The `c_str()` function in C++ is used to obtain a pointer to a null-terminated character array (C-style string) that represents the contents of a `std::string` object.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, C++!";
const char* cStr = str.c_str();
std::cout << cStr << std::endl; // Output: Hello, C++!
return 0;
}
Understanding C++ Strings
In C++, strings can be managed using the `std::string` class, which provides a convenient way to handle text. The `std::string` class offers functionality such as dynamic resizing, ease of use, and built-in memory management. This contrasts sharply with C-style strings, represented as character arrays (`char[]`), which require manual memory handling and are prone to errors such as buffer overflows.
The Need for c_str()
While `std::string` provides numerous advantages, there exist scenarios requiring C-style strings. This necessity often arises when interfacing with C libraries that expect `const char*` as a parameter. Understanding how to convert a `std::string` to a C-style string using `c_str()` is vital for seamless interoperability between C and C++ code.
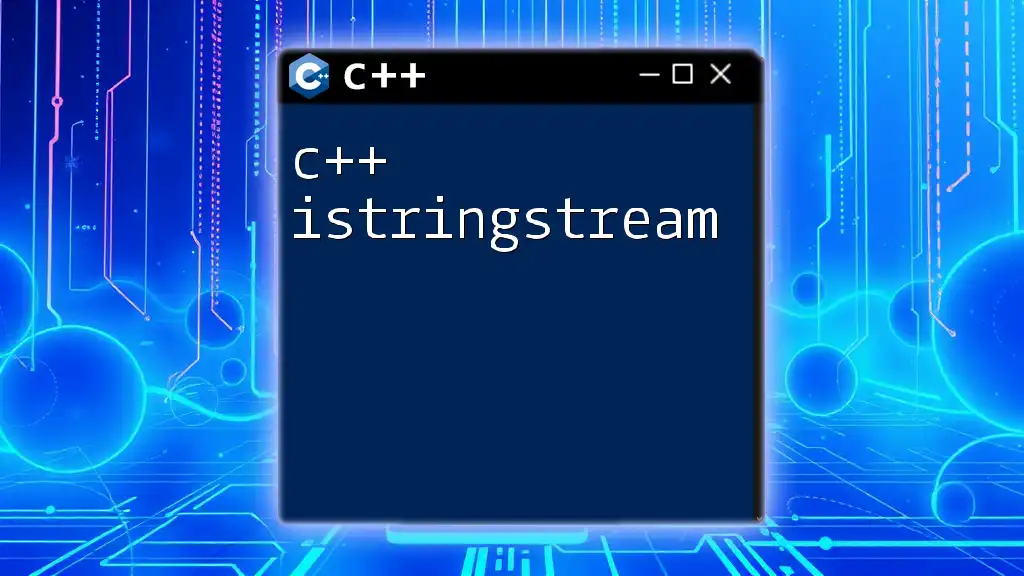
What is c_str()?
The `c_str()` member function is a critical interface of the `std::string` class. It returns a pointer to a null-terminated character array representation of the string’s data.
The syntax for the `c_str()` method is:
const char* c_str() const noexcept;
This method provides a `const char*` pointer, allowing you to work directly with C-style strings.
How c_str() Works
When you call `c_str()` on a `std::string` object, it returns a pointer to an internal buffer containing the string's characters, followed by a null terminator (`'\0'`). The pointer is valid as long as the `std::string` object remains unchanged and is in scope.
Example:
#include <iostream>
#include <string>
int main() {
std::string cppString = "Hello, world!";
const char* cStyleString = cppString.c_str();
std::cout << "C-style string: " << cStyleString << std::endl;
return 0;
}
In this example, we define a `std::string` and convert it to a C-style string for output. The `c_str()` function allows easy access to the inner data without manual conversion.
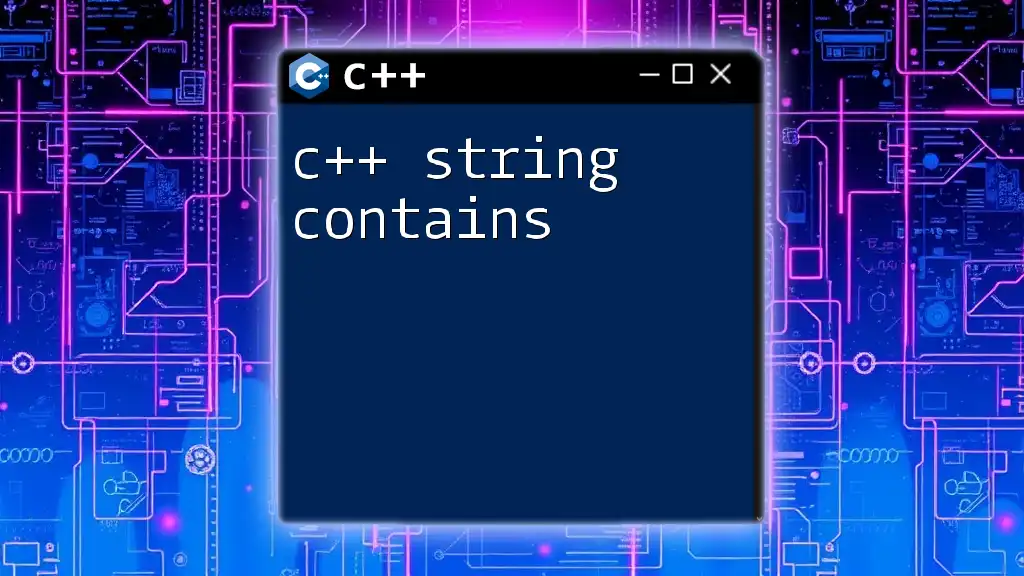
Using c_str() in C++
Interfacing with C Functions
The primary use of `c_str()` comes into play when requiring compatibility with C functions. Many C standard library functions, such as string manipulation functions, take `const char*` parameters.
Example of Passing c_str() to C Functions:
extern "C" void cFunction(const char* str);
int main() {
std::string cppString = "Calling C function!";
cFunction(cppString.c_str());
return 0;
}
In this example, we define an external C function called `cFunction`. By passing the result of `cppString.c_str()` to it, we bridge the gap between C++ and C.
Modifying the String
A crucial point to note is that the pointer returned by `c_str()` should never be modified. Doing so can lead to undefined behavior, as the internal buffer is managed by the `std::string` class.
Example Demonstrating This Rule:
std::string cppString = "Hello, world!";
const char* cStyleString = cppString.c_str();
// This code will give a compilation error
cStyleString[0] = 'h'; // Error: Assignment to read-only location
In this example, attempting to directly modify the value of `cStyleString` will lead to a compilation error. This reinforces the idea that `c_str()` provides a read-only access to the underlying character array.
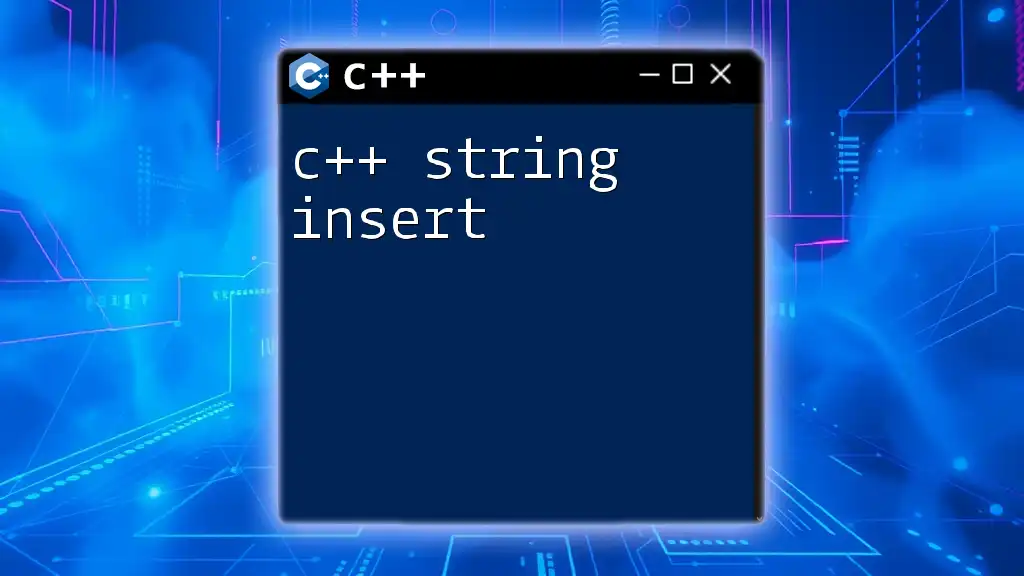
Advantages and Limitations of c_str()
Understanding the advantages and limitations of `c_str()` is essential for effective programming.
Advantages:
- The primary advantage of using `c_str()` is easy access to C-style strings.
- It simplifies the process of passing C++ strings to legacy C libraries, enhancing code compatibility and maintainability.
Limitations:
- A significant limitation is the temporary nature of the pointer returned. The pointer remains valid only while the `std::string` object remains unchanged. Changes to the `std::string`, including resizing, may invalidate the pointer, leading to potential segmentation faults or undefined behavior.
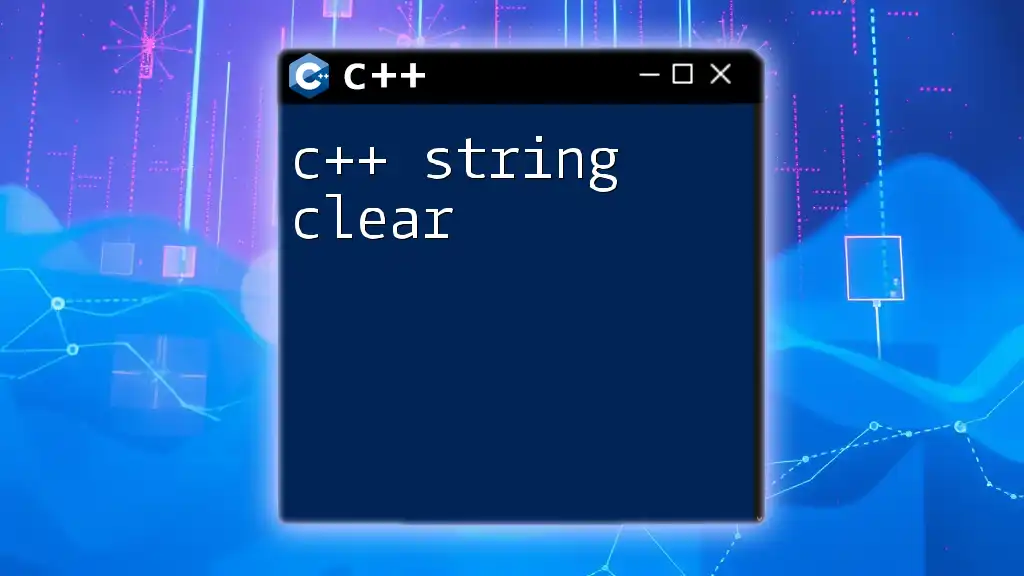
Common Mistakes with c_str()
When using `c_str()`, several common pitfalls may occur that developers should be aware of:
-
Dereferencing an Invalidated Pointer: If you attempt to use a pointer returned by `c_str()` after the `std::string` has been modified, you'll encounter undefined behavior.
-
Forgetting to Null-Terminate When Necessary: While `c_str()` ensures that the string is null-terminated, when manually converting strings to C-style format, remember to include the null terminator to avoid overrunning buffer memory.
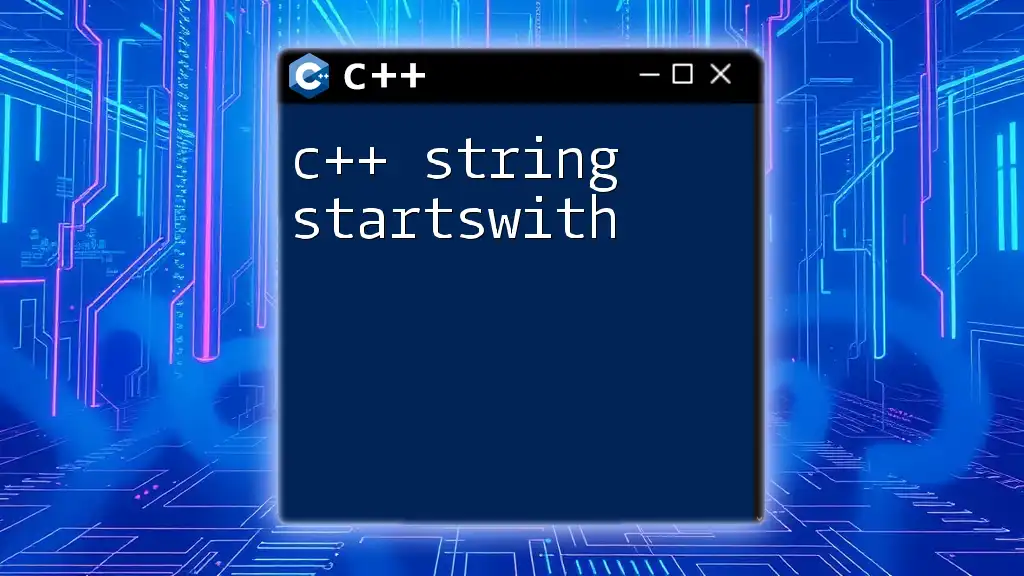
Best Practices for Using c_str()
To safely and effectively use `c_str()`, consider the following best practices:
-
Prefer `std::string` over C-style strings for as long as possible within your C++ programs to leverage automatic memory management and improved safety.
-
Always ensure that the `std::string` object remains unchanged while its `c_str()` pointer is in use.
-
If you need long-term storage of C-style strings, make a copy of the data returned from `c_str()` into a separate `char` array or a dynamically allocated string.
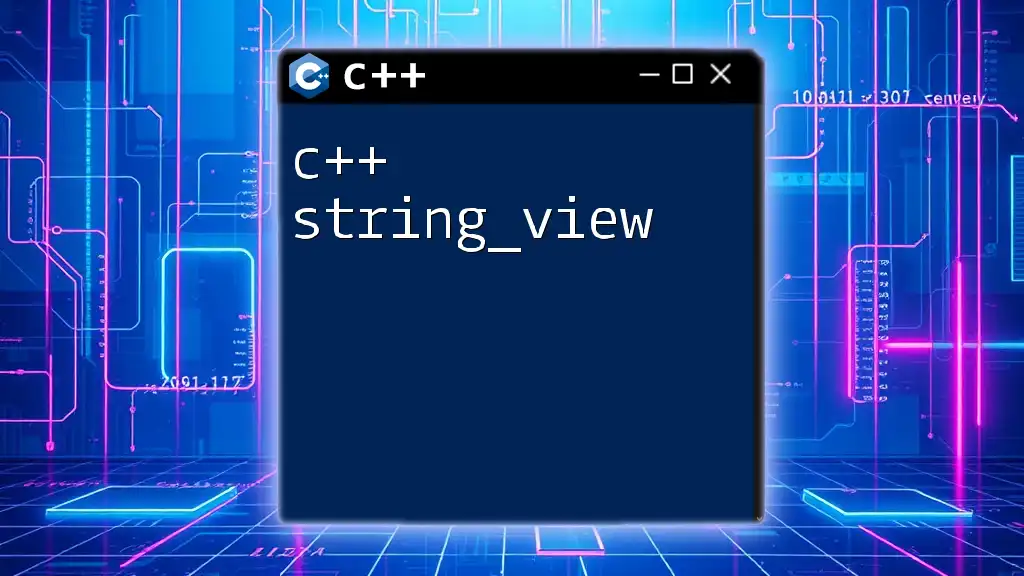
Conclusion
The `c_str()` function in C++ serves as a bridge between the C-style string and C++ string handling methodologies. Mastering its use is an invaluable asset for effective C/C++ programming. Understanding the nuances of `c_str()`, including its advantages and limitations, will ensure that you can effectively navigate the complexities of string handling while maintaining safe and efficient code practices.
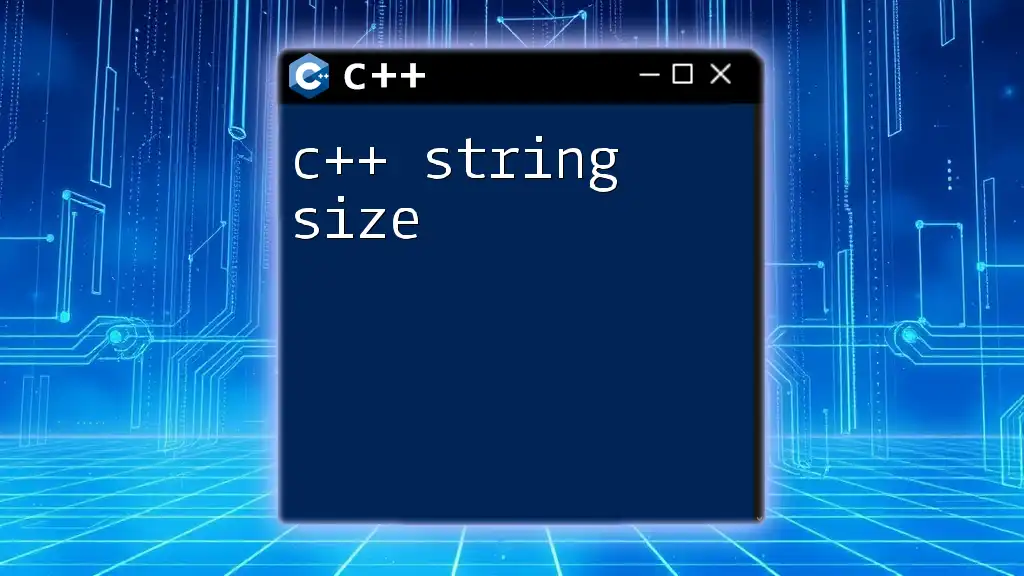
Additional Resources
For further reading and study, consider the following resources:
- Recommended books on advanced C++ concepts.
- Online tutorials and courses that cover C++ string manipulation and memory management in depth.
- Official C++ documentation for `std::string` and related functionalities.
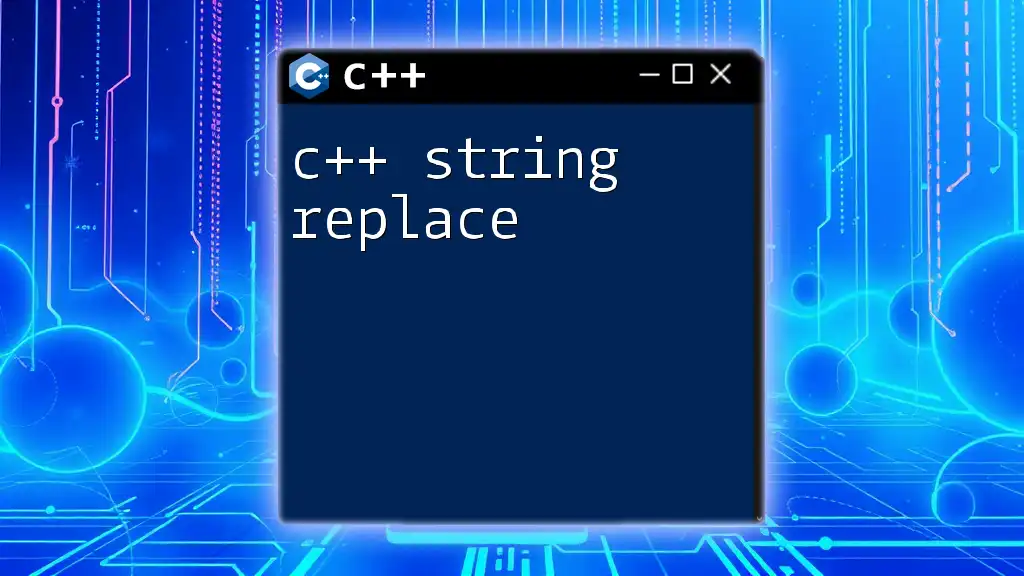
Call to Action
Have you had experience using `c_str()` in your C++ projects? Share your stories or questions in the comments below! Moreover, don't forget to subscribe for more quick and handy C++ programming tips and insights that can enhance your coding journey.