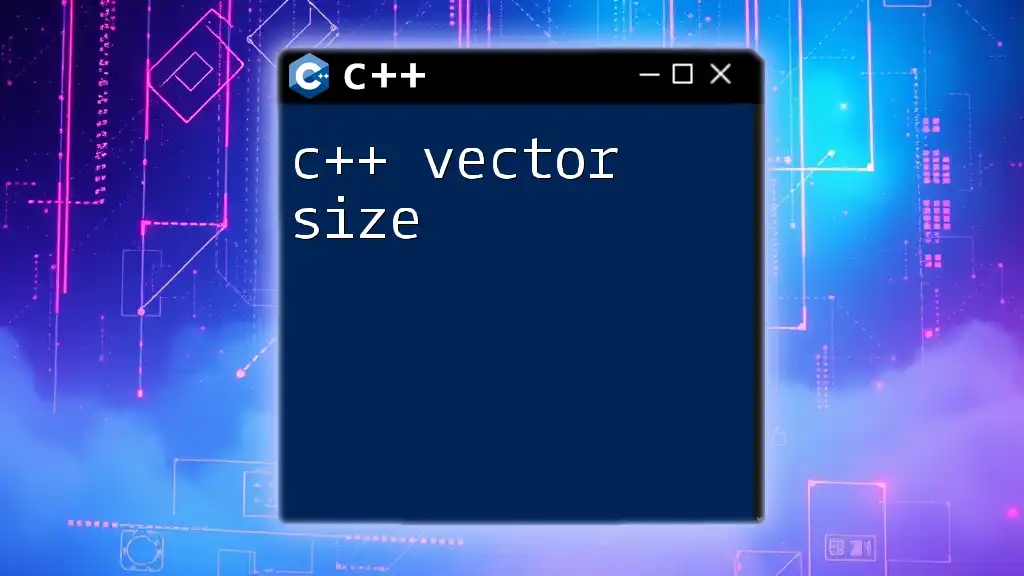
Common Operations on C++ String Vectors
Iterating Over a String Vector
Iterating over a string vector can be done using several methods, including range-based for loops and traditional for loops:
- Range-based for loop:
for (const auto& fruit : myStringVector) {
std::cout << fruit << std::endl;
}
- Traditional for loop:
for (size_t i = 0; i < myStringVector.size(); ++i) {
std::cout << myStringVector[i] << std::endl;
}
Both methods effectively allow you to access each element within the string vector, though range-based loops tend to be cleaner and more concise.
Sorting a String Vector
To sort the strings in a vector, you can use the `std::sort` algorithm:
#include <algorithm> // For std::sort
std::sort(myStringVector.begin(), myStringVector.end());
This line sorts the `myStringVector` in alphabetical order.
Removing Elements from a String Vector
Removing elements can be undertaken using the `erase` method combined with the iterator or index:
myStringVector.erase(myStringVector.begin() + 1); // Removes the second element
Alternatively, if you know the value you want to remove:
myStringVector.erase(std::remove(myStringVector.begin(), myStringVector.end(), "kiwi"), myStringVector.end());
This code snippet removes all instances of "kiwi" from the vector.
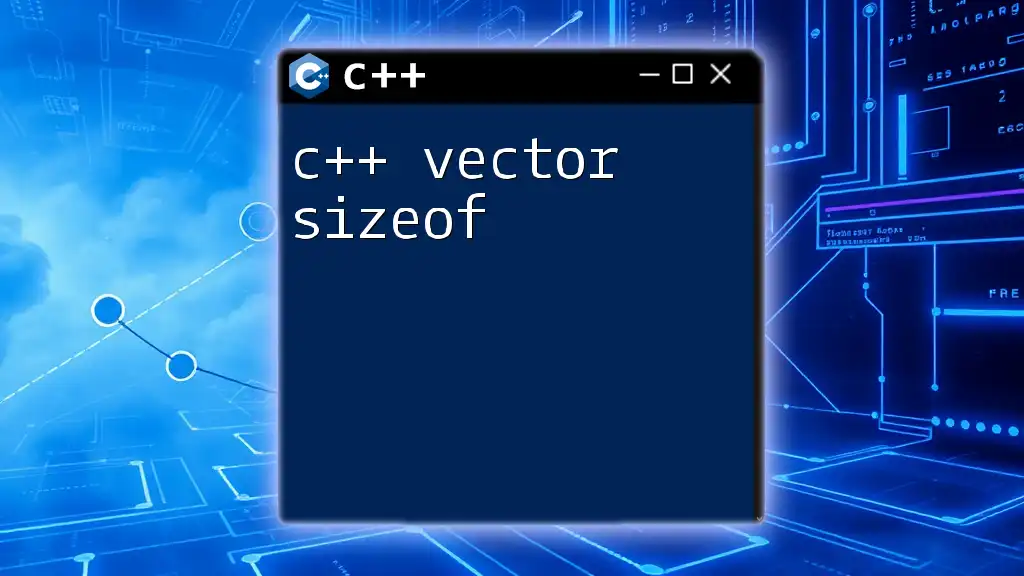
Advanced Usage of C++ String Vectors
Using String Vectors with Functions
Passing a string vector to functions in C++ can enable more modular code. For instance, a function can modify a string vector as shown below:
void appendFruit(std::vector<std::string> &fruits, const std::string &newFruit) {
fruits.push_back(newFruit);
}
Combining and Splitting Strings in a Vector
Combining strings can be easily accomplished using the `std::ostringstream`:
#include <sstream>
std::ostringstream combinedFruits;
for (const auto& fruit : myStringVector) {
combinedFruits << fruit << ", ";
}
// Remove the last comma and space
std::string result = combinedFruits.str();
result = result.substr(0, result.size() - 2);
Splitting a string into a vector of strings can be done using `std::stringstream`:
#include <sstream>
#include <vector>
std::string input = "apple,banana,cherry";
std::vector<std::string> splitFruits;
std::stringstream ss(input);
std::string item;
while (std::getline(ss, item, ',')) {
splitFruits.push_back(item);
}
This approach enables taking a single string containing lists of items and transforming it into a vector for more manageable manipulation.
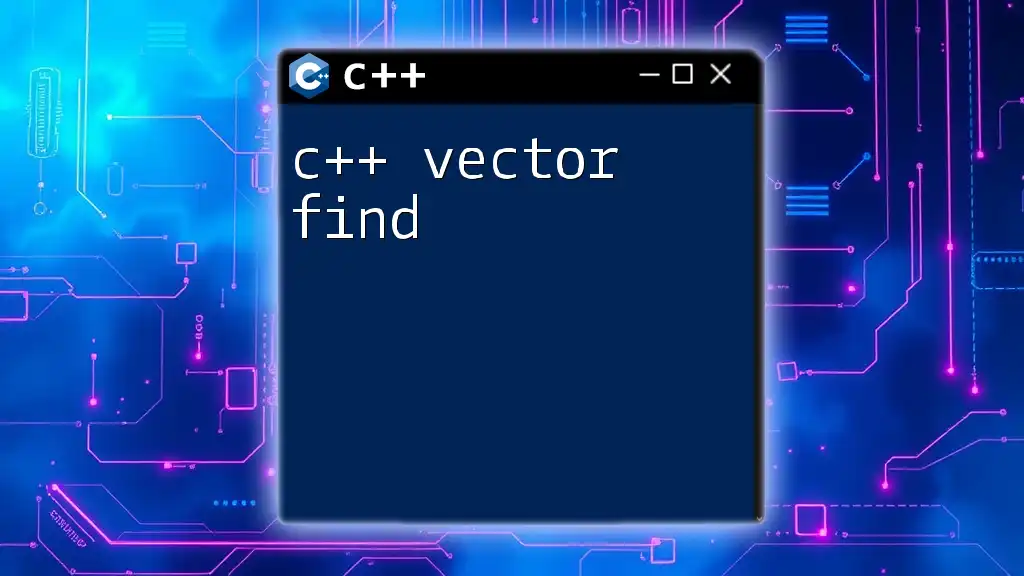
Performance Considerations
When to Use String Vectors vs. Arrays
Choosing between a string vector and an array often depends on the application's requirements. String vectors are dynamic and provide more flexibility in terms of data management, making them ideal for cases where lengths of data sets are uncertain. On the other hand, arrays may still be preferable when dealing with fixed-size collections for performance efficiencies due to their simpler memory allocation and quick access.
Ultimately, the decision must consider memory management and specific performance needs relative to the complexity of operations expected in software applications.
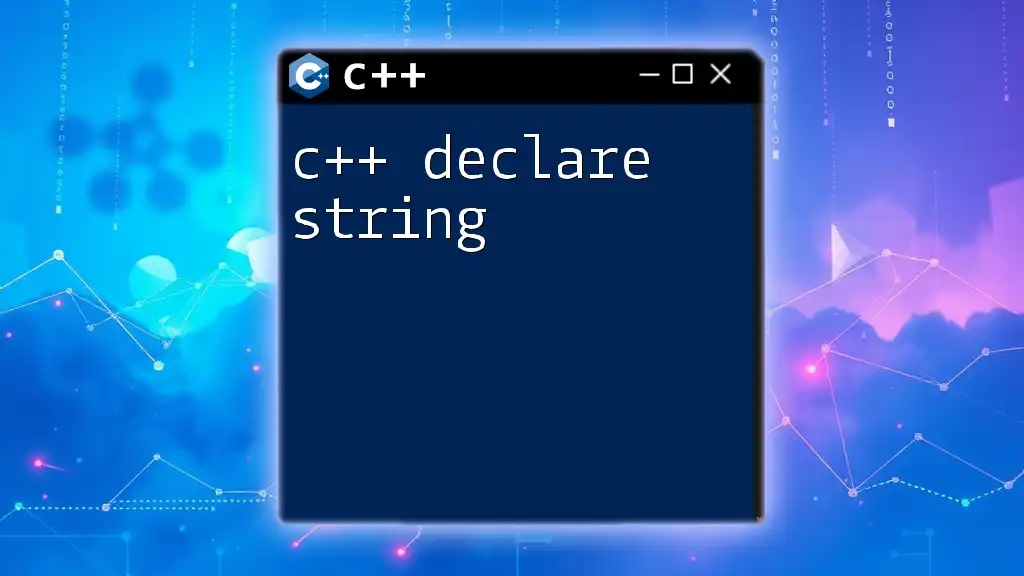
Recap of Key Points
Throughout this article, we explored the essentials of C++ vector strings, addressing how to create, manipulate, and perform operations on `std::vector<std::string>`. Understanding these concepts lays the foundation for efficient data management in C++ applications.
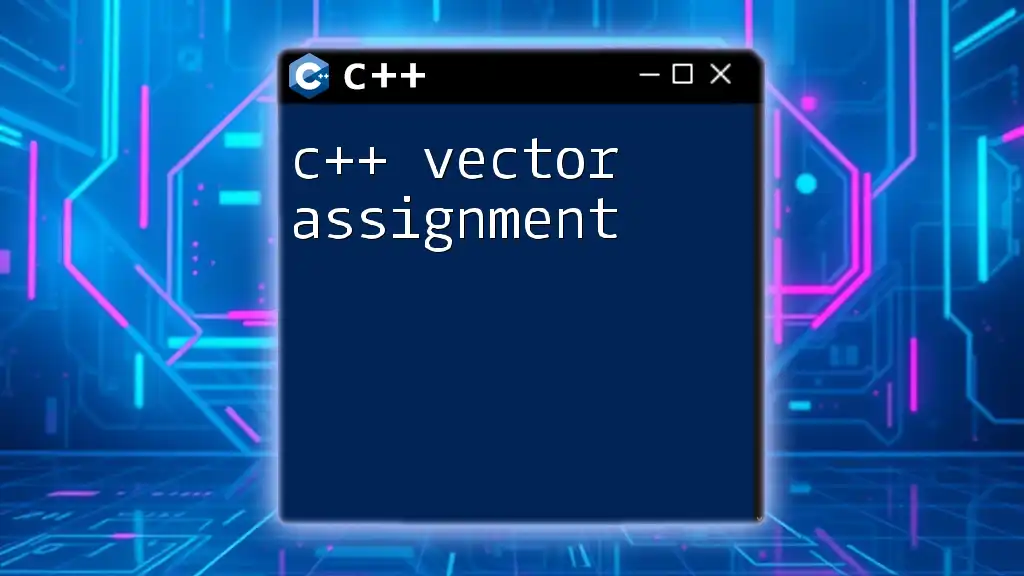
Encouragement for Further Learning
To delve deeper into the world of C++ and enhance your programming skills, consider exploring additional resources and tools that facilitate learning about data structures, algorithms, and other advanced C++ concepts.
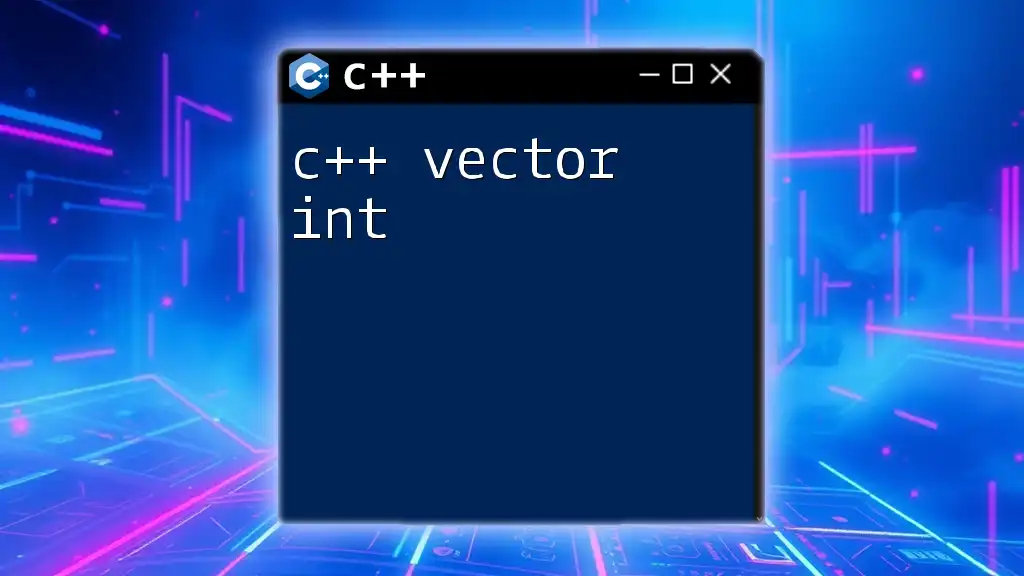
Join Our Courses
If you're excited to improve your C++ proficiency and gain confidence in using vector strings and other commands quickly and concisely, join our courses today! Embrace the power of streamlined learning and elevate your programming career!