In C++, a string can be initialized using various methods such as using a string literal, constructor, or by assigning another string, as shown in the following example:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, World!"; // String literal initialization
std::string str2("Welcome to C++"); // Constructor initialization
std::string str3 = str1; // Copy initialization
std::cout << str1 << std::endl;
std::cout << str2 << std::endl;
std::cout << str3 << std::endl;
return 0;
}
Understanding Strings in C++
In C++, strings are fundamental for handling text and character sequences. The `std::string` class, part of the C++ Standard Template Library (STL), provides a sophisticated suite of functionalities that cater to string manipulations. Using `std::string` is strongly recommended over traditional C-style strings (character arrays) due to its ease of use, automatic memory management, and flexibility.
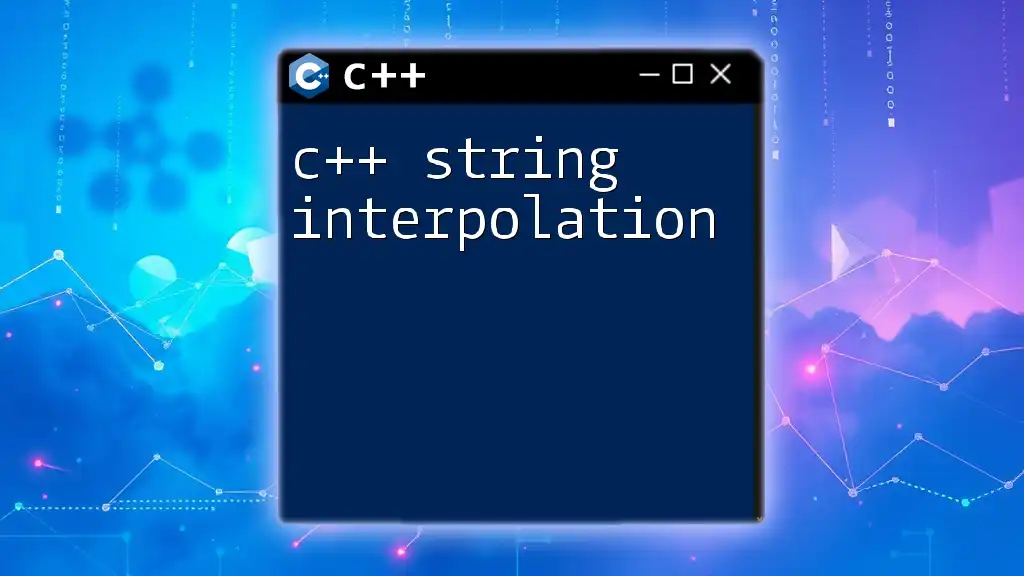
C++ String Initialization Methods
What is String Initialization?
String initialization refers to the process of setting up a string variable with an initial value. Proper initialization is crucial as it determines the state of a string at its creation and helps avoid undefined behavior during program execution.
Default Initialization
Default initialization occurs when a string variable is declared without an assigned value. The constructed string will be empty but valid.
For example:
std::string str;
In this case, `str` is initialized as an empty string (`""`). You can safely perform operations like checking its length or concatenating new content.
Initialization with a String Literal
To initialize a string with a predefined value, you can assign it a string literal directly. This method is straightforward and commonly used.
Example:
std::string str = "Hello, World!";
Here, `str` contains the text "Hello, World!". This type of initialization immediately assigns the content to the string and ensures it is properly sized.
Initialization Using a Character Array
Strings can also be initialized from a C-style character array. While this is a less common technique in modern C++, it can be useful for interoperability with legacy code.
Example:
const char* charArray = "Hello, C++!";
std::string str(charArray);
In this example, `str` uses the content of `charArray`. This method automatically handles memory allocation and copying the content, providing a C++ string object from a raw character array.
Initialization with Another String
Another common initialization method is creating a string based on an existing string. This is achieved by passing the original string as an argument to the `std::string` constructor.
Example:
std::string original = "Original String";
std::string copy(original);
The `copy` string now contains the same content as `original`. This constructor is particularly useful when you want to duplicate a string while maintaining the immutability of the original one.
Initialization with a Specific Size and Character
You can create a string of a specific length filled with a repeating character. This initialization approach is particularly valuable when you need to create placeholder strings or initialize buffers.
Example:
std::string str(10, 'A'); // Creates "AAAAAAAAAA"
In this scenario, `str` will consist of ten 'A' characters. This can be handy when setting up specific formatting or placeholder data.
Using String Constructors
The `std::string` class offers a variety of constructors that facilitate different initialization approaches. Understanding these constructors can help streamline your coding process.
Example demonstrating multiple constructors:
std::string str1("Hello");
std::string str2(5, 'B'); // = "BBBBB"
In this case, `str1` is initialized with the literal "Hello," while `str2` consists of five 'B' characters. The flexibility offered by these constructors enhances code readability and efficiency.
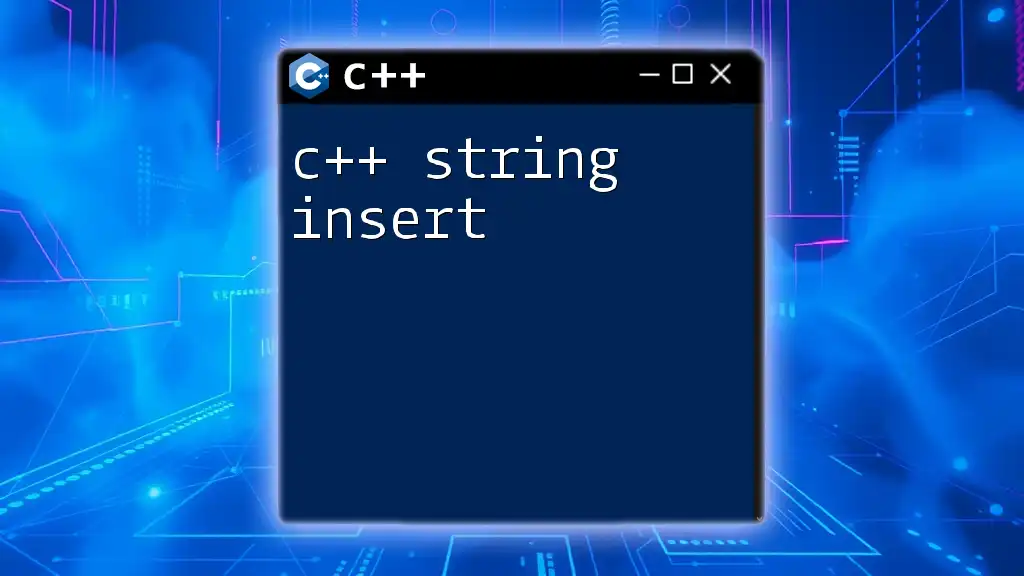
Common Pitfalls in C++ String Initialization
Uninitialized Strings
Using uninitialized strings can lead to undefined behavior, as such strings may access random memory. For instance:
std::string str; // Uninitialized, potential undefined behavior
Operations performed on `str` could result in crashes or garbage values, thus highlighting the necessity of always initializing your strings properly.
Memory Management Issues
Understanding memory management is critical when initializing strings, especially given C++’s manual memory management capabilities. Failing to manage string initialization can lead to memory leaks and other issues.
Choosing the right method for initialization allows the `std::string` class to handle its memory automatically, ensuring that cleanup occurs when strings go out of scope.
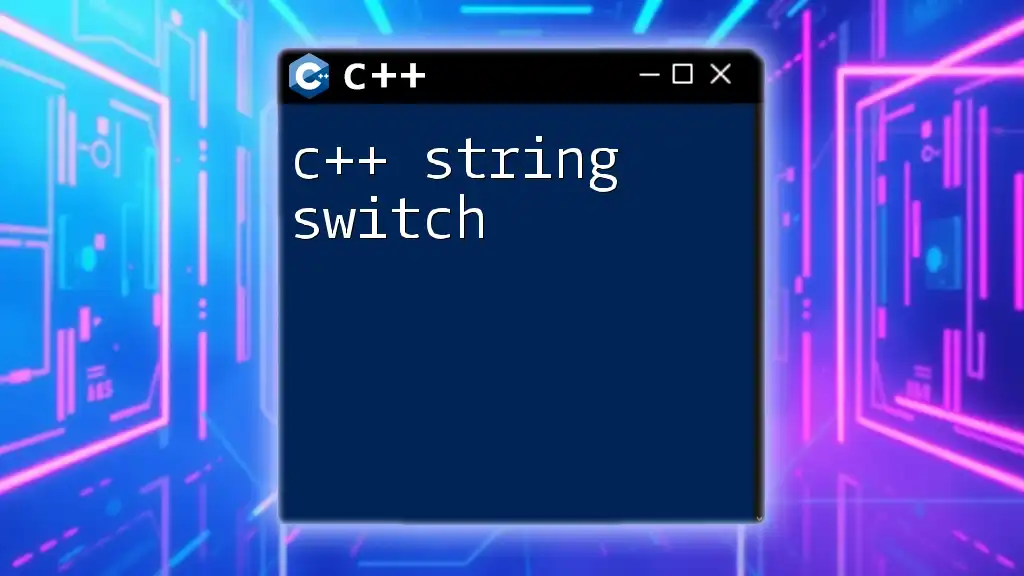
Best Practices for String Initialization in C++
To ensure safe and efficient string initialization:
- Always prefer using `std::string` over C-style strings where possible.
- Initialize strings explicitly, even if it means using an empty string.
- Familiarize yourself with the various constructors of `std::string` to utilize its full potential.
- Avoid using uninitialized strings to prevent unpredictable behavior in your programs.
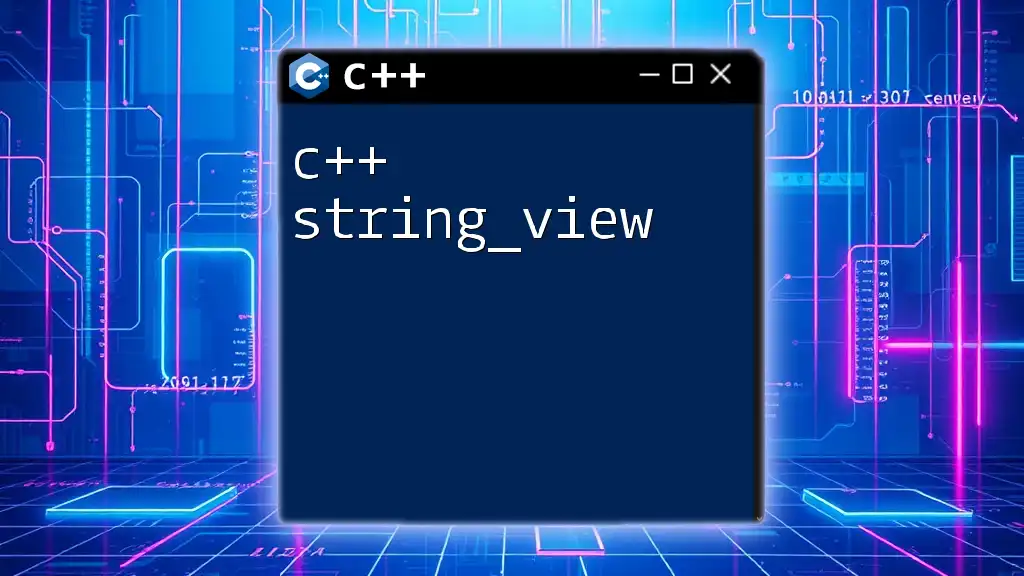
Conclusion
Understanding the methods of C++ string init is fundamental to effective string manipulation in C++. By mastering string initialization, you can enhance your programming efficiency and reliability. Regular practice and exploration of C++ strings will empower you as a developer, ensuring you harness the full capabilities of this versatile language.
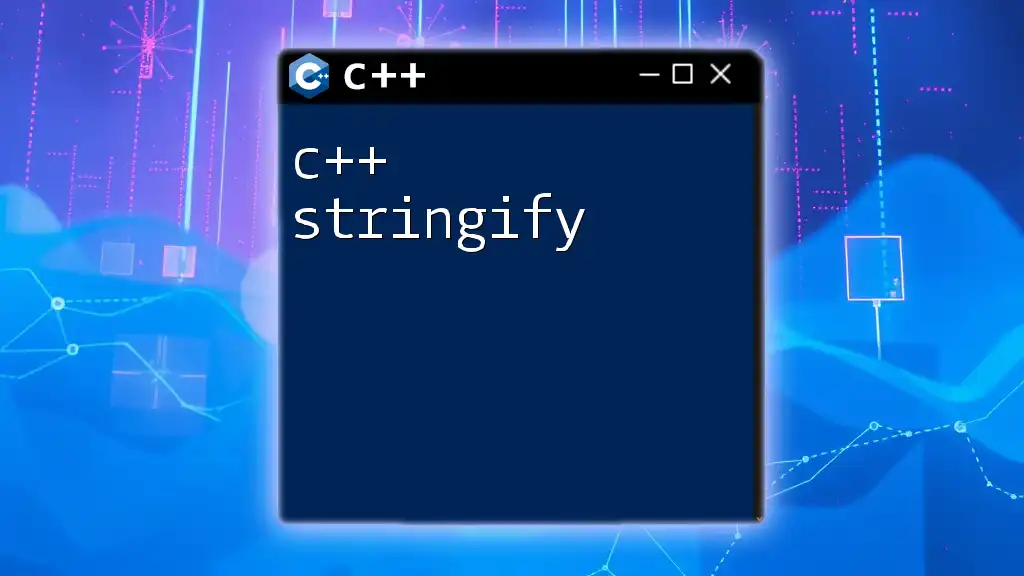
Further Reading & Resources
For further insights on string manipulation in C++, consult additional resources such as official documentation, forums, and coding tutorials. Expanding your knowledge base on `std::string` will pave the way for deeper mastery over text handling in C++.
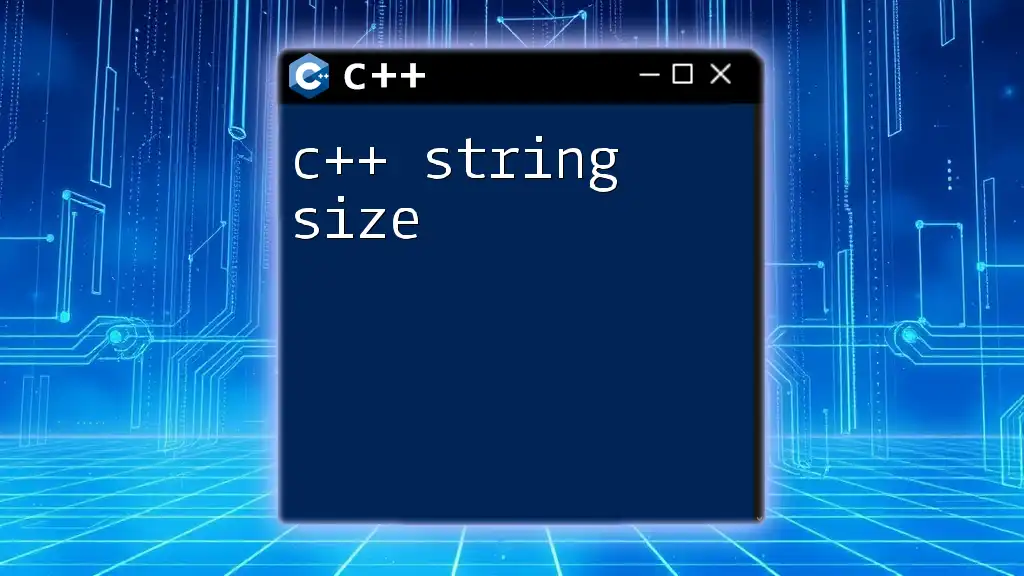
Call to Action
Stay tuned for more concise C++ tutorials and guides from our company, designed to help you navigate the complexities of programming with ease.