The `std::string::at()` function in C++ provides a safe way to access a specific character in a string by index, throwing an exception if the index is out of bounds.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char ch = str.at(7); // Accessing the character at index 7
std::cout << ch; // Output: W
return 0;
}
Understanding C++ Strings
What is a C++ String?
In C++, a string is an object of the `std::string` class, which is part of the C++ Standard Library. Unlike C-style strings, which are simply arrays of characters terminated by a null character (`'\0'`), `std::string` is a more robust and flexible representation of textual data. It handles memory management automatically and provides various member functions for string manipulation, making it easier to work with strings in a more intuitive way.
Why Use `std::string`?
Using `std::string` comes with several advantages, including:
- Memory Management: Automatic memory allocation and deallocation help avoid memory leaks and buffer overflows common with C-style strings.
- Ease of Use: Functions available with `std::string` offer numerous methods for string manipulation, making it easier to perform operations without the need for manual memory management.
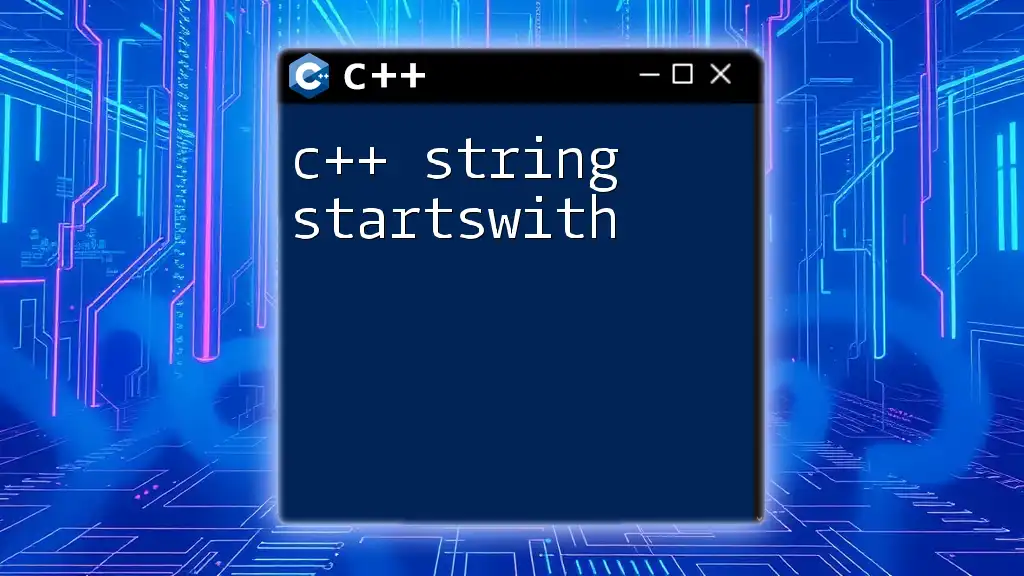
The `at()` Function in C++
What is `string::at`?
The `string::at` function is a member function of the `std::string` class that provides a safe way to access characters in a string. Unlike the array subscript operator `operator[]`, `at()` performs bounds checking, ensuring your program does not access characters outside the valid range of the string.
Syntax of `at()` Function
The basic syntax for using the `at()` function is as follows:
char& at(size_t pos);
- pos: The position of the character you want to access, which should be a valid index within the string.
- The function returns a reference to the character at the specified position.
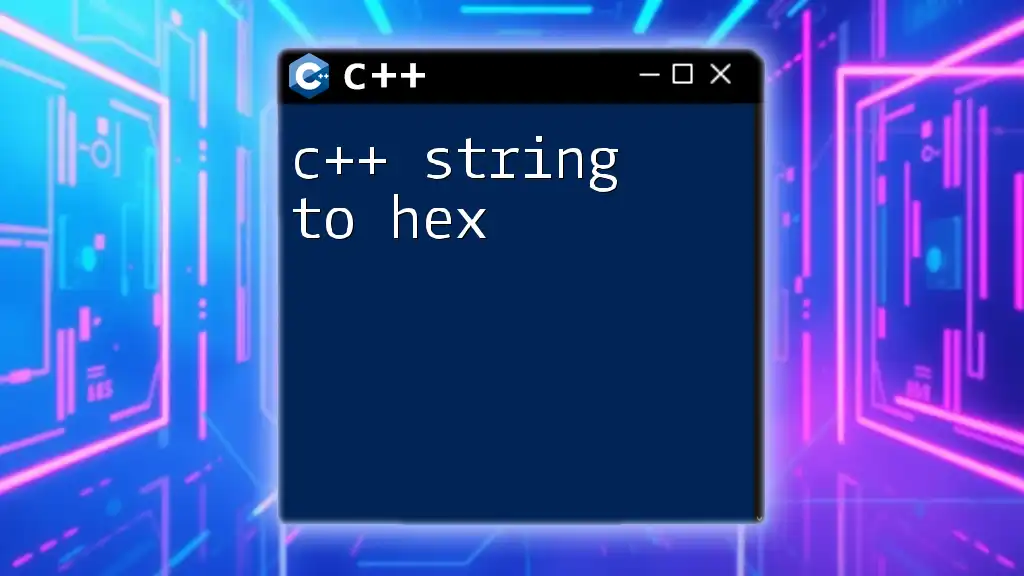
Error Handling with `at()`
Exceptions Thrown by `at()`
One of the significant benefits of using `string::at` is its built-in error handling. If you try to access an index that is out of range—meaning it exceeds the length of the string—`at()` will throw a `std::out_of_range` exception. This feature is crucial for developing robust C++ applications where string manipulations can lead to runtime errors.
Example of Error Handling
Here's an example demonstrating how to handle exceptions thrown by `at()` when an invalid index is accessed:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World";
try {
char c = str.at(20); // Attempting out-of-range access
} catch (const std::out_of_range& e) {
std::cout << "Error: " << e.what() << std::endl; // Handle the exception
}
return 0;
}
In this example, trying to access `str.at(20)` will throw an exception because the index exceeds the string's length. The program gracefully handles this error and prints an informative message.
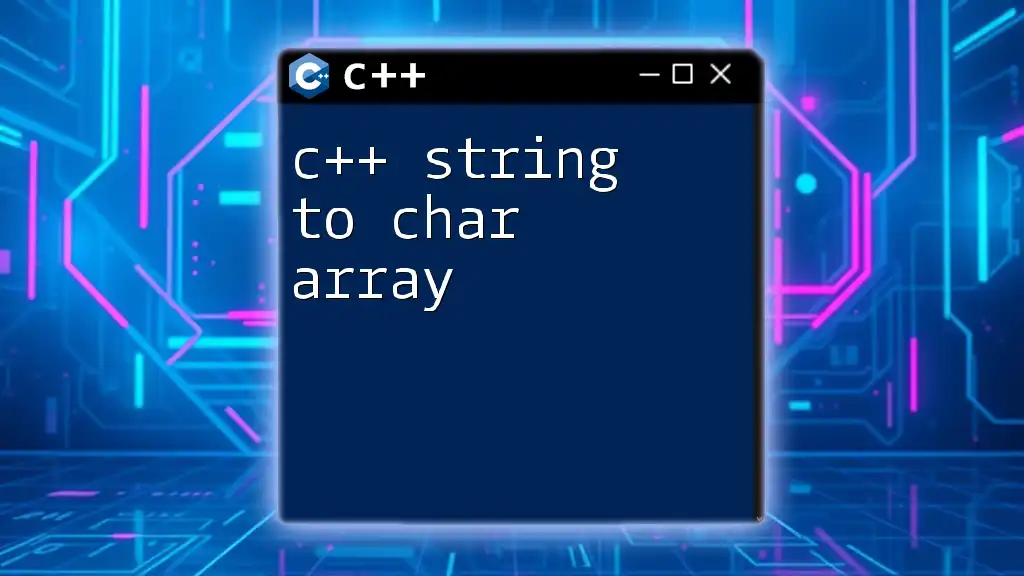
Practical Use Cases of `string::at`
Accessing Characters in a String
Using `at()` allows you to safely access individual characters in a string, like so:
std::string text = "C++ Programming";
char firstChar = text.at(0); // Access the first character
In this example, `firstChar` will hold the value `'C'`. Using `at()` ensures that you do not accidentally exceed the bounds of your string.
Modifying Characters in a String
Another impressive feature of `at()` is that you can also modify characters at specific indexes. Here's how you can change a character:
std::string text = "C++ Programming";
text.at(4) = 'p'; // Modifies 'P' to 'p'
By using `at()` for modification, you ensure that you are working within the safe bounds of the string. This enhances code safety, making it easier to prevent unauthorized access.
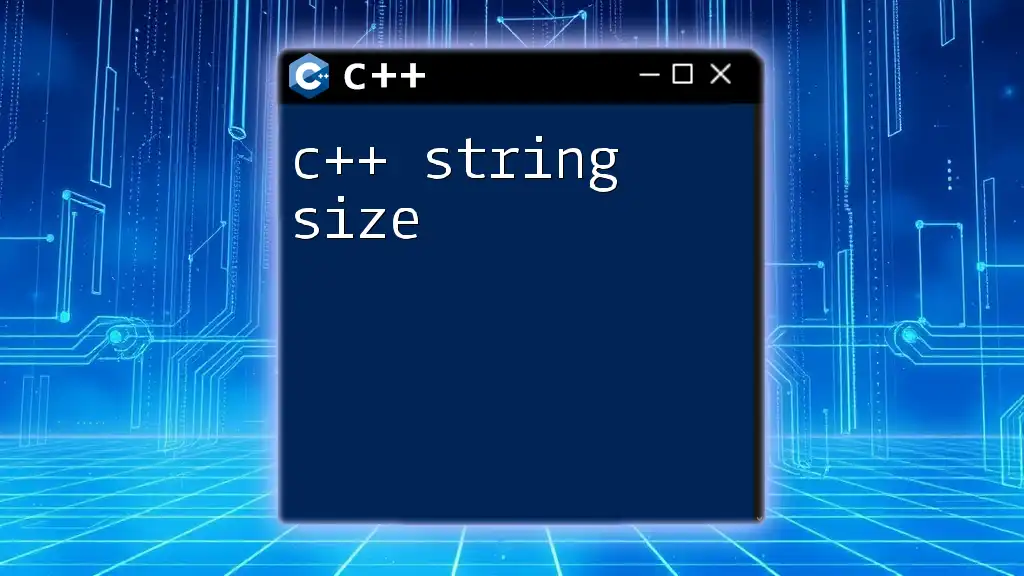
Comparing `at()` with `operator[]`
When to Use `at()` vs. `operator[]`
While both `at()` and `operator[]` serve the purpose of accessing characters in a string, they are not interchangeable.
- Safety: `at()` performs bounds checking and throws an exception if the index is invalid, while `operator[]` does not perform any checks and may lead to undefined behavior if accessed out of range.
- Convenience: `operator[]` can be slightly faster due to the lack of checks, but it sacrifices safety.
Here’s a quick comparison:
std::string text = "Example";
char a = text[2]; // Accessing with operator[]
char b = text.at(2); // Safer access method
The choice between using `at()` and `operator[]` boils down to risk vs. efficiency. For code intended for production, using `at()` is generally the recommended practice due to its built-in safety features.
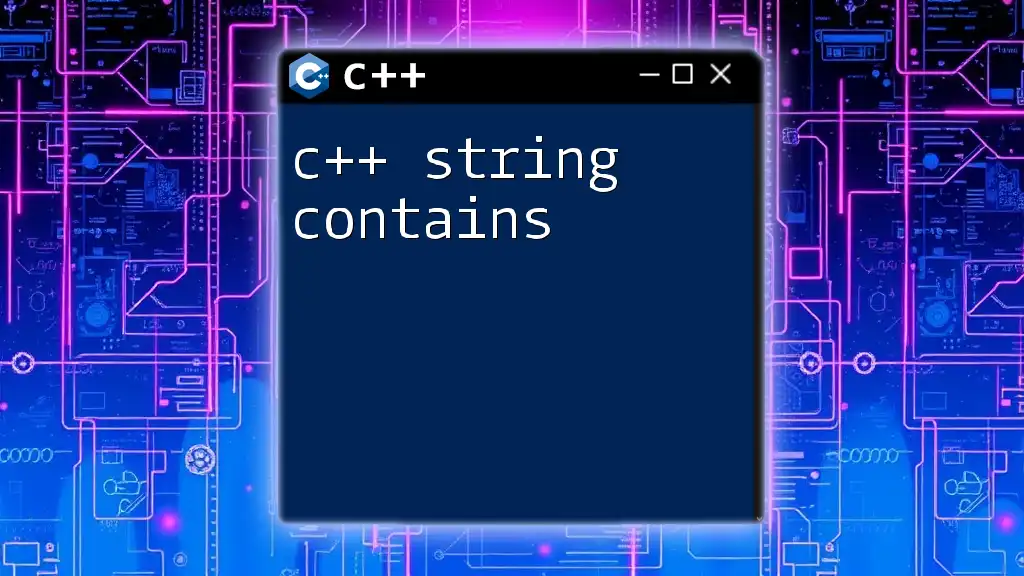
Performance Considerations
Efficiency of Using `at()`
Though `at()` incurs a slight performance overhead because of its bounds checking, this trade-off is often worth it when considering the safety it provides. In most applications, this additional cost is negligible compared to the benefits gained in robustness and error handling.
However, in performance-critical situations, developers might opt to use `operator[]`, but only after ensuring that they have adequate safety checks in place.
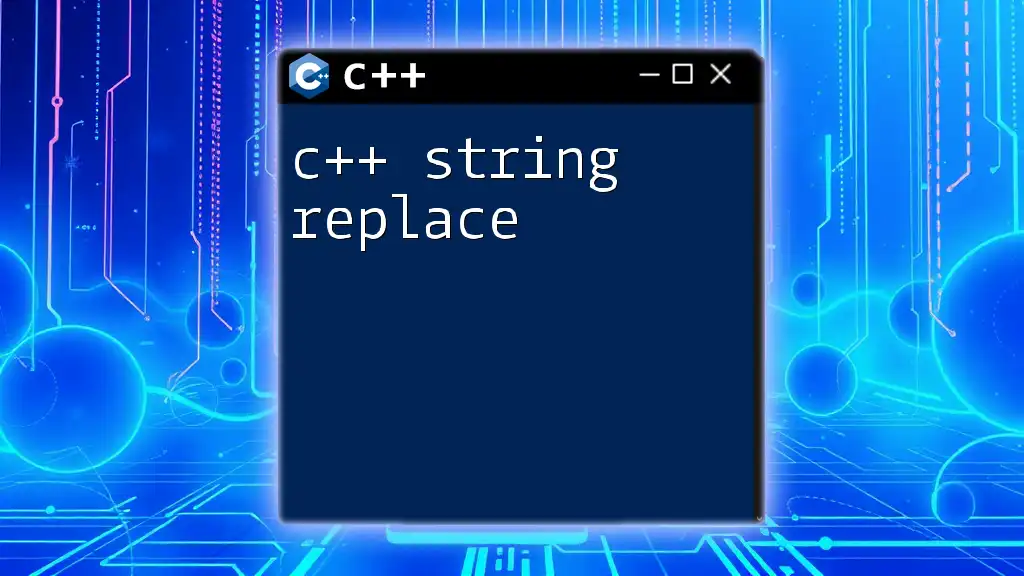
Conclusion
The `c++ string at` function is a powerful tool for accessing and modifying characters in a string while providing essential safety features. By using `at()`, you can write cleaner and safer code, preventing common runtime errors that arise from out-of-bounds access. As you practice using `string::at`, you'll appreciate the balance it establishes between ease of use and safety in C++ string manipulation.
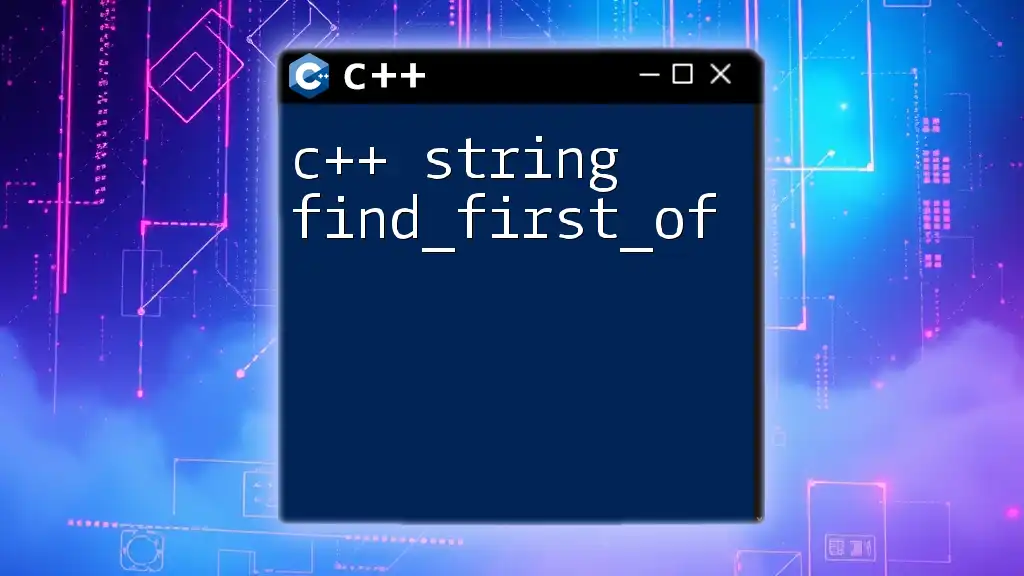
Additional Resources
For further exploration, you may find these resources helpful:
- C++ documentation on `std::string`
- Detailed articles on C++ error handling
- Exercises focused on string manipulation techniques
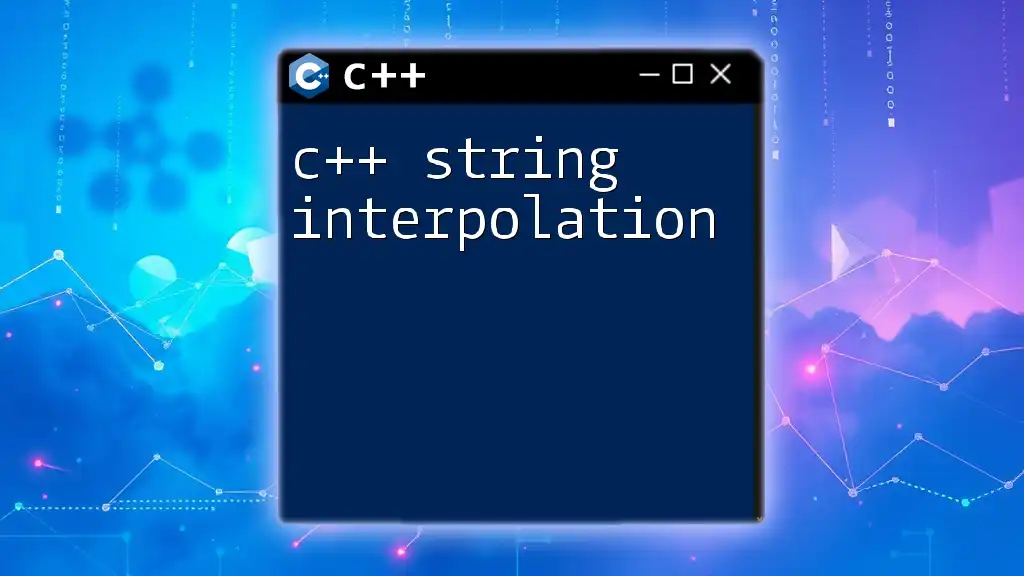
FAQs
Can I use `at()` with C-style strings?
No, `at()` is a member of the `std::string` class in C++ and cannot be directly used with C-style strings (character arrays).
What happens if I pass a negative index to `at()`?
Passing a negative index to `at()` results in undefined behavior, as the function expects a non-negative index value.
Is `string::at` available in older versions of C++?
Yes, the `at()` function has been a part of the `std::string` class since the C++98 standard, making it widely available across different versions of C++.