In C++, you can find a substring within a string using the `find()` method, which returns the index of the first occurrence of the substring or `std::string::npos` if it is not found.
Here’s a simple example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = "World";
size_t found = str.find(subStr);
if (found != std::string::npos) {
std::cout << "Substring found at index: " << found << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a String?
In C++, a string is a sequence of characters that can be manipulated as a single data type. The C++ Standard Library provides the `std::string` class for working with strings, making it user-friendly compared to traditional character arrays. Strings in C++ are mutable, meaning you can change their content even after creation, and their size can dynamically grow or shrink as needed.
Basic String Operations
Before diving into exploring C++ substring find functionalities, it’s important to familiarize yourself with some essential string operations. Here’s a summary of fundamental actions you can perform on strings:
- Concatenation: The combination of two strings into one.
- Length: Getting the number of characters in a string.
- Comparison: Checking if two strings are equal or if one is greater than another.
For instance, you can easily create and manipulate strings like this:
#include <iostream>
#include <string>
int main() {
std::string hello = "Hello";
std::string world = "World!";
std::string greeting = hello + " " + world; // Concatenation
std::cout << greeting << " Length: " << greeting.length() << std::endl; // Length
return 0;
}
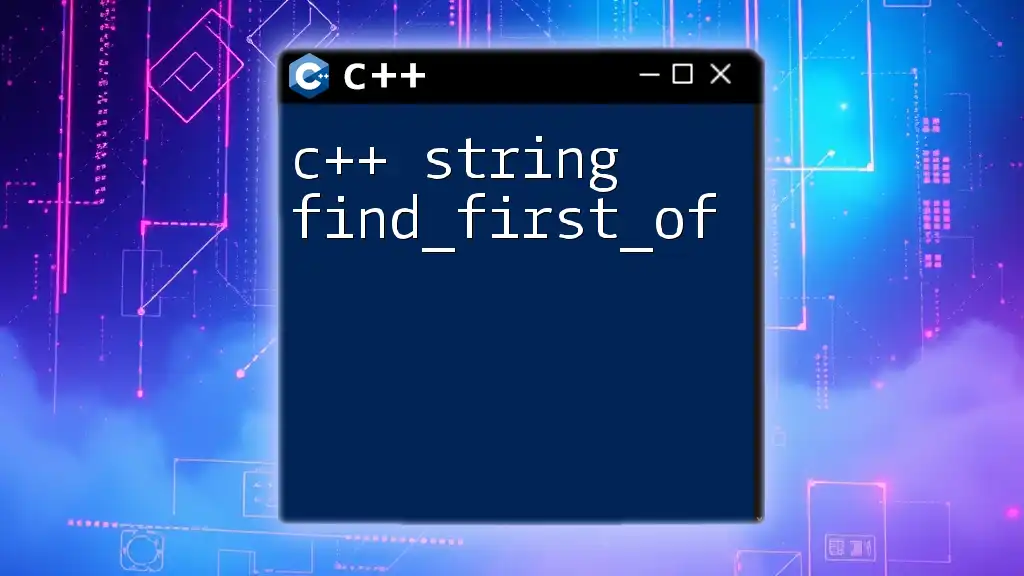
Finding Substrings in C++
Introduction to Substrings
A substring is a contiguous sequence of characters within a string. Being able to find substrings is crucial for tasks such as text parsing, searching for keywords, and data validation. This is where the C++ substring find functions come into play.
Using `std::string::find`
Syntax of `find()`
The `find()` method is a member of the `std::string` class and allows you to search for a substring within a string. Its basic syntax is as follows:
size_t find(const std::string& str, size_t pos = 0) const noexcept;
- Parameters:
- `str`: The substring you want to search for.
- `pos`: The position from which to start searching (default is 0).
Return Values and Behavior
The method returns the index of the first occurrence of the substring if found. If the substring is not found, it returns `std::string::npos`, a constant representing an invalid position.
Here’s an example of using `find()`:
#include <iostream>
#include <string>
int main() {
std::string text = "Welcome to the world of C++ programming!";
size_t position = text.find("world");
if (position != std::string::npos) {
std::cout << "Found 'world' at position: " << position << std::endl;
} else {
std::cout << "'world' not found" << std::endl;
}
return 0;
}
Examples of Using `find()`
Simple Substring Search
You can use the `find()` function to quickly search for a substring, returning its position. Here’s how you can implement a simple substring search:
#include <iostream>
#include <string>
int main() {
std::string phrase = "Learning C++ is fun!";
size_t pos = phrase.find("fun");
std::cout << (pos != std::string::npos
? "'fun' found at index " + std::to_string(pos)
: "'fun' not found") << std::endl;
return 0;
}
Finding Multiple Occurrences
Finding only the first occurrence may not suffice; sometimes, you need to locate all instances of a substring. You can achieve this by using a loop:
#include <iostream>
#include <string>
int main() {
std::string text = "abcabcabc";
std::string to_find = "abc";
size_t pos = text.find(to_find);
while (pos != std::string::npos) {
std::cout << "Found '" << to_find << "' at index " << pos << std::endl;
pos = text.find(to_find, pos + 1); // continues to find the next occurrence
}
return 0;
}
Case Sensitivity in Searches
By default, `find()` is case-sensitive, meaning that "abc" is different from "ABC". If you want to perform a case-insensitive search, you'll need to convert both strings to the same case before searching:
#include <iostream>
#include <string>
#include <algorithm>
std::string toLower(const std::string& str) {
std::string lower_str = str;
std::transform(lower_str.begin(), lower_str.end(), lower_str.begin(), ::tolower);
return lower_str;
}
int main() {
std::string text = "Hello World!";
std::string to_find = "world";
size_t pos = toLower(text).find(toLower(to_find));
std::cout << (pos != std::string::npos
? "'" + to_find + "' found at position: " + std::to_string(pos)
: "'" + to_find + "' not found") << std::endl;
return 0;
}
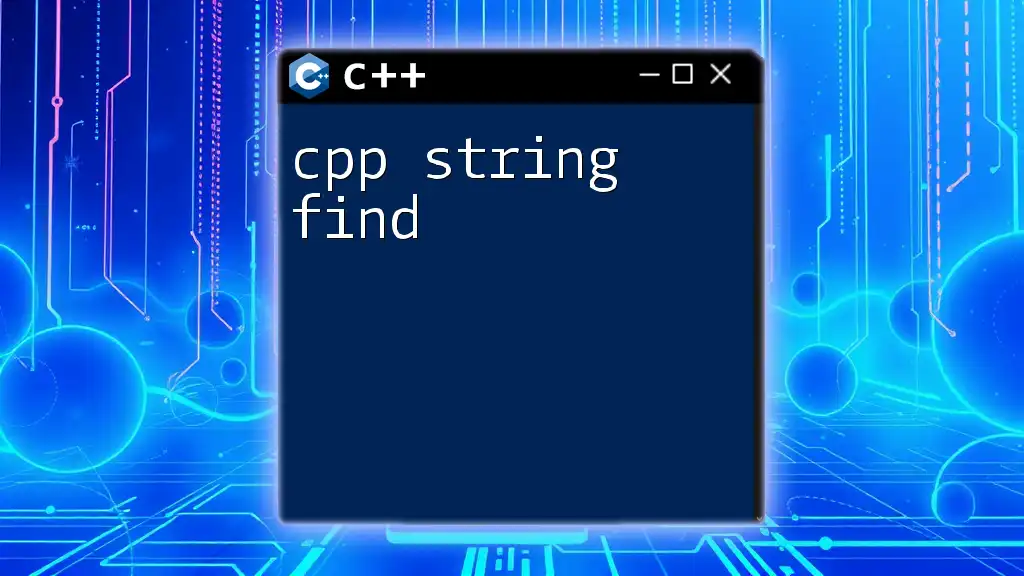
Alternative Methods for Substring Search
Using `find_first_of` and `find_last_of`
The methods `find_first_of` and `find_last_of` can be particularly useful when you need to search for the first or last occurrence of any character from a specified set.
When to Use These Functions
For instance, if you want to find the position of any vowel in a string:
#include <iostream>
#include <string>
int main() {
std::string text = "Programming is rewarding!";
std::string vowels = "aeiou";
// Finding the first occurrence of any vowel
size_t pos = text.find_first_of(vowels);
if (pos != std::string::npos) {
std::cout << "First vowel found at index: " << pos << std::endl;
}
return 0;
}
Using `std::string::rfind`
The `rfind()` function is particularly useful when you want to find the last occurrence of a substring, scanning from the end of the string backward.
Overview of `rfind`
Its syntax is similar to `find()`, and it works in the same way by returning the index of the last occurrence or `std::string::npos` if the substring isn’t found.
Example of using `rfind()`:
#include <iostream>
#include <string>
int main() {
std::string text = "Find the last occurrence of 'is' in this sentence. Is it there?";
size_t pos = text.rfind("is");
// Check if found
if (pos != std::string::npos) {
std::cout << "'is' found at index: " << pos << std::endl;
} else {
std::cout << "'is' not found" << std::endl;
}
return 0;
}
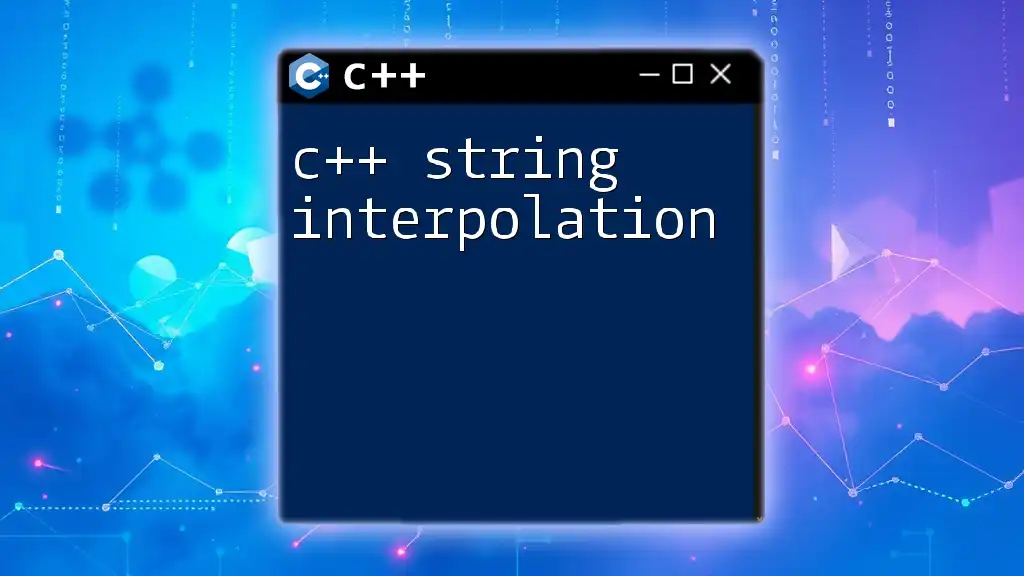
Practical Applications
Real-World Use Cases
Substring searching is commonly used in many applications, such as:
- Text Editors: Implementing features like "Find" and "Replace".
- Data Parsing: Extracting relevant data fields.
- Authentication: Validating user inputs against expected formats.
A simple example of a keyword search in a document:
#include <iostream>
#include <string>
bool keywordExists(const std::string& document, const std::string& keyword) {
return document.find(keyword) != std::string::npos;
}
int main() {
std::string doc = "This is an example document for C++ substring find.";
std::string keyword = "C++";
if (keywordExists(doc, keyword)) {
std::cout << "Keyword found!" << std::endl;
} else {
std::cout << "Keyword not found!" << std::endl;
}
return 0;
}
Performance Considerations
When working with large strings, performance can become a concern. Be mindful of the following:
- Inefficient Searches: Searching for substrings multiple times can lead to performance bottlenecks. Use `find()` wisely.
- Complexity: The time complexity of the `find()` function is generally O(n), where n is the length of the string. Keep this in mind for very large datasets, as it may impact your application's responsiveness.
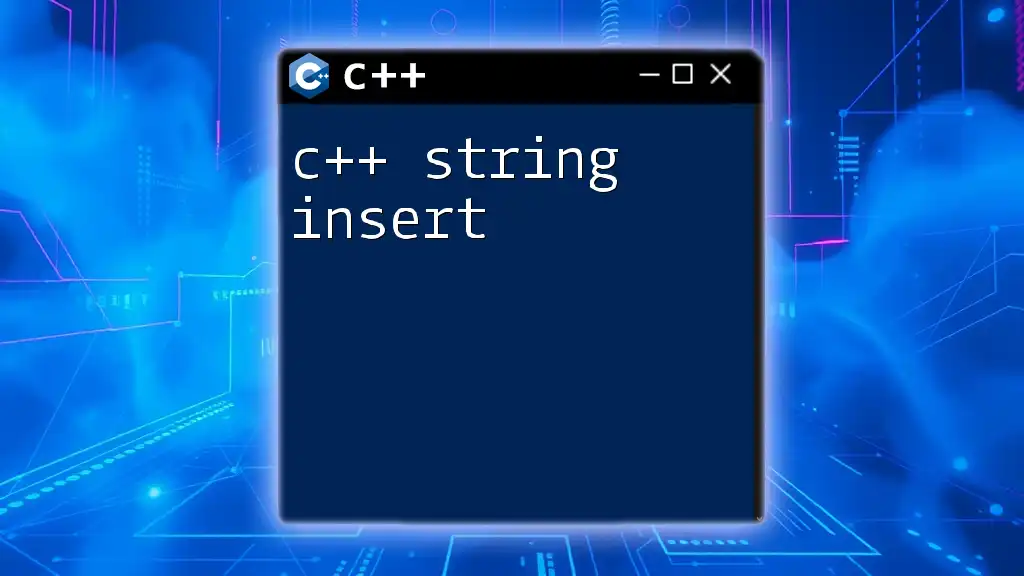
Common Errors and Troubleshooting
Debugging Common Issues
When using C++ substring find functions, common pitfalls arise, such as:
- Incorrect Indexing: Always check the return value to ensure that a valid position was found.
- Handling Different Cases: Not accounting for case sensitivity can lead to unexpected results.
Example of a common mistake:
size_t pos = text.find("example"); // Checking directly without handling return value can lead to issues.
Ensure that you always verify the existence of the substring, as shown previously using `std::string::npos`.
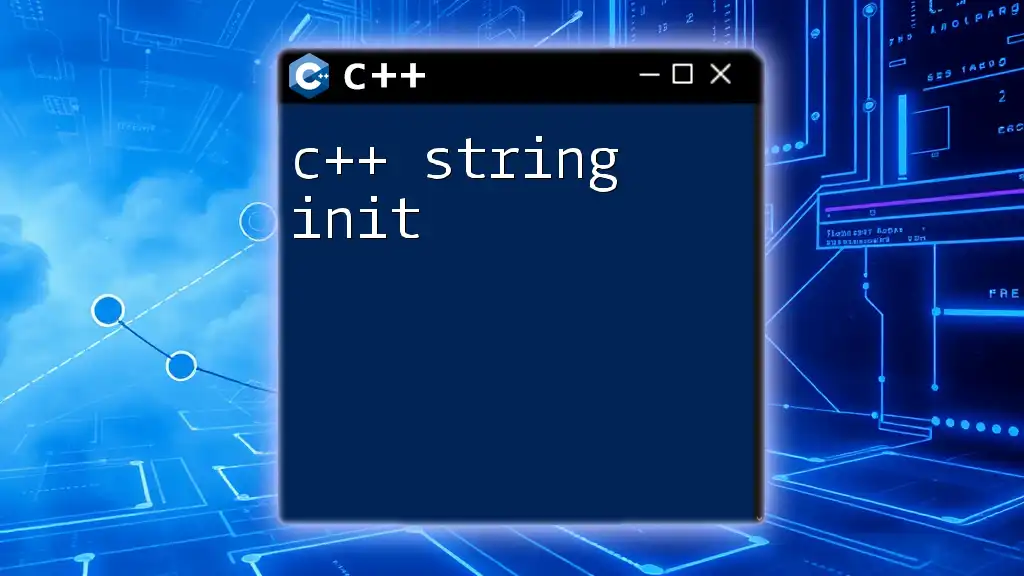
Conclusion
In summary, understanding how to effectively use substring searching in C++ is essential for any programmer working with string manipulation. The `find()`, `find_first_of()`, `find_last_of()`, and `rfind()` functions all provide powerful tools for string searching, each suited to specific scenarios.
Practice using these functions in various contexts to solidify your understanding, and don’t hesitate to experiment with the code examples provided. Happy coding!
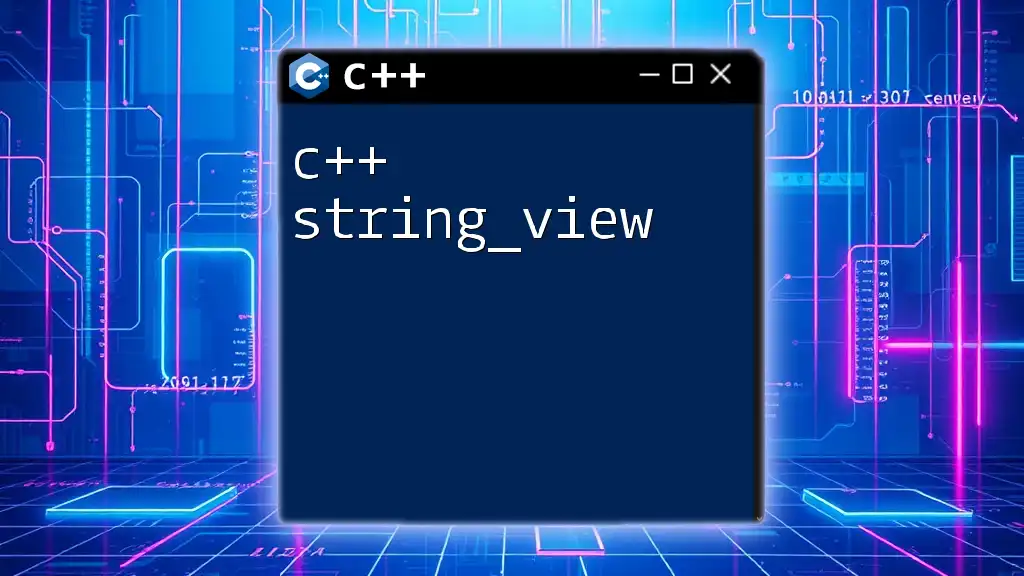
Further Reading and Resources
For those looking to deepen their knowledge of string manipulation in C++, consider exploring the official C++ documentation on `std::string`, and the wealth of resources available online, including tutorials, forums, and community discussions.