In C++, you can join strings using the `std::ostringstream` class or the `std::string::append()` method to concatenate multiple strings into a single string.
Here's a simple example using `std::ostringstream`:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::ostringstream oss;
oss << "Hello, " << "World" << "!";
std::string joinedString = oss.str();
std::cout << joinedString << std::endl; // Output: Hello, World!
return 0;
}
What is C++?
C++ is a powerful general-purpose programming language that emphasizes performance, efficiency, and flexibility. It supports both procedural and object-oriented programming paradigms and has a rich standard library for managing strings and other fundamental data types. Understanding how to manipulate strings effectively is crucial, as strings are one of the most commonly used data structures in programming, especially for applications like data presentation and formatted outputs.
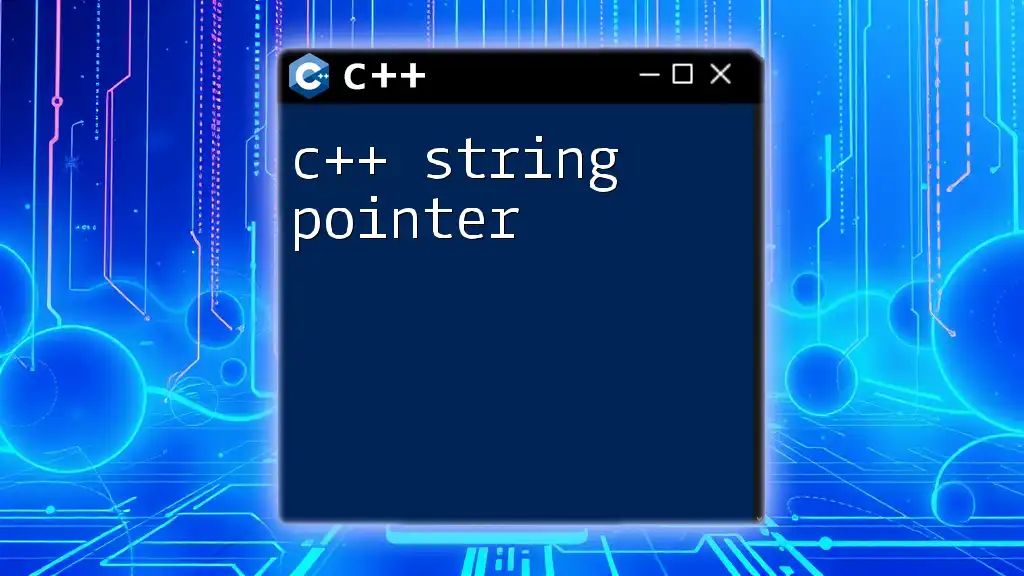
The Concept of Joining Strings
In programming, joining means combining multiple strings into a single string using a specified delimiter. This concept is essential when you want to present textual data in a cohesive manner, such as outputting a list of items or creating a formatted message.
Difference Between Concatenation and Joining
While concatenation typically involves simply appending strings together (e.g., using the `+` operator), joining emphasizes the use of a delimiter between the strings. For instance, concatenating "Hello" and "World" yields "HelloWorld," while joining them with a space results in "Hello World." This subtlety becomes crucial when dealing with lists or arrays of strings.
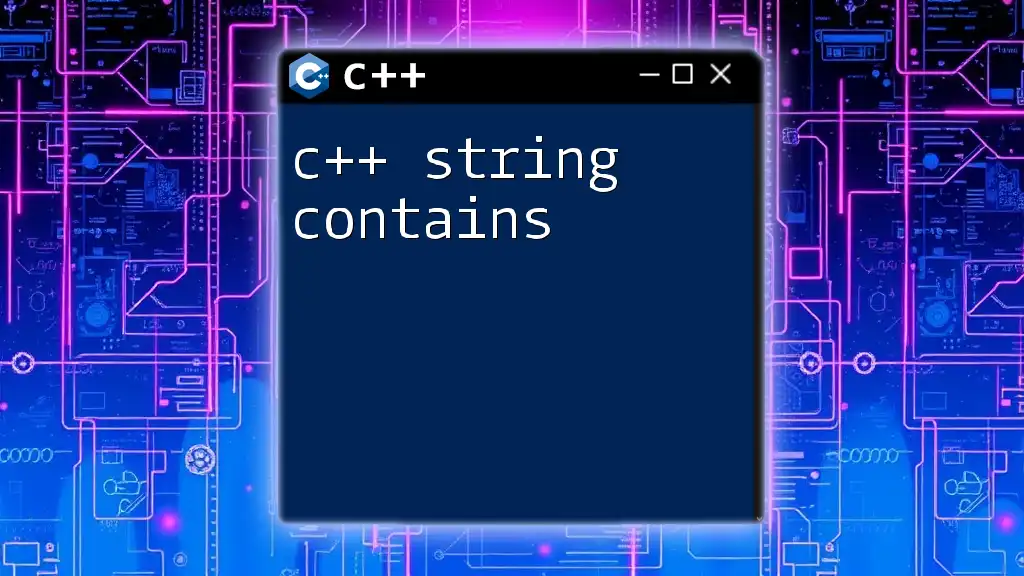
C++ String Join: Basic Concepts
To effectively perform string joining in C++, you'll often utilize the std::string class, which is part of the C++ Standard Library. The versatility of this class allows you to perform various string operations, including joining strings in multiple ways.
Common Joining Techniques
There are several methods to join strings in C++. While manual concatenation is straightforward, leveraging utility functions often proves more efficient and readable. Below are some popular techniques.
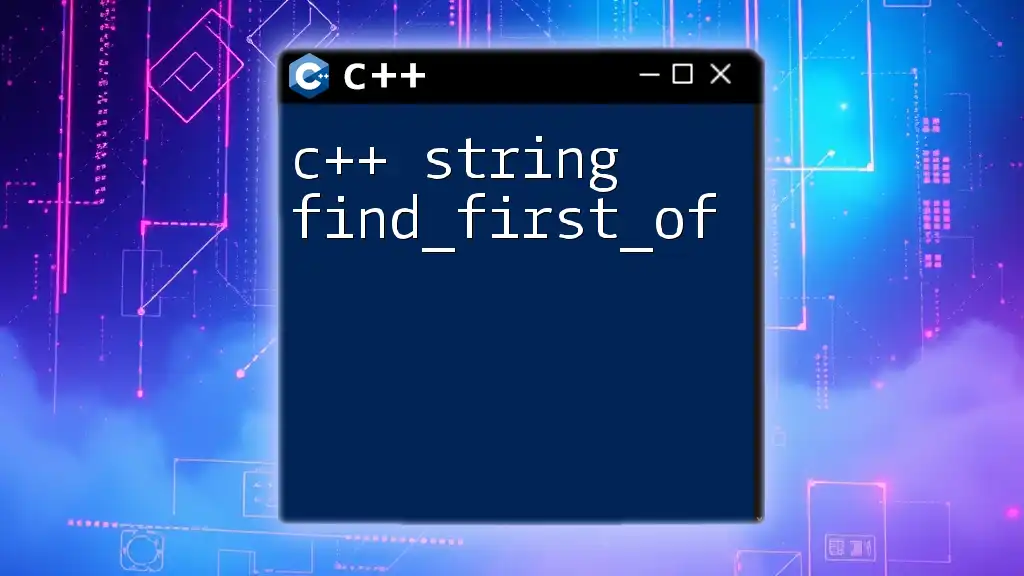
Using std::ostringstream for String Joining
One effective method of joining strings involves using std::ostringstream. This class provides an interface for writing to a string stream, making it a convenient way to build strings piece by piece.
Example: Joining Strings Using ostringstream
#include <iostream>
#include <sstream>
#include <vector>
std::string join(const std::vector<std::string>& strings, const std::string& delimiter) {
std::ostringstream os;
for (size_t i = 0; i < strings.size(); ++i) {
os << strings[i];
if (i != strings.size() - 1) os << delimiter;
}
return os.str();
}
int main() {
std::vector<std::string> words = {"Hello", "world", "C++"};
std::string joined = join(words, " ");
std::cout << joined << std::endl;
return 0;
}
Explanation of Code Functionality: The function `join` takes a vector of strings and a delimiter. It uses an `ostringstream` to concatenate the strings while inserting the delimiter between them. This method is efficient and maintains clean code, as it avoids manual string manipulations.
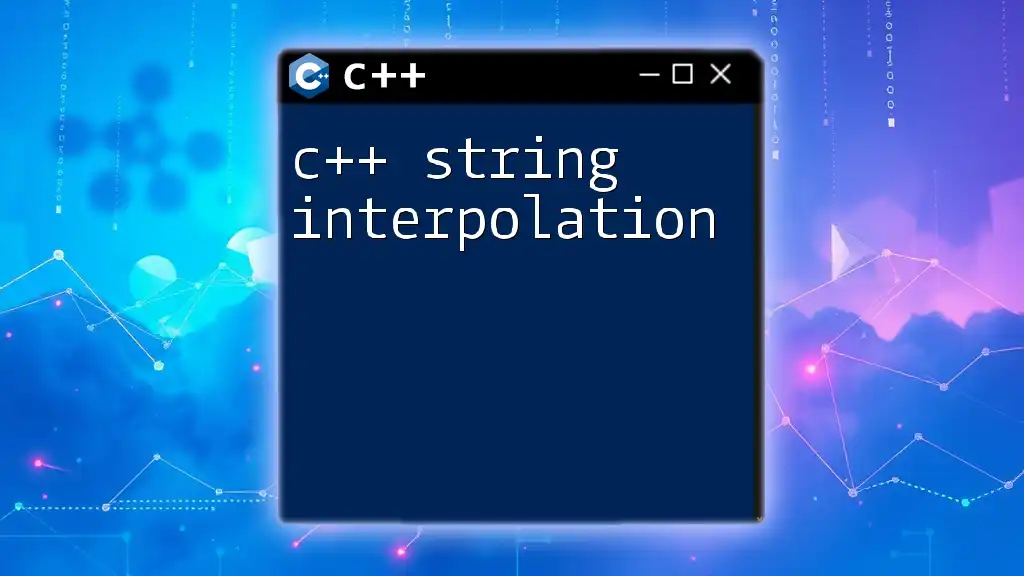
Using std::accumulate for String Joining
C++ offers powerful algorithms in the Standard Library, including std::accumulate. This function can also be used to join strings by iterating over a range and combining them with a specified operation.
Example: Joining Strings Using Accumulate
#include <iostream>
#include <numeric>
#include <vector>
std::string join(const std::vector<std::string>& strings, const std::string& delimiter) {
return std::accumulate(std::next(strings.begin()), strings.end(), strings[0],
[&delimiter](std::string a, std::string b) { return std::move(a) + delimiter + b; });
}
int main() {
std::vector<std::string> words = {"Hello", "world", "C++"};
std::string joined = join(words, " ");
std::cout << joined << std::endl;
return 0;
}
Explanation of Code Functionality: Here, the `join` function uses `std::accumulate` to accumulate the strings while inserting the delimiter. The use of `std::next` allows the first string to be handled separately to avoid placing a delimiter before it.
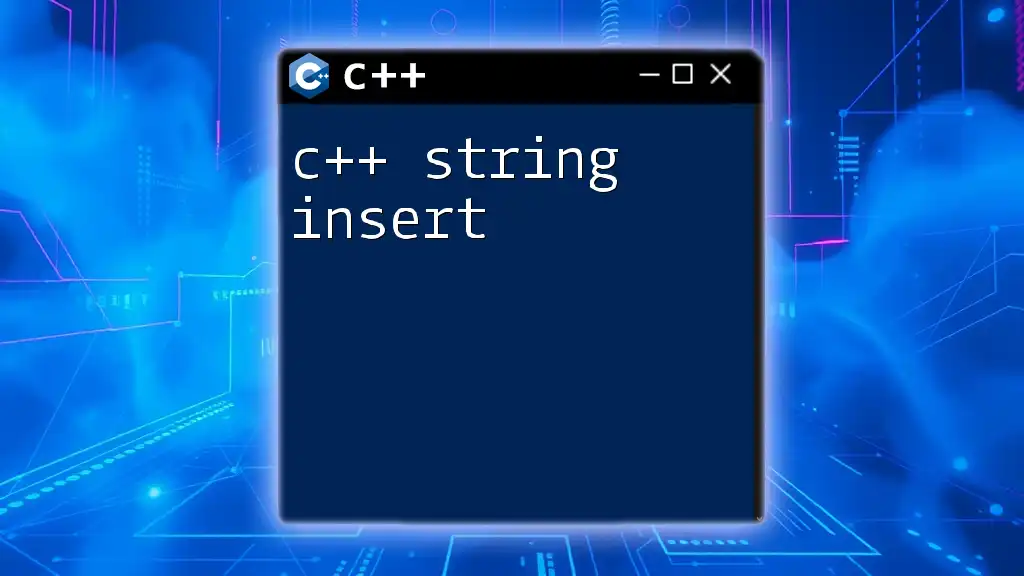
Using std::string Join Function in C++17
With the introduction of C++17, string handling received significant improvements. C++17 enables developers to create more robust and efficient string management functions.
Example: Using String Join with C++17
#include <iostream>
#include <string>
#include <vector>
std::string join(const std::vector<std::string>& strings, const std::string& delimiter) {
if (strings.empty()) return "";
std::string result = strings[0];
for (size_t i = 1; i < strings.size(); ++i) {
result += delimiter + strings[i];
}
return result;
}
int main() {
std::vector<std::string> words = {"C++", "makes", "string", "joining", "easier!"};
std::string joined = join(words, " ");
std::cout << joined << std::endl;
return 0;
}
Explanation of Code Functionality: This example uses a simple loop to iterate through the vector of strings. It initializes the result with the first string and appends each subsequent string with the specified delimiter. This straightforward method is clear and performs well for moderate-sized datasets.
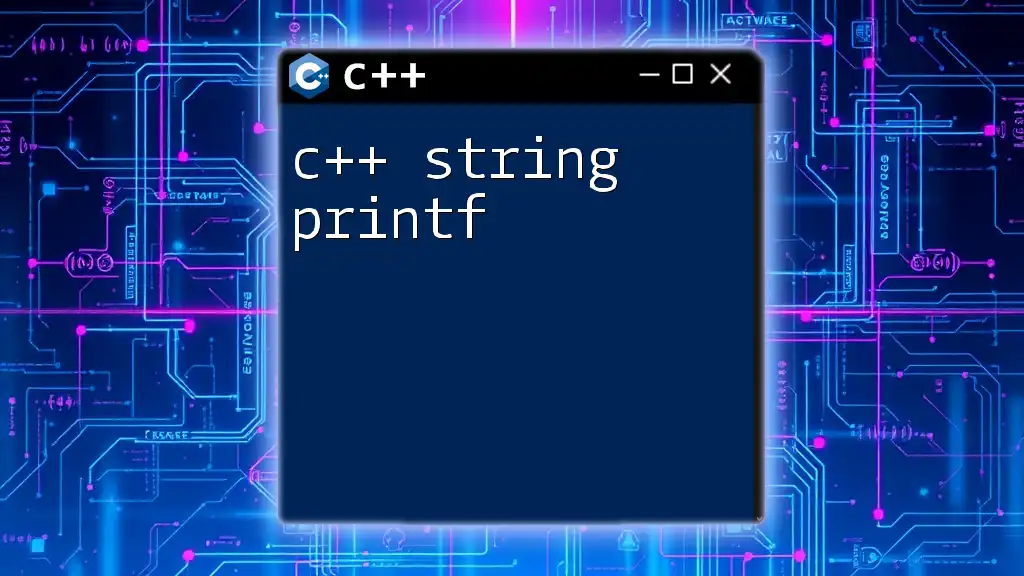
Considerations and Best Practices
When joining strings in C++, it is essential to consider performance, especially for large datasets. Using std::ostringstream or std::accumulate can offer better performance than repeated string concatenation using the `+` operator due to how memory is managed.
Choosing the Right Method for Your Use Case
Each joining method has its strengths:
- std::ostringstream is great for readability and simplicity.
- std::accumulate is suitable for functional programming paradigms.
- The loop method in C++17 is clear and performs adequately for smaller vectors.
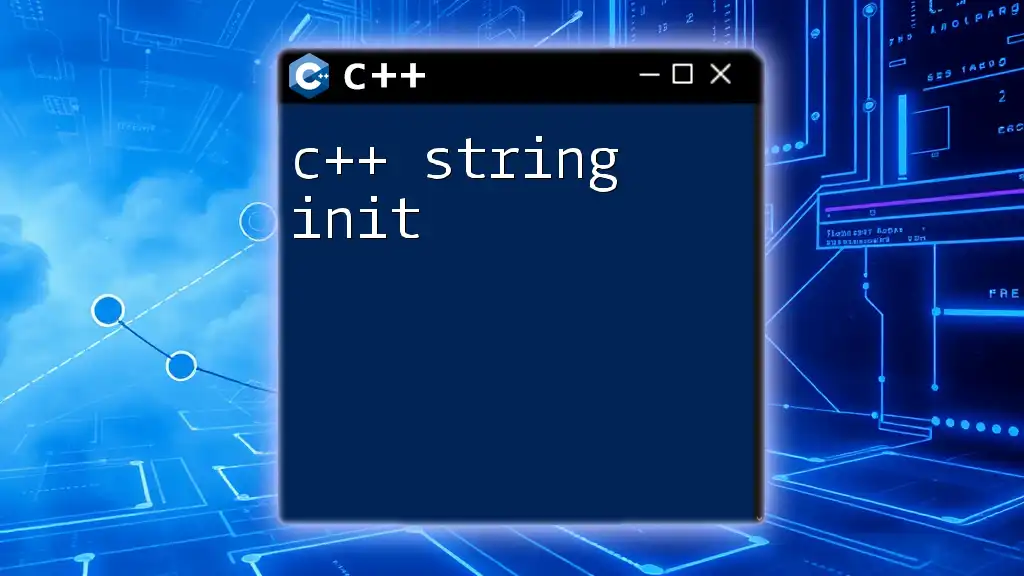
Common Errors and Debugging Tips
When joining strings, common errors include:
- Incorrectly handling delimiters, causing unwanted leading or trailing characters.
- Forgetting to check for empty vectors, which can lead to access violations.
To debug:
- Ensure adequate checks for empty strings or vectors before processing.
- Use print statements to verify intermediate results.
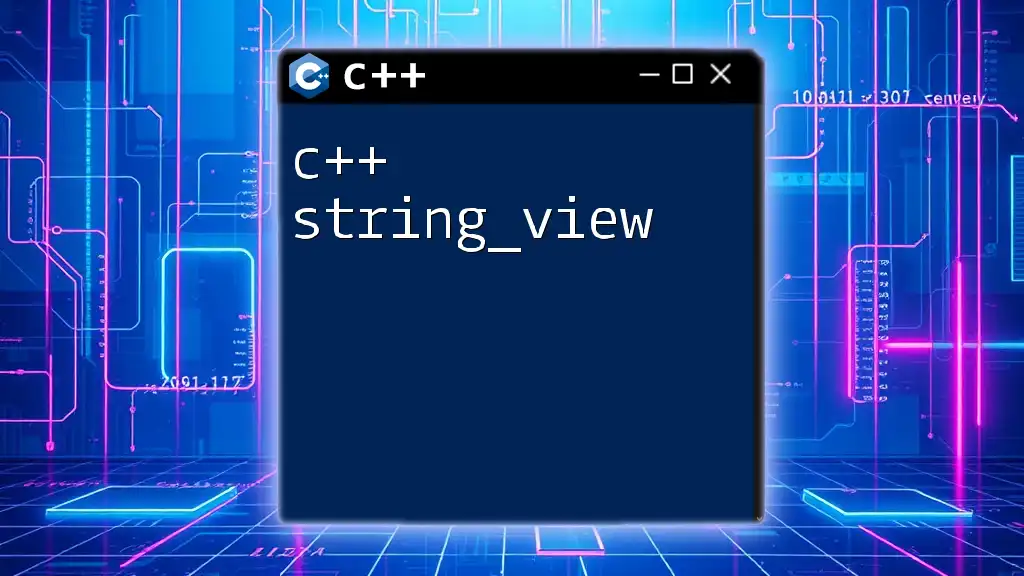
Conclusion
Mastering the concept of c++ string join is vital for effective string manipulation in C++. By exploring various methods, such as std::ostringstream, std::accumulate, and leveraging C++17 features, you can choose the best approach for your specific needs. Whether you're presenting data or producing formatted output, the techniques discussed will empower you to handle strings concisely and efficiently.
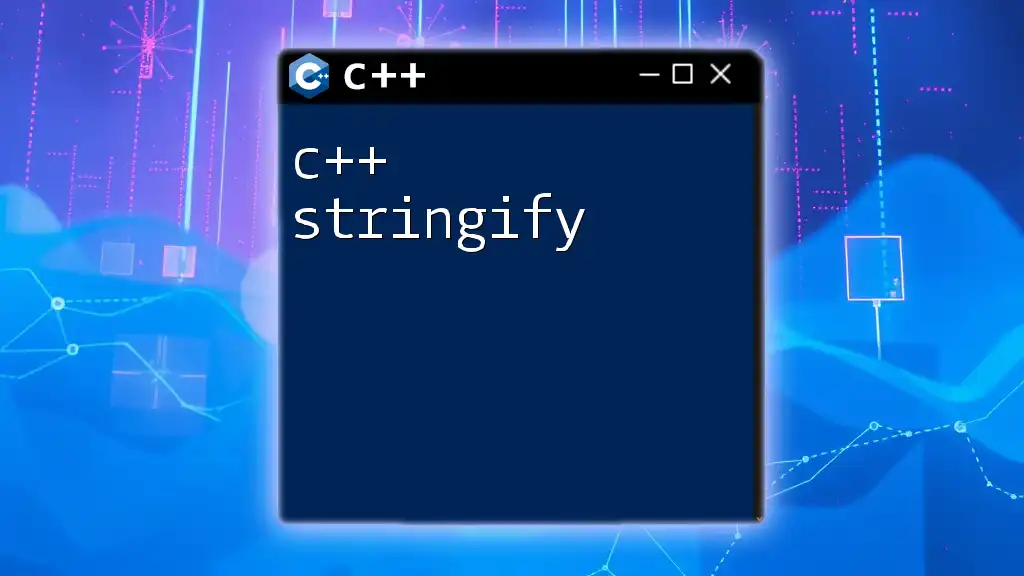
Further Reading and Resources
For those eager to explore string handling further, consider diving into additional C++ resources, checking out official documentation, and experimenting with various string manipulation libraries to enhance your skills.