`std::string` is a part of the C++ Standard Library that provides a flexible way to manage and manipulate sequences of characters. Here's a simple example:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding the Basics of `std::string`
Characteristics of `std::string`
The `std::string` class in C++ is a part of the Standard Template Library (STL). It provides a convenient and robust way to handle strings compared to traditional C-style strings. One of the primary advantages of `std::string` is that it automatically manages memory, which can prevent potential errors like buffer overflow that commonly happen with `char[]` arrays.
Creating and Initializing `std::string`
Default Constructor:
You can declare a string using the default constructor:
std::string str;
This creates an empty string, which can be useful if you plan to assign data later.
Using C-Strings:
Initializing a `std::string` with a C-style string is straightforward:
std::string str = "Hello, World!";
This line converts the literal C-string to an `std::string` object seamlessly.
String with Size:
Creating a string containing repeated characters can be accomplished as follows:
std::string str(5, 'A'); // "AAAAA"
In this case, a string consisting of five 'A' characters is created. This feature is especially useful for padding or initializing strings with default characters.
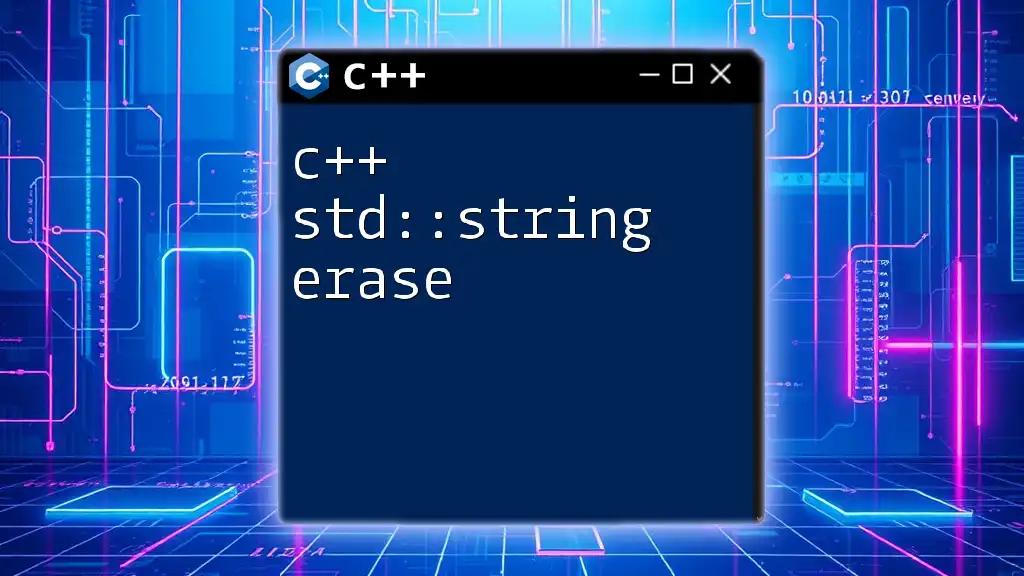
Manipulating `std::string`
Basic Operations
Assignment
You can assign strings with the assignment operator:
std::string str1 = "Hello";
std::string str2 = str1; // Copy assignment
This performs a deep copy of `str1` into `str2`, allowing both strings to operate independently in memory.
Concatenation
Concatenating strings in C++ is simple using the `+` operator:
std::string str1 = "Hello";
std::string str2 = "World!";
std::string result = str1 + " " + str2; // "Hello World!"
This expression effectively joins `str1`, a space, and `str2` to form a new string.
Accessing Characters
Indexing and `at()`
Accessing characters in an `std::string` can be done using either indexing or the `at()` method:
std::string str = "Hello";
char c1 = str[0]; // 'H'
char c2 = str.at(1); // 'e'
While both methods serve the same purpose, `at()` provides bounds checking, throwing an out_of_range exception if the index is invalid, thus enhancing safety.
Modifying Strings
Inserting and Appending
To add content to an `std::string`, use the `insert()` and `append()` methods.
Insert Function:
std::string str = "Hello";
str.insert(5, " World"); // "Hello World"
In this snippet, `" World"` is inserted at index 5, right after `Hello`.
Append Function:
std::string str = "Hello";
str.append(" World!"); // "Hello World!"
The `append` method concatenates the provided string to the end of the original string.
Erasing and Replacing
You might want to modify existing content in a string using the `erase` and `replace` functions.
Erase Function:
std::string str = "Hello World!";
str.erase(5, 6); // "Hello!"
Here, " World!" is removed starting at index 5, reducing the string to "Hello!".
Replace Function:
std::string str = "Hello World!";
str.replace(6, 5, "C++"); // "Hello C++!"
This modifies the string by replacing "World" (5 characters) with "C++".

Searching and Substring Operations
Finding Substrings
To locate a substring within a string, the `find()` function is highly effective:
std::string str = "Hello World!";
size_t found = str.find("World"); // Returns position of "World"
The `find()` function returns the index of the first occurrence of the substring or `std::string::npos` if not found.
Substring Extraction
You can extract a substring using `substr()`:
std::string str = "Hello World!";
std::string substr = str.substr(6, 5); // "World"
This creates a new string comprised of 5 characters starting at index 6, returning "World".

String Comparison
Comparing Strings
C++ offers several methods to compare strings effectively.
Using Comparison Operators:
std::string str1 = "Hello";
std::string str2 = "World";
bool isEqual = (str1 == str2);
This will result in `isEqual` being `false`, as the strings do not match.
Using the `compare()` Method:
std::string str1 = "Hello";
std::string str2 = "Hello";
int result = str1.compare(str2); // 0 if equal
The `compare()` function returns an integer: 0 for equality, less than 0 if `str1` is less than `str2`, and greater than 0 if it's greater.

Working with String Streams
Introduction to `std::stringstream`
The `std::stringstream` class allows for input and output operations on strings, providing great utility in typesetting and converting data formats.
Using String Streams for Input and Output
Input Operations:
std::stringstream ss;
ss << "Age: " << 25;
std::string info = ss.str(); // "Age: 25"
In this case, you can easily build a string with various data types.
Output Operations:
std::stringstream ss("3.14");
double number;
ss >> number; // 3.14
This code reads from a string stream and converts it to a numeric type—in this case, a double.

Advanced Techniques with `std::string`
Understanding Memory Management
A major reason to prefer `std::string` is its automatic memory management. String objects manage their own memory, dynamically resizing as needed. This significantly reduces the risk of memory-related errors that can occur with raw character arrays.
Performance Considerations
When assessing string performance, `std::string` typically outperforms C-style strings for most operations due to its built-in functionalities. However, in performance-critical applications, you may need to consider alternatives like `std::vector<char>` or `std::array<char, N>` when you require direct control over memory allocation and deallocation.

Conclusion
Mastering `std::string` in C++ is essential for any developer looking to manipulate text efficiently and safely. By leveraging its capabilities, understanding its operations, and adhering to best practices, you can significantly improve your coding productivity and program robustness. The next step is to practice through coding challenges to cement your understanding. For further exploration, refer to the official C++ documentation and consider books dedicated to mastering C++ strings.